Android ToggleButton Tutorial with Examples
1. Android ToggleButton
In Android, ToggleButton is a user interface control with the two ON/OFF states. The ToggleButton is a subclass of Button, so it can also display Icons and text.
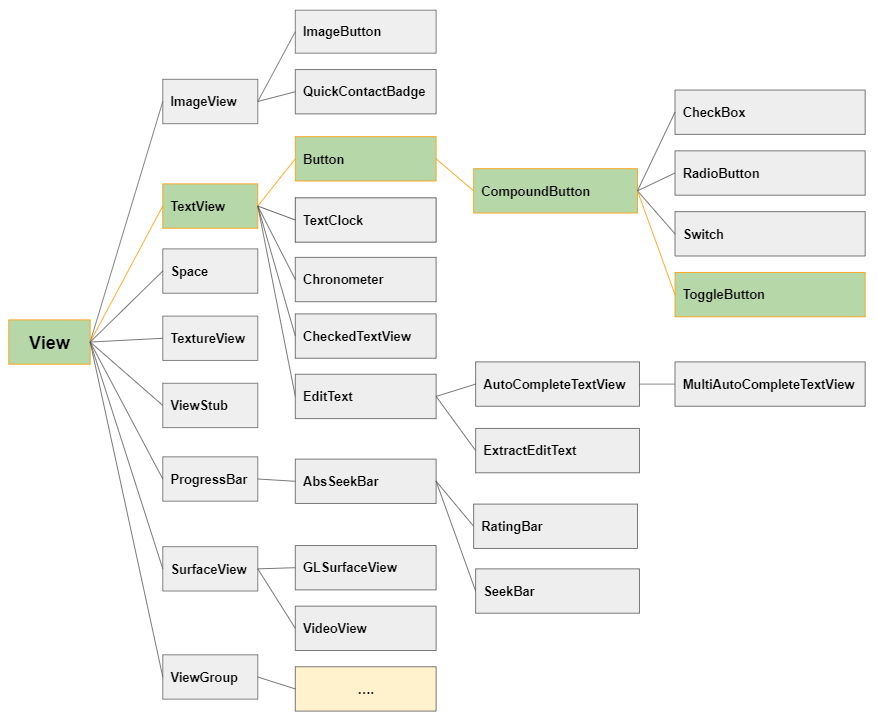
ToggleButton is quite similar to CheckBox and Switch in terms of features and usage. All three classes are subclasses of CompoundButton,and thedifference lies in their interface.
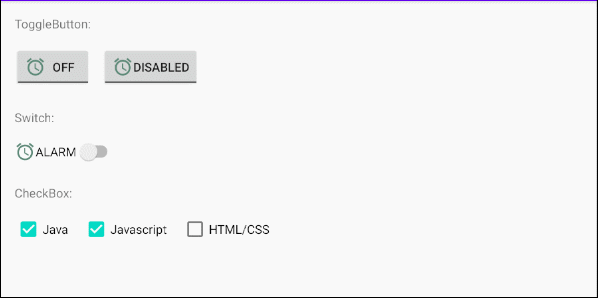
Text, Text On, Text Off
By default the ToggleButton will display the text, "OFF", when it is OFF, and display the text, "ON", when it is ON. The android:text attribute will not work. Instead, you can use 2 other attributes, android:textOff and android:textOn to set the text for ToggleButton in different states.
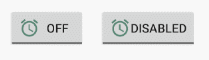
<!-- Default ON/OFF Text -->
<ToggleButton
android:id="@+id/toggleButton"
android:drawableLeft="@drawable/icon_alarm"
android:text="ToggleButton"
... />
<!-- Custom ON/OFF Text -->
<ToggleButton
android:id="@+id/toggleButton2"
android:drawableLeft="@drawable/icon_alarm"
android:text="ToggleButton"
android:textOff="DISABLED"
android:textOn="ENABLED"
... />
Image (Icon)
As mentioned above, ToggleButton is a subclass of Button, so it allows you to display four icons maximum near the four edges by using the attributes of android:drawableLeft, android:drawableTop, android:drawableRight, android:drawableBottom, android:drawableStart, android:drawableEnd.
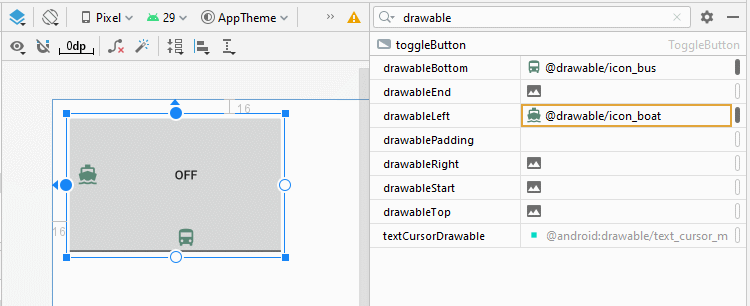
<ToggleButton
android:id="@+id/toggleButton"
android:drawableLeft="@drawable/icon_boat"
android:drawableTop="@drawable/icon_car"
android:drawableRight="@drawable/icon_bus"
android:drawableBottom="@drawable/icon_bus"
... />
toggle()
All four classes of ToggleButton, CheckBox, RadioButton and Switch are subclasses of CompoundButton, so they inherit the toggle() method. This is the commonly used method to switch between two states, from ON (Checked) to OFF (Unchecked) and vice versa.
CompoundButton button = (ToggleButton) findViewById(R.id.toggleButton);
button.toggle();
2. ToggleButton Styles
The style attribute is an option of ToggleButton, which allows you to set the style for the ToggleButton. There are several styles available in Android library that may be ready to use.
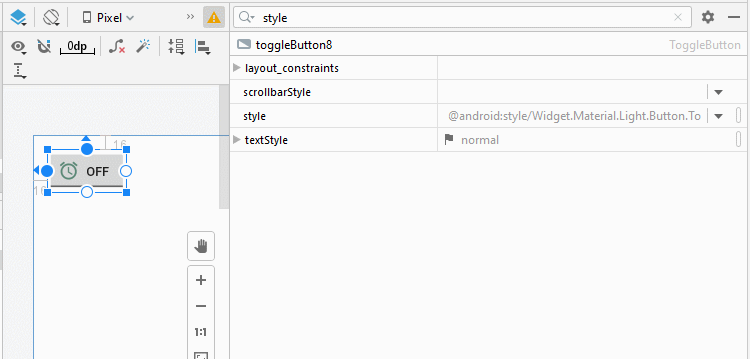
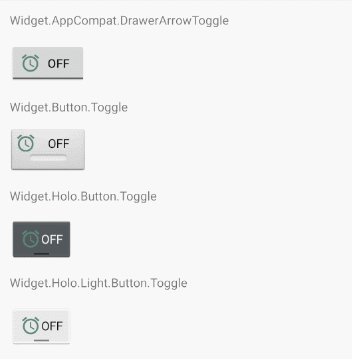
ToggleButton Styles Example
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView24"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Widget.AppCompat.DrawerArrowToggle"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<ToggleButton
android:id="@+id/toggleButton23"
style="@style/Widget.AppCompat.DrawerArrowToggle"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:drawableLeft="@drawable/icon_alarm"
android:text="ToggleButton"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView24" />
<TextView
android:id="@+id/textView25"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Widget.Button.Toggle"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/toggleButton23" />
<ToggleButton
android:id="@+id/toggleButton24"
style="@android:style/Widget.Button.Toggle"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:drawableLeft="@drawable/icon_alarm"
android:text="ToggleButton"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView25" />
<TextView
android:id="@+id/textView26"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Widget.Holo.Button.Toggle"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/toggleButton24" />
<ToggleButton
android:id="@+id/toggleButton25"
style="@android:style/Widget.Holo.Button.Toggle"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:drawableLeft="@drawable/icon_alarm"
android:text="ToggleButton"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView26" />
<TextView
android:id="@+id/textView27"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Widget.Holo.Light.Button.Toggle"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/toggleButton25" />
<ToggleButton
android:id="@+id/toggleButton26"
style="@android:style/Widget.Holo.Light.Button.Toggle"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:drawableLeft="@drawable/icon_alarm"
android:text="ToggleButton"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView27" />
</androidx.constraintlayout.widget.ConstraintLayout>
3. ToggleButton Events
There are quite a few events related to a ToggleButton; however, the following two events are most frequently used:
- setOnClickListener(View.OnClickListener)
- setOnCheckedChangeListener(CompoundButton.OnCheckedChangeListener)
On Click Event:
The event happens when the user clicks the ToggleButton. It is the same as the action of the user clicking a Button.
ToggleButton toggleButton = (ToggleButton) findViewById(R.id.toggleButton);
toggleButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
boolean checked = ((ToggleButton) v).isChecked();
if (checked){
// Your code
}
else{
// Your code
}
}
});
On Checked Change Event:
The event happens when the ToggleButton changes its state due to the user's action or the effect of calling the toggleButton.setChecked(newState) method, etc.
ToggleButton toggleButton = (ToggleButton) findViewById(R.id.toggleButton);
toggleButton.setOnCheckedChangeListener(new CompoundButton.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(CompoundButton buttonView, boolean isChecked) {
if(isChecked) {
// Your code
} else {
// Your code
}
}
});
Android Programming Tutorials
- Configure Android Emulator in Android Studio
- Android ToggleButton Tutorial with Examples
- Create a simple File Finder Dialog in Android
- Android TimePickerDialog Tutorial with Examples
- Android DatePickerDialog Tutorial with Examples
- What is needed to get started with Android?
- Install Android Studio on Windows
- Install Intel® HAXM for Android Studio
- Android AsyncTask Tutorial with Examples
- Android AsyncTaskLoader Tutorial with Examples
- Android Tutorial for Beginners - Basic examples
- How to know the phone number of Android Emulator and change it
- Android TextInputLayout Tutorial with Examples
- Android CardView Tutorial with Examples
- Android ViewPager2 Tutorial with Examples
- Get Phone Number in Android using TelephonyManager
- Android Phone Call Tutorial with Examples
- Android Wifi Scanning Tutorial with Examples
- Android 2D Game Tutorial for Beginners
- Android DialogFragment Tutorial with Examples
- Android CharacterPickerDialog Tutorial with Examples
- Android Tutorial for Beginners - Hello Android
- Using Android Device File Explorer
- Enable USB Debugging on Android Device
- Android UI Layouts Tutorial with Examples
- Android SMS Tutorial with Examples
- Android SQLite Database Tutorial with Examples
- Google Maps Android API Tutorial with Examples
- Android Text to Speech Tutorial with Examples
- Android Space Tutorial with Examples
- Android Toast Tutorial with Examples
- Create a custom Android Toast
- Android SnackBar Tutorial with Examples
- Android TextView Tutorial with Examples
- Android TextClock Tutorial with Examples
- Android EditText Tutorial with Examples
- Android TextWatcher Tutorial with Examples
- Format Credit Card Number with Android TextWatcher
- Android Clipboard Tutorial with Examples
- Create a simple File Chooser in Android
- Android AutoCompleteTextView and MultiAutoCompleteTextView Tutorial with Examples
- Android ImageView Tutorial with Examples
- Android ImageSwitcher Tutorial with Examples
- Android ScrollView and HorizontalScrollView Tutorial with Examples
- Android WebView Tutorial with Examples
- Android SeekBar Tutorial with Examples
- Android Dialog Tutorial with Examples
- Android AlertDialog Tutorial with Examples
- Android RatingBar Tutorial with Examples
- Android ProgressBar Tutorial with Examples
- Android Spinner Tutorial with Examples
- Android Button Tutorial with Examples
- Android Switch Tutorial with Examples
- Android ImageButton Tutorial with Examples
- Android FloatingActionButton Tutorial with Examples
- Android CheckBox Tutorial with Examples
- Android RadioGroup and RadioButton Tutorial with Examples
- Android Chip and ChipGroup Tutorial with Examples
- Using image assets and icon assets of Android Studio
- Setting SD Card for Android Emulator
- ChipGroup and Chip Entry Example
- How to add external libraries to Android Project in Android Studio?
- How to disable the permissions already granted to the Android application?
- How to remove applications from Android Emulator?
- Android LinearLayout Tutorial with Examples
- Android TableLayout Tutorial with Examples
- Android FrameLayout Tutorial with Examples
- Android QuickContactBadge Tutorial with Examples
- Android StackView Tutorial with Examples
- Android Camera Tutorial with Examples
- Android MediaPlayer Tutorial with Examples
- Android VideoView Tutorial with Examples
- Playing Sound effects in Android with SoundPool
- Android Networking Tutorial with Examples
- Android JSON Parser Tutorial with Examples
- Android SharedPreferences Tutorial with Examples
- Android Internal Storage Tutorial with Examples
- Android External Storage Tutorial with Examples
- Android Intents Tutorial with Examples
- Example of an explicit Android Intent, calling another Intent
- Example of implicit Android Intent, open a URL, send an email
- Android Services Tutorial with Examples
- Android Notifications Tutorial with Examples
- Android DatePicker Tutorial with Examples
- Android TimePicker Tutorial with Examples
- Android Chronometer Tutorial with Examples
- Android OptionMenu Tutorial with Examples
- Android ContextMenu Tutorial with Examples
- Android PopupMenu Tutorial with Examples
- Android Fragments Tutorial with Examples
- Android ListView Tutorial with Examples
- Android ListView with Checkbox using ArrayAdapter
- Android GridView Tutorial with Examples
Show More