How to add external libraries to Android Project in Android Studio?
1. The ways to use external libraries
You are developing an Android app on Android Studio, sometimes you want to use an external library for your project, such as a jar file.
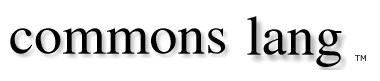
Common langs is an java library with open source code which is provided by the Apache, it has utility methods for working with String, numbers, concurrency ...
Suppose that you want to use this library for your Android project. And using one of its utility methods to test whether a String is a number or not.
import org.apache.commons.lang3.StringUtils;
public class CheckNumeric {
public void test() {
String text1 = "0123a4";
String text2 = "01234";
boolean result1 = StringUtils.isNumeric(text1);
boolean result2 = StringUtils.isNumeric(text2);
System.out.println(text1 + " is a numeric? " + result1);
System.out.println(text2 + " is a numeric? " + result2);
}
}
In this document I will guide you 3 ways to use the external library:
- Add your jar files to libs folder of the project and declare it as a library to use.
- Create a Android module and copy your jar file to this module, and then declare your project using the newly created module.
- Declare and use a remote library.
2. Way 1 - Copy external library to libs folder
Create a project named AddLibsDemo:
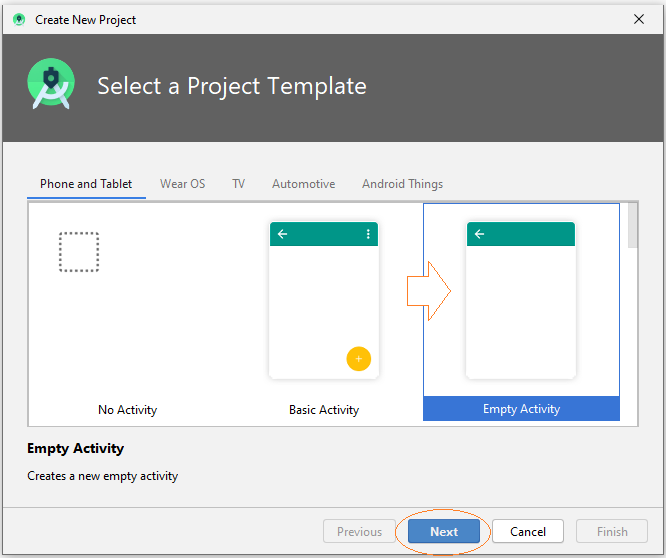
OK, your project has been created.
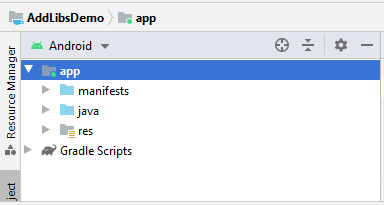
Change to Project tab:
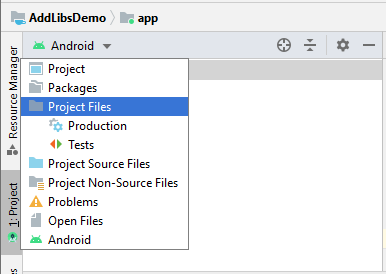
Copy jar file to libs folder:
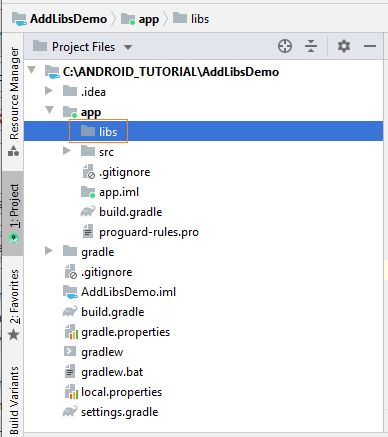
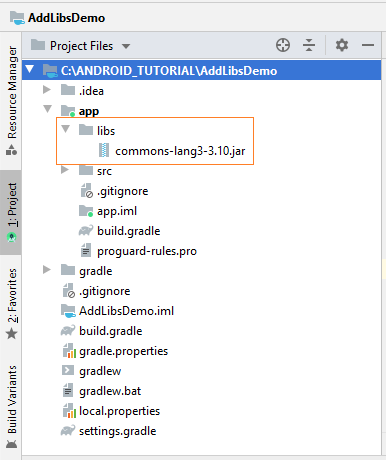
Right click jar files, select "Add as Library..":
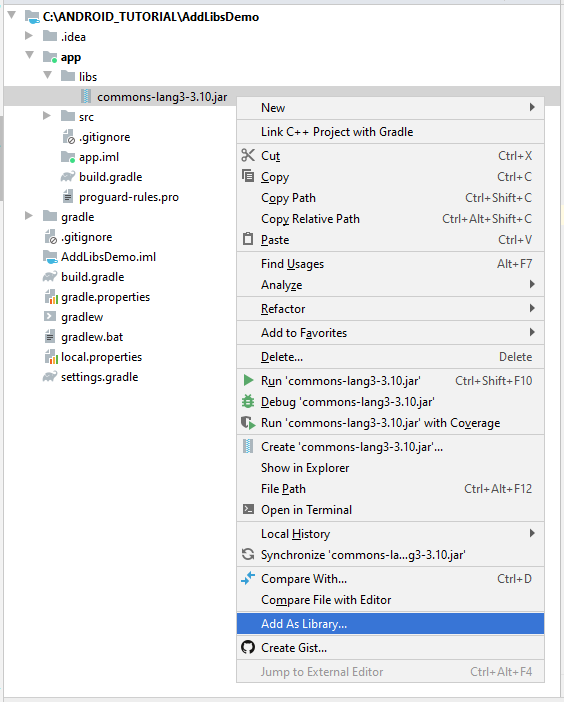
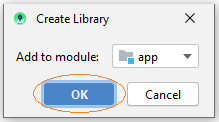
Turn back to "Android" Tab, you can see that your library has been already declared in build.grade (Module: app)
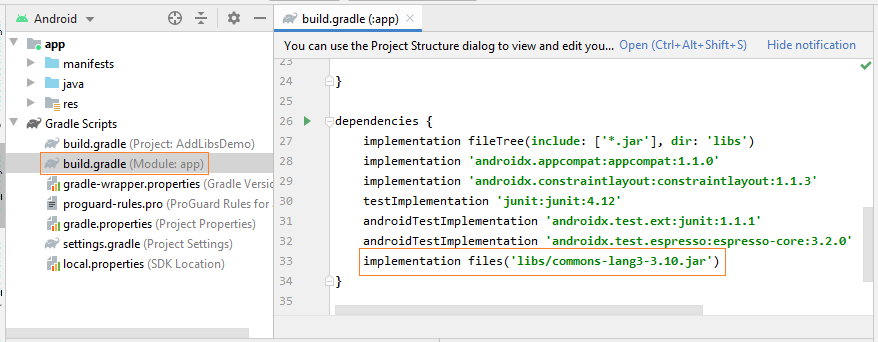
OK, now your library is being already used.
3. Way 2 - Using the module library
Suppose you have a AddLibsDemo2 project, you want to use common-lang3-3.4.jar library for this project.
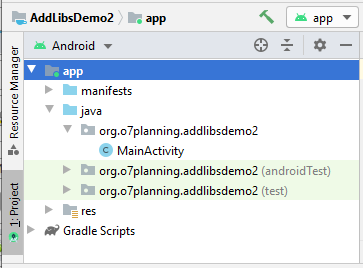
Create a Android Module:
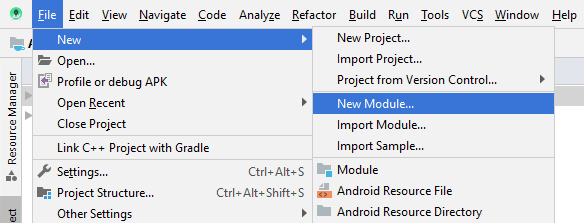
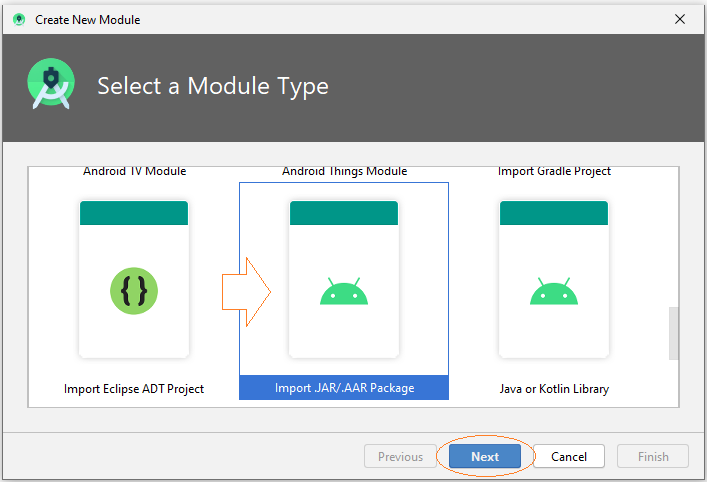
Point to the location of the library.
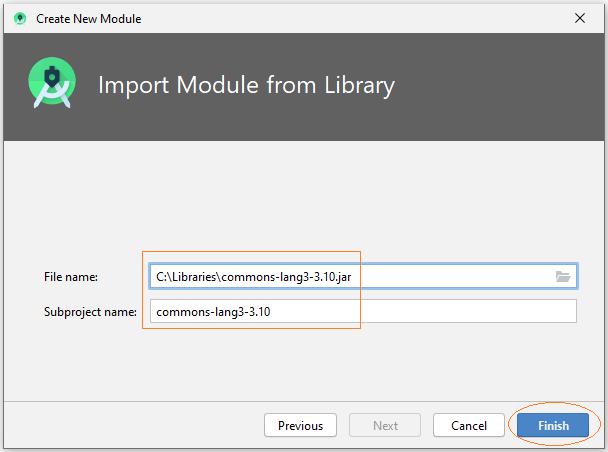
Module library has been created:
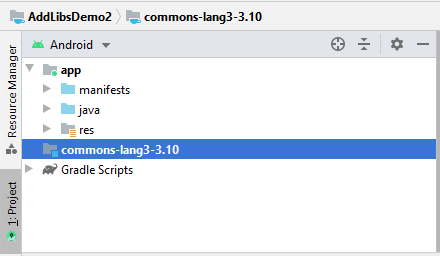
Next, you need to declare the dependency of the main project into the newly created module.
- File/Project Structure
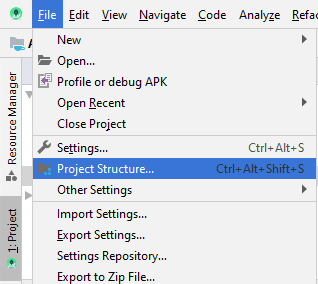
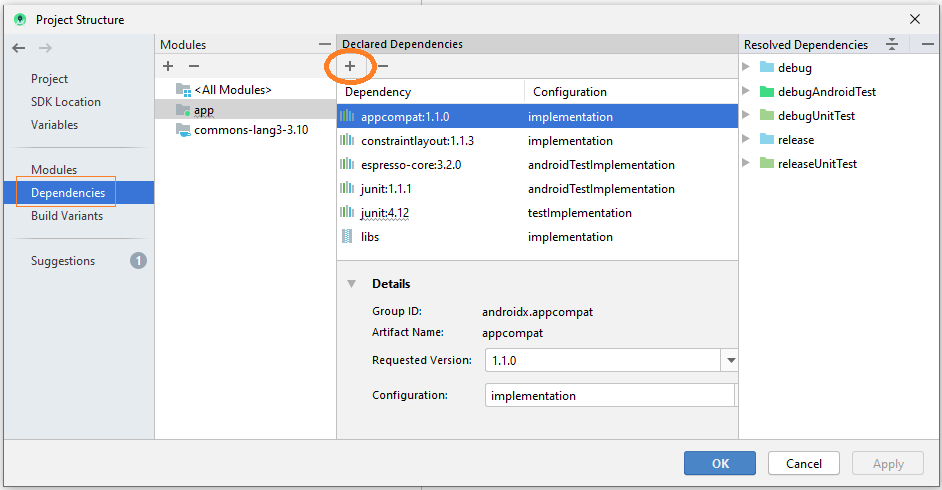
Add module dependencies:
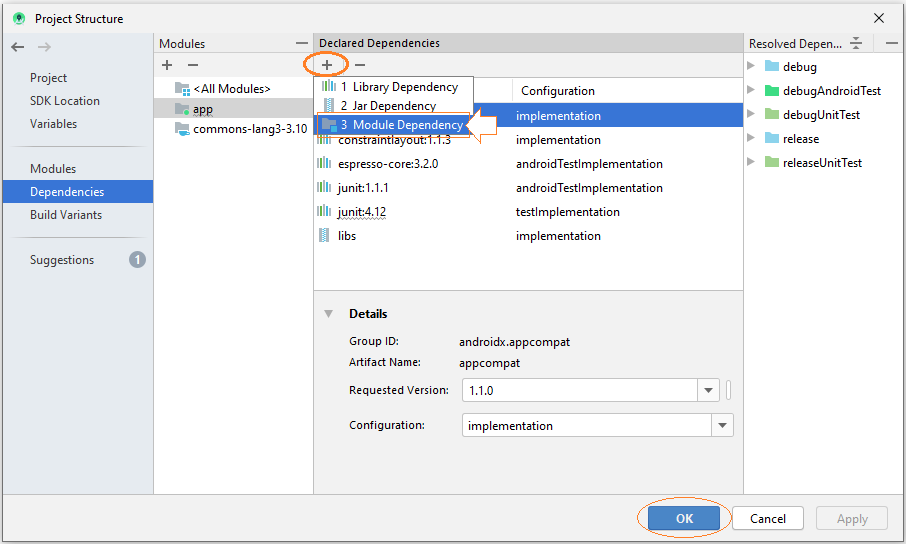
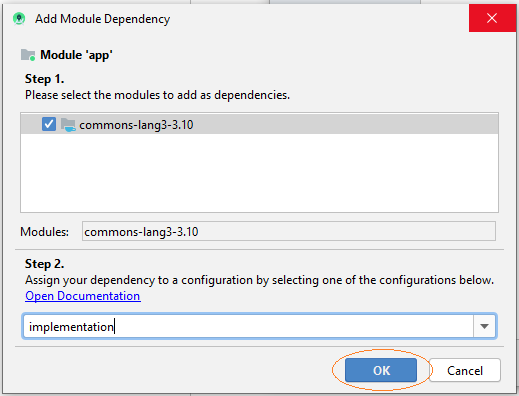
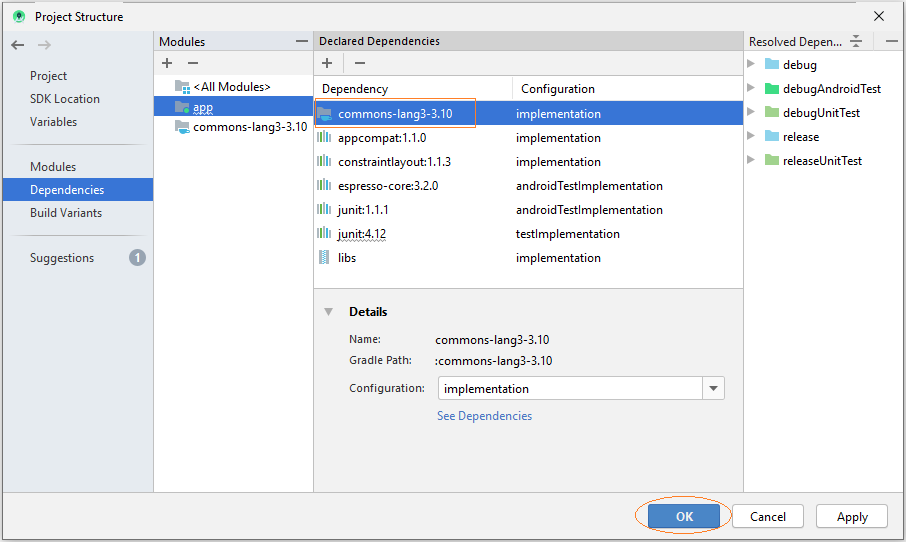
You can check your module which has been declared in build.grade (Module: app).
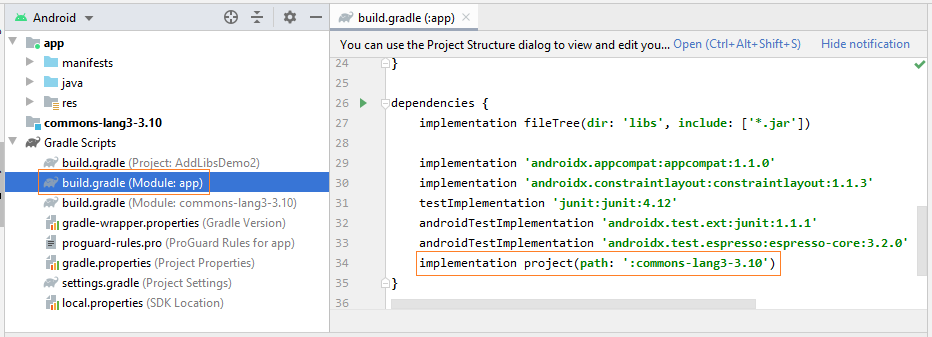
4. Way 3 - Using remote library
Android Studio can use the library on a network, it is located in some repository on the Internet. Suppose you have AddLibDemo3 project, and you need to add the remote library to your project.
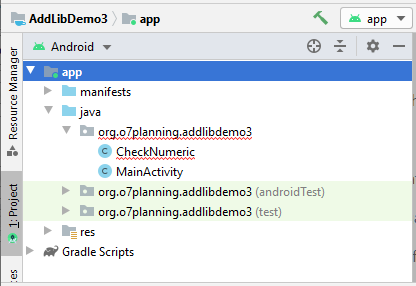
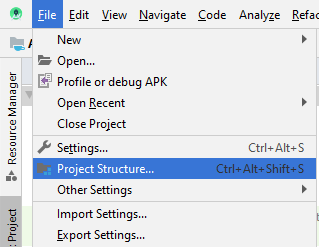
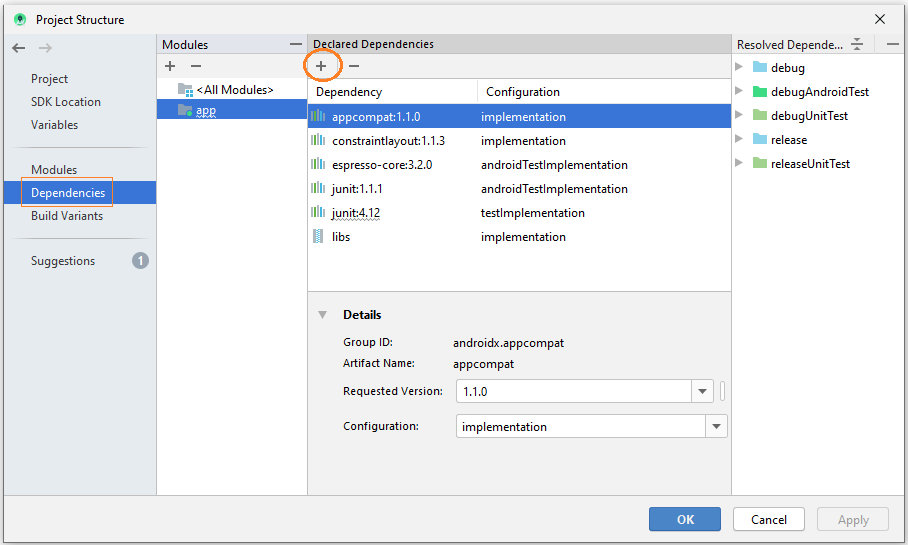
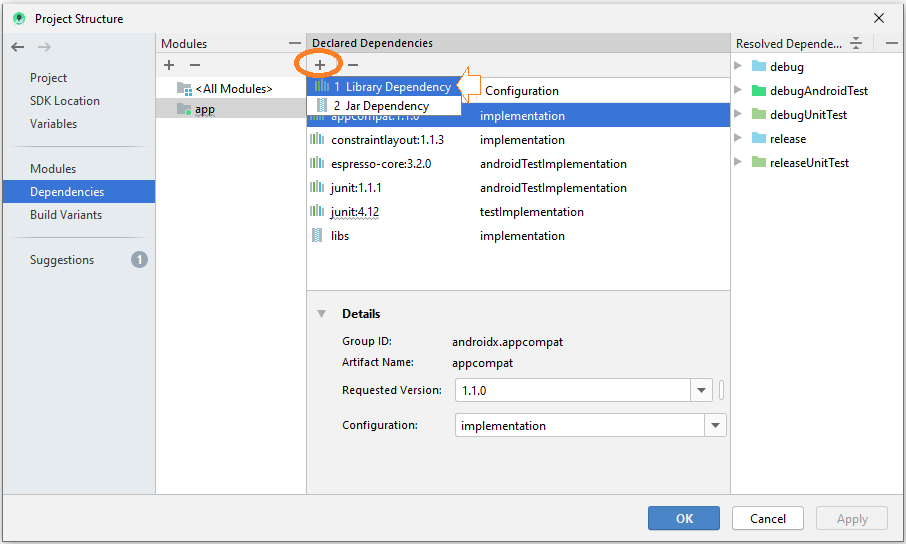
Search the library:
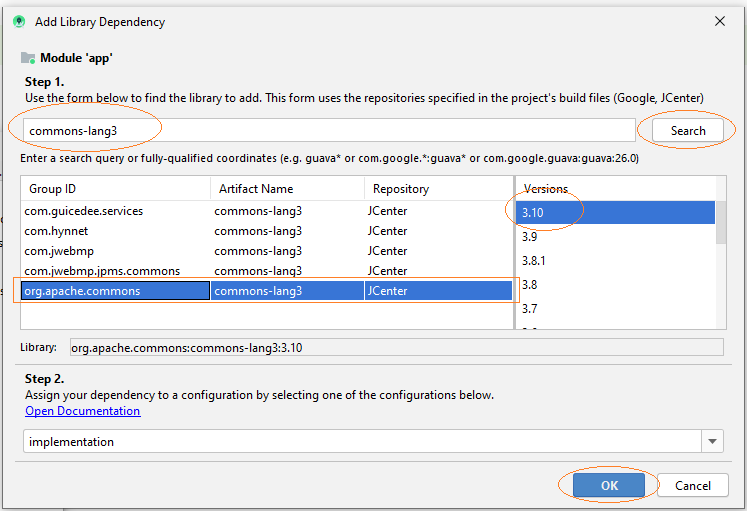
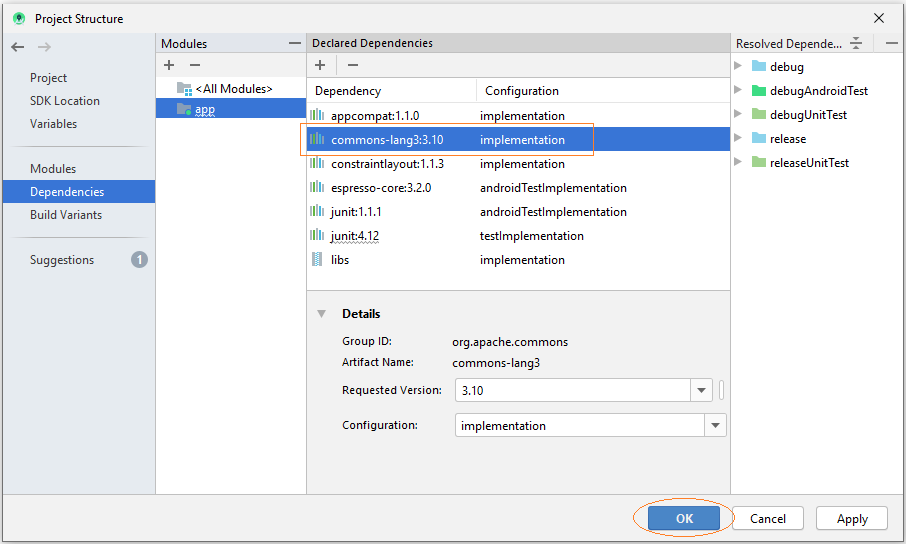
OK, the library has been added to the project, you can see on build.grade (Module: app).
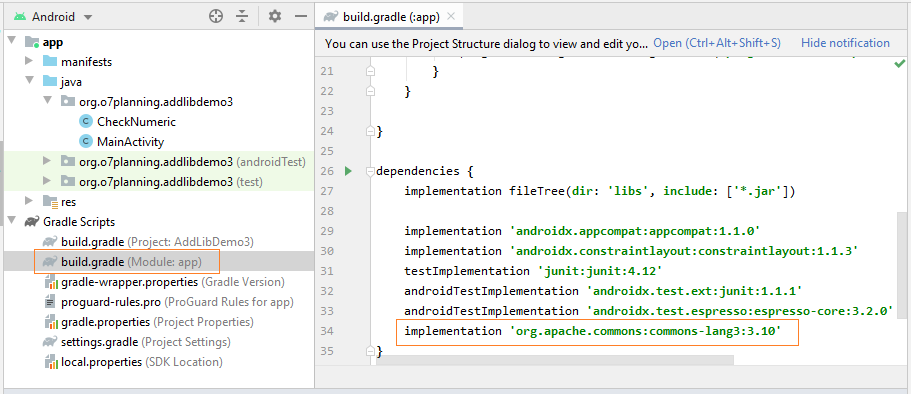
Android Programming Tutorials
- Configure Android Emulator in Android Studio
- Android ToggleButton Tutorial with Examples
- Create a simple File Finder Dialog in Android
- Android TimePickerDialog Tutorial with Examples
- Android DatePickerDialog Tutorial with Examples
- What is needed to get started with Android?
- Install Android Studio on Windows
- Install Intel® HAXM for Android Studio
- Android AsyncTask Tutorial with Examples
- Android AsyncTaskLoader Tutorial with Examples
- Android Tutorial for Beginners - Basic examples
- How to know the phone number of Android Emulator and change it
- Android TextInputLayout Tutorial with Examples
- Android CardView Tutorial with Examples
- Android ViewPager2 Tutorial with Examples
- Get Phone Number in Android using TelephonyManager
- Android Phone Call Tutorial with Examples
- Android Wifi Scanning Tutorial with Examples
- Android 2D Game Tutorial for Beginners
- Android DialogFragment Tutorial with Examples
- Android CharacterPickerDialog Tutorial with Examples
- Android Tutorial for Beginners - Hello Android
- Using Android Device File Explorer
- Enable USB Debugging on Android Device
- Android UI Layouts Tutorial with Examples
- Android SMS Tutorial with Examples
- Android SQLite Database Tutorial with Examples
- Google Maps Android API Tutorial with Examples
- Android Text to Speech Tutorial with Examples
- Android Space Tutorial with Examples
- Android Toast Tutorial with Examples
- Create a custom Android Toast
- Android SnackBar Tutorial with Examples
- Android TextView Tutorial with Examples
- Android TextClock Tutorial with Examples
- Android EditText Tutorial with Examples
- Android TextWatcher Tutorial with Examples
- Format Credit Card Number with Android TextWatcher
- Android Clipboard Tutorial with Examples
- Create a simple File Chooser in Android
- Android AutoCompleteTextView and MultiAutoCompleteTextView Tutorial with Examples
- Android ImageView Tutorial with Examples
- Android ImageSwitcher Tutorial with Examples
- Android ScrollView and HorizontalScrollView Tutorial with Examples
- Android WebView Tutorial with Examples
- Android SeekBar Tutorial with Examples
- Android Dialog Tutorial with Examples
- Android AlertDialog Tutorial with Examples
- Android RatingBar Tutorial with Examples
- Android ProgressBar Tutorial with Examples
- Android Spinner Tutorial with Examples
- Android Button Tutorial with Examples
- Android Switch Tutorial with Examples
- Android ImageButton Tutorial with Examples
- Android FloatingActionButton Tutorial with Examples
- Android CheckBox Tutorial with Examples
- Android RadioGroup and RadioButton Tutorial with Examples
- Android Chip and ChipGroup Tutorial with Examples
- Using image assets and icon assets of Android Studio
- Setting SD Card for Android Emulator
- ChipGroup and Chip Entry Example
- How to add external libraries to Android Project in Android Studio?
- How to disable the permissions already granted to the Android application?
- How to remove applications from Android Emulator?
- Android LinearLayout Tutorial with Examples
- Android TableLayout Tutorial with Examples
- Android FrameLayout Tutorial with Examples
- Android QuickContactBadge Tutorial with Examples
- Android StackView Tutorial with Examples
- Android Camera Tutorial with Examples
- Android MediaPlayer Tutorial with Examples
- Android VideoView Tutorial with Examples
- Playing Sound effects in Android with SoundPool
- Android Networking Tutorial with Examples
- Android JSON Parser Tutorial with Examples
- Android SharedPreferences Tutorial with Examples
- Android Internal Storage Tutorial with Examples
- Android External Storage Tutorial with Examples
- Android Intents Tutorial with Examples
- Example of an explicit Android Intent, calling another Intent
- Example of implicit Android Intent, open a URL, send an email
- Android Services Tutorial with Examples
- Android Notifications Tutorial with Examples
- Android DatePicker Tutorial with Examples
- Android TimePicker Tutorial with Examples
- Android Chronometer Tutorial with Examples
- Android OptionMenu Tutorial with Examples
- Android ContextMenu Tutorial with Examples
- Android PopupMenu Tutorial with Examples
- Android Fragments Tutorial with Examples
- Android ListView Tutorial with Examples
- Android ListView with Checkbox using ArrayAdapter
- Android GridView Tutorial with Examples
Show More