Android JSON Parser Tutorial with Examples
1. What is JSON?
JSON (JavaScript Object Notation) is a data exchange format. It stores data in key and value pair. Compared to XML, JSON is more simple and easier to read.
Hãy xem một ví dụ đơn giản của JSON:
{
"name" : "Tran",
"address" : "Hai Duong, Vietnam",
"phones" : [0121111111, 012222222]
}
Key-value pairs can be nested:
{
"id": 111 ,
"name":"Microsoft",
"websites": [
"http://microsoft.com",
"http://msn.com",
"http://hotmail.com"
],
"address":{
"street":"1 Microsoft Way",
"city":"Redmond"
}
}
In Java, there are very many open source code libraries which help manipulate with JSON documents, for example:
- JSONP
- json.org
- Jackson
- Google GSON
- json-lib
- javax json
- json-simple
- json-smart
- flexjson
- fastjson
Android provides available supports for the library to work with JSON, you don't need to declare any other libraries. In this manual, I will instruct you how to work with the JSON using the JSON API available in the operating system of Android.
2. JSON & Java Object
The following illustration describes the relationship between JSON and Java classes.
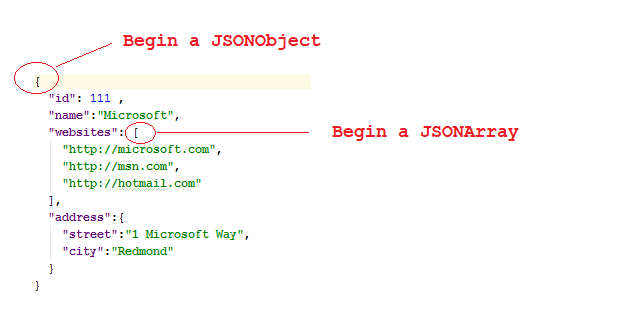
3. Quickly generate a project to work with JSON
I quickly generate a project named JSONTutorial to work with JSON through examples.
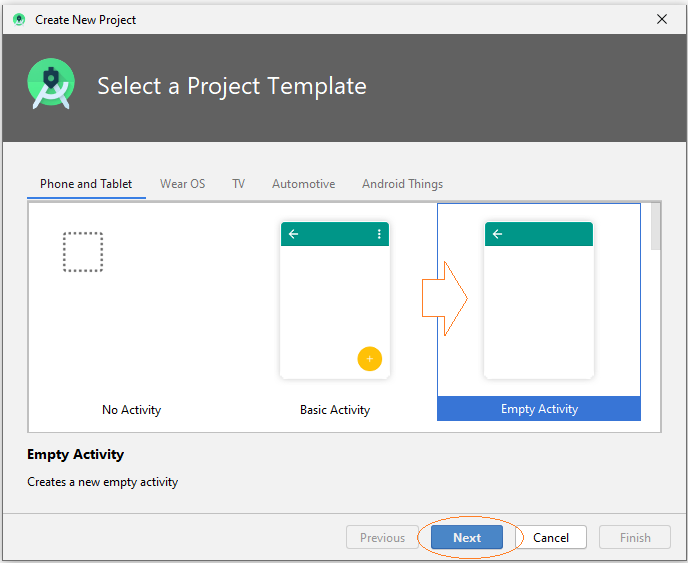
- Name: JSONTutorial
- Package name: org.o7planning.jsontutorial
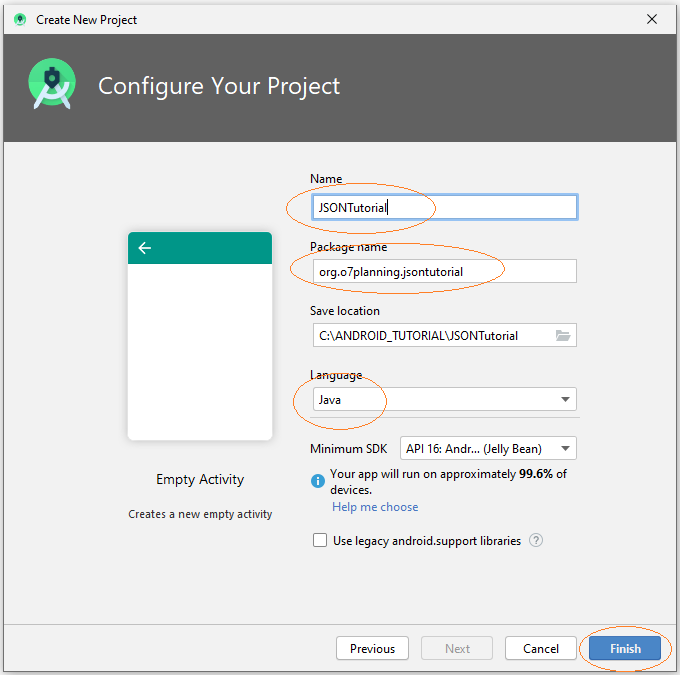
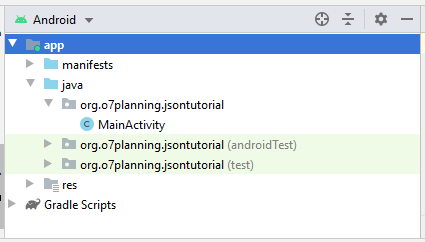
Create a raw folder:
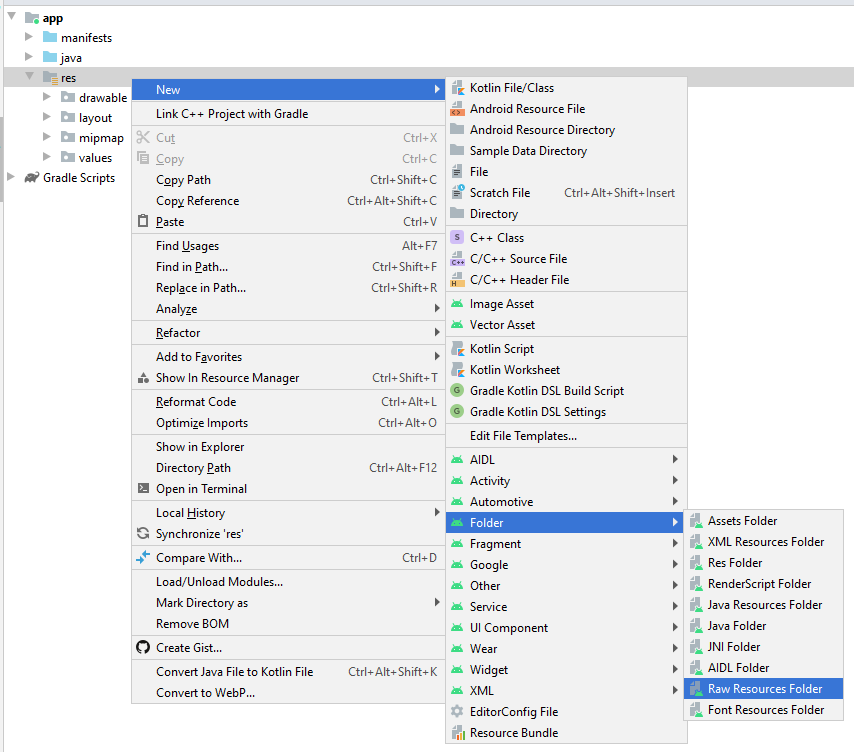
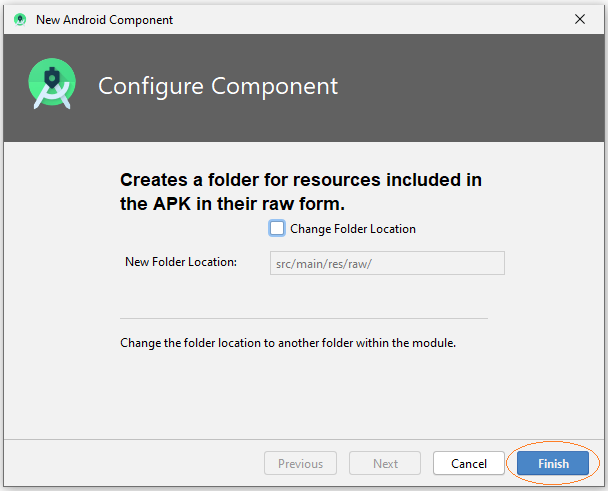
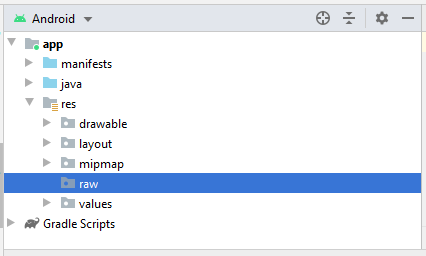
I create some json files in the 'raw' folder, which will be involved in the examples in this document.
The sources of providing JSON data can be from files or URLs, etc. At the end of the document you can see the example of taking JSON data from URL, analyzed and displayed on an Android application.
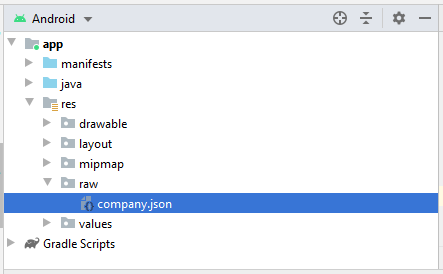
company.json
{
"id": 111 ,
"name":"Microsoft",
"websites": [
"http://microsoft.com",
"http://msn.com",
"http://hotmail.com"
],
"address":{
"street":"1 Microsoft Way",
"city":"Redmond"
}
}
Design activity_main.xml:
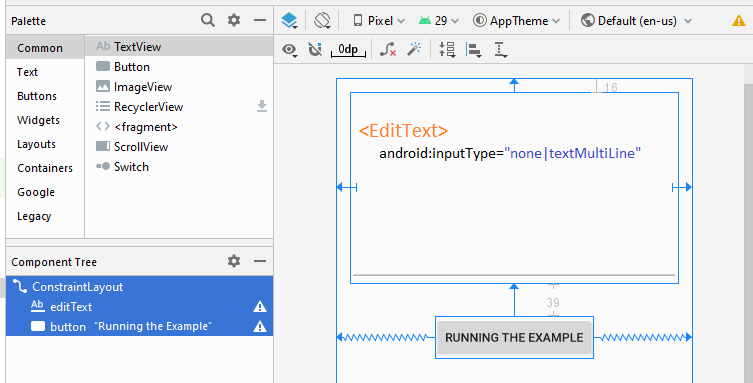
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<EditText
android:id="@+id/editText"
android:layout_width="0dp"
android:layout_height="220dp"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:ems="10"
android:inputType="none|textMultiLine"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="39dp"
android:text="Running the Example"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/editText" />
</androidx.constraintlayout.widget.ConstraintLayout>
4. Java Beans classes
Some classes participated in the examples:
Address.java
package org.o7planning.jsontutorial.beans;
public class Address {
private String street;
private String city;
public Address() {
}
public Address(String street, String city) {
this.street = street;
this.city = city;
}
public String getStreet() {
return street;
}
public void setStreet(String street) {
this.street = street;
}
public String getCity() {
return city;
}
public void setCity(String city) {
this.city = city;
}
@Override
public String toString() {
return street + ", " + city;
}
}
Company.java
package org.o7planning.jsontutorial.beans;
public class Company {
private int id;
private String name;
private String[] websites;
private Address address;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String[] getWebsites() {
return websites;
}
public void setWebsites(String[] websites) {
this.websites = websites;
}
public Address getAddress() {
return address;
}
public void setAddress(Address address) {
this.address = address;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("\n id:" + this.id);
sb.append("\n name:" + this.name);
if (this.websites != null) {
sb.append("\n website: ");
for (String website : this.websites) {
sb.append(website + ", ");
}
}
if (this.address != null) {
sb.append("\n address:" + this.address.toString());
}
return sb.toString();
}
}
5. Example, read JSON data transferred into a Java object
In this example, we will read the JSON data file and transfer it into a Java object.
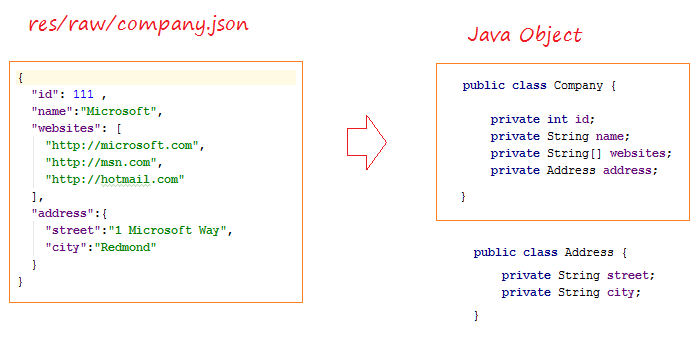
ReadJSONExample.java
package org.o7planning.jsontutorial.json;
import android.content.Context;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import org.o7planning.jsontutorial.R;
import org.o7planning.jsontutorial.beans.Address;
import org.o7planning.jsontutorial.beans.Company;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
public class ReadJSONExample {
// Read the company.json file and convert it to a java object.
public static Company readCompanyJSONFile(Context context) throws IOException,JSONException {
// Read content of company.json
String jsonText = readText(context, R.raw.company);
JSONObject jsonRoot = new JSONObject(jsonText);
int id= jsonRoot.getInt("id");
String name = jsonRoot.getString("name");
JSONArray jsonArray = jsonRoot.getJSONArray("websites");
String[] websites = new String[jsonArray.length()];
for(int i=0;i < jsonArray.length();i++) {
websites[i] = jsonArray.getString(i);
}
JSONObject jsonAddress = jsonRoot.getJSONObject("address");
String street = jsonAddress.getString("street");
String city = jsonAddress.getString("city");
Address address= new Address(street, city);
Company company = new Company();
company.setId(id);
company.setName(name);
company.setAddress(address);
company.setWebsites(websites);
return company;
}
private static String readText(Context context, int resId) throws IOException {
InputStream is = context.getResources().openRawResource(resId);
BufferedReader br= new BufferedReader(new InputStreamReader(is));
StringBuilder sb= new StringBuilder();
String s= null;
while(( s = br.readLine())!=null) {
sb.append(s);
sb.append("\n");
}
return sb.toString();
}
}
MainActivity.java
package org.o7planning.jsontutorial;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import org.o7planning.jsontutorial.beans.Company;
import org.o7planning.jsontutorial.json.ReadJSONExample;
public class MainActivity extends AppCompatActivity {
private EditText outputText;
private Button button;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.outputText = (EditText)this.findViewById(R.id.editText);
this.button = (Button) this.findViewById(R.id.button);
this.button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
runExample(view);
}
});
}
public void runExample(View view) {
try {
Company company = ReadJSONExample.readCompanyJSONFile(this);
outputText.setText(company.toString());
} catch(Exception e) {
outputText.setText(e.getMessage());
e.printStackTrace();
}
}
}
Run the example:
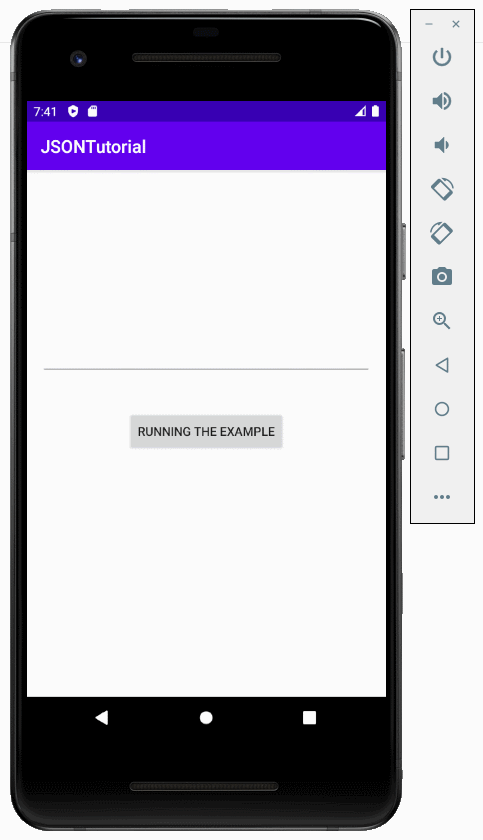
6. Example of converting a Java object into JSON data
The following example converts a Java object into a JSON data.
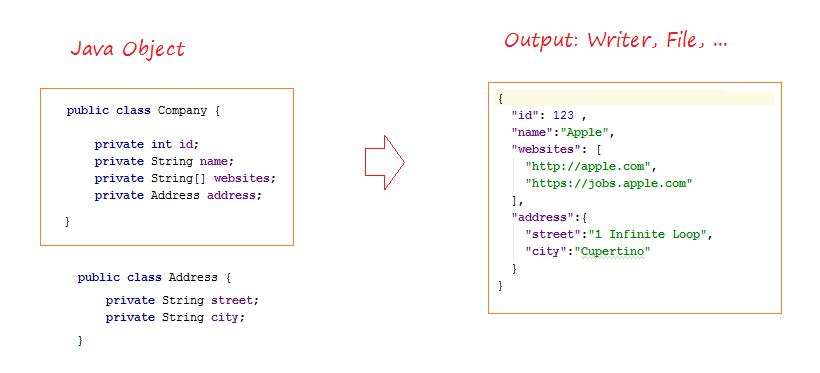
JsonWriterExample.java
package org.o7planning.jsontutorial.json;
import android.util.JsonWriter;
import org.o7planning.jsontutorial.beans.Address;
import org.o7planning.jsontutorial.beans.Company;
import java.io.IOException;
import java.io.Writer;
public class JsonWriterExample {
public static void writeJsonStream(Writer output, Company company ) throws IOException {
JsonWriter jsonWriter = new JsonWriter(output);
jsonWriter.beginObject();// begin root
jsonWriter.name("id").value(company.getId());
jsonWriter.name("name").value(company.getName());
String[] websites= company.getWebsites();
// "websites": [ ....]
jsonWriter.name("websites").beginArray(); // begin websites
for(String website: websites) {
jsonWriter.value(website);
}
jsonWriter.endArray();// end websites
// "address": { ... }
jsonWriter.name("address").beginObject(); // begin address
jsonWriter.name("street").value(company.getAddress().getStreet());
jsonWriter.name("city").value(company.getAddress().getCity());
jsonWriter.endObject();// end address
// end root
jsonWriter.endObject();
}
public static Company createCompany() {
Company company = new Company();
company.setId(123);
company.setName("Apple");
String[] websites = { "http://apple.com", "https://jobs.apple.com" };
company.setWebsites(websites);
Address address = new Address();
address.setCity("Cupertino");
address.setStreet("1 Infinite Loop");
company.setAddress(address);
return company;
}
}
MainActivity.java (2)
package org.o7planning.jsontutorial;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import org.o7planning.jsontutorial.beans.Company;
import org.o7planning.jsontutorial.json.JsonWriterExample;
import java.io.StringWriter;
public class MainActivity extends AppCompatActivity {
private EditText outputText;
private Button button;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.outputText = (EditText)this.findViewById(R.id.editText);
this.button = (Button) this.findViewById(R.id.button);
this.button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
runExample(view);
}
});
}
public void runExample(View view) {
try {
StringWriter output = new StringWriter();
Company company = JsonWriterExample.createCompany();
JsonWriterExample.writeJsonStream(output, company);
String jsonText = output.toString();
outputText.setText(jsonText);
} catch(Exception e) {
outputText.setText(e.getMessage());
e.printStackTrace();
}
}
}
Running the example:
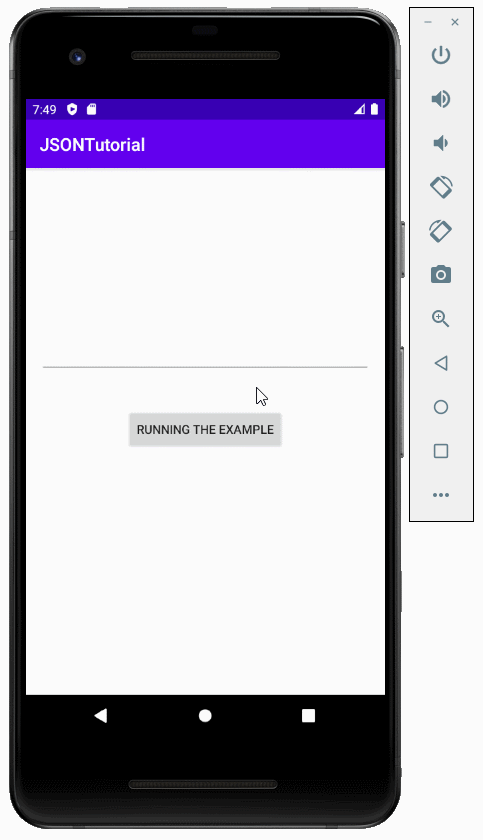
Android Programming Tutorials
- Configure Android Emulator in Android Studio
- Android ToggleButton Tutorial with Examples
- Create a simple File Finder Dialog in Android
- Android TimePickerDialog Tutorial with Examples
- Android DatePickerDialog Tutorial with Examples
- What is needed to get started with Android?
- Install Android Studio on Windows
- Install Intel® HAXM for Android Studio
- Android AsyncTask Tutorial with Examples
- Android AsyncTaskLoader Tutorial with Examples
- Android Tutorial for Beginners - Basic examples
- How to know the phone number of Android Emulator and change it
- Android TextInputLayout Tutorial with Examples
- Android CardView Tutorial with Examples
- Android ViewPager2 Tutorial with Examples
- Get Phone Number in Android using TelephonyManager
- Android Phone Call Tutorial with Examples
- Android Wifi Scanning Tutorial with Examples
- Android 2D Game Tutorial for Beginners
- Android DialogFragment Tutorial with Examples
- Android CharacterPickerDialog Tutorial with Examples
- Android Tutorial for Beginners - Hello Android
- Using Android Device File Explorer
- Enable USB Debugging on Android Device
- Android UI Layouts Tutorial with Examples
- Android SMS Tutorial with Examples
- Android SQLite Database Tutorial with Examples
- Google Maps Android API Tutorial with Examples
- Android Text to Speech Tutorial with Examples
- Android Space Tutorial with Examples
- Android Toast Tutorial with Examples
- Create a custom Android Toast
- Android SnackBar Tutorial with Examples
- Android TextView Tutorial with Examples
- Android TextClock Tutorial with Examples
- Android EditText Tutorial with Examples
- Android TextWatcher Tutorial with Examples
- Format Credit Card Number with Android TextWatcher
- Android Clipboard Tutorial with Examples
- Create a simple File Chooser in Android
- Android AutoCompleteTextView and MultiAutoCompleteTextView Tutorial with Examples
- Android ImageView Tutorial with Examples
- Android ImageSwitcher Tutorial with Examples
- Android ScrollView and HorizontalScrollView Tutorial with Examples
- Android WebView Tutorial with Examples
- Android SeekBar Tutorial with Examples
- Android Dialog Tutorial with Examples
- Android AlertDialog Tutorial with Examples
- Android RatingBar Tutorial with Examples
- Android ProgressBar Tutorial with Examples
- Android Spinner Tutorial with Examples
- Android Button Tutorial with Examples
- Android Switch Tutorial with Examples
- Android ImageButton Tutorial with Examples
- Android FloatingActionButton Tutorial with Examples
- Android CheckBox Tutorial with Examples
- Android RadioGroup and RadioButton Tutorial with Examples
- Android Chip and ChipGroup Tutorial with Examples
- Using image assets and icon assets of Android Studio
- Setting SD Card for Android Emulator
- ChipGroup and Chip Entry Example
- How to add external libraries to Android Project in Android Studio?
- How to disable the permissions already granted to the Android application?
- How to remove applications from Android Emulator?
- Android LinearLayout Tutorial with Examples
- Android TableLayout Tutorial with Examples
- Android FrameLayout Tutorial with Examples
- Android QuickContactBadge Tutorial with Examples
- Android StackView Tutorial with Examples
- Android Camera Tutorial with Examples
- Android MediaPlayer Tutorial with Examples
- Android VideoView Tutorial with Examples
- Playing Sound effects in Android with SoundPool
- Android Networking Tutorial with Examples
- Android JSON Parser Tutorial with Examples
- Android SharedPreferences Tutorial with Examples
- Android Internal Storage Tutorial with Examples
- Android External Storage Tutorial with Examples
- Android Intents Tutorial with Examples
- Example of an explicit Android Intent, calling another Intent
- Example of implicit Android Intent, open a URL, send an email
- Android Services Tutorial with Examples
- Android Notifications Tutorial with Examples
- Android DatePicker Tutorial with Examples
- Android TimePicker Tutorial with Examples
- Android Chronometer Tutorial with Examples
- Android OptionMenu Tutorial with Examples
- Android ContextMenu Tutorial with Examples
- Android PopupMenu Tutorial with Examples
- Android Fragments Tutorial with Examples
- Android ListView Tutorial with Examples
- Android ListView with Checkbox using ArrayAdapter
- Android GridView Tutorial with Examples
Show More