Android CharacterPickerDialog Tutorial with Examples
1. Android CharacterPickerDialog
Android CharacterPickerDialog is a dialog box that permits the user to select "accented characters" of a base character. Oftentimes, the CharacterPickerDialog is useful because not all of the phones have the Keyboard Layout that is appropriate for a particular language.
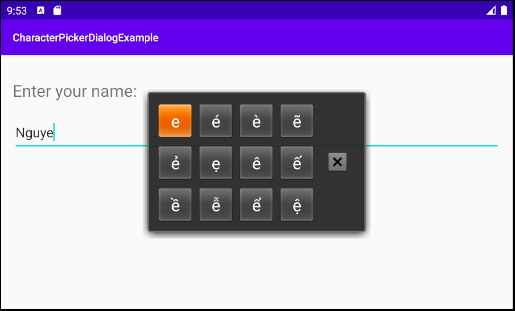
For example, Vietnamese language has a list of many accented characters, which probably look strange for you:
a á à ã ả ạ ă ắ ằ ẵ ẳ ặ â ấ ầ ẫ ẩ ậ
A Á À Ã Ả Ạ Ă Ắ Ằ Ẵ Ẳ Ặ Â Ấ Ầ Ẫ Ẩ Ậ
d đ
D Đ
e é è ẽ ẻ ẹ ê ế ề ễ ể ệ
E É È Ẽ Ẻ Ẹ Ê Ế Ề Ễ Ể Ệ
i í ì ĩ ỉ ị
I Í Ì Ĩ Ỉ Ị
o ó ò õ ỏ ọ ô ố ồ ỗ ổ ộ ơ ớ ờ ỡ ở ợ
O Ó Ò Õ Ỏ Ọ Ô Ố Ồ Ỗ Ổ Ộ Ơ Ớ Ờ Ỡ Ở Ợ
u ú ù ũ ủ ụ ư ứ ừ ữ ử ự
U Ú Ù Ũ Ủ Ụ Ư Ứ Ừ Ữ Ử Ự
y ý ỳ ỹ ỷ ỵ
Y Ý Ỳ Ỹ Ỷ Ỵ
Here are some special characters in German:
↵
ä ö ü ß
And some special characters in French:
à â æ ç é è ê ë ï î ô œ ù û ü ÿ
2. Example of CharacterPickerDialog
Here is a simple application that uses CharacterPickerDialog to assist the user to select the special characters in Vietnamese.
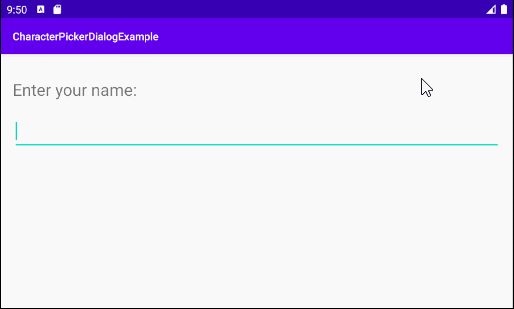
Create a new project on Android Studio:
- File > New > New Project > Empty Activity
- Name: CharacterPickerDialogExample
- Package name: org.o7planning.characterpickerexample
- Language: Java
This is what the interface of the application looks like:
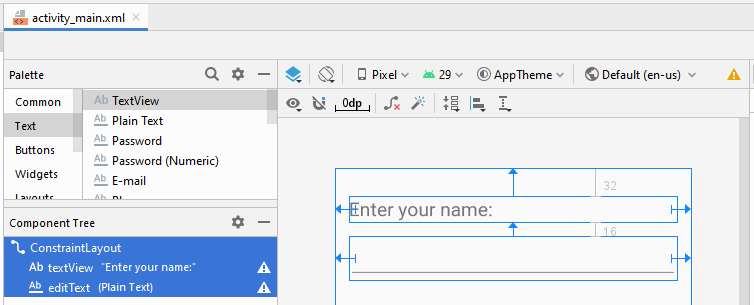
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="32dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Enter your name:"
android:textSize="22sp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<EditText
android:id="@+id/editText"
android:layout_width="0dp"
android:layout_height="51dp"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:ems="10"
android:inputType="textPersonName"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView" />
</androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.java
package org.o7planning.characterpickerdialogexample;
import android.os.Bundle;
import android.text.Editable;
import android.text.method.CharacterPickerDialog;
import android.util.Log;
import android.view.KeyEvent;
import android.view.View;
import android.widget.AdapterView;
import android.widget.EditText;
import androidx.appcompat.app.AppCompatActivity;
public class MainActivity extends AppCompatActivity {
private static final String LOG_TAG = "AndroidExample";
private EditText editText;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.editText = this.findViewById(R.id.editText);
this.editText.setOnKeyListener(new View.OnKeyListener() {
@Override
public boolean onKey(View v, int keyCode, KeyEvent event) {
return handleOnKey( keyCode, event);
}
});
}
private boolean handleOnKey(int keyCode, KeyEvent event) {
if(event.getAction() == KeyEvent.ACTION_DOWN) {
return false;
}
char base = (char) event.getUnicodeChar();
Log.i(LOG_TAG, "keyCode: " + keyCode + ", Base character: " + base);
final String accentedString = AccentedStringUtils.getAccentedString(base);
if(accentedString == null) {
return false;
}
final Editable editable = this.editText.getText();
CharacterPickerDialog dialog= new CharacterPickerDialog(this, new View(this), editable, accentedString,false) {
// User click on Cancel button.
@Override
public void onClick (View v) {
// Do something here
// ...
super.onClick(v);
}
// User click on Character button.
@Override
public void onItemClick(AdapterView parent, View view, int position, long id){
// Do something here
// ...
super.onItemClick(parent, view, position, id);
}
};
dialog.show();
return false;
}
}
AccentedStringUtils.java
package org.o7planning.characterpickerdialogexample;
public class AccentedStringUtils {
//
// Vietnamese special characters.
//
private static final String[] ACCENTED_STRINGS = {
"aáàãảạăắằẵẳặâấầẫẩậ",
"AÁÀÃẢẠĂẮẰẴẲẶÂẤẦẪẨẬ",
"dđ",
"DĐ",
"eéèẽẻẹêếềễểệ",
"EÉÈẼẺẸÊẾỀỄỂỆ",
"iíìĩỉị",
"IÍÌĨỈỊ",
"oóòõỏọôốồỗổộơớờỡởợ",
"OÓÒÕỎỌÔỐỒỖỔỘƠỚỜỠỞỢ",
"uúùũủụưứừữửự",
"UÚÙŨỦỤƯỨỪỮỬỰ",
"yýỳỹỷỵ",
"YÝỲỸỶỴ"
};
public static String getAccentedString(char base) {
for(int i = 0; i< ACCENTED_STRINGS.length; i++) {
String accentedString = ACCENTED_STRINGS[i];
if(accentedString.charAt(0) == base) {
return accentedString;
}
}
return null;
}
}
Android Programming Tutorials
- Configure Android Emulator in Android Studio
- Android ToggleButton Tutorial with Examples
- Create a simple File Finder Dialog in Android
- Android TimePickerDialog Tutorial with Examples
- Android DatePickerDialog Tutorial with Examples
- What is needed to get started with Android?
- Install Android Studio on Windows
- Install Intel® HAXM for Android Studio
- Android AsyncTask Tutorial with Examples
- Android AsyncTaskLoader Tutorial with Examples
- Android Tutorial for Beginners - Basic examples
- How to know the phone number of Android Emulator and change it
- Android TextInputLayout Tutorial with Examples
- Android CardView Tutorial with Examples
- Android ViewPager2 Tutorial with Examples
- Get Phone Number in Android using TelephonyManager
- Android Phone Call Tutorial with Examples
- Android Wifi Scanning Tutorial with Examples
- Android 2D Game Tutorial for Beginners
- Android DialogFragment Tutorial with Examples
- Android CharacterPickerDialog Tutorial with Examples
- Android Tutorial for Beginners - Hello Android
- Using Android Device File Explorer
- Enable USB Debugging on Android Device
- Android UI Layouts Tutorial with Examples
- Android SMS Tutorial with Examples
- Android SQLite Database Tutorial with Examples
- Google Maps Android API Tutorial with Examples
- Android Text to Speech Tutorial with Examples
- Android Space Tutorial with Examples
- Android Toast Tutorial with Examples
- Create a custom Android Toast
- Android SnackBar Tutorial with Examples
- Android TextView Tutorial with Examples
- Android TextClock Tutorial with Examples
- Android EditText Tutorial with Examples
- Android TextWatcher Tutorial with Examples
- Format Credit Card Number with Android TextWatcher
- Android Clipboard Tutorial with Examples
- Create a simple File Chooser in Android
- Android AutoCompleteTextView and MultiAutoCompleteTextView Tutorial with Examples
- Android ImageView Tutorial with Examples
- Android ImageSwitcher Tutorial with Examples
- Android ScrollView and HorizontalScrollView Tutorial with Examples
- Android WebView Tutorial with Examples
- Android SeekBar Tutorial with Examples
- Android Dialog Tutorial with Examples
- Android AlertDialog Tutorial with Examples
- Android RatingBar Tutorial with Examples
- Android ProgressBar Tutorial with Examples
- Android Spinner Tutorial with Examples
- Android Button Tutorial with Examples
- Android Switch Tutorial with Examples
- Android ImageButton Tutorial with Examples
- Android FloatingActionButton Tutorial with Examples
- Android CheckBox Tutorial with Examples
- Android RadioGroup and RadioButton Tutorial with Examples
- Android Chip and ChipGroup Tutorial with Examples
- Using image assets and icon assets of Android Studio
- Setting SD Card for Android Emulator
- ChipGroup and Chip Entry Example
- How to add external libraries to Android Project in Android Studio?
- How to disable the permissions already granted to the Android application?
- How to remove applications from Android Emulator?
- Android LinearLayout Tutorial with Examples
- Android TableLayout Tutorial with Examples
- Android FrameLayout Tutorial with Examples
- Android QuickContactBadge Tutorial with Examples
- Android StackView Tutorial with Examples
- Android Camera Tutorial with Examples
- Android MediaPlayer Tutorial with Examples
- Android VideoView Tutorial with Examples
- Playing Sound effects in Android with SoundPool
- Android Networking Tutorial with Examples
- Android JSON Parser Tutorial with Examples
- Android SharedPreferences Tutorial with Examples
- Android Internal Storage Tutorial with Examples
- Android External Storage Tutorial with Examples
- Android Intents Tutorial with Examples
- Example of an explicit Android Intent, calling another Intent
- Example of implicit Android Intent, open a URL, send an email
- Android Services Tutorial with Examples
- Android Notifications Tutorial with Examples
- Android DatePicker Tutorial with Examples
- Android TimePicker Tutorial with Examples
- Android Chronometer Tutorial with Examples
- Android OptionMenu Tutorial with Examples
- Android ContextMenu Tutorial with Examples
- Android PopupMenu Tutorial with Examples
- Android Fragments Tutorial with Examples
- Android ListView Tutorial with Examples
- Android ListView with Checkbox using ArrayAdapter
- Android GridView Tutorial with Examples
Show More