Android Networking Tutorial with Examples
1. Android Networking Overview
In Android, Network programming includes a request to the server and retrieve the data returned. Basically you have two APIs for working with the network:
Apache HttpClient:
- This is an open source library provided by the Apache.
HttpURLConnection
- This is an official API of Android, it began to be included in the version of Android 2.3, in the previous, Android using Apache HttpClient to work with the network.
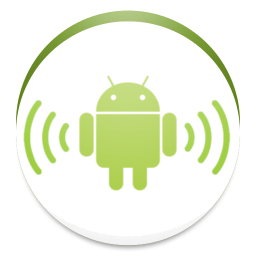
You need to grant some permissions to the application if you want to work with the network
<uses-permission android:name="android.permission.INTERNET"/>
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE"/>
android.permission.INTERNET:
- Add this permission, allowing your application will have the ability to connect to the network.
android.permission.ACCESS_NETWORK_STATE:
- Allows the app to check the status of your network connection.
The following code checks the network connection status:
private boolean checkInternetConnection() {
ConnectivityManager connManager =
(ConnectivityManager) this.getSystemService(Context.CONNECTIVITY_SERVICE);
NetworkInfo networkInfo = connManager.getActiveNetworkInfo();
if (networkInfo == null) {
Toast.makeText(this, "No default network is currently active", Toast.LENGTH_LONG).show();
return false;
}
if (!networkInfo.isConnected()) {
Toast.makeText(this, "Network is not connected", Toast.LENGTH_LONG).show();
return false;
}
if (!networkInfo.isAvailable()) {
Toast.makeText(this, "Network not available", Toast.LENGTH_LONG).show();
return false;
}
Toast.makeText(this, "Network OK", Toast.LENGTH_LONG).show();
return true;
}
2. NetworkOnMainThreadException
By default, when you work with a network in Android, you should create a new thread to send and receive data returned. If you work on the main thread you will get android.os.NetworkOnMainThreadException, this is the default policy of android. However you can override this policy of Android to be able to work with the Network on the main thread.
StrictMode.ThreadPolicy policy = new StrictMode.ThreadPolicy.Builder().permitAll().build();
StrictMode.setThreadPolicy(policy);
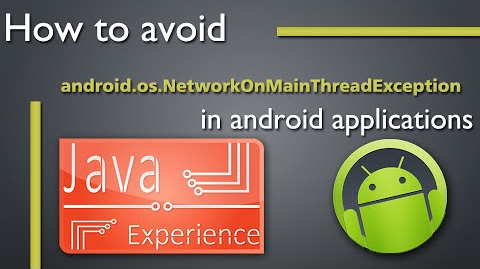
It is recommended that you create a class extending from AsyncTask <Params, Progress, Result>, this class is not a thread, it extends from Object, but when to do their task (Call AsyncTask.execute(params) method), it creates a new thread to execute the doInBackground (params) method. After thread is completed, onPostExecute (result) method will be called.
You can see the illustration below:
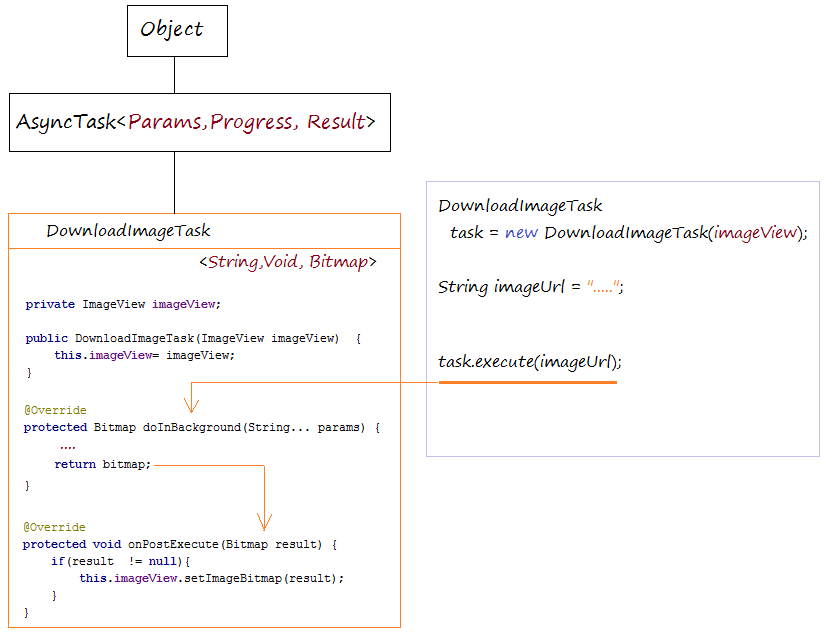
3. HttpURLConnection Example
In this example, you download the Image and json from URL and displays it on the ImageView and TextView.
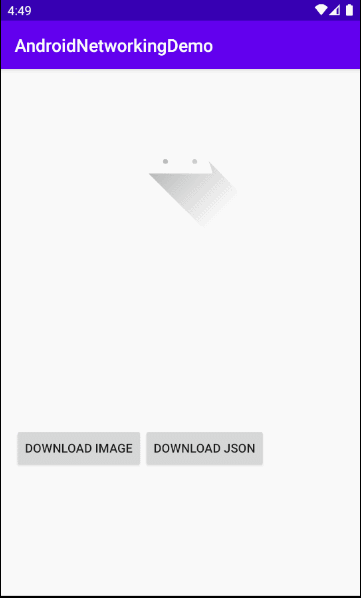
Create project AndroidNetworkingDemo:
- File > New > New Project > Empty Activity
- Name: AndroidNetworkingDemo
- Package name: org.o7planning.androidnetworkingdemo
- Language: Java
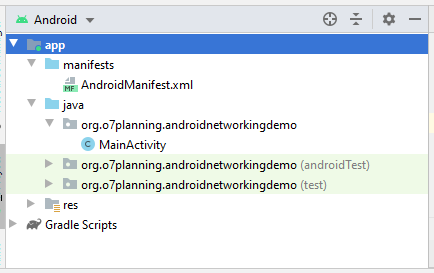
You must grant permissions to the app to access the Internet and the permission to check the status of the network.
<uses-permission android:name="android.permission.INTERNET"/>
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE"/>
Add code to AndroidManifest.xml:
AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="org.o7planning.androidnetworkingdemo">
<uses-permission android:name="android.permission.INTERNET"/>
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE"/>
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
Apps interface:
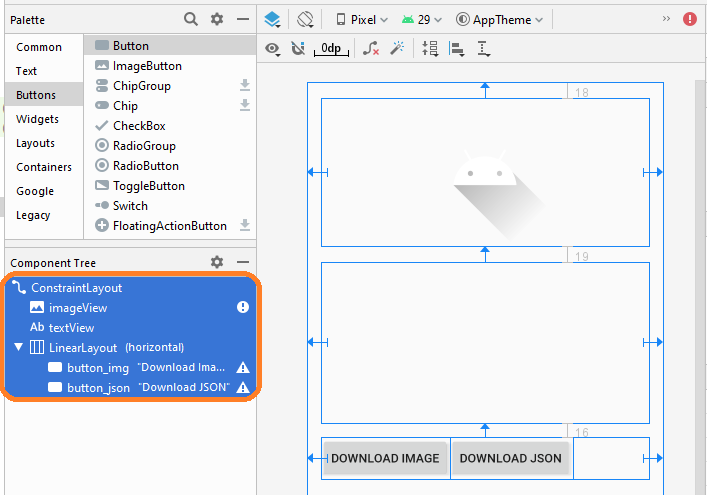
If you are interested in the steps to design this application interface, please see the appendix at the end of the article.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<ImageView
android:id="@+id/imageView"
android:layout_width="0dp"
android:layout_height="171dp"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="18dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:contentDescription="Image"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:srcCompat="@drawable/ic_launcher_foreground" />
<TextView
android:id="@+id/textView"
android:layout_width="0dp"
android:layout_height="186dp"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="19dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/imageView" />
<LinearLayout
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:orientation="horizontal"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView">
<Button
android:id="@+id/button_img"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="0"
android:text="Download Image" />
<Button
android:id="@+id/button_json"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="0"
android:text="Download JSON" />
</LinearLayout>
</androidx.constraintlayout.widget.ConstraintLayout>
Code:
IOUtils.java
package org.o7planning.androidnetworkingdemo;
import java.io.InputStream;
import java.io.Reader;
public class IOUtils {
public static void closeQuietly(InputStream in) {
try {
in.close();
}catch (Exception e) {
}
}
public static void closeQuietly(Reader reader) {
try {
reader.close();
}catch (Exception e) {
}
}
}
DownloadJsonTask.java
package org.o7planning.androidnetworkingdemo;
import android.os.AsyncTask;
import android.util.Log;
import android.widget.TextView;
import java.io.BufferedReader;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
// A task with String input parameter, and returns the result as String.
public class DownloadJsonTask
// AsyncTask<Params, Progress, Result>
extends AsyncTask<String, Void, String> {
private TextView textView;
public DownloadJsonTask(TextView textView) {
this.textView= textView;
}
@Override
protected String doInBackground(String... params) {
String textUrl = params[0];
InputStream in = null;
BufferedReader br= null;
try {
URL url = new URL(textUrl);
HttpURLConnection httpConn = (HttpURLConnection) url.openConnection();
httpConn.setAllowUserInteraction(false);
httpConn.setInstanceFollowRedirects(true);
httpConn.setRequestMethod("GET");
httpConn.connect();
int resCode = httpConn.getResponseCode();
if (resCode == HttpURLConnection.HTTP_OK) {
in = httpConn.getInputStream();
br= new BufferedReader(new InputStreamReader(in));
StringBuilder sb= new StringBuilder();
String s= null;
while((s= br.readLine())!= null) {
sb.append(s);
sb.append("\n");
}
return sb.toString();
} else {
return null;
}
} catch (Exception e) {
e.printStackTrace();
} finally {
IOUtils.closeQuietly(in);
IOUtils.closeQuietly(br);
}
return null;
}
// When the task is completed, this method will be called
// Download complete. Lets update UI
@Override
protected void onPostExecute(String result) {
if(result != null){
this.textView.setText(result);
} else{
Log.e("MyMessage", "Failed to fetch data!");
}
}
}
DownloadImageTask.java
package org.o7planning.androidnetworkingdemo;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.os.AsyncTask;
import android.util.Log;
import android.widget.ImageView;
import java.io.InputStream;
import java.net.HttpURLConnection;
import java.net.URL;
// A task with String input parameter, and returns the result as bitmap.
public class DownloadImageTask
// AsyncTask<Params, Progress, Result>
extends AsyncTask<String, Void, Bitmap> {
private ImageView imageView;
public DownloadImageTask(ImageView imageView) {
this.imageView= imageView;
}
@Override
protected Bitmap doInBackground(String... params) {
String imageUrl = params[0];
InputStream in = null;
try {
URL url = new URL(imageUrl);
HttpURLConnection httpConn = (HttpURLConnection) url.openConnection();
httpConn.setAllowUserInteraction(false);
httpConn.setInstanceFollowRedirects(true);
httpConn.setRequestMethod("GET");
httpConn.connect();
int resCode = httpConn.getResponseCode();
if (resCode == HttpURLConnection.HTTP_OK) {
in = httpConn.getInputStream();
} else {
return null;
}
Bitmap bitmap = BitmapFactory.decodeStream(in);
return bitmap;
} catch (Exception e) {
e.printStackTrace();
} finally {
IOUtils.closeQuietly(in);
}
return null;
}
// When the task is completed, this method will be called
// Download complete. Lets update UI
@Override
protected void onPostExecute(Bitmap result) {
if(result != null){
this.imageView.setImageBitmap(result);
} else{
Log.e("MyMessage", "Failed to fetch data!");
}
}
}
MainActivity.java
package org.o7planning.androidnetworkingdemo;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.content.Context;
import android.net.ConnectivityManager;
import android.net.NetworkInfo;
import android.view.View;
import android.widget.Button;
import android.widget.ImageView;
import android.widget.TextView;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
private ImageView imageView;
private TextView textView;
private Button buttonImg;
private Button buttonJson;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.imageView = (ImageView) this.findViewById(R.id.imageView);
this.textView = (TextView) this.findViewById(R.id.textView);
this.buttonImg = (Button) this.findViewById(R.id.button_img);
this.buttonJson = (Button) this.findViewById(R.id.button_json);
this.buttonImg.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
downloadAndShowImage(v);
}
});
this.buttonJson.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
downloadAndShowJson(v);
}
});
}
private boolean checkInternetConnection() {
// Get Connectivity Manager
ConnectivityManager connManager =
(ConnectivityManager) this.getSystemService(Context.CONNECTIVITY_SERVICE);
// Details about the currently active default data network
NetworkInfo networkInfo = connManager.getActiveNetworkInfo();
if (networkInfo == null) {
Toast.makeText(this, "No default network is currently active", Toast.LENGTH_LONG).show();
return false;
}
if (!networkInfo.isConnected()) {
Toast.makeText(this, "Network is not connected", Toast.LENGTH_LONG).show();
return false;
}
if (!networkInfo.isAvailable()) {
Toast.makeText(this, "Network not available", Toast.LENGTH_LONG).show();
return false;
}
Toast.makeText(this, "Network OK", Toast.LENGTH_LONG).show();
return true;
}
// When user click on the "Download Image".
public void downloadAndShowImage(View view) {
boolean networkOK = this.checkInternetConnection();
if (!networkOK) {
return;
}
String imageUrl = "https://o7planning.org/download/static/default/demo-data/logo.png";
// Create a task to download and display image.
DownloadImageTask task = new DownloadImageTask(this.imageView);
// Execute task (Pass imageUrl).
task.execute(imageUrl);
}
// When user click on the "Download Json".
public void downloadAndShowJson(View view) {
boolean networkOK = this.checkInternetConnection();
if (!networkOK) {
return;
}
String jsonUrl = "https://o7planning.org/download/static/default/demo-data/company.json";
// Create a task to download and display json content.
DownloadJsonTask task = new DownloadJsonTask(this.textView);
// Execute task (Pass jsonUrl).
task.execute(jsonUrl);
}
}
Android Programming Tutorials
- Configure Android Emulator in Android Studio
- Android ToggleButton Tutorial with Examples
- Create a simple File Finder Dialog in Android
- Android TimePickerDialog Tutorial with Examples
- Android DatePickerDialog Tutorial with Examples
- What is needed to get started with Android?
- Install Android Studio on Windows
- Install Intel® HAXM for Android Studio
- Android AsyncTask Tutorial with Examples
- Android AsyncTaskLoader Tutorial with Examples
- Android Tutorial for Beginners - Basic examples
- How to know the phone number of Android Emulator and change it
- Android TextInputLayout Tutorial with Examples
- Android CardView Tutorial with Examples
- Android ViewPager2 Tutorial with Examples
- Get Phone Number in Android using TelephonyManager
- Android Phone Call Tutorial with Examples
- Android Wifi Scanning Tutorial with Examples
- Android 2D Game Tutorial for Beginners
- Android DialogFragment Tutorial with Examples
- Android CharacterPickerDialog Tutorial with Examples
- Android Tutorial for Beginners - Hello Android
- Using Android Device File Explorer
- Enable USB Debugging on Android Device
- Android UI Layouts Tutorial with Examples
- Android SMS Tutorial with Examples
- Android SQLite Database Tutorial with Examples
- Google Maps Android API Tutorial with Examples
- Android Text to Speech Tutorial with Examples
- Android Space Tutorial with Examples
- Android Toast Tutorial with Examples
- Create a custom Android Toast
- Android SnackBar Tutorial with Examples
- Android TextView Tutorial with Examples
- Android TextClock Tutorial with Examples
- Android EditText Tutorial with Examples
- Android TextWatcher Tutorial with Examples
- Format Credit Card Number with Android TextWatcher
- Android Clipboard Tutorial with Examples
- Create a simple File Chooser in Android
- Android AutoCompleteTextView and MultiAutoCompleteTextView Tutorial with Examples
- Android ImageView Tutorial with Examples
- Android ImageSwitcher Tutorial with Examples
- Android ScrollView and HorizontalScrollView Tutorial with Examples
- Android WebView Tutorial with Examples
- Android SeekBar Tutorial with Examples
- Android Dialog Tutorial with Examples
- Android AlertDialog Tutorial with Examples
- Android RatingBar Tutorial with Examples
- Android ProgressBar Tutorial with Examples
- Android Spinner Tutorial with Examples
- Android Button Tutorial with Examples
- Android Switch Tutorial with Examples
- Android ImageButton Tutorial with Examples
- Android FloatingActionButton Tutorial with Examples
- Android CheckBox Tutorial with Examples
- Android RadioGroup and RadioButton Tutorial with Examples
- Android Chip and ChipGroup Tutorial with Examples
- Using image assets and icon assets of Android Studio
- Setting SD Card for Android Emulator
- ChipGroup and Chip Entry Example
- How to add external libraries to Android Project in Android Studio?
- How to disable the permissions already granted to the Android application?
- How to remove applications from Android Emulator?
- Android LinearLayout Tutorial with Examples
- Android TableLayout Tutorial with Examples
- Android FrameLayout Tutorial with Examples
- Android QuickContactBadge Tutorial with Examples
- Android StackView Tutorial with Examples
- Android Camera Tutorial with Examples
- Android MediaPlayer Tutorial with Examples
- Android VideoView Tutorial with Examples
- Playing Sound effects in Android with SoundPool
- Android Networking Tutorial with Examples
- Android JSON Parser Tutorial with Examples
- Android SharedPreferences Tutorial with Examples
- Android Internal Storage Tutorial with Examples
- Android External Storage Tutorial with Examples
- Android Intents Tutorial with Examples
- Example of an explicit Android Intent, calling another Intent
- Example of implicit Android Intent, open a URL, send an email
- Android Services Tutorial with Examples
- Android Notifications Tutorial with Examples
- Android DatePicker Tutorial with Examples
- Android TimePicker Tutorial with Examples
- Android Chronometer Tutorial with Examples
- Android OptionMenu Tutorial with Examples
- Android ContextMenu Tutorial with Examples
- Android PopupMenu Tutorial with Examples
- Android Fragments Tutorial with Examples
- Android ListView Tutorial with Examples
- Android ListView with Checkbox using ArrayAdapter
- Android GridView Tutorial with Examples
Show More