Android SMS Tutorial with Examples
1. Sending SMS in Android
Basically, there are two ways for you to send an SMS text message in Android, which are SmsManager and Intent.ACTION_SEND.
SmsManager
SmsManager allows you to send an SMS text message directly from your application to any other phone number.
Intent.ACTION_SEND
In your application, you can create an implicit Intent and sent it to the Android system in order to have the system send your messages. The system will launch the best SMS sending app available in the system to do this, and it is most likely to use the Messenger application.
The Messenger application is an SMS messagesending application available in Android which is familiar to almost all users.
If you test some SMS sending apps on Android Emulator, you need to know its phone number, then you can test the apps by sending SMS text messages to that phone number.
2. Send an SMS text message by SmsManager
SmsManager is a class available in Android, which allows you to send an SMS text message directly to any phone number.
In this example, I sent an SMS text message to the phone number of the tested device. As soon as I press the send button, the notification will show up to inform me of an SMS text message. Let's see the example below:
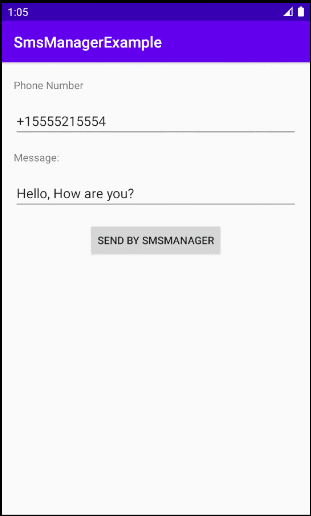
Note: If the user has allowed the application to send SMS text messages, the application will not ask the user again in the next SMS text message sending. Thus, oftentimes you need to remove the permissions given to the application in order to test the application more thoroughly.
Alright, now on Android Studio create a project:
- Name: SmsManagerExample
- Package name: org.o7planning.smsmanagerexample
Next, add the following XML code to the AndroidManifest.xml file to allow the application to send SMS text messages. Note: In Android 6.0+ (API Level 23+), the application needs to ask its user for permission to send SMS text messages(See also in the example code).
<uses-permission android:name="android.permission.SEND_SMS"/>
AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="org.o7planning.smsmanagerexample">
<uses-permission android:name="android.permission.SEND_SMS"/>
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
Finally, the interface of the application looks like this:
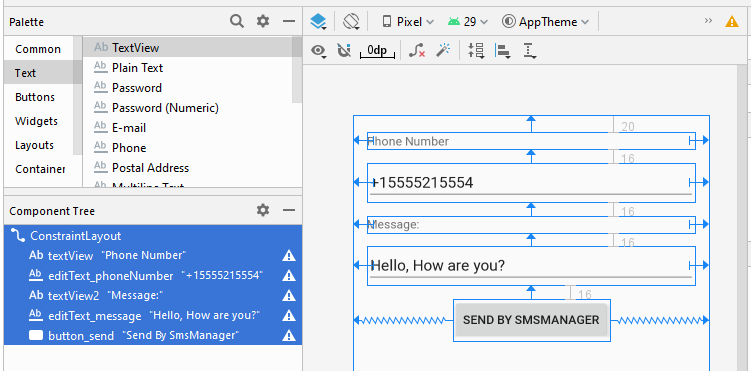
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="20dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Phone Number"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<EditText
android:id="@+id/editText_phoneNumber"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:ems="10"
android:hint="Phone Number"
android:inputType="phone"
android:text="+15555215554"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView" />
<TextView
android:id="@+id/textView2"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Message:"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/editText_phoneNumber" />
<EditText
android:id="@+id/editText_message"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:ems="10"
android:inputType="text"
android:text="Hello, How are you?"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView2" />
<Button
android:id="@+id/button_send"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="16dp"
android:text="Send By SmsManager"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/editText_message" />
</androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.xml
package org.o7planning.smsmanagerexample;
import androidx.appcompat.app.AppCompatActivity;
import androidx.core.app.ActivityCompat;
import android.Manifest;
import android.content.Intent;
import android.content.pm.PackageManager;
import android.os.Bundle;
import android.telephony.SmsManager;
import android.util.Log;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
private static final int MY_PERMISSION_REQUEST_CODE_SEND_SMS = 1;
private static final String LOG_TAG = "AndroidExample";
private EditText editTextPhoneNumber;
private EditText editTextMessage;
private Button buttonSend;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.editTextPhoneNumber = (EditText) this.findViewById(R.id.editText_phoneNumber);
this.editTextMessage = (EditText) this.findViewById(R.id.editText_message);
this.buttonSend = (Button) this.findViewById(R.id.button_send);
this.buttonSend.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
askPermissionAndSendSMS();
}
});
}
private void askPermissionAndSendSMS() {
// With Android Level >= 23, you have to ask the user
// for permission to send SMS.
if (android.os.Build.VERSION.SDK_INT >= android.os.Build.VERSION_CODES.M) { // 23
// Check if we have send SMS permission
int sendSmsPermisson = ActivityCompat.checkSelfPermission(this,
Manifest.permission.SEND_SMS);
if (sendSmsPermisson != PackageManager.PERMISSION_GRANTED) {
// If don't have permission so prompt the user.
this.requestPermissions(
new String[]{Manifest.permission.SEND_SMS},
MY_PERMISSION_REQUEST_CODE_SEND_SMS
);
return;
}
}
this.sendSMS_by_smsManager();
}
private void sendSMS_by_smsManager() {
String phoneNumber = this.editTextPhoneNumber.getText().toString();
String message = this.editTextMessage.getText().toString();
try {
// Get the default instance of the SmsManager
SmsManager smsManager = SmsManager.getDefault();
// Send Message
smsManager.sendTextMessage(phoneNumber,
null,
message,
null,
null);
Log.i( LOG_TAG,"Your sms has successfully sent!");
Toast.makeText(getApplicationContext(),"Your sms has successfully sent!",
Toast.LENGTH_LONG).show();
} catch (Exception ex) {
Log.e( LOG_TAG,"Your sms has failed...", ex);
Toast.makeText(getApplicationContext(),"Your sms has failed... " + ex.getMessage(),
Toast.LENGTH_LONG).show();
ex.printStackTrace();
}
}
// When you have the request results
@Override
public void onRequestPermissionsResult(int requestCode,
String permissions[], int[] grantResults) {
super.onRequestPermissionsResult(requestCode, permissions, grantResults);
//
switch (requestCode) {
case MY_PERMISSION_REQUEST_CODE_SEND_SMS: {
// Note: If request is cancelled, the result arrays are empty.
// Permissions granted (SEND_SMS).
if (grantResults.length > 0
&& grantResults[0] == PackageManager.PERMISSION_GRANTED) {
Log.i( LOG_TAG,"Permission granted!");
Toast.makeText(this, "Permission granted!", Toast.LENGTH_LONG).show();
this.sendSMS_by_smsManager();
}
// Cancelled or denied.
else {
Log.i( LOG_TAG,"Permission denied!");
Toast.makeText(this, "Permission denied!", Toast.LENGTH_LONG).show();
}
break;
}
}
}
// When results returned
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (requestCode == MY_PERMISSION_REQUEST_CODE_SEND_SMS) {
if (resultCode == RESULT_OK) {
// Do something with data (Result returned).
Toast.makeText(this, "Action OK", Toast.LENGTH_LONG).show();
} else if (resultCode == RESULT_CANCELED) {
Toast.makeText(this, "Action canceled", Toast.LENGTH_LONG).show();
} else {
Toast.makeText(this, "Action Failed", Toast.LENGTH_LONG).show();
}
}
}
}
3. Send an SMS text message by Intent.ACTION_SEND
In your application, you can create an implicit Intent and sent it to the Android system in order to have the system send your messages. The system will launch the best SMS sending app available in the system to do this, and it is most likely to be the Messenger application.
The Messenger is an SMS message sending application available in Android that is familiar to almost all users.
Example preview:
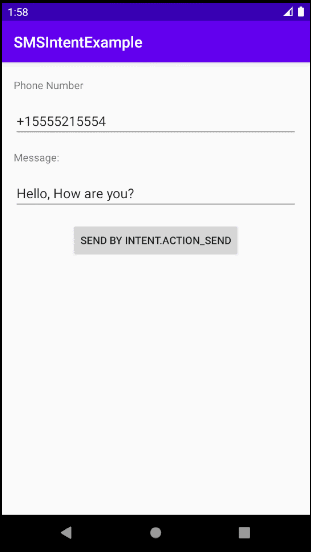
On Android Studio, create a project:
- Name: SMSIntentExample
- Package name: org.o7planning.smsintentexample
Then add the following XML code to the AndroidManifest.xml file to allow the application to send SMS text messages.
<uses-permission android:name="android.permission.SEND_SMS"/>
AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="org.o7planning.smsintentexample">
<uses-permission android:name="android.permission.SEND_SMS"/>
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
The interface of the example application:
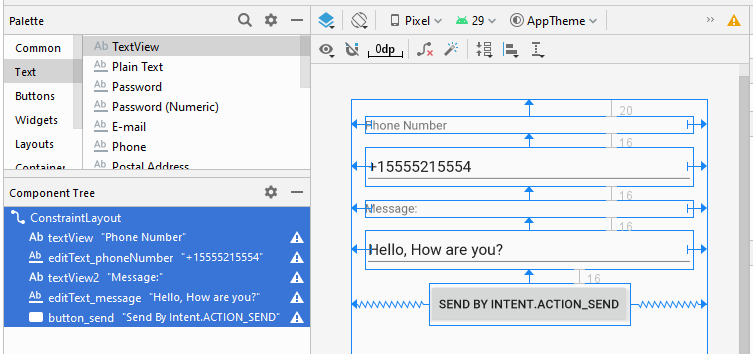
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="20dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Phone Number"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<EditText
android:id="@+id/editText_phoneNumber"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:ems="10"
android:hint="Phone Number"
android:inputType="phone"
android:text="+15555215554"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView" />
<TextView
android:id="@+id/textView2"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Message:"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/editText_phoneNumber" />
<EditText
android:id="@+id/editText_message"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:ems="10"
android:inputType="text"
android:text="Hello, How are you?"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView2" />
<Button
android:id="@+id/button_send"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="16dp"
android:text="Send By Intent.ACTION_SEND"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/editText_message" />
</androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.java
package org.o7planning.smsintentexample;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Intent;
import android.net.Uri;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
private static final String LOG_TAG = "AndroidExample";
private EditText editTextPhoneNumber;
private EditText editTextMessage;
private Button buttonSend;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.editTextPhoneNumber = (EditText) this.findViewById(R.id.editText_phoneNumber);
this.editTextMessage = (EditText) this.findViewById(R.id.editText_message);
this.buttonSend = (Button) this.findViewById(R.id.button_send);
this.buttonSend.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
sendSMS_by_Intent_ACTION_SEND();
}
});
}
private void sendSMS_by_Intent_ACTION_SEND() {
String phoneNumber = this.editTextPhoneNumber.getText().toString();
String message = this.editTextMessage.getText().toString();
// Add the phone number in the data
Uri uri = Uri.parse("smsto:" + phoneNumber);
Intent smsIntent = new Intent(Intent.ACTION_SENDTO, uri);
// Add the message at the sms_body extra field
smsIntent.putExtra("sms_body", message);
try {
startActivity(smsIntent);
} catch (Exception ex) {
Log.e(LOG_TAG, "Your sms has failed... " + ex.getMessage(), ex);
Toast.makeText(MainActivity.this, "Your sms has failed... " + ex.getMessage(),
Toast.LENGTH_LONG).show();
ex.printStackTrace();
}
}
}
Android Programming Tutorials
- Configure Android Emulator in Android Studio
- Android ToggleButton Tutorial with Examples
- Create a simple File Finder Dialog in Android
- Android TimePickerDialog Tutorial with Examples
- Android DatePickerDialog Tutorial with Examples
- What is needed to get started with Android?
- Install Android Studio on Windows
- Install Intel® HAXM for Android Studio
- Android AsyncTask Tutorial with Examples
- Android AsyncTaskLoader Tutorial with Examples
- Android Tutorial for Beginners - Basic examples
- How to know the phone number of Android Emulator and change it
- Android TextInputLayout Tutorial with Examples
- Android CardView Tutorial with Examples
- Android ViewPager2 Tutorial with Examples
- Get Phone Number in Android using TelephonyManager
- Android Phone Call Tutorial with Examples
- Android Wifi Scanning Tutorial with Examples
- Android 2D Game Tutorial for Beginners
- Android DialogFragment Tutorial with Examples
- Android CharacterPickerDialog Tutorial with Examples
- Android Tutorial for Beginners - Hello Android
- Using Android Device File Explorer
- Enable USB Debugging on Android Device
- Android UI Layouts Tutorial with Examples
- Android SMS Tutorial with Examples
- Android SQLite Database Tutorial with Examples
- Google Maps Android API Tutorial with Examples
- Android Text to Speech Tutorial with Examples
- Android Space Tutorial with Examples
- Android Toast Tutorial with Examples
- Create a custom Android Toast
- Android SnackBar Tutorial with Examples
- Android TextView Tutorial with Examples
- Android TextClock Tutorial with Examples
- Android EditText Tutorial with Examples
- Android TextWatcher Tutorial with Examples
- Format Credit Card Number with Android TextWatcher
- Android Clipboard Tutorial with Examples
- Create a simple File Chooser in Android
- Android AutoCompleteTextView and MultiAutoCompleteTextView Tutorial with Examples
- Android ImageView Tutorial with Examples
- Android ImageSwitcher Tutorial with Examples
- Android ScrollView and HorizontalScrollView Tutorial with Examples
- Android WebView Tutorial with Examples
- Android SeekBar Tutorial with Examples
- Android Dialog Tutorial with Examples
- Android AlertDialog Tutorial with Examples
- Android RatingBar Tutorial with Examples
- Android ProgressBar Tutorial with Examples
- Android Spinner Tutorial with Examples
- Android Button Tutorial with Examples
- Android Switch Tutorial with Examples
- Android ImageButton Tutorial with Examples
- Android FloatingActionButton Tutorial with Examples
- Android CheckBox Tutorial with Examples
- Android RadioGroup and RadioButton Tutorial with Examples
- Android Chip and ChipGroup Tutorial with Examples
- Using image assets and icon assets of Android Studio
- Setting SD Card for Android Emulator
- ChipGroup and Chip Entry Example
- How to add external libraries to Android Project in Android Studio?
- How to disable the permissions already granted to the Android application?
- How to remove applications from Android Emulator?
- Android LinearLayout Tutorial with Examples
- Android TableLayout Tutorial with Examples
- Android FrameLayout Tutorial with Examples
- Android QuickContactBadge Tutorial with Examples
- Android StackView Tutorial with Examples
- Android Camera Tutorial with Examples
- Android MediaPlayer Tutorial with Examples
- Android VideoView Tutorial with Examples
- Playing Sound effects in Android with SoundPool
- Android Networking Tutorial with Examples
- Android JSON Parser Tutorial with Examples
- Android SharedPreferences Tutorial with Examples
- Android Internal Storage Tutorial with Examples
- Android External Storage Tutorial with Examples
- Android Intents Tutorial with Examples
- Example of an explicit Android Intent, calling another Intent
- Example of implicit Android Intent, open a URL, send an email
- Android Services Tutorial with Examples
- Android Notifications Tutorial with Examples
- Android DatePicker Tutorial with Examples
- Android TimePicker Tutorial with Examples
- Android Chronometer Tutorial with Examples
- Android OptionMenu Tutorial with Examples
- Android ContextMenu Tutorial with Examples
- Android PopupMenu Tutorial with Examples
- Android Fragments Tutorial with Examples
- Android ListView Tutorial with Examples
- Android ListView with Checkbox using ArrayAdapter
- Android GridView Tutorial with Examples
Show More