Android Camera Tutorial with Examples
1. Android Camera
Camera is a device that allows you to take pictures or record a video. In Android there are 2 ways that you work with the camera.
Method 1:
On the Android operating system, application to work with the camera is available , your application can call the application via an implicit Intent to require a certain action with the camera, such ask Camera to open and take pictures, or ask Camera to record a video, and then the results returned.
Method 2:
Android provides you the API to work directly with the camera.
With Android Level < 21 you can work directly with the camera via android.hardware.Camera class, but this class is Deprecated and is no longer used in Android Level >= 21, recommend camera2 API use.
With Android Level < 21 you can work directly with the camera via android.hardware.Camera class, but this class is Deprecated and is no longer used in Android Level >= 21, recommend camera2 API use.
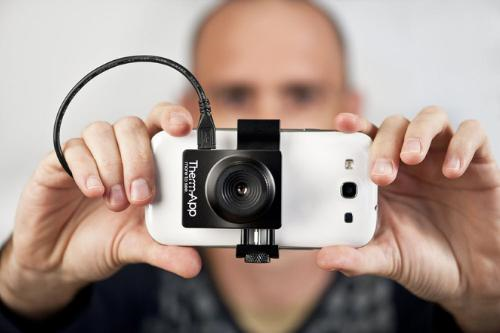
In this document I will guide you to use implicit intent to call into the Camera application is available in the system for opening camera to take a picture or record a video.
You can see "Android Camera2 API" at:
- Android Camera API2
2. Overview
On the Android operating system, it is available on application to work with the camera, on your application you can create an implicit Intent to call this application, request for camera to take a picture or record a video.
// Create an implicit intent, for image capture.
Intent intent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE);
int REQUEST_ID_IMAGE_CAPTURE = 100;
// Start camera and wait for the results.
this.startActivityForResult(intent, REQUEST_ID_IMAGE_CAPTURE);
Intent type for Camera:
Intent Type | Description |
ACTION_IMAGE_CAPTURE_SECURE | It returns the image captured from the camera , when the device is secured |
ACTION_VIDEO_CAPTURE | It calls the existing video application in android to capture video |
EXTRA_SCREEN_ORIENTATION | It is used to set the orientation of the screen to vertical or landscape |
EXTRA_FULL_SCREEN | It is used to control the user interface of the ViewImage |
INTENT_ACTION_VIDEO_CAMERA | This intent is used to launch the camera in the video mode |
EXTRA_SIZE_LIMIT | It is used to specify the size limit of video or image capture size |
In case you want to save photos or video just recorded on the device you need to configure the permissions to read and write data to the device. Configure on AndroidManifest.xml.
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE" />
With Android Level> = 23, you need to use the code to ask the user for permission to read and write data to the device.
// With Android Level >= 23, you have to ask the user
// for permission to read/write data on the device.
if (android.os.Build.VERSION.SDK_INT >= 23) {
// Check if we have read/write permission
// Kiểm tra quyền đọc/ghi dữ liệu vào thiết bị lưu trữ ngoài.
int readPermission = ActivityCompat.checkSelfPermission(this,
Manifest.permission.READ_EXTERNAL_STORAGE);
int writePermission = ActivityCompat.checkSelfPermission(this,
Manifest.permission.WRITE_EXTERNAL_STORAGE);
if (writePermission != PackageManager.PERMISSION_GRANTED ||
readPermission != PackageManager.PERMISSION_GRANTED) {
// If don't have permission so prompt the user.
this.requestPermissions(
new String[]{Manifest.permission.WRITE_EXTERNAL_STORAGE,
Manifest.permission.READ_EXTERNAL_STORAGE},
REQUEST_ID_READ_WRITE_PERMISSION
);
}
}
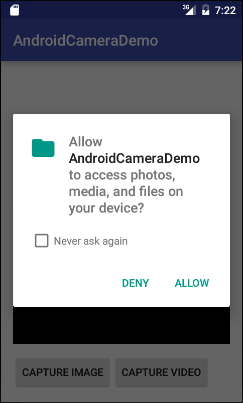
Handling when users reply to the request.
// When you have the request results
@Override
public void onRequestPermissionsResult(int requestCode,
String permissions[], int[] grantResults) {
super.onRequestPermissionsResult(requestCode, permissions, grantResults);
//
switch (requestCode) {
case REQUEST_ID_READ_WRITE_PERMISSION: {
// Note: If request is cancelled, the result arrays are empty.
// Permissions granted (read/write).
if (grantResults.length > 1
&& grantResults[0] == PackageManager.PERMISSION_GRANTED
&& grantResults[1] == PackageManager.PERMISSION_GRANTED) {
Toast.makeText(this, "Permission granted!", Toast.LENGTH_LONG).show();
this.captureVideo();
}
// Cancelled or denied.
else {
Toast.makeText(this, "Permission denied!", Toast.LENGTH_LONG).show();
}
break;
}
}
}
3. Example
Create project named AndroidCameraDemo:
- File > New > New Project > Empty Activity
- Name: AndroidCameraDemo
- Package name: org.o7planning.androidcamerademo
- Language: Java
Add Configuration allows read and write data on the device.
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE" />
AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="org.o7planning.androidcamerademo">
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE" />
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
Interface design
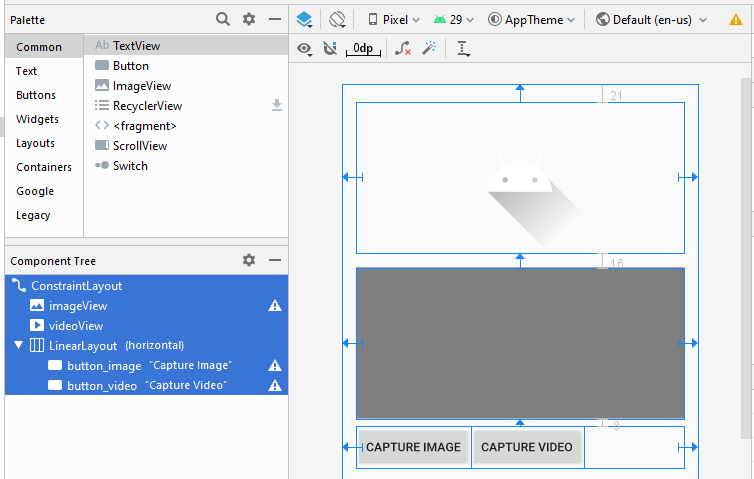
If you are interested in the steps to design this application interface, see the appendix at the end of the article.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<ImageView
android:id="@+id/imageView"
android:layout_width="0dp"
android:layout_height="175dp"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="21dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:srcCompat="@drawable/ic_launcher_foreground"
tools:ignore="VectorDrawableCompat" />
<VideoView
android:id="@+id/videoView"
android:layout_width="0dp"
android:layout_height="175dp"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/imageView" />
<LinearLayout
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:orientation="horizontal"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/videoView">
<Button
android:id="@+id/button_image"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="0"
android:text="Capture Image" />
<Button
android:id="@+id/button_video"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="0"
android:text="Capture Video" />
</LinearLayout>
</androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.java
package org.o7planning.androidcamerademo;
import androidx.appcompat.app.AppCompatActivity;
import androidx.core.app.ActivityCompat;
import android.os.Bundle;
import android.Manifest;
import android.content.Intent;
import android.content.pm.PackageManager;
import android.graphics.Bitmap;
import android.net.Uri;
import android.os.Environment;
import android.os.StrictMode;
import android.provider.MediaStore;
import android.util.Log;
import android.view.View;
import android.widget.Button;
import android.widget.ImageView;
import android.widget.Toast;
import android.widget.VideoView;
import java.io.File;
public class MainActivity extends AppCompatActivity {
private Button buttonImage;
private Button buttonVideo;
private VideoView videoView;
private ImageView imageView;
private static final int REQUEST_ID_READ_WRITE_PERMISSION = 99;
private static final int REQUEST_ID_IMAGE_CAPTURE = 100;
private static final int REQUEST_ID_VIDEO_CAPTURE = 101;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.buttonImage = (Button) this.findViewById(R.id.button_image);
this.buttonVideo = (Button) this.findViewById(R.id.button_video);
this.videoView = (VideoView) this.findViewById(R.id.videoView);
this.imageView = (ImageView) this.findViewById(R.id.imageView);
this.buttonImage.setOnClickListener(new Button.OnClickListener() {
@Override
public void onClick(View v) {
captureImage();
}
});
this.buttonVideo.setOnClickListener(new Button.OnClickListener() {
@Override
public void onClick(View v) {
askPermissionAndCaptureVideo();
}
});
}
private void captureImage() {
// Create an implicit intent, for image capture.
Intent intent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE);
// Start camera and wait for the results.
this.startActivityForResult(intent, REQUEST_ID_IMAGE_CAPTURE);
}
private void askPermissionAndCaptureVideo() {
// With Android Level >= 23, you have to ask the user
// for permission to read/write data on the device.
if (android.os.Build.VERSION.SDK_INT >= 23) {
// Check if we have read/write permission
int readPermission = ActivityCompat.checkSelfPermission(this,
Manifest.permission.READ_EXTERNAL_STORAGE);
int writePermission = ActivityCompat.checkSelfPermission(this,
Manifest.permission.WRITE_EXTERNAL_STORAGE);
if (writePermission != PackageManager.PERMISSION_GRANTED ||
readPermission != PackageManager.PERMISSION_GRANTED) {
// If don't have permission so prompt the user.
this.requestPermissions(
new String[]{Manifest.permission.WRITE_EXTERNAL_STORAGE,
Manifest.permission.READ_EXTERNAL_STORAGE},
REQUEST_ID_READ_WRITE_PERMISSION
);
return;
}
}
this.captureVideo();
}
private void captureVideo() {
try {
// Create an implicit intent, for video capture.
Intent intent = new Intent(MediaStore.ACTION_VIDEO_CAPTURE);
// The external storage directory.
File dir = Environment.getExternalStorageDirectory();
if (!dir.exists()) {
dir.mkdirs();
}
// file:///storage/emulated/0/myvideo.mp4
String savePath = dir.getAbsolutePath() + "/myvideo.mp4";
File videoFile = new File(savePath);
Uri videoUri = Uri.fromFile(videoFile);
// Specify where to save video files.
intent.putExtra(MediaStore.EXTRA_OUTPUT, videoUri);
intent.addFlags(Intent.FLAG_GRANT_READ_URI_PERMISSION);
// ================================================================================================
// To Fix Error (**)
// ================================================================================================
StrictMode.VmPolicy.Builder builder = new StrictMode.VmPolicy.Builder();
StrictMode.setVmPolicy(builder.build());
// ================================================================================================
// You may get an Error (**) If your app targets API 24+
// "android.os.FileUriExposedException: file:///storage/emulated/0/xxx exposed beyond app through.."
// Explanation: https://stackoverflow.com/questions/38200282
// ================================================================================================
// Start camera and wait for the results.
this.startActivityForResult(intent, REQUEST_ID_VIDEO_CAPTURE); // (**)
} catch(Exception e) {
Toast.makeText(this, "Error capture video: " +e.getMessage(), Toast.LENGTH_LONG).show();
e.printStackTrace();
}
}
// When you have the request results
@Override
public void onRequestPermissionsResult(int requestCode,
String permissions[], int[] grantResults) {
super.onRequestPermissionsResult(requestCode, permissions, grantResults);
//
switch (requestCode) {
case REQUEST_ID_READ_WRITE_PERMISSION: {
// Note: If request is cancelled, the result arrays are empty.
// Permissions granted (read/write).
if (grantResults.length > 1
&& grantResults[0] == PackageManager.PERMISSION_GRANTED
&& grantResults[1] == PackageManager.PERMISSION_GRANTED) {
Toast.makeText(this, "Permission granted!", Toast.LENGTH_LONG).show();
this.captureVideo();
}
// Cancelled or denied.
else {
Toast.makeText(this, "Permission denied!", Toast.LENGTH_LONG).show();
}
break;
}
}
}
// When results returned
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (requestCode == REQUEST_ID_IMAGE_CAPTURE) {
if (resultCode == RESULT_OK) {
Bitmap bp = (Bitmap) data.getExtras().get("data");
this.imageView.setImageBitmap(bp);
} else if (resultCode == RESULT_CANCELED) {
Toast.makeText(this, "Action canceled", Toast.LENGTH_LONG).show();
} else {
Toast.makeText(this, "Action Failed", Toast.LENGTH_LONG).show();
}
} else if (requestCode == REQUEST_ID_VIDEO_CAPTURE) {
if (resultCode == RESULT_OK) {
Uri videoUri = data.getData();
Log.i("MyLog", "Video saved to: " + videoUri);
Toast.makeText(this, "Video saved to:\n" +
videoUri, Toast.LENGTH_LONG).show();
this.videoView.setVideoURI(videoUri);
this.videoView.start();
} else if (resultCode == RESULT_CANCELED) {
Toast.makeText(this, "Action Cancelled.",
Toast.LENGTH_LONG).show();
} else {
Toast.makeText(this, "Action Failed",
Toast.LENGTH_LONG).show();
}
}
}
}
The app will save video to SD Card of the Emulator, so make sure you have SD Card set up.
OK, now you can run the application. Here, I run the app on simulation device with simulation camera.
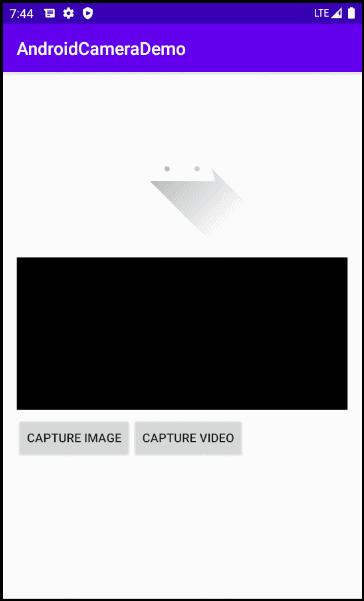
Android Programming Tutorials
- Configure Android Emulator in Android Studio
- Android ToggleButton Tutorial with Examples
- Create a simple File Finder Dialog in Android
- Android TimePickerDialog Tutorial with Examples
- Android DatePickerDialog Tutorial with Examples
- What is needed to get started with Android?
- Install Android Studio on Windows
- Install Intel® HAXM for Android Studio
- Android AsyncTask Tutorial with Examples
- Android AsyncTaskLoader Tutorial with Examples
- Android Tutorial for Beginners - Basic examples
- How to know the phone number of Android Emulator and change it
- Android TextInputLayout Tutorial with Examples
- Android CardView Tutorial with Examples
- Android ViewPager2 Tutorial with Examples
- Get Phone Number in Android using TelephonyManager
- Android Phone Call Tutorial with Examples
- Android Wifi Scanning Tutorial with Examples
- Android 2D Game Tutorial for Beginners
- Android DialogFragment Tutorial with Examples
- Android CharacterPickerDialog Tutorial with Examples
- Android Tutorial for Beginners - Hello Android
- Using Android Device File Explorer
- Enable USB Debugging on Android Device
- Android UI Layouts Tutorial with Examples
- Android SMS Tutorial with Examples
- Android SQLite Database Tutorial with Examples
- Google Maps Android API Tutorial with Examples
- Android Text to Speech Tutorial with Examples
- Android Space Tutorial with Examples
- Android Toast Tutorial with Examples
- Create a custom Android Toast
- Android SnackBar Tutorial with Examples
- Android TextView Tutorial with Examples
- Android TextClock Tutorial with Examples
- Android EditText Tutorial with Examples
- Android TextWatcher Tutorial with Examples
- Format Credit Card Number with Android TextWatcher
- Android Clipboard Tutorial with Examples
- Create a simple File Chooser in Android
- Android AutoCompleteTextView and MultiAutoCompleteTextView Tutorial with Examples
- Android ImageView Tutorial with Examples
- Android ImageSwitcher Tutorial with Examples
- Android ScrollView and HorizontalScrollView Tutorial with Examples
- Android WebView Tutorial with Examples
- Android SeekBar Tutorial with Examples
- Android Dialog Tutorial with Examples
- Android AlertDialog Tutorial with Examples
- Android RatingBar Tutorial with Examples
- Android ProgressBar Tutorial with Examples
- Android Spinner Tutorial with Examples
- Android Button Tutorial with Examples
- Android Switch Tutorial with Examples
- Android ImageButton Tutorial with Examples
- Android FloatingActionButton Tutorial with Examples
- Android CheckBox Tutorial with Examples
- Android RadioGroup and RadioButton Tutorial with Examples
- Android Chip and ChipGroup Tutorial with Examples
- Using image assets and icon assets of Android Studio
- Setting SD Card for Android Emulator
- ChipGroup and Chip Entry Example
- How to add external libraries to Android Project in Android Studio?
- How to disable the permissions already granted to the Android application?
- How to remove applications from Android Emulator?
- Android LinearLayout Tutorial with Examples
- Android TableLayout Tutorial with Examples
- Android FrameLayout Tutorial with Examples
- Android QuickContactBadge Tutorial with Examples
- Android StackView Tutorial with Examples
- Android Camera Tutorial with Examples
- Android MediaPlayer Tutorial with Examples
- Android VideoView Tutorial with Examples
- Playing Sound effects in Android with SoundPool
- Android Networking Tutorial with Examples
- Android JSON Parser Tutorial with Examples
- Android SharedPreferences Tutorial with Examples
- Android Internal Storage Tutorial with Examples
- Android External Storage Tutorial with Examples
- Android Intents Tutorial with Examples
- Example of an explicit Android Intent, calling another Intent
- Example of implicit Android Intent, open a URL, send an email
- Android Services Tutorial with Examples
- Android Notifications Tutorial with Examples
- Android DatePicker Tutorial with Examples
- Android TimePicker Tutorial with Examples
- Android Chronometer Tutorial with Examples
- Android OptionMenu Tutorial with Examples
- Android ContextMenu Tutorial with Examples
- Android PopupMenu Tutorial with Examples
- Android Fragments Tutorial with Examples
- Android ListView Tutorial with Examples
- Android ListView with Checkbox using ArrayAdapter
- Android GridView Tutorial with Examples
Show More