Android Intents Tutorial with Examples
1. What is Intent?
Intents are asynchronous messages which allow application components to request functionality from other Android components. Intents allow you to interact with components from the same applications as well as with components contributed by other applications. For example, an activity can start an external activity for taking a picture.
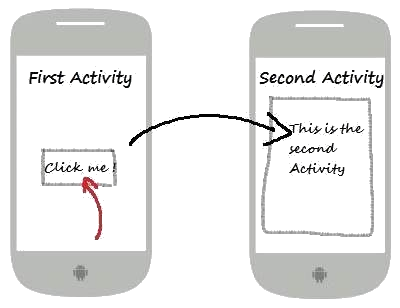
Intents are objects of the android.content.Intent type. Your code can send them to the Android system defining the components you are targeting.
For example, via the startActivity() method you can define that the intent should be used to start an activity. In the target Activity, via startActivity() method, you can determine the sender's intention to start this Activity.
An intent can contain data via a Bundle. This data can be used by the receiving component.
For example, via the startActivity() method you can define that the intent should be used to start an activity. In the target Activity, via startActivity() method, you can determine the sender's intention to start this Activity.
An intent can contain data via a Bundle. This data can be used by the receiving component.
Intent can be used to:
- Start an Activity
- Start sub-activity.
- Start a Service.
After learning about Intent, you can find more details about Android Service at:
2. The types of Intent
Android supports explicit and implicit intents.
An application can define the target component directly in the intent (explicit intent) or ask the Android system to evaluate registered components based on the intent data (implicit intents).
An application can define the target component directly in the intent (explicit intent) or ask the Android system to evaluate registered components based on the intent data (implicit intents).
3. Explicit Intent
Explicit intents: These explicitly specify the name of the target component to handle the intent; in these, the optional component name field (above) is set to a particular value, through the setComponent() or setClass() methods.
Create Intent example:
// Create the Intent with the target of Greeting Activity.
// Intent(FirstActivity, SecondActivity.class)
Intent intent = new Intent(this,GreetingActivity.class);
// The data attached
intent.putExtra("firstName",firstName);
intent.putExtra("lastName", lastName);
// Start Activity (GreetingActivity)
// (No need feedback from the activity is called)
this.startActivity(intent);
// Start Activity and will have a feedback from the activity is called.
this.startActivityForResult(intent, MY_REQUEST_CODE);
Or:
// Way 1.
Intent mIntent = new Intent(this, GreetingActivity.class);
Bundle extras = mIntent.getExtras();
extras.putString("firstName", "<firstName>");
extras.putString("látName", "<lastName>");
// Way 2.
Intent mIntent2 = new Intent(this, GreetingActivity.class);
Bundle mBundle = new Bundle();
mBundle.putString("firstName", "<firstName>");
mBundle.putString("látName", "<lastName>");
mIntent2.putExtras(mBundle);
// Way 3:
// Using putExtra()
Intent mIntent3 = new Intent(this, GreetingActivity.class);
mIntent3.putExtra("firstName", "<firstName>");
mIntent3.putExtra("látName", "<lastName>");
In the target Activity:
Intent intent = this.getIntent();
String firstName= intent.getStringExtra("firstName");
String lastName = intent.getStringExtra("lastName");
// Or
Bundle extras = this.getIntent().getExtras();
String firstName1 = extras.getString("firstName");
String lastName2 = extras.getString("lastName");
OK, you can see the following simple example:
4. Intent Filter
When you create new an Activity or Service, you need to declare it to AndroidManifest.xml, normally, when you create it with helps of wizard in Android Studio, registration code is automatically created in AndroidManifest.xml. For example:
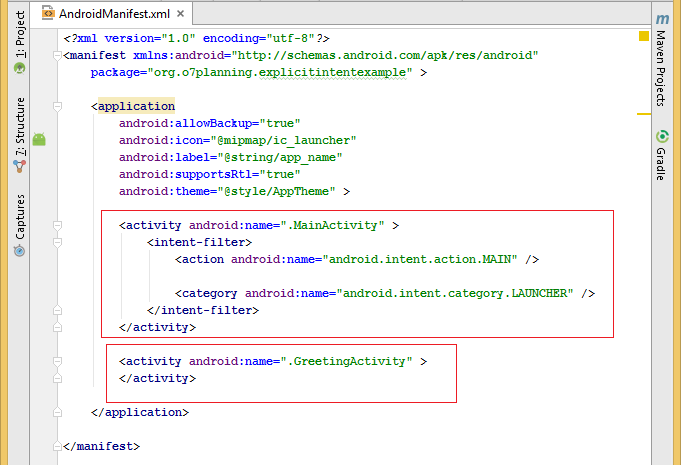
You may notice that in the declaration of Activity on AndroidManifest.xml may have <intent-filter> tag, this tag specifies the type of Intent sent to it (Activity) that it can accept.
When you create an implicit intent, the Android system finds the appropriate component to start by comparing the contents of the intent to the intent filters declared in the manifest file of other apps on the device. If the intent matches an intent filter, the system starts that component and delivers it the Intent object.
Example:
** AndroidManifest.xml **
.....
<activity android:name=".HelloWorld"
android:label="@string/app_name">
<intent-filter>
<action android:name="android.intent.action.VIEW"/>
<category android:name="android.intent.category.DEFAULT"/>
<category android:name="android.intent.category.BROWSABLE"/>
<data android:scheme="http" android:host="o7planning.org"/>
</intent-filter>
</activity>
...
For example, create an implicit intent can call the above Activity (It matches the intent filters).
// An implicit intent, requested to view a URL
Intent intent = new Intent (Intent.ACTION_VIEW, Uri.parse("http://o7planning.org"));
startActivity(intent);
5. Implicit intent
Implicit intents: These do not specify a target component, but include enough information for the system to determine which of the available components is best to run for that intent. Consider an app that lists the available restaurants near you. When you click a particular restaurant option, the application has to ask another application to display the route to that restaurant. To achieve this, it could either send an explicit intent directly to the Google Maps application, or send an implicit intent, which would be delivered to any application that provides the Maps functionality (e.g., Yahoo Maps).
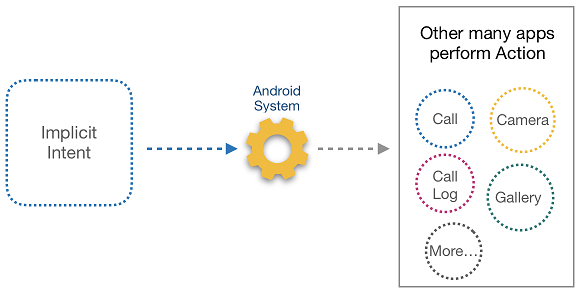
Some examples with implicit Intent:An example with implicit Intent, when you click a button, the application sent to the Android system a request to take a photograph by Camera and save image to a file.
Android Programming Tutorials
- Configure Android Emulator in Android Studio
- Android ToggleButton Tutorial with Examples
- Create a simple File Finder Dialog in Android
- Android TimePickerDialog Tutorial with Examples
- Android DatePickerDialog Tutorial with Examples
- What is needed to get started with Android?
- Install Android Studio on Windows
- Install Intel® HAXM for Android Studio
- Android AsyncTask Tutorial with Examples
- Android AsyncTaskLoader Tutorial with Examples
- Android Tutorial for Beginners - Basic examples
- How to know the phone number of Android Emulator and change it
- Android TextInputLayout Tutorial with Examples
- Android CardView Tutorial with Examples
- Android ViewPager2 Tutorial with Examples
- Get Phone Number in Android using TelephonyManager
- Android Phone Call Tutorial with Examples
- Android Wifi Scanning Tutorial with Examples
- Android 2D Game Tutorial for Beginners
- Android DialogFragment Tutorial with Examples
- Android CharacterPickerDialog Tutorial with Examples
- Android Tutorial for Beginners - Hello Android
- Using Android Device File Explorer
- Enable USB Debugging on Android Device
- Android UI Layouts Tutorial with Examples
- Android SMS Tutorial with Examples
- Android SQLite Database Tutorial with Examples
- Google Maps Android API Tutorial with Examples
- Android Text to Speech Tutorial with Examples
- Android Space Tutorial with Examples
- Android Toast Tutorial with Examples
- Create a custom Android Toast
- Android SnackBar Tutorial with Examples
- Android TextView Tutorial with Examples
- Android TextClock Tutorial with Examples
- Android EditText Tutorial with Examples
- Android TextWatcher Tutorial with Examples
- Format Credit Card Number with Android TextWatcher
- Android Clipboard Tutorial with Examples
- Create a simple File Chooser in Android
- Android AutoCompleteTextView and MultiAutoCompleteTextView Tutorial with Examples
- Android ImageView Tutorial with Examples
- Android ImageSwitcher Tutorial with Examples
- Android ScrollView and HorizontalScrollView Tutorial with Examples
- Android WebView Tutorial with Examples
- Android SeekBar Tutorial with Examples
- Android Dialog Tutorial with Examples
- Android AlertDialog Tutorial with Examples
- Android RatingBar Tutorial with Examples
- Android ProgressBar Tutorial with Examples
- Android Spinner Tutorial with Examples
- Android Button Tutorial with Examples
- Android Switch Tutorial with Examples
- Android ImageButton Tutorial with Examples
- Android FloatingActionButton Tutorial with Examples
- Android CheckBox Tutorial with Examples
- Android RadioGroup and RadioButton Tutorial with Examples
- Android Chip and ChipGroup Tutorial with Examples
- Using image assets and icon assets of Android Studio
- Setting SD Card for Android Emulator
- ChipGroup and Chip Entry Example
- How to add external libraries to Android Project in Android Studio?
- How to disable the permissions already granted to the Android application?
- How to remove applications from Android Emulator?
- Android LinearLayout Tutorial with Examples
- Android TableLayout Tutorial with Examples
- Android FrameLayout Tutorial with Examples
- Android QuickContactBadge Tutorial with Examples
- Android StackView Tutorial with Examples
- Android Camera Tutorial with Examples
- Android MediaPlayer Tutorial with Examples
- Android VideoView Tutorial with Examples
- Playing Sound effects in Android with SoundPool
- Android Networking Tutorial with Examples
- Android JSON Parser Tutorial with Examples
- Android SharedPreferences Tutorial with Examples
- Android Internal Storage Tutorial with Examples
- Android External Storage Tutorial with Examples
- Android Intents Tutorial with Examples
- Example of an explicit Android Intent, calling another Intent
- Example of implicit Android Intent, open a URL, send an email
- Android Services Tutorial with Examples
- Android Notifications Tutorial with Examples
- Android DatePicker Tutorial with Examples
- Android TimePicker Tutorial with Examples
- Android Chronometer Tutorial with Examples
- Android OptionMenu Tutorial with Examples
- Android ContextMenu Tutorial with Examples
- Android PopupMenu Tutorial with Examples
- Android Fragments Tutorial with Examples
- Android ListView Tutorial with Examples
- Android ListView with Checkbox using ArrayAdapter
- Android GridView Tutorial with Examples
Show More