Android OptionMenu Tutorial with Examples
1. Android Option Menu
In Android, an Option Menu is a set of primary options of an application which users can select one of the options to perform an action. The Option Menu appears on the right side of the App Bar.
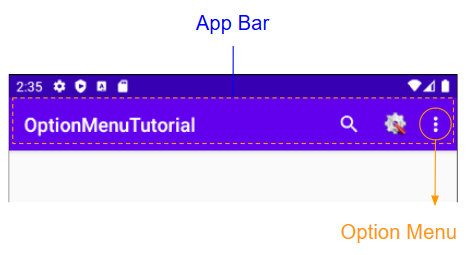
Let's have a look at the XML codes below to create a simple Option Menu.
<?xml version="1.0" encoding="utf-8"?>
<menu xmlns:android="http://schemas.android.com/apk/res/android">
<item android:title="Menu Item 1" />
<item android:title="Menu Item 2" >
<menu >
<item android:title="Menu Item 2.1" />
<item android:title="Menu Item 2.2" />
</menu>
</item>
<item android:title="Menu Item 3" />
</menu>
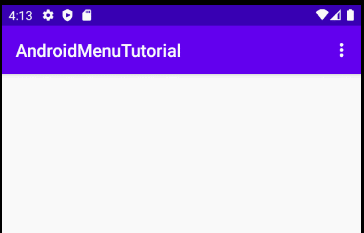
Users need to press the icon
to display the Option Menu.
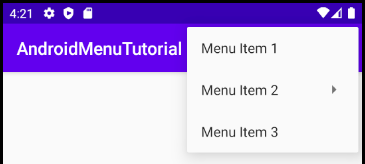
A Menu may contain one or more Menu Items and Sub Menus.
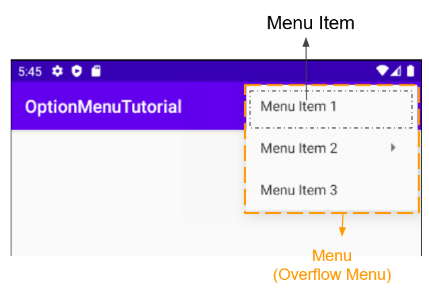
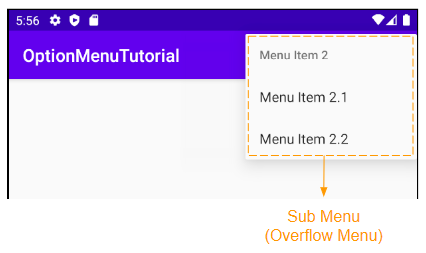
Before Android 3.0, you could easily set icons for the Menu Items. However, after Google's design thinking had changed, they wanted developers to put all the functions on the App Bar instead of the Menu, and they no longer allowed icons to be displayed on the Menu Items of the Overflow Menu. Yet, there are a few exceptions, such as Samsung-related Android devices still support to show icons on the Overflow Menu.
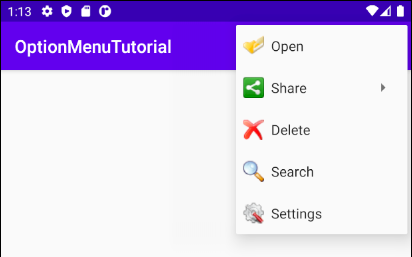
activity_main_menu.xml (2)
<?xml version="1.0" encoding="utf-8"?>
<menu xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:android="http://schemas.android.com/apk/res/android">
<item
android:icon="@drawable/icon_open"
android:title="Open"
app:showAsAction="collapseActionView" />
<item
android:icon="@drawable/icon_share"
android:title="Share">
<menu>
<item android:title="Facebook" />
<item android:title="Instagram" />
</menu>
</item>
<item
android:icon="@drawable/icon_delete"
android:title="Delete" />
<item
android:id="@+id/app_bar_search"
android:icon="@drawable/icon_search"
android:title="Search"
app:actionViewClass="android.widget.SearchView" />
<item
android:icon="@drawable/icon_settings"
android:title="Settings" />
</menu>
According to a post on StackOverflow, you still have a "tricky" way to make the icons appear on the Item Menu of the Overflow Menu, but there is no guarantee that it will work in later versions.
ActivityMain.java
package org.o7planning.optionmenututorial;
import androidx.appcompat.app.AppCompatActivity;
import androidx.appcompat.view.menu.MenuBuilder;
import android.annotation.SuppressLint;
import android.os.Bundle;
import android.view.Menu;
import android.view.MenuInflater;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
@SuppressLint("RestrictedApi")
@Override
public boolean onCreateOptionsMenu(Menu menu) {
MenuInflater menuInflater = getMenuInflater();
menuInflater.inflate(R.menu.activity_main_menu, menu);
// If you want Icon display in Overflow Menu.
// https://stackoverflow.com/questions/19750635/icon-in-menu-not-showing-in-android
if(menu instanceof MenuBuilder){
MenuBuilder m = (MenuBuilder) menu;
m.setOptionalIconsVisible(true);
}
return true;
}
}
app:showAsAction="always | ifRoom"
Google's new mindset is that the common Menu Items of an Option Menu should be displayed on the App Bar, but users will only see their icons. What they claim is that that will upgrade the experience of the users on the application.
activity_main_menu.xml (3)
<?xml version="1.0" encoding="utf-8"?>
<menu xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:android="http://schemas.android.com/apk/res/android">
<item
android:icon="@drawable/icon_open"
android:title="Open"
app:showAsAction="collapseActionView" />
<item
android:icon="@drawable/icon_share"
android:title="Share"
app:showAsAction="ifRoom">
<menu>
<item android:title="Facebook" />
<item android:title="Instagram" />
</menu>
</item>
<item
android:icon="@drawable/icon_delete"
android:title="Delete" />
<item
android:id="@+id/app_bar_search"
android:icon="@drawable/icon_search"
android:title="Search"
app:actionViewClass="android.widget.SearchView"
app:showAsAction="always" />
<item
android:icon="@drawable/icon_settings"
android:title="Settings"
app:showAsAction="always" />
</menu>
The Menu Item with theattribute of app:showAsAction="always" will always be shown on the App Bar, and you will not see it on the Overflow Menu.
<item
android:icon="@drawable/icon_settings"
android:title="Settings"
app:showAsAction="always" />
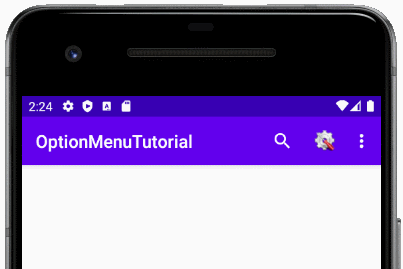
The Menu Item with the attribute of app:showAsAction="ifRoom" will be displayed on the App Bar if there is enough space for it. As soon as it appears on the App Bar, you won't see it on the Overflow Menu.
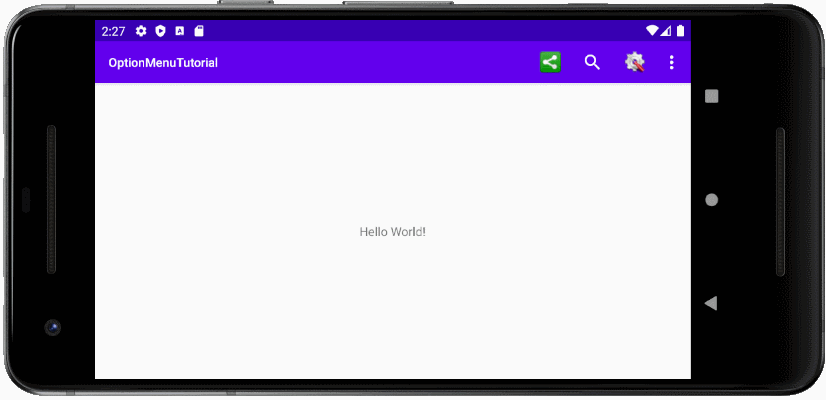
Because Search and Settings functions are two of the important functions of the application, it is quite easy to understand why they are often viewed on the App Bar.
2. Example of Option Menu
Let's see the example:
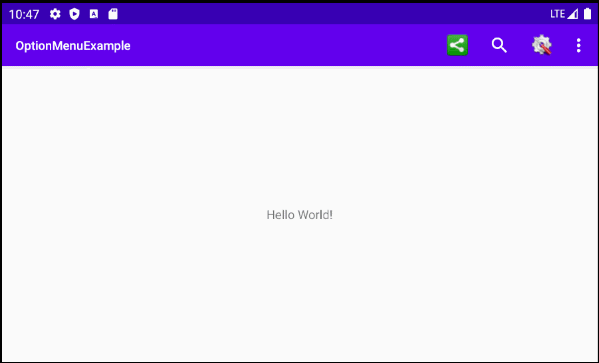
On Android Studio, create a new project:
- File > New > New Project > Empty Activity
- Name: OptionMenuExample
- Package name: org.o7planning.optionmenuexample
- Language: Java
Copy some icons and paste in the drawable folder of your project:
icon_open.png | icon_delete.png | icon_settings.png | icon_search.png | icon_share.png |
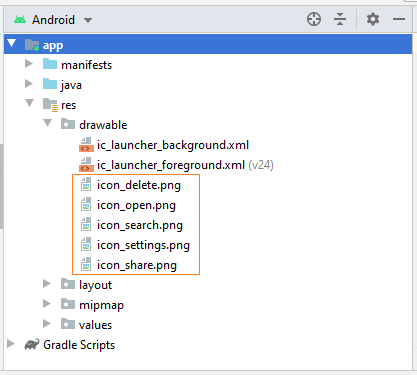
On Android Studio,select:
- File > New > Android Resource File
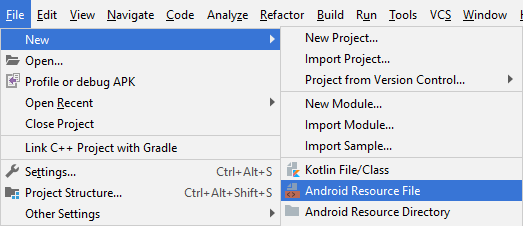
- File name: activity_main_menu.xml
- Resource Type: Menu
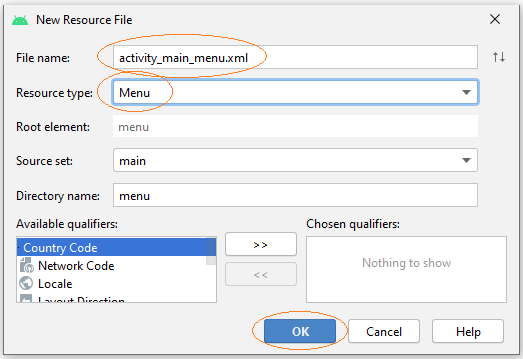
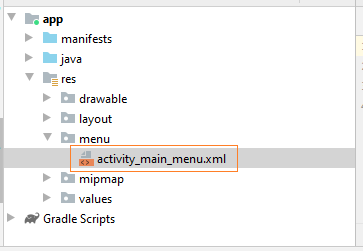
You can visually design the Menu on Android Studio.
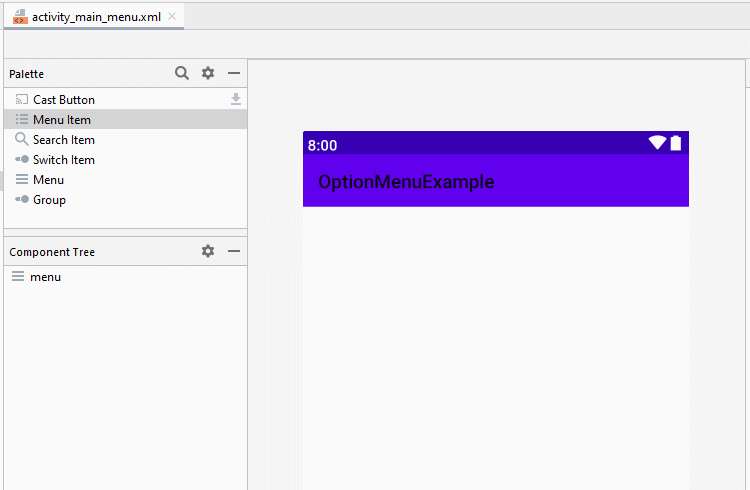
Set ID, Title,and Icon for the Menu Items:
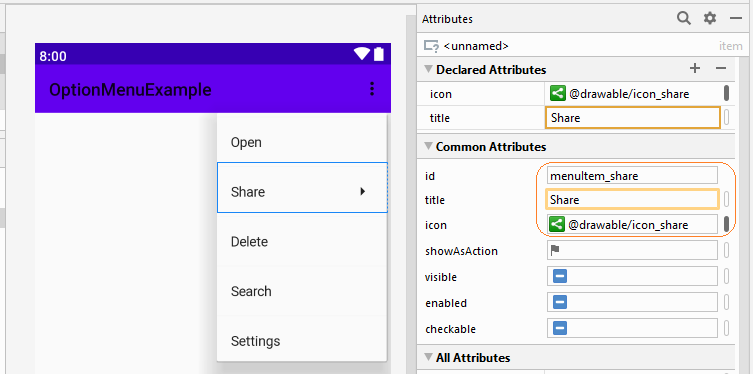
The setting of the "Search" and "Settings" functions will always be on display on the App Bar, and the "Share" function will be shown on the App Bar if there is enough free space.
- Search: showAsAction="always"
- Settings: showAsAction="always"
- Share: showAsAction="ifRoom"
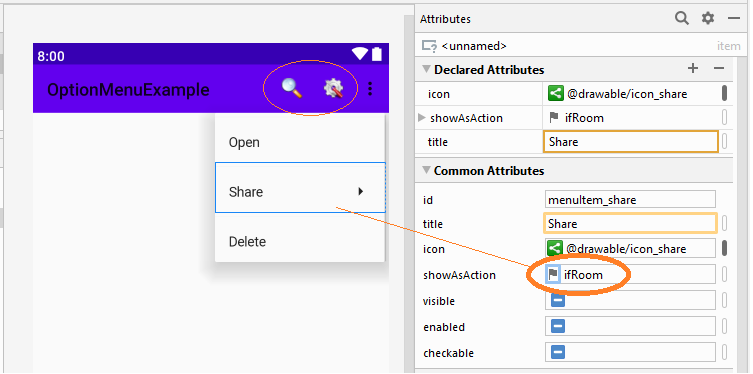
activity_main_menu.xml
<?xml version="1.0" encoding="utf-8"?>
<menu xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:android="http://schemas.android.com/apk/res/android">
<item
android:id="@+id/menuItem_open"
android:icon="@drawable/icon_open"
android:title="Open" />
<item
android:id="@+id/menuItem_share"
android:icon="@drawable/icon_share"
android:title="Share"
app:showAsAction="ifRoom">
<menu>
<item
android:id="@+id/menuItem_facebook"
android:title="Facebook" />
<item
android:id="@+id/menuItem_instagram"
android:title="Instagram" />
</menu>
</item>
<item
android:id="@+id/menuItem_delete"
android:icon="@drawable/icon_delete"
android:title="Delete" />
<item
android:id="@+id/menuItem_search"
android:icon="@drawable/icon_search"
android:title="Search"
app:actionViewClass="android.widget.SearchView"
app:showAsAction="always" />
<item
android:id="@+id/menuItem_settings"
android:icon="@drawable/icon_settings"
android:title="Settings"
app:showAsAction="always" />
</menu>
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello World!"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.java
package org.o7planning.optionmenuexample;
import androidx.appcompat.app.AppCompatActivity;
import androidx.appcompat.view.menu.MenuBuilder;
import android.annotation.SuppressLint;
import android.app.SearchManager;
import android.content.Context;
import android.os.Bundle;
import android.util.Log;
import android.view.Menu;
import android.view.MenuInflater;
import android.view.MenuItem;
import android.view.View;
import android.widget.SearchView;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
private static final String LOG_TAG= "OptionMenuExample";
private SearchView searchView;
private String lastQuery;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
@SuppressLint("RestrictedApi")
@Override
public boolean onCreateOptionsMenu(Menu menu) {
MenuInflater menuInflater = getMenuInflater();
menuInflater.inflate(R.menu.activity_main_menu, menu);
// If you want Icon display in Overflow Menu.
// https://stackoverflow.com/questions/19750635/icon-in-menu-not-showing-in-android
if (menu instanceof MenuBuilder) {
MenuBuilder m = (MenuBuilder) menu;
m.setOptionalIconsVisible(true);
}
SearchManager searchManager = (SearchManager) getSystemService(Context.SEARCH_SERVICE);
this.searchView = (SearchView) menu.findItem(R.id.menuItem_search).getActionView();
this.searchView.setSearchableInfo(searchManager.getSearchableInfo(getComponentName()));
// Need click "search" icon to expand SearchView.
this.searchView.setIconifiedByDefault(true);
searchView.setOnQueryTextListener(new SearchView.OnQueryTextListener() {
// Typing search text.
public boolean onQueryTextChange(String newText) {
// This is your adapter that will be filtered
Log.i(LOG_TAG, "onQueryTextChange: " + newText);
return true;
}
// Press Enter to search (Or something to search).
public boolean onQueryTextSubmit(String query) {
// IMPORTANT!
// Prevent onQueryTextSubmit() method called twice.
// https://stackoverflow.com/questions/34207670
searchView.clearFocus();
Log.i(LOG_TAG, "onQueryTextSubmit: " + query);
return doSearch(query);
}
});
searchView.setOnSearchClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Log.i(LOG_TAG, "SearchView.onSearchClickListener!" );
}
}) ;
return super.onCreateOptionsMenu(menu);
}
private boolean doSearch(String query) {
if (query == null || query.isEmpty()) {
return false; // Cancel search.
}
this.lastQuery = query;
Toast.makeText(this, "Search: " + query, Toast.LENGTH_LONG).show();
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
switch (item.getItemId()) {
case R.id.menuItem_open:
Toast.makeText(this, "Open ...", Toast.LENGTH_LONG).show();
return true;
case R.id.menuItem_delete:
Toast.makeText(this, "Delete ...", Toast.LENGTH_LONG).show();
return true;
case R.id.menuItem_facebook:
Toast.makeText(this, "Facebook Share ...", Toast.LENGTH_LONG).show();
return true;
case R.id.menuItem_instagram:
Toast.makeText(this, "Instagram Share ...", Toast.LENGTH_LONG).show();
return true;
case R.id.menuItem_settings:
Toast.makeText(this, "Settings ...", Toast.LENGTH_LONG).show();
return true;
case R.id.menuItem_search:
Log.i(LOG_TAG, "onOptionsItemSelected (R.id.menuItem_search)");
Toast.makeText(this, "Search ...", Toast.LENGTH_LONG).show();
return true;
default:
return super.onOptionsItemSelected(item);
}
}
}
Android Programming Tutorials
- Configure Android Emulator in Android Studio
- Android ToggleButton Tutorial with Examples
- Create a simple File Finder Dialog in Android
- Android TimePickerDialog Tutorial with Examples
- Android DatePickerDialog Tutorial with Examples
- What is needed to get started with Android?
- Install Android Studio on Windows
- Install Intel® HAXM for Android Studio
- Android AsyncTask Tutorial with Examples
- Android AsyncTaskLoader Tutorial with Examples
- Android Tutorial for Beginners - Basic examples
- How to know the phone number of Android Emulator and change it
- Android TextInputLayout Tutorial with Examples
- Android CardView Tutorial with Examples
- Android ViewPager2 Tutorial with Examples
- Get Phone Number in Android using TelephonyManager
- Android Phone Call Tutorial with Examples
- Android Wifi Scanning Tutorial with Examples
- Android 2D Game Tutorial for Beginners
- Android DialogFragment Tutorial with Examples
- Android CharacterPickerDialog Tutorial with Examples
- Android Tutorial for Beginners - Hello Android
- Using Android Device File Explorer
- Enable USB Debugging on Android Device
- Android UI Layouts Tutorial with Examples
- Android SMS Tutorial with Examples
- Android SQLite Database Tutorial with Examples
- Google Maps Android API Tutorial with Examples
- Android Text to Speech Tutorial with Examples
- Android Space Tutorial with Examples
- Android Toast Tutorial with Examples
- Create a custom Android Toast
- Android SnackBar Tutorial with Examples
- Android TextView Tutorial with Examples
- Android TextClock Tutorial with Examples
- Android EditText Tutorial with Examples
- Android TextWatcher Tutorial with Examples
- Format Credit Card Number with Android TextWatcher
- Android Clipboard Tutorial with Examples
- Create a simple File Chooser in Android
- Android AutoCompleteTextView and MultiAutoCompleteTextView Tutorial with Examples
- Android ImageView Tutorial with Examples
- Android ImageSwitcher Tutorial with Examples
- Android ScrollView and HorizontalScrollView Tutorial with Examples
- Android WebView Tutorial with Examples
- Android SeekBar Tutorial with Examples
- Android Dialog Tutorial with Examples
- Android AlertDialog Tutorial with Examples
- Android RatingBar Tutorial with Examples
- Android ProgressBar Tutorial with Examples
- Android Spinner Tutorial with Examples
- Android Button Tutorial with Examples
- Android Switch Tutorial with Examples
- Android ImageButton Tutorial with Examples
- Android FloatingActionButton Tutorial with Examples
- Android CheckBox Tutorial with Examples
- Android RadioGroup and RadioButton Tutorial with Examples
- Android Chip and ChipGroup Tutorial with Examples
- Using image assets and icon assets of Android Studio
- Setting SD Card for Android Emulator
- ChipGroup and Chip Entry Example
- How to add external libraries to Android Project in Android Studio?
- How to disable the permissions already granted to the Android application?
- How to remove applications from Android Emulator?
- Android LinearLayout Tutorial with Examples
- Android TableLayout Tutorial with Examples
- Android FrameLayout Tutorial with Examples
- Android QuickContactBadge Tutorial with Examples
- Android StackView Tutorial with Examples
- Android Camera Tutorial with Examples
- Android MediaPlayer Tutorial with Examples
- Android VideoView Tutorial with Examples
- Playing Sound effects in Android with SoundPool
- Android Networking Tutorial with Examples
- Android JSON Parser Tutorial with Examples
- Android SharedPreferences Tutorial with Examples
- Android Internal Storage Tutorial with Examples
- Android External Storage Tutorial with Examples
- Android Intents Tutorial with Examples
- Example of an explicit Android Intent, calling another Intent
- Example of implicit Android Intent, open a URL, send an email
- Android Services Tutorial with Examples
- Android Notifications Tutorial with Examples
- Android DatePicker Tutorial with Examples
- Android TimePicker Tutorial with Examples
- Android Chronometer Tutorial with Examples
- Android OptionMenu Tutorial with Examples
- Android ContextMenu Tutorial with Examples
- Android PopupMenu Tutorial with Examples
- Android Fragments Tutorial with Examples
- Android ListView Tutorial with Examples
- Android ListView with Checkbox using ArrayAdapter
- Android GridView Tutorial with Examples
Show More