Android MediaPlayer Tutorial with Examples
1. MediaPlayer
Android provides a component to play music, it's MediaPlayer. MediaPlayer can run audio and video files, the source file is located on your device or from a URL.
Just like with other music player that you know, MediaPlayer provides methods to control playback of audio/video, including start, stop, rewind .....
Just like with other music player that you know, MediaPlayer provides methods to control playback of audio/video, including start, stop, rewind .....
You can also call MediaPlayer from a service.
MediaPlayer is a component that don't have interface, it help you play a music file easily, but to play a video file you need to combine it with SuffaceView to display imagine.
MediaPlayer is a component that don't have interface, it help you play a music file easily, but to play a video file you need to combine it with SuffaceView to display imagine.
2. MediaPlayer example
The following simple example, use Media Player to play a music file and some controls of the play such as play, pause, rewind.
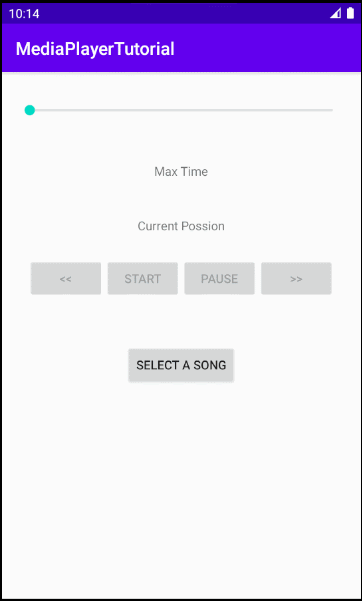
Create a project named MediaPlayerTutorial:
- File > New > New Project > Empty Activity
- Name: MediaPlayerTutorial
- Package name: org.o7planning.mediaplayertutorial
- Language: Java
Create raw to contain the music file.
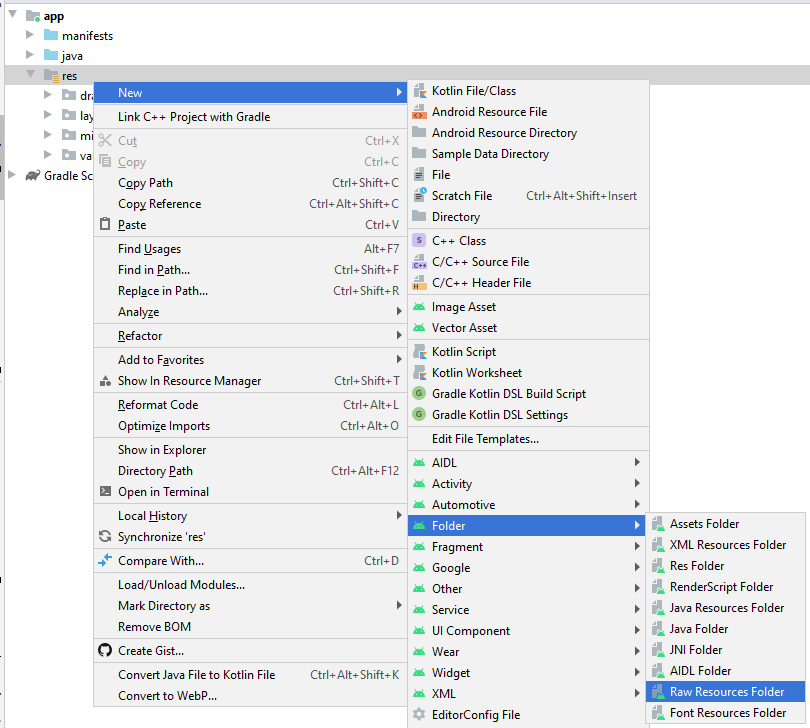
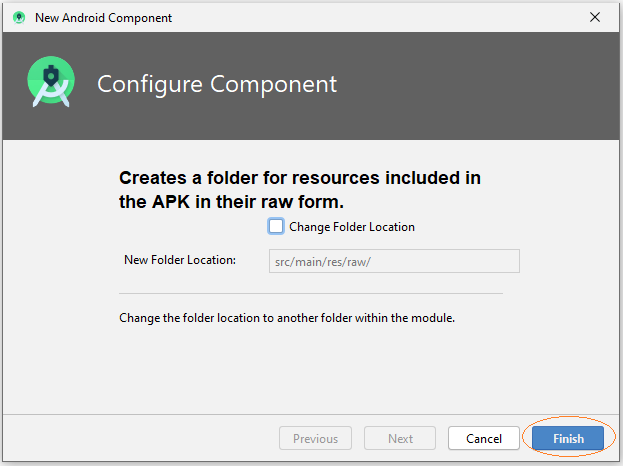
Prepare a music file, copy and paste to raw folder.
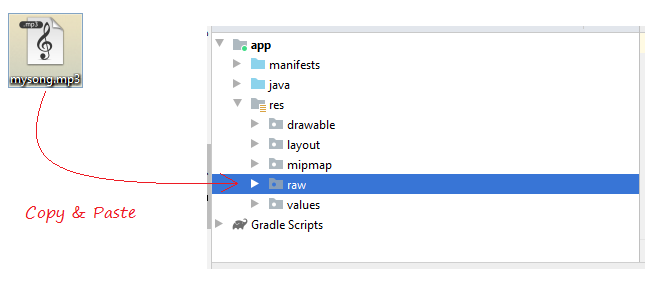
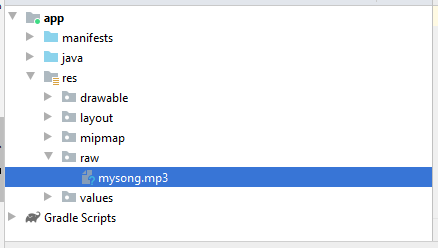
If you want to play a song from a URL you must allow the application to access the network, adding the following XML code to AndroidManifest.xml.
<uses-permission android:name="android.permission.INTERNET" />
Allows the app to access external storage (Such as SD Card) if you want to play media here.
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE"/>
AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="org.o7planning.mediaplayertutorial">
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE"/>
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
Design the interface:
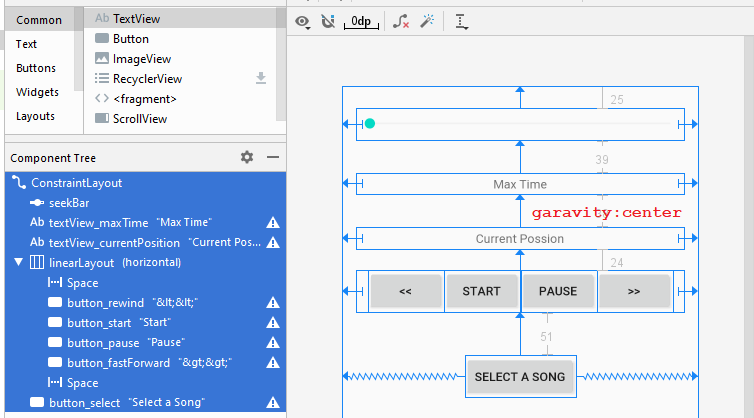
If you are interested in the steps to design the interface of this application, please see the appendix at the end of the article.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<SeekBar
android:id="@+id/seekBar"
android:layout_width="0dp"
android:layout_height="37dp"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="25dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<TextView
android:id="@+id/textView_maxTime"
android:layout_width="0dp"
android:layout_height="24dp"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="39dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:gravity="center"
android:text="Max Time"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/seekBar" />
<TextView
android:id="@+id/textView_currentPosition"
android:layout_width="0dp"
android:layout_height="25dp"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="38dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:gravity="center"
android:text="Current Possion"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView_maxTime" />
<LinearLayout
android:id="@+id/linearLayout"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="24dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:orientation="horizontal"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView_currentPosition">
<Space
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1" />
<Button
android:id="@+id/button_rewind"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="0"
android:text="<<" />
<Button
android:id="@+id/button_start"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="0"
android:text="Start" />
<Button
android:id="@+id/button_pause"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="0"
android:text="Pause" />
<Button
android:id="@+id/button_fastForward"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="0"
android:text=">>" />
<Space
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1" />
</LinearLayout>
<Button
android:id="@+id/button_select"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="51dp"
android:text="Select a Song"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/linearLayout" />
</androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.java
package org.o7planning.mediaplayertutorial;
import androidx.appcompat.app.AppCompatActivity;
import android.media.AudioManager;
import android.os.Bundle;
import android.media.MediaPlayer;
import android.os.Handler;
import android.view.View;
import android.widget.Button;
import android.widget.SeekBar;
import android.widget.TextView;
import java.util.concurrent.TimeUnit;
public class MainActivity extends AppCompatActivity {
private TextView textMaxTime;
private TextView textCurrentPosition;
private Button buttonPause;
private Button buttonStart;
private Button buttonRewind;
private Button buttonFastForward;
private Button buttonSelect;
private SeekBar seekBar;
private Handler threadHandler = new Handler();
private MediaPlayer mediaPlayer;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.textCurrentPosition = (TextView)this.findViewById(R.id.textView_currentPosition);
this.textMaxTime=(TextView) this.findViewById(R.id.textView_maxTime);
this.buttonSelect = (Button) this.findViewById(R.id.button_select);
this.buttonStart= (Button) this.findViewById(R.id.button_start);
this.buttonPause= (Button) this.findViewById(R.id.button_pause);
this.buttonRewind= (Button) this.findViewById(R.id.button_rewind);
this.buttonFastForward= (Button) this.findViewById(R.id.button_fastForward);
this.buttonStart.setEnabled(false);
this.buttonPause.setEnabled(false);
this.buttonRewind.setEnabled(false);
this.buttonFastForward.setEnabled(false);
this.seekBar= (SeekBar) this.findViewById(R.id.seekBar);
this.seekBar.setClickable(false);
this.buttonSelect.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// Select new media source.
selectMediaResource();
}
});
this.buttonStart.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
doStart( );
}
});
this.buttonPause.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
doPause( );
}
});
this.buttonRewind.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
doRewind( );
}
});
this.buttonFastForward .setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
doFastForward( );
}
});
// Create MediaPlayer.
this.mediaPlayer= new MediaPlayer();
this.mediaPlayer.setAudioStreamType(AudioManager.STREAM_MUSIC);
this.mediaPlayer.setOnPreparedListener(new MediaPlayer.OnPreparedListener() {
@Override
public void onPrepared(MediaPlayer mp) {
doStop(); // Stop current media.
}
});
this.mediaPlayer.setOnCompletionListener(new MediaPlayer.OnCompletionListener() {
@Override
public void onCompletion(MediaPlayer mp) {
doComplete();
}
});
}
// When user click "Select Media Source" button.
private void selectMediaResource() {
// this.selectRawMediaSource();
// this.selectURLMediaSource();
// this.selectLocalMediaSource();
this.selectRawMediaSource();
}
private void selectRawMediaSource() {
// "mysong.mp3" ==> resName = "mysong".
String resName = MediaPlayerUtils.RAW_MEDIA_SAMPLE;
MediaPlayerUtils.playRawMedia(this, this.mediaPlayer, resName);
}
private void selectURLMediaSource() {
// http://example.coom/mysong.mp3
String mediaURL = MediaPlayerUtils.URL_MEDIA_SAMPLE;
MediaPlayerUtils.playURLMedia(this, this.mediaPlayer, mediaURL);
}
private void selectLocalMediaSource() {
// @localPath = "/storage/emulated/0/DCIM/Music/mysong.mp3"; (For example).
String localPath = MediaPlayerUtils.LOCAL_MEDIA_SAMPLE;
MediaPlayerUtils.playLocalMedia(this, this.mediaPlayer, localPath);
}
// Convert millisecond to string.
private String millisecondsToString(int milliseconds) {
long minutes = TimeUnit.MILLISECONDS.toMinutes((long) milliseconds);
long seconds = TimeUnit.MILLISECONDS.toSeconds((long) milliseconds) ;
return minutes + ":"+ seconds;
}
private void doStart( ) {
if(this.mediaPlayer.isPlaying()) {
return;
}
// The duration in milliseconds
int duration = this.mediaPlayer.getDuration();
int currentPosition = this.mediaPlayer.getCurrentPosition();
if(currentPosition== 0) {
this.seekBar.setMax(duration);
String maxTimeString = this.millisecondsToString(duration);
this.textMaxTime.setText(maxTimeString);
} else if(currentPosition== duration) {
// Resets the MediaPlayer to its uninitialized state.
this.mediaPlayer.reset();
}
this.mediaPlayer.start();
// Create a thread to update position of SeekBar.
UpdateSeekBarThread updateSeekBarThread= new UpdateSeekBarThread();
threadHandler.postDelayed(updateSeekBarThread,50);
this.buttonPause.setEnabled(true);
this.buttonStart.setEnabled(false);
this.buttonRewind.setEnabled(true);
this.buttonFastForward.setEnabled(true);
}
// Called by MediaPlayer.OnCompletionListener
// When Player cocmplete
private void doComplete() {
buttonStart.setEnabled(true);
buttonPause.setEnabled(false);
buttonRewind.setEnabled(true);
buttonFastForward.setEnabled(false);
}
// Called by MediaPlayer.OnPreparedListener.
// When user select a new media source, then stop current.
private void doStop() {
if(this.mediaPlayer.isPlaying()) {
this.mediaPlayer.stop();
}
buttonStart.setEnabled(true);
buttonPause.setEnabled(false);
buttonRewind.setEnabled(false);
buttonFastForward.setEnabled(false);
}
// When user click to "Pause".
private void doPause( ) {
this.mediaPlayer.pause();
this.buttonPause.setEnabled(false);
this.buttonStart.setEnabled(true);
}
// When user click to "Rewind".
private void doRewind( ) {
int currentPosition = this.mediaPlayer.getCurrentPosition();
int duration = this.mediaPlayer.getDuration();
// 5 seconds.
int SUBTRACT_TIME = 5000;
if(currentPosition - SUBTRACT_TIME > 0 ) {
this.mediaPlayer.seekTo(currentPosition - SUBTRACT_TIME);
}
this.buttonFastForward.setEnabled(true);
}
// When user click to "Fast-Forward".
private void doFastForward( ) {
int currentPosition = this.mediaPlayer.getCurrentPosition();
int duration = this.mediaPlayer.getDuration();
// 5 seconds.
int ADD_TIME = 5000;
if(currentPosition + ADD_TIME < duration) {
this.mediaPlayer.seekTo(currentPosition + ADD_TIME);
}
}
// Thread to Update position for SeekBar.
class UpdateSeekBarThread implements Runnable {
public void run() {
int currentPosition = mediaPlayer.getCurrentPosition();
String currentPositionStr = millisecondsToString(currentPosition);
textCurrentPosition.setText(currentPositionStr);
seekBar.setProgress(currentPosition);
// Delay thread 50 milisecond.
threadHandler.postDelayed(this, 50);
}
}
}
MediaPlayerUtils.java
package org.o7planning.mediaplayertutorial;
import android.content.Context;
import android.media.MediaPlayer;
import android.net.Uri;
import android.util.Log;
import android.widget.Toast;
public class MediaPlayerUtils {
// "mysong.mp3" in directory "raw".
public static final String RAW_MEDIA_SAMPLE = "mysong";
// Example Path: /sdcard/Music/mysong.mp3
// Example Path: /storage/emulated/0/DCIM/Music/mysong.mp3
public static final String LOCAL_MEDIA_SAMPLE ="/sdcard/Music/mysong.mp3";
public static final String URL_MEDIA_SAMPLE = "https://ex1.o7planning.com/_testdatas_/yodel.mp3";
public static final String LOG_TAG= "MediaPlayerTutorial";
// Play a media in directory RAW.
// Media name = "mysong.mp3" ==> resName = "mysong".
public static void playRawMedia(Context context, MediaPlayer mediaPlayer, String resName) {
try {
// ID of video file.
int id = MediaPlayerUtils.getRawResIdByName( context,resName);
Uri uri = Uri.parse("android.resource://" + context.getPackageName() + "/" + id);
Log.i(LOG_TAG, "Media URI: "+ uri);
Toast.makeText(context,"Select source: "+ uri,Toast.LENGTH_SHORT).show();
mediaPlayer.setDataSource(context, uri);
mediaPlayer.prepareAsync();
} catch (Exception e) {
Log.e(LOG_TAG, "Error Play Raw Media: "+e.getMessage());
Toast.makeText(context,"Error Play Raw Media: "+ e.getMessage(),Toast.LENGTH_SHORT).show();
e.printStackTrace();
}
}
// Example Path: /sdcard/Music/mysong.mp3
// Example Path: /storage/emulated/0/DCIM/Music/mysong.mp3
public static void playLocalMedia(Context context, MediaPlayer mediaPlayer, String localPath) {
try {
Uri uri = Uri.parse("android.resource://" + localPath);
Log.i(LOG_TAG, "Media URI: "+ uri);
Toast.makeText(context,"Select source: "+ uri,Toast.LENGTH_SHORT).show();
mediaPlayer.setDataSource(context, uri);
mediaPlayer.prepareAsync();
} catch(Exception e) {
Log.e(LOG_TAG, "Error Play Local Media: "+ e.getMessage());
Toast.makeText(context,"Error Play Local Media: "+ e.getMessage(),Toast.LENGTH_SHORT).show();
e.printStackTrace();
}
}
// String videoURL = "https://ex1.o7planning.com/_testdatas_/yodel.mp3";
public static void playURLMedia(Context context, MediaPlayer mediaPlayer, String videoURL) {
try {
Log.i(LOG_TAG, "Media URL: "+ videoURL);
Uri uri= Uri.parse( videoURL );
Toast.makeText(context,"Select source: "+ uri,Toast.LENGTH_SHORT).show();
mediaPlayer.setDataSource(context, uri);
mediaPlayer.prepareAsync();
} catch(Exception e) {
Log.e(LOG_TAG, "Error Play URL Media: "+ e.getMessage());
Toast.makeText(context,"Error Play URL Media: "+ e.getMessage(),Toast.LENGTH_SHORT).show();
e.printStackTrace();
}
}
// Find ID corresponding to the name of the resource (in the directory RAW).
public static int getRawResIdByName(Context context, String resName) {
String pkgName = context.getPackageName();
// Return 0 if not found.
int resID = context.getResources().getIdentifier(resName, "raw", pkgName);
Log.i(LOG_TAG, "Res Name: " + resName + "==> Res ID = " + resID);
return resID;
}
}
Android Programming Tutorials
- Configure Android Emulator in Android Studio
- Android ToggleButton Tutorial with Examples
- Create a simple File Finder Dialog in Android
- Android TimePickerDialog Tutorial with Examples
- Android DatePickerDialog Tutorial with Examples
- What is needed to get started with Android?
- Install Android Studio on Windows
- Install Intel® HAXM for Android Studio
- Android AsyncTask Tutorial with Examples
- Android AsyncTaskLoader Tutorial with Examples
- Android Tutorial for Beginners - Basic examples
- How to know the phone number of Android Emulator and change it
- Android TextInputLayout Tutorial with Examples
- Android CardView Tutorial with Examples
- Android ViewPager2 Tutorial with Examples
- Get Phone Number in Android using TelephonyManager
- Android Phone Call Tutorial with Examples
- Android Wifi Scanning Tutorial with Examples
- Android 2D Game Tutorial for Beginners
- Android DialogFragment Tutorial with Examples
- Android CharacterPickerDialog Tutorial with Examples
- Android Tutorial for Beginners - Hello Android
- Using Android Device File Explorer
- Enable USB Debugging on Android Device
- Android UI Layouts Tutorial with Examples
- Android SMS Tutorial with Examples
- Android SQLite Database Tutorial with Examples
- Google Maps Android API Tutorial with Examples
- Android Text to Speech Tutorial with Examples
- Android Space Tutorial with Examples
- Android Toast Tutorial with Examples
- Create a custom Android Toast
- Android SnackBar Tutorial with Examples
- Android TextView Tutorial with Examples
- Android TextClock Tutorial with Examples
- Android EditText Tutorial with Examples
- Android TextWatcher Tutorial with Examples
- Format Credit Card Number with Android TextWatcher
- Android Clipboard Tutorial with Examples
- Create a simple File Chooser in Android
- Android AutoCompleteTextView and MultiAutoCompleteTextView Tutorial with Examples
- Android ImageView Tutorial with Examples
- Android ImageSwitcher Tutorial with Examples
- Android ScrollView and HorizontalScrollView Tutorial with Examples
- Android WebView Tutorial with Examples
- Android SeekBar Tutorial with Examples
- Android Dialog Tutorial with Examples
- Android AlertDialog Tutorial with Examples
- Android RatingBar Tutorial with Examples
- Android ProgressBar Tutorial with Examples
- Android Spinner Tutorial with Examples
- Android Button Tutorial with Examples
- Android Switch Tutorial with Examples
- Android ImageButton Tutorial with Examples
- Android FloatingActionButton Tutorial with Examples
- Android CheckBox Tutorial with Examples
- Android RadioGroup and RadioButton Tutorial with Examples
- Android Chip and ChipGroup Tutorial with Examples
- Using image assets and icon assets of Android Studio
- Setting SD Card for Android Emulator
- ChipGroup and Chip Entry Example
- How to add external libraries to Android Project in Android Studio?
- How to disable the permissions already granted to the Android application?
- How to remove applications from Android Emulator?
- Android LinearLayout Tutorial with Examples
- Android TableLayout Tutorial with Examples
- Android FrameLayout Tutorial with Examples
- Android QuickContactBadge Tutorial with Examples
- Android StackView Tutorial with Examples
- Android Camera Tutorial with Examples
- Android MediaPlayer Tutorial with Examples
- Android VideoView Tutorial with Examples
- Playing Sound effects in Android with SoundPool
- Android Networking Tutorial with Examples
- Android JSON Parser Tutorial with Examples
- Android SharedPreferences Tutorial with Examples
- Android Internal Storage Tutorial with Examples
- Android External Storage Tutorial with Examples
- Android Intents Tutorial with Examples
- Example of an explicit Android Intent, calling another Intent
- Example of implicit Android Intent, open a URL, send an email
- Android Services Tutorial with Examples
- Android Notifications Tutorial with Examples
- Android DatePicker Tutorial with Examples
- Android TimePicker Tutorial with Examples
- Android Chronometer Tutorial with Examples
- Android OptionMenu Tutorial with Examples
- Android ContextMenu Tutorial with Examples
- Android PopupMenu Tutorial with Examples
- Android Fragments Tutorial with Examples
- Android ListView Tutorial with Examples
- Android ListView with Checkbox using ArrayAdapter
- Android GridView Tutorial with Examples
Show More