Android TextView Tutorial with Examples
1. Android TextView
In Android, TextView is a user interface control, which is used to display text. It works like a label. By default, the user will not be able to edit the text content.
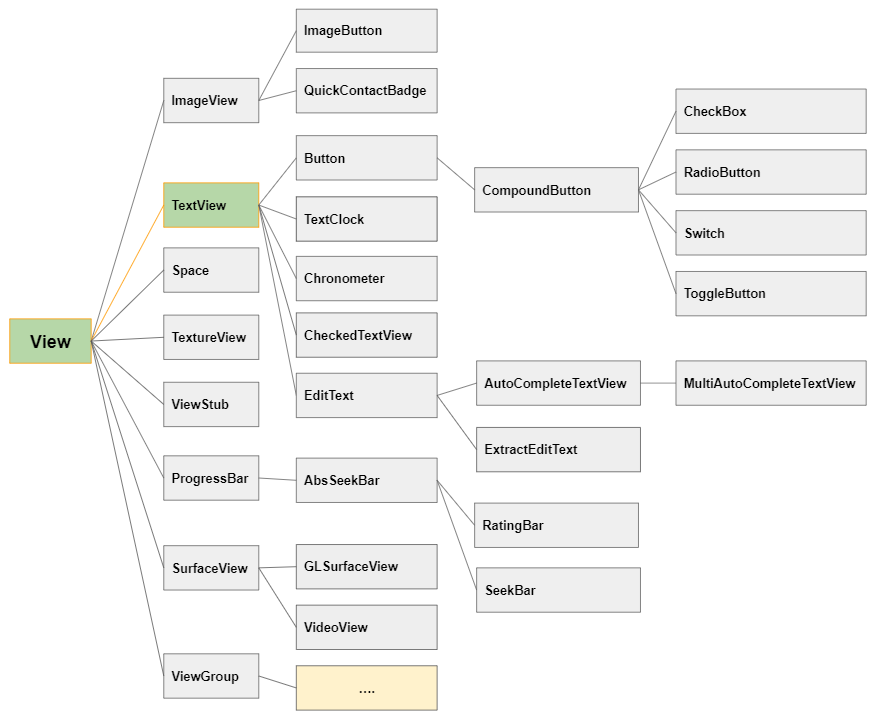
2. TextView Attributes
android:id | It is used to uniquely identify the control |
android:autoLink | It will automatically found and convert URL(s) and email addresses as clickable links. |
android:ems | It is used to make the TextView be exactly this many ems wide. |
android:hint | It is used to display the hint text when text is empty |
android:width | It makes the TextView be exactly this many pixels wide. |
android:height | It makes the TextView be exactly this many pixels tall. |
android:text | It is used to display the text. |
android:textColor | It is used to change the color of the text. |
android:gravity | It is used to specify how to align the text by the view's x and y-axis. |
android:maxWidth | It is used to make the TextView be at most this many pixels wide. |
android:minWidth | It is used to make the TextView be at least this many pixels wide. |
android:textSize | It is used to specify the size of the text. |
android:textStyle | It is used to change the style (bold, italic, bolditalic) of text. |
android:textAllCaps | It is used to present the text in all CAPS. |
android:typeface | It is used to specify the Typeface (normal, sans, serif, monospace) for the text. |
android:textColorHighlight | It is used to change the color of text selection highlight. |
android:textColorLink | It is used to change the text color of links. |
android:inputType | It is used to specify the type of text being placed in text fields. |
android:fontFamily | It is used to specify the fontFamily for the text. |
android:editable | If we set, it specifies that this TextView has an input method. |
android:autoLink
This attribute tells Android to automatically generate links for phone numbers, addresses, email addresses and website addresses found in the TextView text.
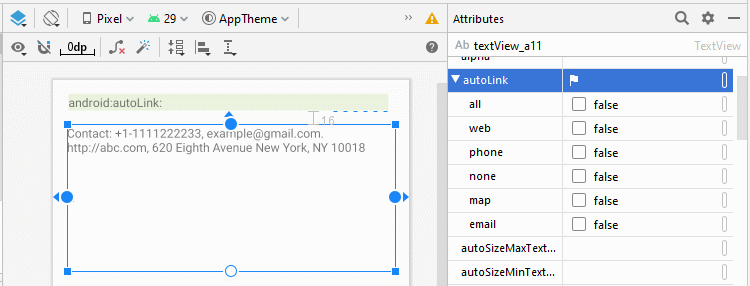
Constant | Value | Description |
all | f | Match all patterns (equivalent to web|email|phone|map). |
email | 2 | Match email addresses. |
map | 8 | Match map addresses. |
none | 0 | Match no patterns (default). |
phone | 4 | Match phone numbers. |
web | 1 | Match Web URLs |
<TextView
android:id="@+id/textView_a11"
android:autoLink="email|phone|map|web"
android:textColorLink="#FF0000"
android:text="Contact: +1-1111222233, example@gmail.com. http://abc.com, 620 Eighth Avenue New York, NY 10018"
... />
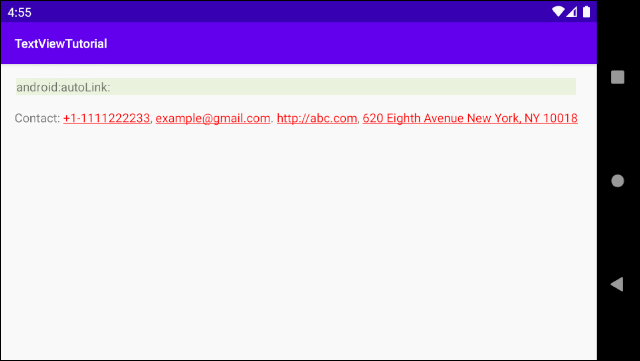
Click on Phone Link.
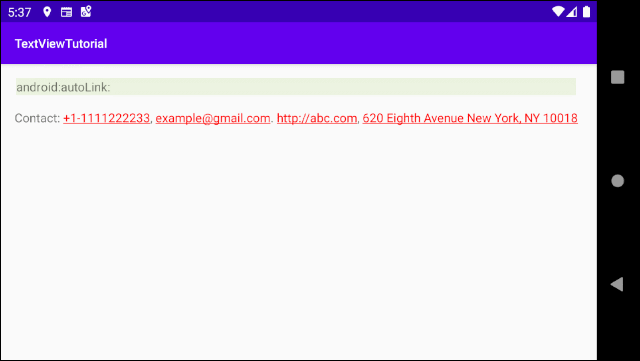
Click on Email Link
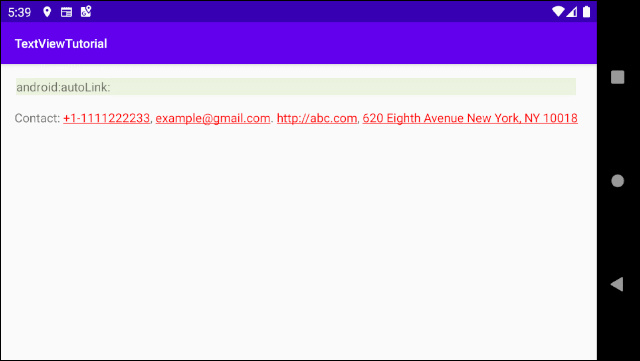
Click on Web URL
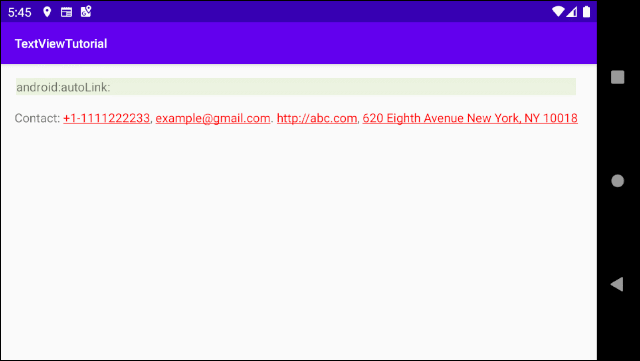
Click on Address Link (Will open Google Map)
android:ems
This attribute is used to specify a value for the width of the TextView in the EM measurement units. EM is a unit in Typography whose value is the width of the letter "M".
So, android:ems="5" means that it sets the width of the TextView to 5 times as much as the width of the letter "M".
android:text
Specifies the text content for TextView.
android:textColor
Specifies the text color for TextView.

android:textSize
Specifies the font size for the text.
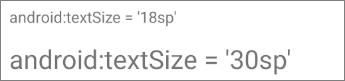
android:textStyle
Specifies a style for the font, which has four values:
- android:textStyle="normal" (Default)
- android:textStyle="bold"
- android:textStyle="italic"
- android:textStyle="bold|italic"
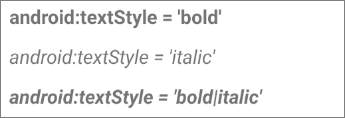
android:textAllCaps
This attribute has two values, true/false. With the value of true, the TextView text will be in upper case when displayed. Its default value is false.
android:textColorHighlight
Sets the background color for the selected sub-text. This attribute comes in handy when you use EditText (a subclass of TextView). EditText permits the user to select and edit its text.
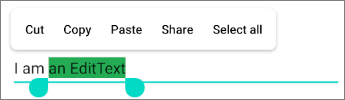
android:textColorLink
Specifies the font color for the links in the text. (See more about the android:autoLink attribute).

android:fontFamily
Specifies the font family for the TextView text.

android:editable
Android Programming Tutorials
- Configure Android Emulator in Android Studio
- Android ToggleButton Tutorial with Examples
- Create a simple File Finder Dialog in Android
- Android TimePickerDialog Tutorial with Examples
- Android DatePickerDialog Tutorial with Examples
- What is needed to get started with Android?
- Install Android Studio on Windows
- Install Intel® HAXM for Android Studio
- Android AsyncTask Tutorial with Examples
- Android AsyncTaskLoader Tutorial with Examples
- Android Tutorial for Beginners - Basic examples
- How to know the phone number of Android Emulator and change it
- Android TextInputLayout Tutorial with Examples
- Android CardView Tutorial with Examples
- Android ViewPager2 Tutorial with Examples
- Get Phone Number in Android using TelephonyManager
- Android Phone Call Tutorial with Examples
- Android Wifi Scanning Tutorial with Examples
- Android 2D Game Tutorial for Beginners
- Android DialogFragment Tutorial with Examples
- Android CharacterPickerDialog Tutorial with Examples
- Android Tutorial for Beginners - Hello Android
- Using Android Device File Explorer
- Enable USB Debugging on Android Device
- Android UI Layouts Tutorial with Examples
- Android SMS Tutorial with Examples
- Android SQLite Database Tutorial with Examples
- Google Maps Android API Tutorial with Examples
- Android Text to Speech Tutorial with Examples
- Android Space Tutorial with Examples
- Android Toast Tutorial with Examples
- Create a custom Android Toast
- Android SnackBar Tutorial with Examples
- Android TextView Tutorial with Examples
- Android TextClock Tutorial with Examples
- Android EditText Tutorial with Examples
- Android TextWatcher Tutorial with Examples
- Format Credit Card Number with Android TextWatcher
- Android Clipboard Tutorial with Examples
- Create a simple File Chooser in Android
- Android AutoCompleteTextView and MultiAutoCompleteTextView Tutorial with Examples
- Android ImageView Tutorial with Examples
- Android ImageSwitcher Tutorial with Examples
- Android ScrollView and HorizontalScrollView Tutorial with Examples
- Android WebView Tutorial with Examples
- Android SeekBar Tutorial with Examples
- Android Dialog Tutorial with Examples
- Android AlertDialog Tutorial with Examples
- Android RatingBar Tutorial with Examples
- Android ProgressBar Tutorial with Examples
- Android Spinner Tutorial with Examples
- Android Button Tutorial with Examples
- Android Switch Tutorial with Examples
- Android ImageButton Tutorial with Examples
- Android FloatingActionButton Tutorial with Examples
- Android CheckBox Tutorial with Examples
- Android RadioGroup and RadioButton Tutorial with Examples
- Android Chip and ChipGroup Tutorial with Examples
- Using image assets and icon assets of Android Studio
- Setting SD Card for Android Emulator
- ChipGroup and Chip Entry Example
- How to add external libraries to Android Project in Android Studio?
- How to disable the permissions already granted to the Android application?
- How to remove applications from Android Emulator?
- Android LinearLayout Tutorial with Examples
- Android TableLayout Tutorial with Examples
- Android FrameLayout Tutorial with Examples
- Android QuickContactBadge Tutorial with Examples
- Android StackView Tutorial with Examples
- Android Camera Tutorial with Examples
- Android MediaPlayer Tutorial with Examples
- Android VideoView Tutorial with Examples
- Playing Sound effects in Android with SoundPool
- Android Networking Tutorial with Examples
- Android JSON Parser Tutorial with Examples
- Android SharedPreferences Tutorial with Examples
- Android Internal Storage Tutorial with Examples
- Android External Storage Tutorial with Examples
- Android Intents Tutorial with Examples
- Example of an explicit Android Intent, calling another Intent
- Example of implicit Android Intent, open a URL, send an email
- Android Services Tutorial with Examples
- Android Notifications Tutorial with Examples
- Android DatePicker Tutorial with Examples
- Android TimePicker Tutorial with Examples
- Android Chronometer Tutorial with Examples
- Android OptionMenu Tutorial with Examples
- Android ContextMenu Tutorial with Examples
- Android PopupMenu Tutorial with Examples
- Android Fragments Tutorial with Examples
- Android ListView Tutorial with Examples
- Android ListView with Checkbox using ArrayAdapter
- Android GridView Tutorial with Examples
Show More