Android TextClock Tutorial with Examples
1. Android TextClock
In Android, TextClock is a subclass of TextView. It is used to display the current date and time of the system.
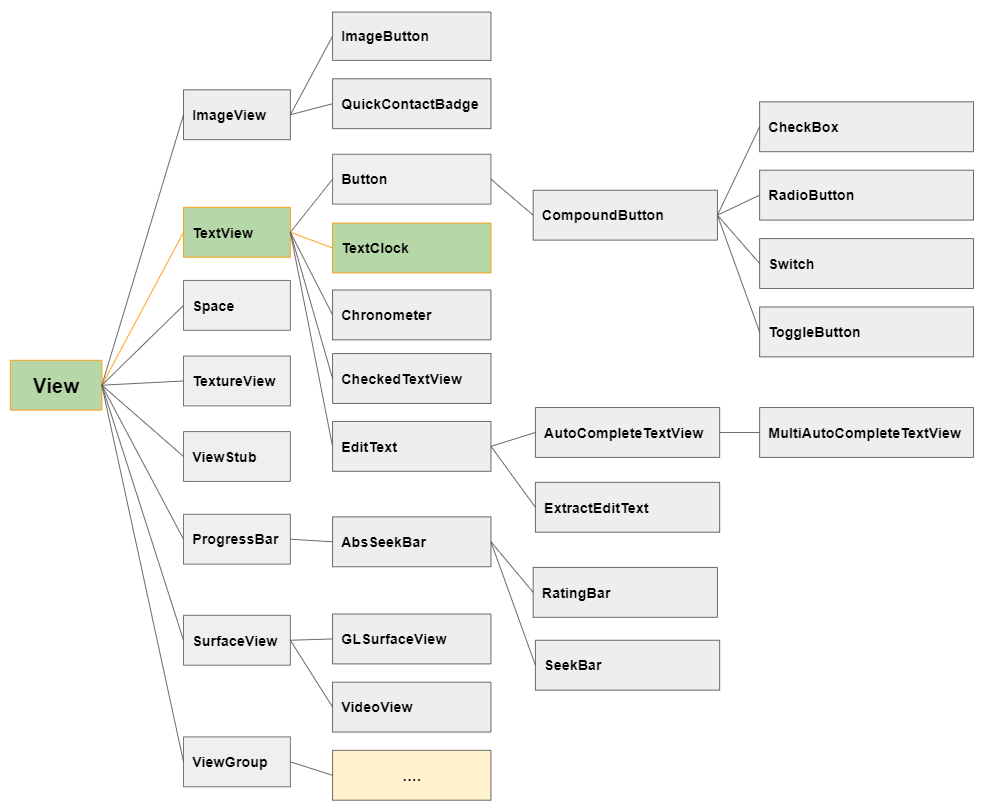
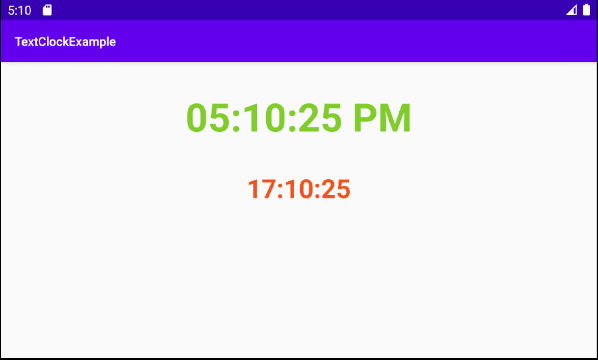
TextClock requires API Level 17 (Android 4.2) or a later version. So if you want to use the TextClock in your project, you need to change the value of minSdkVersion in the build.gradle (Module: app) file, and make sure it is greater than or equal to 17.
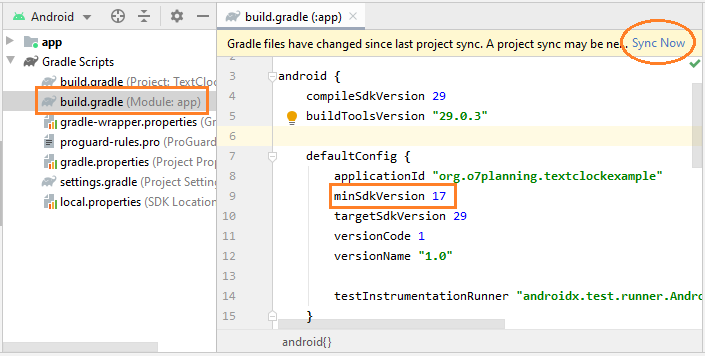
TextClock is not available in the Palette of the design window, probably because it is not a common component. Therefore, you need to use the following XML code to add it to the interface.
<!--
IMPORTANT NOTE:
You may get "Exception raised during rendering" error on design screen.
-->
<TextClock
android:id="@+id/textClock"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:format12Hour="hh:mm:ss a" />
Note: You may receive an error notification when you try to design the interface in the presence of the TextClock. This issue has been confirmed on Android Studio 3.x, 4.0. Some people have submitted their reports to Google to address the problem:
Exception raised during rendering.
java.lang.NullPointerException
at android.content.ContentResolver.registerContentObserver(ContentResolver.java:2263)
at android.widget.TextClock.registerObserver(TextClock.java:626)
at android.widget.TextClock.onAttachedToWindow(TextClock.java:545)
at android.view.View.dispatchAttachedToWindow(View.java:19575)
at android.view.ViewGroup.dispatchAttachedToWindow(ViewGroup.java:3437)
at android.view.ViewGroup.dispatchAttachedToWindow(ViewGroup.java:3437)
at android.view.ViewGroup.dispatchAttachedToWindow(ViewGroup.java:3437)
at android.view.ViewGroup.dispatchAttachedToWindow(ViewGroup.java:3437)
at android.view.AttachInfo_Accessor.setAttachInfo(AttachInfo_Accessor.java:42)
at com.android.layoutlib.bridge.impl.RenderSessionImpl.inflate(RenderSessionImpl.java:335)
at com.android.layoutlib.bridge.Bridge.createSession(Bridge.java:396)
at com.android.tools.idea.layoutlib.LayoutLibrary.createSession(LayoutLibrary.java:209)
at com.android.tools.idea.rendering.RenderTask.createRenderSession(RenderTask.java:608)
at com.android.tools.idea.rendering.RenderTask.lambda$inflate$6(RenderTask.java:734)
at java.util.concurrent.CompletableFuture$AsyncSupply.run(CompletableFuture.java:1590)
at java.util.concurrent.ThreadPoolExecutor.runWorker(ThreadPoolExecutor.java:1149)
at java.util.concurrent.ThreadPoolExecutor$Worker.run(ThreadPoolExecutor.java:624)
at java.lang.Thread.run(Thread.java:748)
Anyway, I have resolved this problem by creating a subclass of the TextClock and using it (See more in the example).
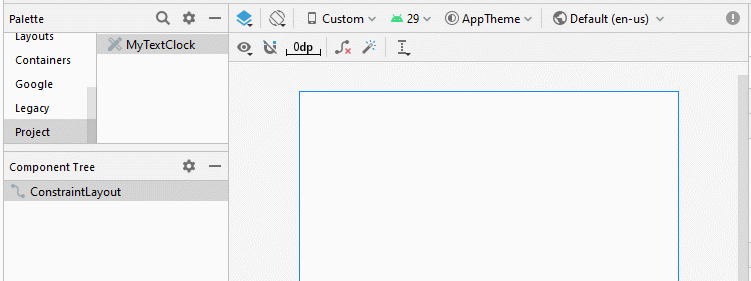
TextClock has two modes of time display:
- 12Hour Mode
- 24Hour Mode
First, the TextClock object will call the is24HourModeEnabled() method to check if the user's device is using 24-hour mode. Note: User can switch between 12-hour mode and 24-hour mode in the Settings section of the device.
There are 2 possibilities:
1 - If the user's device is in 24-hour mode.
- TextClock will display the time according to the format of the value returned from getFormat24Hour(), in case it is not null.
- Otherwise, it will display the time according to the format of the value returned from getFormat12Hour(), in case it is not null.
- Otherwise, it will display the time in the default format, "h:mm a", for example.
2 - If the user's device is in 12-hour mode,
- TextClock will display the time according to the format of the value returned from getFormat12Hour(), in case it is not null.
- Otherwise, it will display the time according to the format of the value returned from getFormat24Hour(), in case it is not null.
- Otherwise, it will display the time in the default format, "h:mm a", for example.
2. Example of TextClock
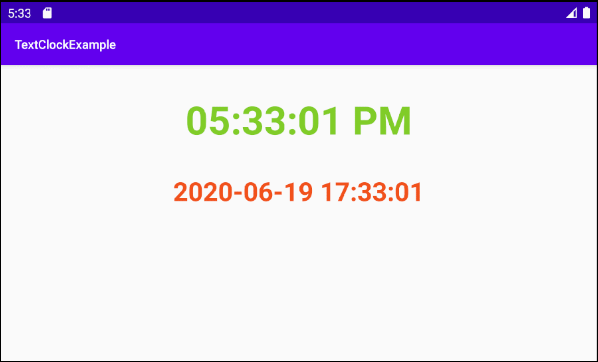
Great, now on Android Studio create a project:
- File > New > New Project > Empty Activity
- Name: TextClockExample
- Package name: org.o7planning.textclockexample
- Language: Java
TextClock requires API Level 17 (Android 4.2) or a later version. So if you want to use the TextClock in your project, you need to change the value of minSdkVersion in the build.gradle (Module: app) file, and make sure it is greater than or equal to 17.
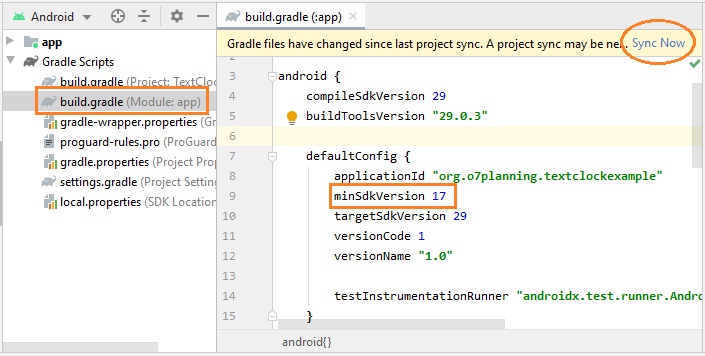
As mentioned above, you may receive an error notification when attempting to design the interface in the presence of the TextClock. It is possible that the issue will be addressed by Google in later versions of Android Studio. But for now, to overcome this problem, we create the MyTextClock class extending from the TextClock, then use it.
MyTextClock.java
package org.o7planning.textclockexample;
import android.content.Context;
import android.os.Build;
import android.util.AttributeSet;
import android.widget.TextClock;
import androidx.annotation.RequiresApi;
public class MyTextClock extends TextClock {
public MyTextClock(Context context) {
super(context);
//
this.setDesigningText();
}
public MyTextClock(Context context, AttributeSet attrs) {
super(context, attrs);
//
this.setDesigningText();
}
public MyTextClock(Context context, AttributeSet attrs, int defStyleAttr) {
super(context, attrs, defStyleAttr);
//
this.setDesigningText();
}
@RequiresApi(api = Build.VERSION_CODES.LOLLIPOP)
public MyTextClock(Context context, AttributeSet attrs, int defStyleAttr, int defStyleRes) {
super(context, attrs, defStyleAttr, defStyleRes);
//
this.setDesigningText();
}
private void setDesigningText() {
// The default text is displayed when designing the interface.
this.setText("11:30:00");
}
//
// Fix error: Exception raised during rendering.
//
// java.lang.NullPointerException
// at android.content.ContentResolver.registerContentObserver(ContentResolver.java:2263)
// at android.widget.TextClock.registerObserver(TextClock.java:626)
// at android.widget.TextClock.onAttachedToWindow(TextClock.java:545)
// at android.view.View.dispatchAttachedToWindow(View.java:19575)
// at android.view.ViewGroup.dispatchAttachedToWindow(ViewGroup.java:3437)
// at android.view.ViewGroup.dispatchAttachedToWindow(ViewGroup.java:3437)
// at android.view.ViewGroup.dispatchAttachedToWindow(ViewGroup.java:3437)
// at android.view.ViewGroup.dispatchAttachedToWindow(ViewGroup.java:3437)
// at android.view.AttachInfo_Accessor.setAttachInfo(AttachInfo_Accessor.java:42)
// at com.android.layoutlib.bridge.impl.RenderSessionImpl.inflate(RenderSessionImpl.java:335)
// at com.android.layoutlib.bridge.Bridge.createSession(Bridge.java:396)
// at com.android.tools.idea.layoutlib.LayoutLibrary.createSession(LayoutLibrary.java:209)
// at com.android.tools.idea.rendering.RenderTask.createRenderSession(RenderTask.java:608)
// at com.android.tools.idea.rendering.RenderTask.lambda$inflate$6(RenderTask.java:734)
// at java.util.concurrent.CompletableFuture$AsyncSupply.run(CompletableFuture.java:1590)
// at java.util.concurrent.ThreadPoolExecutor.runWorker(ThreadPoolExecutor.java:1149)
// at java.util.concurrent.ThreadPoolExecutor$Worker.run(ThreadPoolExecutor.java:624)
// at java.lang.Thread.run(Thread.java:748)
@Override
protected void onAttachedToWindow() {
try {
super.onAttachedToWindow();
} catch(Exception e) {
}
}
}
Design the application interface:
Note: You need to Re-Build project, and then you will see MyTextClock appear on the Palette of design window.
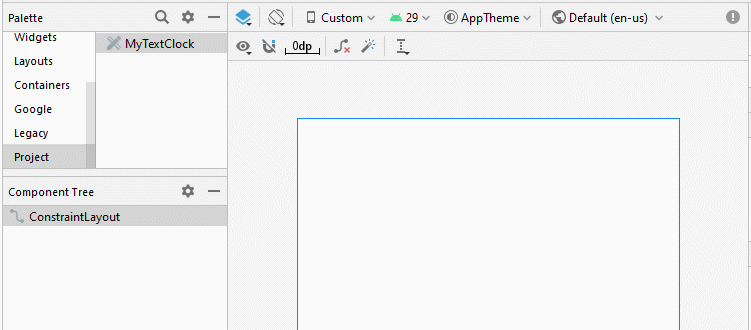
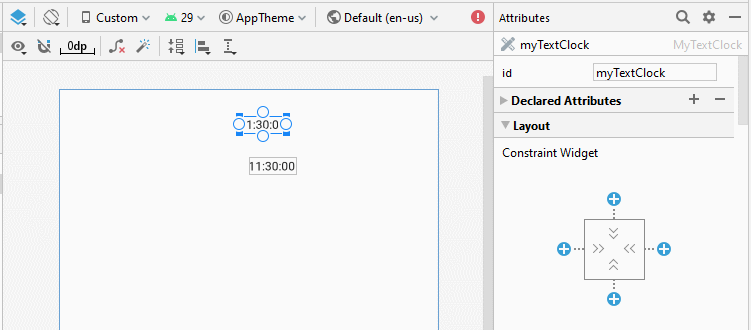
Set textSize, textStyle, textColor, format12Hour, format24Hour for TextClock(s).
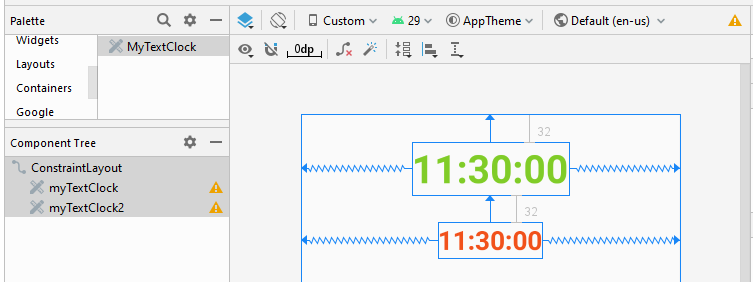
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<!-- 12 Hour Mode -->
<org.o7planning.textclockexample.MyTextClock
android:id="@+id/myTextClock"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="32dp"
android:format12Hour="hh:mm:ss a"
android:format24Hour="@null"
android:textColor="#80CC28"
android:textSize="45dp"
android:textStyle="bold"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<!-- 24 Hour Mode -->
<org.o7planning.textclockexample.MyTextClock
android:id="@+id/myTextClock2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="32dp"
android:format12Hour="@null"
android:format24Hour="yyyy-MM-dd HH:mm:ss"
android:textColor="#F1511B"
android:textSize="30dp"
android:textStyle="bold"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/myTextClock" />
</androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.java
package org.o7planning.textclockexample;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.widget.TextClock;
public class MainActivity extends AppCompatActivity {
private TextClock textClock;
private TextClock textClock2;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.textClock = (TextClock) this.findViewById(R.id.myTextClock);
this.textClock2 = (TextClock) this.findViewById(R.id.myTextClock2);
// Disable 24 Hour mode (To use 12 Hour mode).
// (Make sure getFormat12Hour() is not null).
this.textClock.setFormat24Hour(null);
// Disable 12 Hour mode (To use 24 Hour mode).
// (Make sure getFormat24Hour() is not null).
this.textClock2.setFormat12Hour(null);
}
}
Android Programming Tutorials
- Configure Android Emulator in Android Studio
- Android ToggleButton Tutorial with Examples
- Create a simple File Finder Dialog in Android
- Android TimePickerDialog Tutorial with Examples
- Android DatePickerDialog Tutorial with Examples
- What is needed to get started with Android?
- Install Android Studio on Windows
- Install Intel® HAXM for Android Studio
- Android AsyncTask Tutorial with Examples
- Android AsyncTaskLoader Tutorial with Examples
- Android Tutorial for Beginners - Basic examples
- How to know the phone number of Android Emulator and change it
- Android TextInputLayout Tutorial with Examples
- Android CardView Tutorial with Examples
- Android ViewPager2 Tutorial with Examples
- Get Phone Number in Android using TelephonyManager
- Android Phone Call Tutorial with Examples
- Android Wifi Scanning Tutorial with Examples
- Android 2D Game Tutorial for Beginners
- Android DialogFragment Tutorial with Examples
- Android CharacterPickerDialog Tutorial with Examples
- Android Tutorial for Beginners - Hello Android
- Using Android Device File Explorer
- Enable USB Debugging on Android Device
- Android UI Layouts Tutorial with Examples
- Android SMS Tutorial with Examples
- Android SQLite Database Tutorial with Examples
- Google Maps Android API Tutorial with Examples
- Android Text to Speech Tutorial with Examples
- Android Space Tutorial with Examples
- Android Toast Tutorial with Examples
- Create a custom Android Toast
- Android SnackBar Tutorial with Examples
- Android TextView Tutorial with Examples
- Android TextClock Tutorial with Examples
- Android EditText Tutorial with Examples
- Android TextWatcher Tutorial with Examples
- Format Credit Card Number with Android TextWatcher
- Android Clipboard Tutorial with Examples
- Create a simple File Chooser in Android
- Android AutoCompleteTextView and MultiAutoCompleteTextView Tutorial with Examples
- Android ImageView Tutorial with Examples
- Android ImageSwitcher Tutorial with Examples
- Android ScrollView and HorizontalScrollView Tutorial with Examples
- Android WebView Tutorial with Examples
- Android SeekBar Tutorial with Examples
- Android Dialog Tutorial with Examples
- Android AlertDialog Tutorial with Examples
- Android RatingBar Tutorial with Examples
- Android ProgressBar Tutorial with Examples
- Android Spinner Tutorial with Examples
- Android Button Tutorial with Examples
- Android Switch Tutorial with Examples
- Android ImageButton Tutorial with Examples
- Android FloatingActionButton Tutorial with Examples
- Android CheckBox Tutorial with Examples
- Android RadioGroup and RadioButton Tutorial with Examples
- Android Chip and ChipGroup Tutorial with Examples
- Using image assets and icon assets of Android Studio
- Setting SD Card for Android Emulator
- ChipGroup and Chip Entry Example
- How to add external libraries to Android Project in Android Studio?
- How to disable the permissions already granted to the Android application?
- How to remove applications from Android Emulator?
- Android LinearLayout Tutorial with Examples
- Android TableLayout Tutorial with Examples
- Android FrameLayout Tutorial with Examples
- Android QuickContactBadge Tutorial with Examples
- Android StackView Tutorial with Examples
- Android Camera Tutorial with Examples
- Android MediaPlayer Tutorial with Examples
- Android VideoView Tutorial with Examples
- Playing Sound effects in Android with SoundPool
- Android Networking Tutorial with Examples
- Android JSON Parser Tutorial with Examples
- Android SharedPreferences Tutorial with Examples
- Android Internal Storage Tutorial with Examples
- Android External Storage Tutorial with Examples
- Android Intents Tutorial with Examples
- Example of an explicit Android Intent, calling another Intent
- Example of implicit Android Intent, open a URL, send an email
- Android Services Tutorial with Examples
- Android Notifications Tutorial with Examples
- Android DatePicker Tutorial with Examples
- Android TimePicker Tutorial with Examples
- Android Chronometer Tutorial with Examples
- Android OptionMenu Tutorial with Examples
- Android ContextMenu Tutorial with Examples
- Android PopupMenu Tutorial with Examples
- Android Fragments Tutorial with Examples
- Android ListView Tutorial with Examples
- Android ListView with Checkbox using ArrayAdapter
- Android GridView Tutorial with Examples
Show More