Android Button Tutorial with Examples
1. Android Button
In Android, Button is a "user interface control" which is used to perform an action when the user clicks on it.
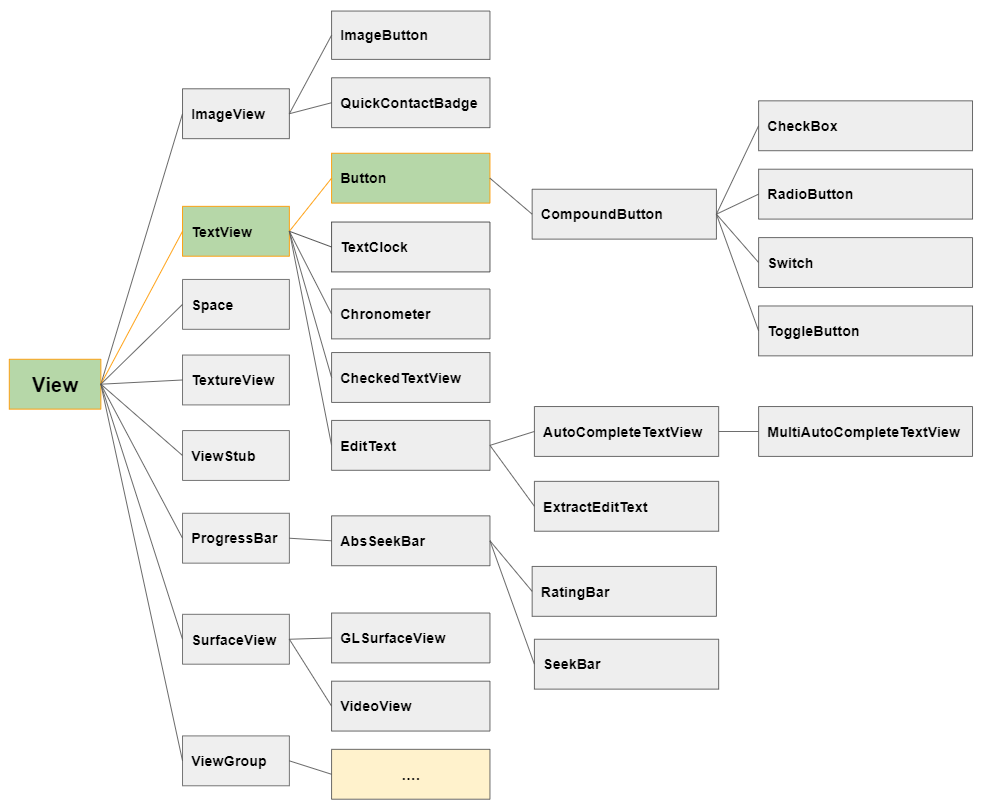
In the class hierarchy, Button is a subclass of TextView, so it inherits all the features of a TextView.
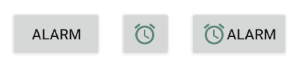
android:textAllCaps
By default, when displaying content, the text of Button will be turned into uppercase; therefore, you should set android:textAllCaps="false" to ensure that the text content will be correctly displayed as the original.
<Button
android:id="@+id/button3"
android:text="Alarm"
android:drawableLeft="@drawable/icon_alarm"
android:textAllCaps="false"
... />
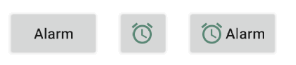
android:gravity
The android:gravity attribute is used to set the text display position of a Button. Its value is a combination of the following values:
Constant in Java | Value | Description |
Gravity.LEFT | left | |
Gravity.CENTER_HORIZONTAL | center_horizontal | |
Gravity.RIGHT | right | |
Gravity.TOP | top | |
Gravity.CENTER_VERTICAL | center_vertical | |
Gravity.BOTTOM | bottom | |
Gravity.START | start | |
Gravity.END | end | |
Gravity.CENTER | center | |
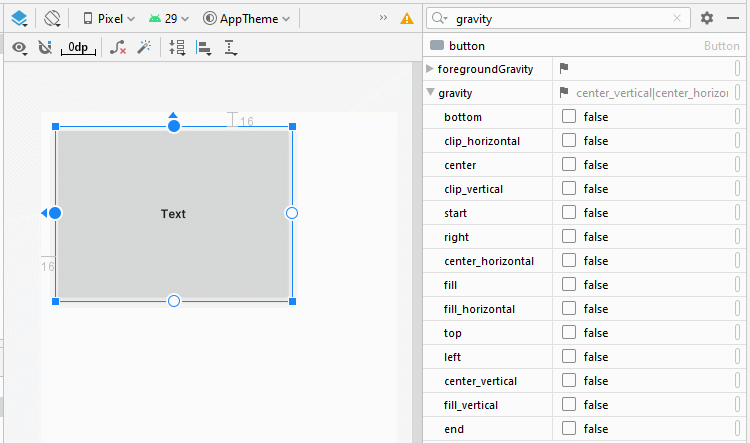
<Button
android:id="@+id/button"
android:gravity="center_horizontal|top"
android:text="Text"
... />
Icon - android:drawableLef, android:drawableTop,..
Android allows you to add four icons into a Button through the attributes of android:drawableLef, android:drawableTop, android:drawableRight, android:drawableBottom, android:drawableStart, android:drawableEnd.
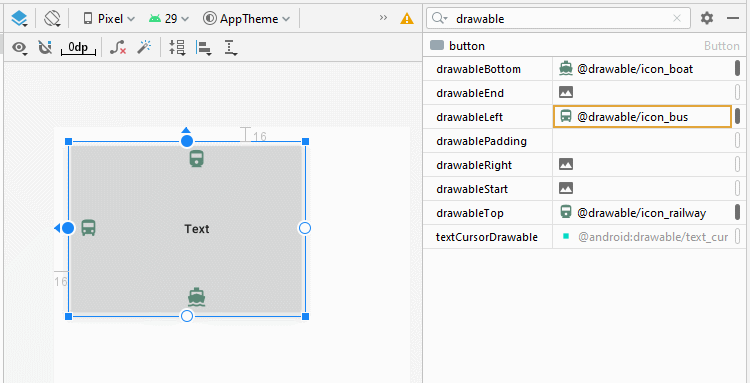
<Button
android:id="@+id/button"
android:drawableLeft="@drawable/icon_bus"
android:drawableTop="@drawable/icon_railway"
android:drawableRight="@drawable/icon_car"
android:drawableBottom="@drawable/icon_boat"
android:text="Text"
... />
Android 4.1 started to support text layout differences among different languages. More specifically, in English, text is written from left to right, while in Arabic languages, text is from right to left.
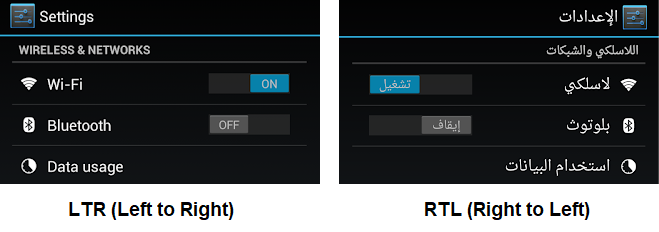
LTR (Left to Right)
In LTR (Left to Right) layout mode: The android:drawableStart attribute will work in the same way as the android:drawableLeft, and the android:drawableEnd will work in the same way as the android:drawableRight.
RTL (Right to Left)
In RTL (Right to Left) layout mode: The android:drawableStart attribute will work in the same way as the android:drawableRight, and android:drawableEnd attribute will work in the same way as the android:drawableStart.
- Android LTR, RTL Support
2. Button Click Event
Click event happens after the user presses down and releases the Button.
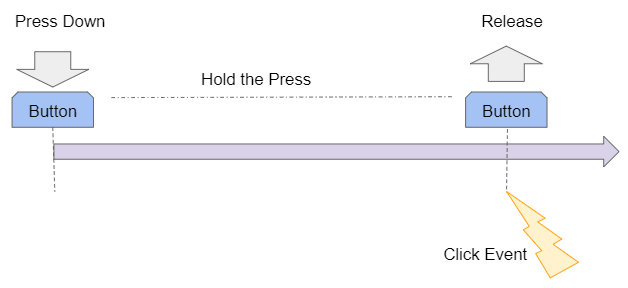
You can define the name of the method which will be called when the user clicks on the Button byusing the android.onClick attribute.
<Button
android:id="@+id/button_clickMe"
android:onClick="onClickHandler"
android:text="Click Me"
... />
Simultaneously create this method in MainActivity class.
// MainActivity
public void onClickHandler(View view) {
Toast.makeText(this, "You click on 'Click Me' button!", Toast.LENGTH_SHORT).show();
}
You can define the method that will be called when the user clicks on the Button by Java code:
this.buttonClickMe = (Button) this.findViewById(R.id.button_clickMe);
this.buttonClickMe.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Toast.makeText(MainActivity.this, "You click on 'Click Me' button!", Toast.LENGTH_SHORT).show();
}
});
3. Button Long Click Event
Long Click events in Android happen when the user presses down and hold the View for a long time. Specifically, the event will happen at the LONG_PRESS_TIMEOUT milliseconds since the user presses it down.You can get the value of the LONG_PRESS_TIMEOUT from the ViewConfiguration.getLongPressTimeout() method.
The default duration of a Long-Click in Android is DEFAULT_LONG_PRESS_TIMEOUT milliseconds. The user can change the Long-Click duration in the Settings of the device, which will be applied for all applications in the system. Application developers are not able to change this value.
Constant (private) | Method | Value (Milliseconds) |
DEFAULT_LONG_PRESS_TIMEOUT | 500 | |
ViewConfiguration.getLongPressTimeout() | 500 (default) |
For example: Handling the event when the user Long Click ina Button by Java codes (Note that you cannot do this with XML).
this.buttonClickMe = (Button) this.findViewById(R.id.button_clickMe);
this.buttonClickMe.setOnLongClickListener(new View.OnLongClickListener() {
@Override
public boolean onLongClick(View v) {
Toast.makeText(MainActivity.this, "You long click on 'Click Me' button!", Toast.LENGTH_SHORT).show();
return true;
}
});
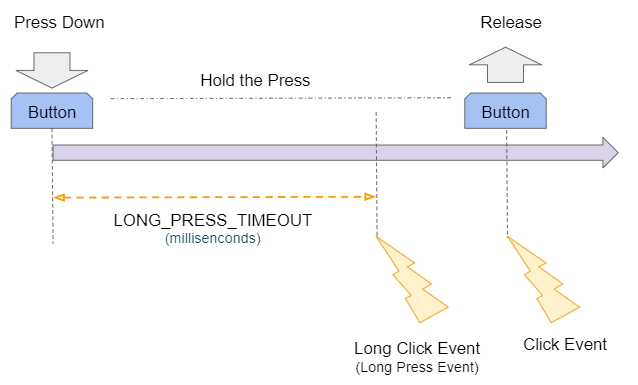
If the user clicks on View for a long time (longer than LONG_PRESS_TIMEOUT milliseconds), that action can generate two consecutive events, Long-Click and Click.
The onLongClick(View) method returns a boolean value. Returning true means that you have consumed the Long-Click event, and the Click event which happens after that will be ignored. Otherwise, if the onLongClick(View) method returns the false value, it means the Click event which happens after that will be executed.
4. Example of Android Button
Here is a simple example: the user will enter two numbers, and click the Button to sum these two numbers.
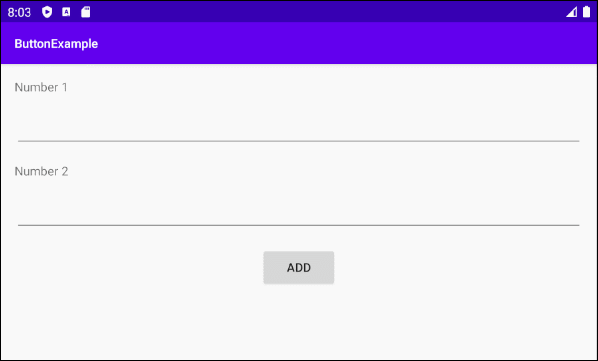
Design the example interface:
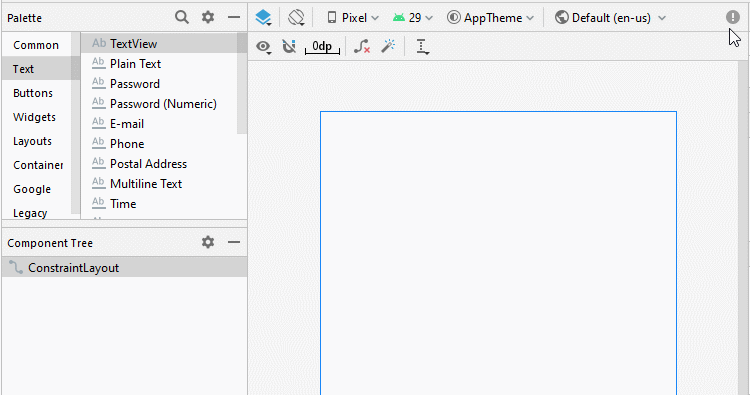
Align the position of components in the interface:
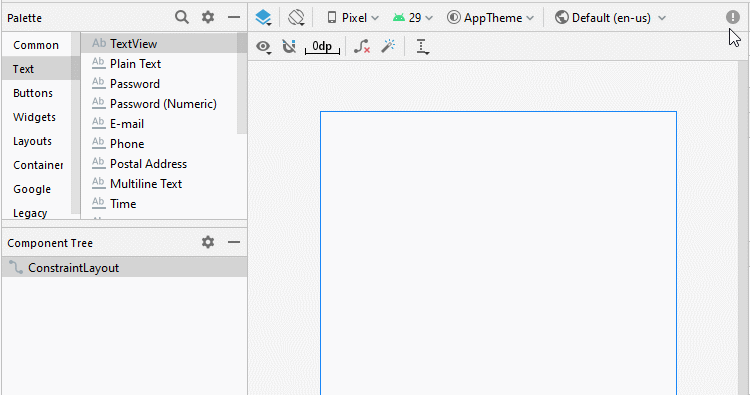
Set ID, and Text for the components in the interface:
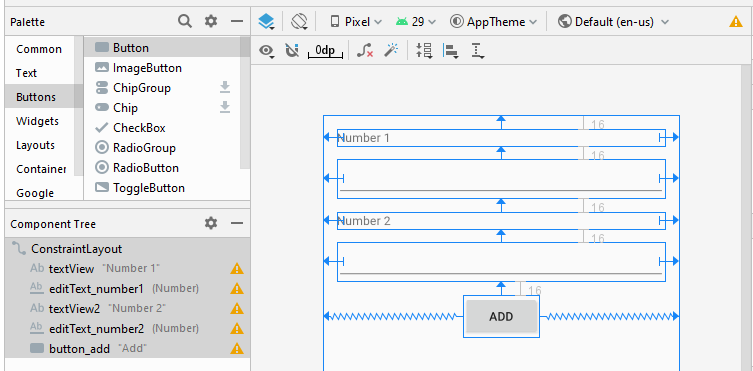
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Number 1"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<EditText
android:id="@+id/editText_number1"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:ems="10"
android:inputType="number"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView" />
<TextView
android:id="@+id/textView2"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Number 2"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/editText_number1" />
<EditText
android:id="@+id/editText_number2"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:ems="10"
android:inputType="number"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView2" />
<Button
android:id="@+id/button_add"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="16dp"
android:text="Add"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/editText_number2" />
</androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.java
package org.o7planning.buttonexample;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.view.ViewConfiguration;
import android.widget.Button;
import android.widget.EditText;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
private EditText editTextNumber1;
private EditText editTextNumber2;
private Button buttonAdd;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.editTextNumber1 = (EditText) this.findViewById(R.id.editText_number1);
this.editTextNumber2 = (EditText) this.findViewById(R.id.editText_number2);
this.buttonAdd = (Button) this.findViewById(R.id.button_add);
this.buttonAdd.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
add2Number();
}
});
}
private void add2Number() {
String str1 = this.editTextNumber1.getText().toString();
String str2 = this.editTextNumber2.getText().toString();
try {
double value1 = Double.parseDouble(str1);
double value2 = Double.parseDouble(str2);
double result = value1 + value2;
Toast.makeText(this, "Result: " + result, Toast.LENGTH_SHORT).show();
} catch(Exception e) {
Toast.makeText(this, "Error: "+ e, Toast.LENGTH_SHORT).show();
}
}
}
Android Programming Tutorials
- Configure Android Emulator in Android Studio
- Android ToggleButton Tutorial with Examples
- Create a simple File Finder Dialog in Android
- Android TimePickerDialog Tutorial with Examples
- Android DatePickerDialog Tutorial with Examples
- What is needed to get started with Android?
- Install Android Studio on Windows
- Install Intel® HAXM for Android Studio
- Android AsyncTask Tutorial with Examples
- Android AsyncTaskLoader Tutorial with Examples
- Android Tutorial for Beginners - Basic examples
- How to know the phone number of Android Emulator and change it
- Android TextInputLayout Tutorial with Examples
- Android CardView Tutorial with Examples
- Android ViewPager2 Tutorial with Examples
- Get Phone Number in Android using TelephonyManager
- Android Phone Call Tutorial with Examples
- Android Wifi Scanning Tutorial with Examples
- Android 2D Game Tutorial for Beginners
- Android DialogFragment Tutorial with Examples
- Android CharacterPickerDialog Tutorial with Examples
- Android Tutorial for Beginners - Hello Android
- Using Android Device File Explorer
- Enable USB Debugging on Android Device
- Android UI Layouts Tutorial with Examples
- Android SMS Tutorial with Examples
- Android SQLite Database Tutorial with Examples
- Google Maps Android API Tutorial with Examples
- Android Text to Speech Tutorial with Examples
- Android Space Tutorial with Examples
- Android Toast Tutorial with Examples
- Create a custom Android Toast
- Android SnackBar Tutorial with Examples
- Android TextView Tutorial with Examples
- Android TextClock Tutorial with Examples
- Android EditText Tutorial with Examples
- Android TextWatcher Tutorial with Examples
- Format Credit Card Number with Android TextWatcher
- Android Clipboard Tutorial with Examples
- Create a simple File Chooser in Android
- Android AutoCompleteTextView and MultiAutoCompleteTextView Tutorial with Examples
- Android ImageView Tutorial with Examples
- Android ImageSwitcher Tutorial with Examples
- Android ScrollView and HorizontalScrollView Tutorial with Examples
- Android WebView Tutorial with Examples
- Android SeekBar Tutorial with Examples
- Android Dialog Tutorial with Examples
- Android AlertDialog Tutorial with Examples
- Android RatingBar Tutorial with Examples
- Android ProgressBar Tutorial with Examples
- Android Spinner Tutorial with Examples
- Android Button Tutorial with Examples
- Android Switch Tutorial with Examples
- Android ImageButton Tutorial with Examples
- Android FloatingActionButton Tutorial with Examples
- Android CheckBox Tutorial with Examples
- Android RadioGroup and RadioButton Tutorial with Examples
- Android Chip and ChipGroup Tutorial with Examples
- Using image assets and icon assets of Android Studio
- Setting SD Card for Android Emulator
- ChipGroup and Chip Entry Example
- How to add external libraries to Android Project in Android Studio?
- How to disable the permissions already granted to the Android application?
- How to remove applications from Android Emulator?
- Android LinearLayout Tutorial with Examples
- Android TableLayout Tutorial with Examples
- Android FrameLayout Tutorial with Examples
- Android QuickContactBadge Tutorial with Examples
- Android StackView Tutorial with Examples
- Android Camera Tutorial with Examples
- Android MediaPlayer Tutorial with Examples
- Android VideoView Tutorial with Examples
- Playing Sound effects in Android with SoundPool
- Android Networking Tutorial with Examples
- Android JSON Parser Tutorial with Examples
- Android SharedPreferences Tutorial with Examples
- Android Internal Storage Tutorial with Examples
- Android External Storage Tutorial with Examples
- Android Intents Tutorial with Examples
- Example of an explicit Android Intent, calling another Intent
- Example of implicit Android Intent, open a URL, send an email
- Android Services Tutorial with Examples
- Android Notifications Tutorial with Examples
- Android DatePicker Tutorial with Examples
- Android TimePicker Tutorial with Examples
- Android Chronometer Tutorial with Examples
- Android OptionMenu Tutorial with Examples
- Android ContextMenu Tutorial with Examples
- Android PopupMenu Tutorial with Examples
- Android Fragments Tutorial with Examples
- Android ListView Tutorial with Examples
- Android ListView with Checkbox using ArrayAdapter
- Android GridView Tutorial with Examples
Show More