Android PopupMenu Tutorial with Examples
1. Android Popup Menu
In Android, Popup Menu is a floating menu that you can create and anchor it to any View. In terms of its interface and use, there is no difference as opposed to a Context Menu.
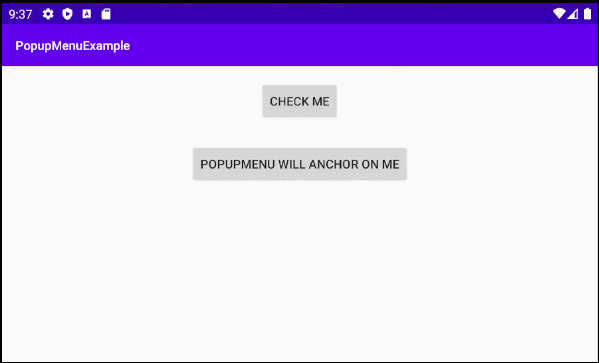
You can use Android Resource File (XML) to design the interface of a PopupMenu. However, the PopupMenu of an application is quite simple, so creating a PopupMenu completely from Java code is also a good choice (See more in the example below).
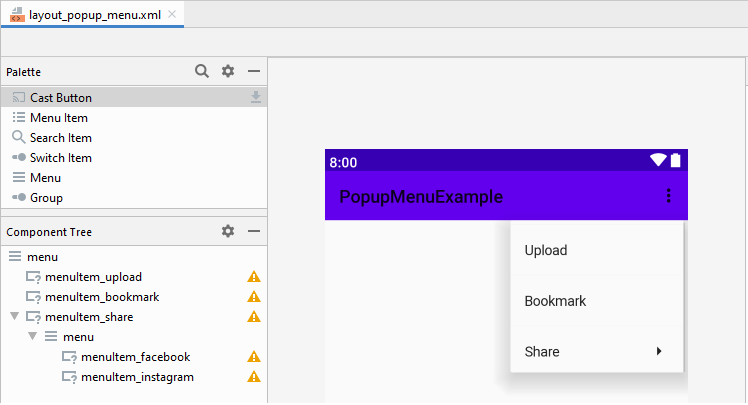
Note: Some attributes of the <item> (Menu Item) have no effect when placed in a Popup Menu, for example:
- app:showAsAction
- android:icon (Not work in Android 3.0+/API 11+)
2. An example of the Popup Menu
On Android Studio, create a new project.
- File > New > New Project > Empty Activity
- Name: PopupMenu
- Package name: org.o7planning.popupmenuexample
- Language: Java
Note: Since Android 3.0 (API 11), PopupMenu have no longer supported icons. However, you can copy some icons below into the drawable folder to support older Android devices, or you can just skip this step.
icon_upload.png | icon_bookmark.png | icon_share.png |
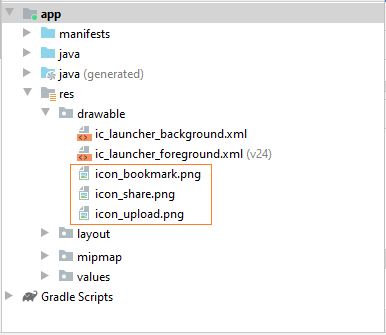
The main interface of the application is very simple with 2 Buttons. When users click on Button1, a PopupMenu will be displayed and anchored into Button2.
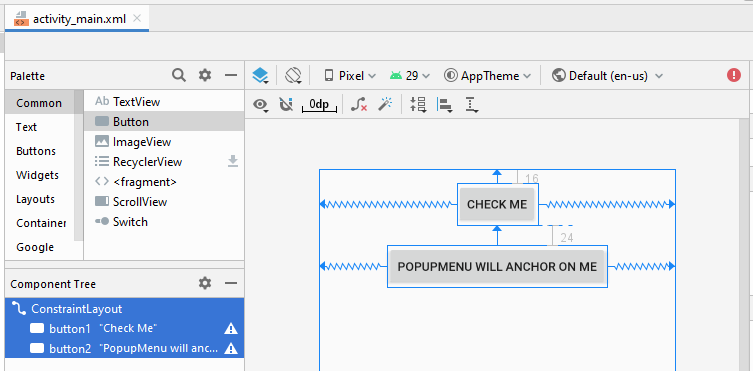
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<Button
android:id="@+id/button1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="16dp"
android:text="Check Me"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/button2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="24dp"
android:text="PopupMenu will anchor on me"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/button1" />
</androidx.constraintlayout.widget.ConstraintLayout>
On Android Studio, select:
- File > New > Android Resource File
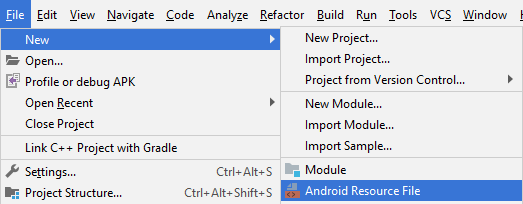
- File name: layout_popup_menu.xml
- Resource type: Menu
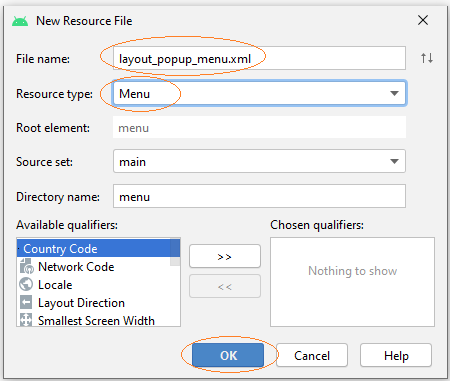
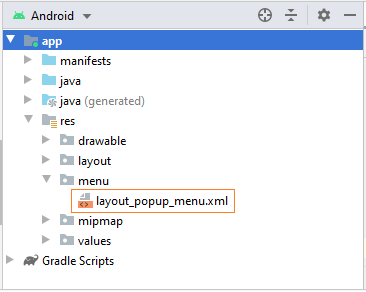
On Android Studio, design the interface of the Popup Menu:
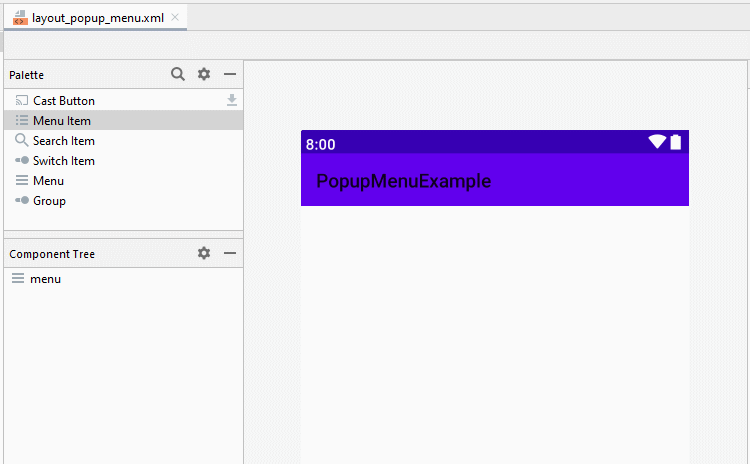
Later, set ID, Title and Icon for the Menu Items:
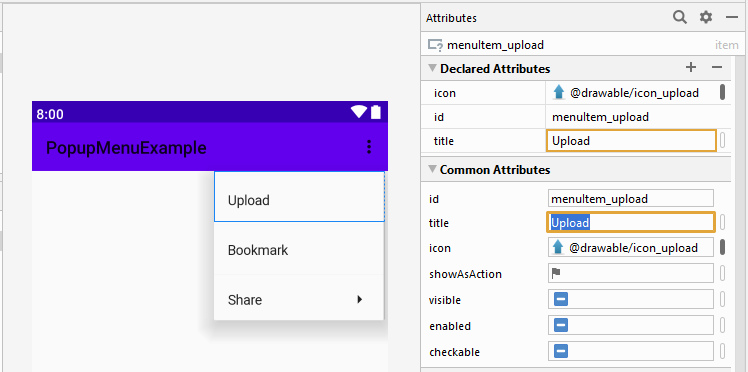
layout_popup_menu.xml
<?xml version="1.0" encoding="utf-8"?>
<menu xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:android="http://schemas.android.com/apk/res/android">
<item
android:id="@+id/menuItem_upload"
android:icon="@drawable/icon_upload"
android:title="Upload" />
<item
android:id="@+id/menuItem_bookmark"
android:icon="@drawable/icon_bookmark"
android:title="Bookmark" />
<item
android:id="@+id/menuItem_share"
android:icon="@drawable/icon_share"
android:title="Share">
<menu>
<item
android:id="@+id/menuItem_facebook"
android:title="Facebook" />
<item
android:id="@+id/menuItem_instagram"
android:title="Instagram" />
</menu>
</item>
</menu>
MainActivity.java
package org.o7planning.popupmenuexample;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.util.Log;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import android.widget.Button;
import android.widget.PopupMenu;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
public static final String LOG_TAG = "PopupMenuExample";
private Button button1;
private Button button2;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.button1 = (Button) findViewById(R.id.button1);
this.button2 = (Button) findViewById(R.id.button2);
this.button1.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
button1Clicked( );
}
});
}
// User click on the Button 1.
private void button1Clicked( ) {
// When user click on the Button 1, create a PopupMenu.
// And anchor Popup to the Button 2.
PopupMenu popup = new PopupMenu(this, this.button2);
popup.inflate(R.menu.layout_popup_menu);
Menu menu = popup.getMenu();
// com.android.internal.view.menu.MenuBuilder
Log.i(LOG_TAG, "Menu class: " + menu.getClass().getName());
// Register Menu Item Click event.
popup.setOnMenuItemClickListener(new PopupMenu.OnMenuItemClickListener() {
@Override
public boolean onMenuItemClick(MenuItem item) {
return menuItemClicked(item);
}
});
// Show the PopupMenu.
popup.show();
}
// When user click on Menu Item.
// @return true if event was handled.
private boolean menuItemClicked(MenuItem item) {
switch (item.getItemId()) {
case R.id.menuItem_bookmark:
Toast.makeText(this, "Bookmark", Toast.LENGTH_SHORT).show();
break;
case R.id.menuItem_upload:
Toast.makeText(this, "Upload", Toast.LENGTH_SHORT).show();
break;
case R.id.menuItem_facebook:
Toast.makeText(this, "Share Facebook", Toast.LENGTH_SHORT).show();
break;
case R.id.menuItem_instagram:
Toast.makeText(this, "Share Instagram", Toast.LENGTH_SHORT).show();
break;
default:
Toast.makeText(this, item.getTitle(), Toast.LENGTH_SHORT).show();
break;
}
return true;
}
}
You can also create a PopupMenu by using Java code only:
// Create a PopupMenu using Java.
public void createPopupMenu(Context activity, View anchorView ) {
// Create a PopupMenu and anchor it on a View.
PopupMenu popupMenu = new PopupMenu(activity, anchorView);
Menu menu = popupMenu.getMenu();
// groupId, itemId, order, title
MenuItem menuItemUpload = menu.add(1, 1, 1, "Upload");
MenuItem menuItemBookmark = menu.add(2, 2, 2, "Bookmark");
menuItemUpload.setIcon(R.drawable.icon_upload);
menuItemBookmark.setIcon(R.drawable.icon_bookmark);
// groupId, itemId, order, title
SubMenu subMenuShare= menu.addSubMenu(3, 3, 3, "Share");
subMenuShare.setIcon(R.drawable.icon_share);
subMenuShare.add(4, 31, 1, "Google" );
subMenuShare.add(5, 32, 2, "Instagram");
}
Android Programming Tutorials
- Configure Android Emulator in Android Studio
- Android ToggleButton Tutorial with Examples
- Create a simple File Finder Dialog in Android
- Android TimePickerDialog Tutorial with Examples
- Android DatePickerDialog Tutorial with Examples
- What is needed to get started with Android?
- Install Android Studio on Windows
- Install Intel® HAXM for Android Studio
- Android AsyncTask Tutorial with Examples
- Android AsyncTaskLoader Tutorial with Examples
- Android Tutorial for Beginners - Basic examples
- How to know the phone number of Android Emulator and change it
- Android TextInputLayout Tutorial with Examples
- Android CardView Tutorial with Examples
- Android ViewPager2 Tutorial with Examples
- Get Phone Number in Android using TelephonyManager
- Android Phone Call Tutorial with Examples
- Android Wifi Scanning Tutorial with Examples
- Android 2D Game Tutorial for Beginners
- Android DialogFragment Tutorial with Examples
- Android CharacterPickerDialog Tutorial with Examples
- Android Tutorial for Beginners - Hello Android
- Using Android Device File Explorer
- Enable USB Debugging on Android Device
- Android UI Layouts Tutorial with Examples
- Android SMS Tutorial with Examples
- Android SQLite Database Tutorial with Examples
- Google Maps Android API Tutorial with Examples
- Android Text to Speech Tutorial with Examples
- Android Space Tutorial with Examples
- Android Toast Tutorial with Examples
- Create a custom Android Toast
- Android SnackBar Tutorial with Examples
- Android TextView Tutorial with Examples
- Android TextClock Tutorial with Examples
- Android EditText Tutorial with Examples
- Android TextWatcher Tutorial with Examples
- Format Credit Card Number with Android TextWatcher
- Android Clipboard Tutorial with Examples
- Create a simple File Chooser in Android
- Android AutoCompleteTextView and MultiAutoCompleteTextView Tutorial with Examples
- Android ImageView Tutorial with Examples
- Android ImageSwitcher Tutorial with Examples
- Android ScrollView and HorizontalScrollView Tutorial with Examples
- Android WebView Tutorial with Examples
- Android SeekBar Tutorial with Examples
- Android Dialog Tutorial with Examples
- Android AlertDialog Tutorial with Examples
- Android RatingBar Tutorial with Examples
- Android ProgressBar Tutorial with Examples
- Android Spinner Tutorial with Examples
- Android Button Tutorial with Examples
- Android Switch Tutorial with Examples
- Android ImageButton Tutorial with Examples
- Android FloatingActionButton Tutorial with Examples
- Android CheckBox Tutorial with Examples
- Android RadioGroup and RadioButton Tutorial with Examples
- Android Chip and ChipGroup Tutorial with Examples
- Using image assets and icon assets of Android Studio
- Setting SD Card for Android Emulator
- ChipGroup and Chip Entry Example
- How to add external libraries to Android Project in Android Studio?
- How to disable the permissions already granted to the Android application?
- How to remove applications from Android Emulator?
- Android LinearLayout Tutorial with Examples
- Android TableLayout Tutorial with Examples
- Android FrameLayout Tutorial with Examples
- Android QuickContactBadge Tutorial with Examples
- Android StackView Tutorial with Examples
- Android Camera Tutorial with Examples
- Android MediaPlayer Tutorial with Examples
- Android VideoView Tutorial with Examples
- Playing Sound effects in Android with SoundPool
- Android Networking Tutorial with Examples
- Android JSON Parser Tutorial with Examples
- Android SharedPreferences Tutorial with Examples
- Android Internal Storage Tutorial with Examples
- Android External Storage Tutorial with Examples
- Android Intents Tutorial with Examples
- Example of an explicit Android Intent, calling another Intent
- Example of implicit Android Intent, open a URL, send an email
- Android Services Tutorial with Examples
- Android Notifications Tutorial with Examples
- Android DatePicker Tutorial with Examples
- Android TimePicker Tutorial with Examples
- Android Chronometer Tutorial with Examples
- Android OptionMenu Tutorial with Examples
- Android ContextMenu Tutorial with Examples
- Android PopupMenu Tutorial with Examples
- Android Fragments Tutorial with Examples
- Android ListView Tutorial with Examples
- Android ListView with Checkbox using ArrayAdapter
- Android GridView Tutorial with Examples
Show More