Playing Sound effects in Android with SoundPool
1. SoundPool & AudioManager
First I put out a situation, you are creating a game, it plays out the sounds such as gunfire, bombs, that's the sound effects in the game. Android gives you SoundPool class, it's like a pool and ready to play sounds when requested.
SoundPool contains a set of source music, the sound source can be from music file in the app or in the file system, .. SoundPool support play music sources simultaneously.
SourcePool use MediaPlayer service to make a sound.
SoundPool contains a set of source music, the sound source can be from music file in the app or in the file system, .. SoundPool support play music sources simultaneously.
SourcePool use MediaPlayer service to make a sound.
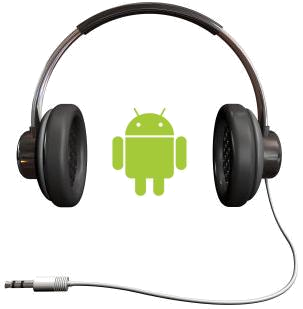
You can setup SoundPool to make a sound in specific stream type. There are several types of stream as follows:
Stream Type | Description |
AudioManager.STREAM_ALARM | The audio stream for alarms |
AudioManager.STREAM DTMF | The audio stream for DTMF Tones |
AudioManager.STREAM_MUSIC | The audio stream for music playback |
AudioManager.STREAM_NOTIFICATION | The audio stream for notifications |
AudioManager.STREAM_RING | The audio stream for the phone ring |
AudioManager.STREAM_SYSTEM | The audio stream for system sounds |
AudioManager.STREAM_VOICE_CALL | The audio stream for phone calls |
AudioManager allows you to adjust the volume on the different audio streams. For example, you are listening to songs on the device while playing games, you can change the volume of the game without affecting the volume of the song.
Other unmentioned thing is which audio device will produce a sound.
Using STREAM_MUSIC the sound will be produced through one audio device(phone speaker, earphone, bluetooth speaker or something else) connected to the phone.
Using STREAM_RING the sound will be produced through all audio device connected to the phone. This behaviour might be differed for each devices.
Using STREAM_MUSIC the sound will be produced through one audio device(phone speaker, earphone, bluetooth speaker or something else) connected to the phone.
Using STREAM_RING the sound will be produced through all audio device connected to the phone. This behaviour might be differed for each devices.
SoundPool API:
Phương thức | Mô tả |
int play(int soundID, float leftVolume, float rightVolume,
int priority, int loop, float rate) |
Play a sound source, and returns the ID of the newly stream is playing (streamID).
|
void pause(int streamID) | Pause sound stream with ID streamID. |
void stop(int streamID) | Stop sound stream with ID streamID. |
void setVolume(int streamID, float leftVolume, float rightVolume) | Set volumn for sound stream with ID is streamID |
void setLoop(int streamID, int loop) | Set loop number for sound stream with ID is streamID. |
2. SoundPool example
Create a project named SoundPoolDemo.
- Name: SoundPoolDemo
- Package name: org.o7planning.soundpooldemo
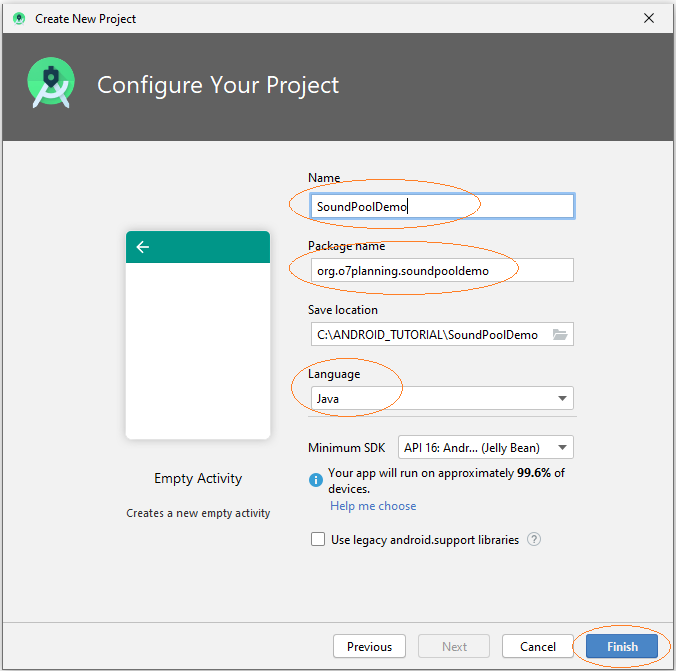
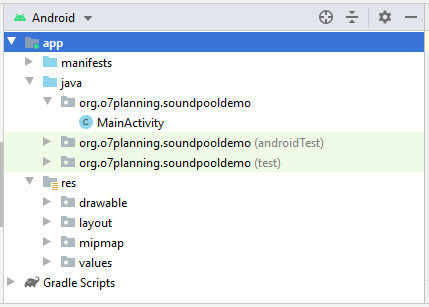
Create raw folder is sub-folder of res. Copy 2 music files to raw folder. Here, I copy two sound files: destroy.wav and gun.wav. Note that you can use mp3 or wav audio file extension.
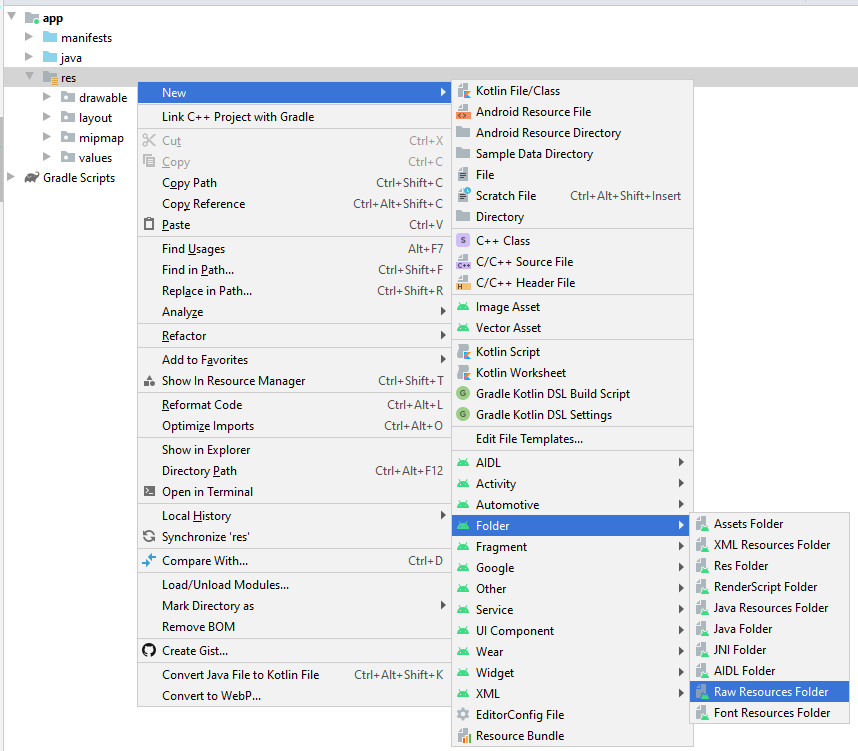
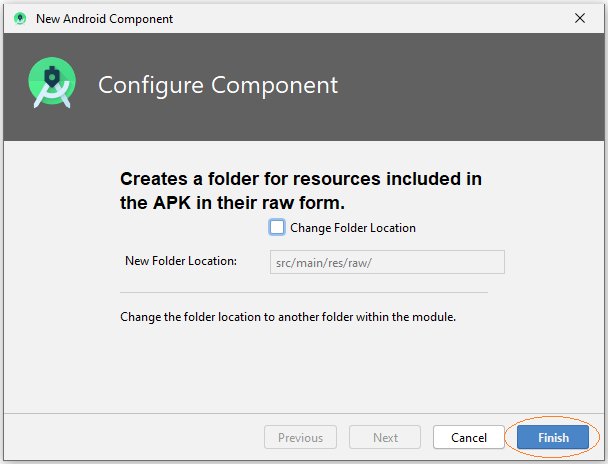
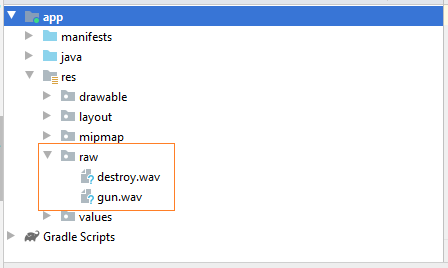
Application interface:
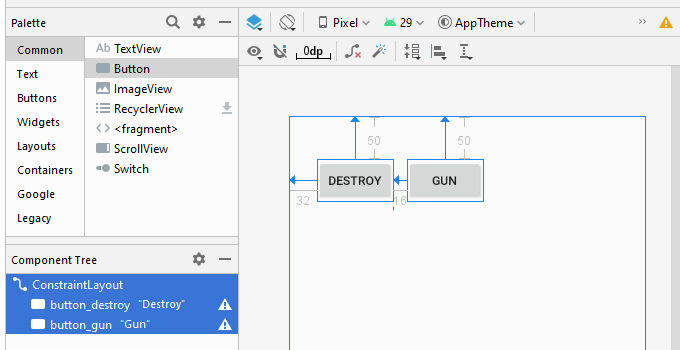
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<Button
android:id="@+id/button_destroy"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="32dp"
android:layout_marginLeft="32dp"
android:layout_marginTop="50dp"
android:text="Destroy"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/button_gun"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="50dp"
android:text="Gun"
app:layout_constraintStart_toEndOf="@+id/button_destroy"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.java
package org.o7planning.soundpooldemo;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.media.AudioAttributes;
import android.media.AudioManager;
import android.media.SoundPool;
import android.os.Build;
import android.view.View;
import android.widget.Button;
public class MainActivity extends AppCompatActivity {
private SoundPool soundPool;
private AudioManager audioManager;
private Button buttonDestroy;
private Button buttonGun;
// Maximumn sound stream.
private static final int MAX_STREAMS = 5;
// Stream type.
private static final int streamType = AudioManager.STREAM_MUSIC;
private boolean loaded;
private int soundIdDestroy;
private int soundIdGun;
private float volume;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.buttonDestroy = (Button) this.findViewById(R.id.button_destroy);
this.buttonGun = (Button) this.findViewById(R.id.button_gun);
this.buttonDestroy.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
playSoundDestroy();
}
});
this.buttonGun.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
playSoundGun();
}
});
// AudioManager audio settings for adjusting the volume
audioManager = (AudioManager) getSystemService(AUDIO_SERVICE);
// Current volumn Index of particular stream type.
float currentVolumeIndex = (float) audioManager.getStreamVolume(streamType);
// Get the maximum volume index for a particular stream type.
float maxVolumeIndex = (float) audioManager.getStreamMaxVolume(streamType);
// Volumn (0 --> 1)
this.volume = currentVolumeIndex / maxVolumeIndex;
// Suggests an audio stream whose volume should be changed by
// the hardware volume controls.
this.setVolumeControlStream(streamType);
// For Android SDK >= 21
if (Build.VERSION.SDK_INT >= 21 ) {
AudioAttributes audioAttrib = new AudioAttributes.Builder()
.setUsage(AudioAttributes.USAGE_GAME)
.setContentType(AudioAttributes.CONTENT_TYPE_SONIFICATION)
.build();
SoundPool.Builder builder= new SoundPool.Builder();
builder.setAudioAttributes(audioAttrib).setMaxStreams(MAX_STREAMS);
this.soundPool = builder.build();
}
// for Android SDK < 21
else {
// SoundPool(int maxStreams, int streamType, int srcQuality)
this.soundPool = new SoundPool(MAX_STREAMS, AudioManager.STREAM_MUSIC, 0);
}
// When Sound Pool load complete.
this.soundPool.setOnLoadCompleteListener(new SoundPool.OnLoadCompleteListener() {
@Override
public void onLoadComplete(SoundPool soundPool, int sampleId, int status) {
loaded = true;
}
});
// Load sound file (destroy.wav) into SoundPool.
this.soundIdDestroy = this.soundPool.load(this, R.raw.destroy,1);
// Load sound file (gun.wav) into SoundPool.
this.soundIdGun = this.soundPool.load(this, R.raw.gun,1);
}
// When users click on the button "Gun"
public void playSoundGun( ) {
if(loaded) {
float leftVolumn = volume;
float rightVolumn = volume;
// Play sound of gunfire. Returns the ID of the new stream.
int streamId = this.soundPool.play(this.soundIdGun,leftVolumn, rightVolumn, 1, 0, 1f);
}
}
// When users click on the button "Destroy"
public void playSoundDestroy( ) {
if(loaded) {
float leftVolumn = volume;
float rightVolumn = volume;
// Play sound objects destroyed. Returns the ID of the new stream.
int streamId = this.soundPool.play(this.soundIdDestroy,leftVolumn, rightVolumn, 1, 0, 1f);
}
}
}
Running apps:
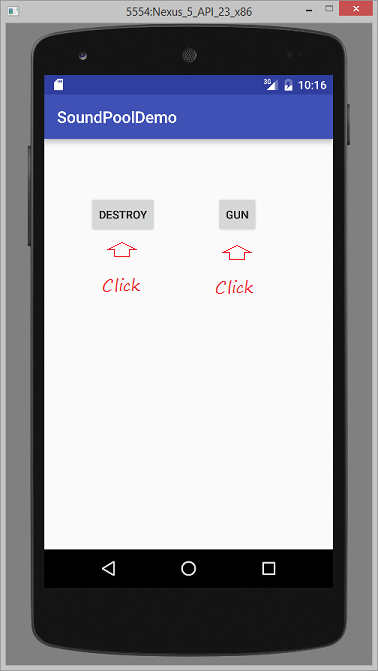
Android Programming Tutorials
- Configure Android Emulator in Android Studio
- Android ToggleButton Tutorial with Examples
- Create a simple File Finder Dialog in Android
- Android TimePickerDialog Tutorial with Examples
- Android DatePickerDialog Tutorial with Examples
- What is needed to get started with Android?
- Install Android Studio on Windows
- Install Intel® HAXM for Android Studio
- Android AsyncTask Tutorial with Examples
- Android AsyncTaskLoader Tutorial with Examples
- Android Tutorial for Beginners - Basic examples
- How to know the phone number of Android Emulator and change it
- Android TextInputLayout Tutorial with Examples
- Android CardView Tutorial with Examples
- Android ViewPager2 Tutorial with Examples
- Get Phone Number in Android using TelephonyManager
- Android Phone Call Tutorial with Examples
- Android Wifi Scanning Tutorial with Examples
- Android 2D Game Tutorial for Beginners
- Android DialogFragment Tutorial with Examples
- Android CharacterPickerDialog Tutorial with Examples
- Android Tutorial for Beginners - Hello Android
- Using Android Device File Explorer
- Enable USB Debugging on Android Device
- Android UI Layouts Tutorial with Examples
- Android SMS Tutorial with Examples
- Android SQLite Database Tutorial with Examples
- Google Maps Android API Tutorial with Examples
- Android Text to Speech Tutorial with Examples
- Android Space Tutorial with Examples
- Android Toast Tutorial with Examples
- Create a custom Android Toast
- Android SnackBar Tutorial with Examples
- Android TextView Tutorial with Examples
- Android TextClock Tutorial with Examples
- Android EditText Tutorial with Examples
- Android TextWatcher Tutorial with Examples
- Format Credit Card Number with Android TextWatcher
- Android Clipboard Tutorial with Examples
- Create a simple File Chooser in Android
- Android AutoCompleteTextView and MultiAutoCompleteTextView Tutorial with Examples
- Android ImageView Tutorial with Examples
- Android ImageSwitcher Tutorial with Examples
- Android ScrollView and HorizontalScrollView Tutorial with Examples
- Android WebView Tutorial with Examples
- Android SeekBar Tutorial with Examples
- Android Dialog Tutorial with Examples
- Android AlertDialog Tutorial with Examples
- Android RatingBar Tutorial with Examples
- Android ProgressBar Tutorial with Examples
- Android Spinner Tutorial with Examples
- Android Button Tutorial with Examples
- Android Switch Tutorial with Examples
- Android ImageButton Tutorial with Examples
- Android FloatingActionButton Tutorial with Examples
- Android CheckBox Tutorial with Examples
- Android RadioGroup and RadioButton Tutorial with Examples
- Android Chip and ChipGroup Tutorial with Examples
- Using image assets and icon assets of Android Studio
- Setting SD Card for Android Emulator
- ChipGroup and Chip Entry Example
- How to add external libraries to Android Project in Android Studio?
- How to disable the permissions already granted to the Android application?
- How to remove applications from Android Emulator?
- Android LinearLayout Tutorial with Examples
- Android TableLayout Tutorial with Examples
- Android FrameLayout Tutorial with Examples
- Android QuickContactBadge Tutorial with Examples
- Android StackView Tutorial with Examples
- Android Camera Tutorial with Examples
- Android MediaPlayer Tutorial with Examples
- Android VideoView Tutorial with Examples
- Playing Sound effects in Android with SoundPool
- Android Networking Tutorial with Examples
- Android JSON Parser Tutorial with Examples
- Android SharedPreferences Tutorial with Examples
- Android Internal Storage Tutorial with Examples
- Android External Storage Tutorial with Examples
- Android Intents Tutorial with Examples
- Example of an explicit Android Intent, calling another Intent
- Example of implicit Android Intent, open a URL, send an email
- Android Services Tutorial with Examples
- Android Notifications Tutorial with Examples
- Android DatePicker Tutorial with Examples
- Android TimePicker Tutorial with Examples
- Android Chronometer Tutorial with Examples
- Android OptionMenu Tutorial with Examples
- Android ContextMenu Tutorial with Examples
- Android PopupMenu Tutorial with Examples
- Android Fragments Tutorial with Examples
- Android ListView Tutorial with Examples
- Android ListView with Checkbox using ArrayAdapter
- Android GridView Tutorial with Examples
Show More