Android RadioGroup and RadioButton Tutorial with Examples
1. RadioButton & RadioGroup
RadioButton is a view in Android which is usually used with RadioGroup. In which, RadioGroup is container which contains RadioButton(s), when you check and chose a radio button in group, all other radio button in group will be deselected.
Here are image that RadioButton(s) grouped in different groups..
Here are image that RadioButton(s) grouped in different groups..
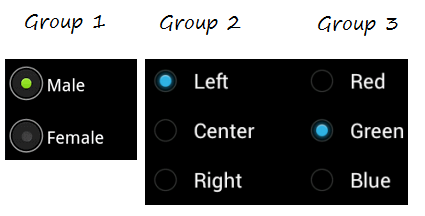
RadioGroup can arrange RadioButton horizontally or vertically.
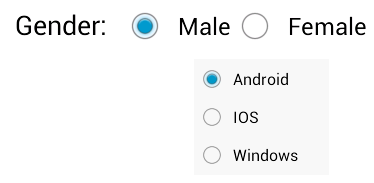
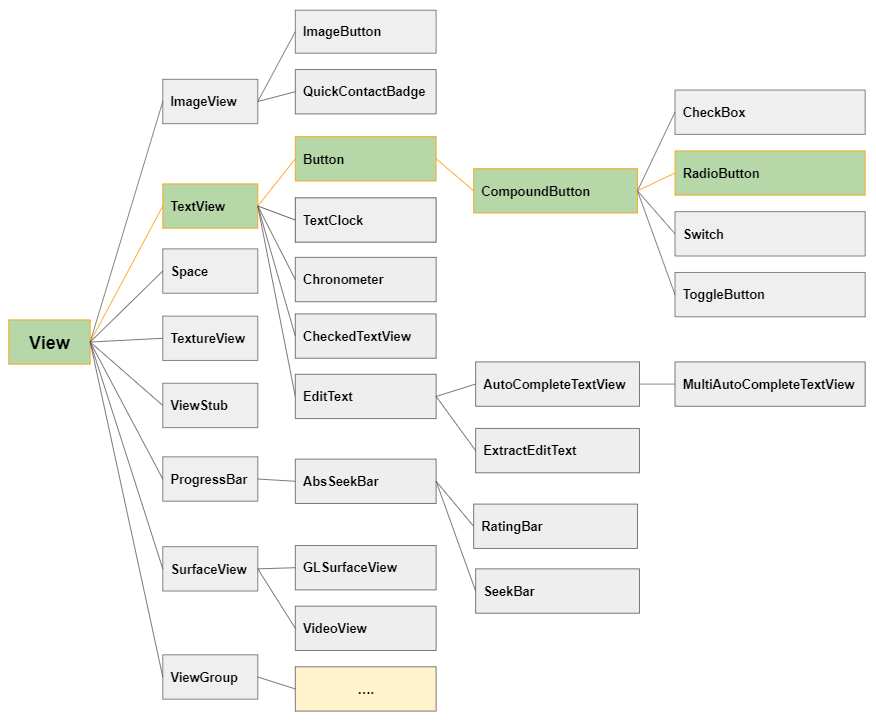
2. RadioGroup & RadioButtons example
Example preview:
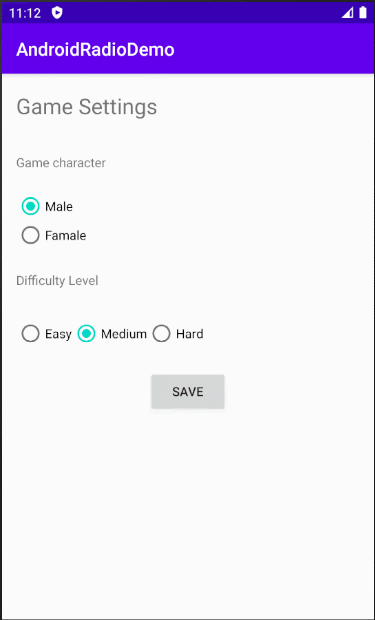
Create a project named AndroidRadioDemo.
- File > New > New Project > Empty Activity
- Name: AndroidRadioDemo
- Package name: org.o7planning.androidradiodemo
- Language: Java
The application interface:
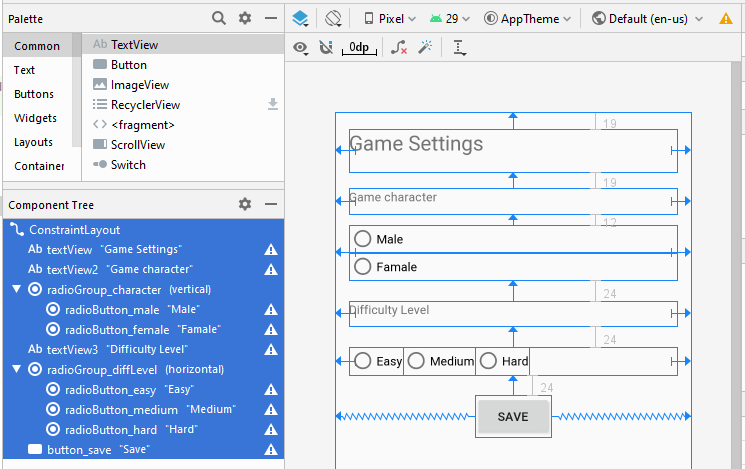
If you are interested in the steps to design the interface of this application, please see the appendix at the end of the article.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView"
android:layout_width="0dp"
android:layout_height="50dp"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="19dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Game Settings"
android:textSize="24sp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<TextView
android:id="@+id/textView2"
android:layout_width="0dp"
android:layout_height="30dp"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="19dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Game character"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView" />
<RadioGroup
android:id="@+id/radioGroup_character"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="12dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView2">
<RadioButton
android:id="@+id/radioButton_male"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Male" />
<RadioButton
android:id="@+id/radioButton_female"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Famale" />
</RadioGroup>
<TextView
android:id="@+id/textView3"
android:layout_width="0dp"
android:layout_height="29dp"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="24dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Difficulty Level"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/radioGroup_character" />
<RadioGroup
android:id="@+id/radioGroup_diffLevel"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="24dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:orientation="horizontal"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView3">
<RadioButton
android:id="@+id/radioButton_easy"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="0"
android:text="Easy" />
<RadioButton
android:id="@+id/radioButton_medium"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="0"
android:text="Medium" />
<RadioButton
android:id="@+id/radioButton_hard"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="0"
android:text="Hard" />
</RadioGroup>
<Button
android:id="@+id/button_save"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="24dp"
android:text="Save"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/radioGroup_diffLevel" />
</androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.java
package org.o7planning.androidradiodemo;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.widget.Button;
import android.widget.CompoundButton;
import android.widget.RadioButton;
import android.widget.RadioGroup;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
private RadioGroup radioGroupCharacter;
private RadioButton radioButtonMale;
private RadioButton radioButtonFemale;
private RadioGroup radioGroupDiffLevel;
private RadioButton radioButtonEasy;
private RadioButton radioButtonMedium;
private RadioButton radioButtonHard;
private Button buttonSave;
private String LOGTAG = "AndroidRadioDemo";
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//
this.radioGroupCharacter= (RadioGroup) this.findViewById(R.id.radioGroup_character);
this.radioButtonMale = (RadioButton) this.findViewById(R.id.radioButton_male);
this.radioButtonFemale = (RadioButton)this.findViewById(R.id.radioButton_female);
this.radioButtonMale.setChecked(true);
//
this.radioGroupDiffLevel= (RadioGroup) this.findViewById(R.id.radioGroup_diffLevel);
this.radioButtonEasy = (RadioButton) this.findViewById(R.id.radioButton_easy);
this.radioButtonMedium = (RadioButton)this.findViewById(R.id.radioButton_medium);
this.radioButtonHard = (RadioButton)this.findViewById(R.id.radioButton_hard);
this.radioButtonMedium.setChecked(true);
this.buttonSave= (Button) this.findViewById(R.id.button_save);
// When radio group "Difficulty Level" checked change.
this.radioGroupDiffLevel.setOnCheckedChangeListener(new RadioGroup.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(RadioGroup group, int checkedId) {
doOnDifficultyLevelChanged(group, checkedId);
}
});
// When radio button "Female" checked change.
this.radioButtonMale.setOnCheckedChangeListener(new RadioButton.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(CompoundButton buttonView, boolean isChecked) {
doOnGameCharacterChanged(buttonView,isChecked);
}
});
// When radio button "Male" checked change.
this.radioButtonFemale.setOnCheckedChangeListener(new RadioButton.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(CompoundButton buttonView, boolean isChecked) {
doOnGameCharacterChanged(buttonView,isChecked);
}
});
// When button "Save" clicked.
this.buttonSave.setOnClickListener(new Button.OnClickListener() {
@Override
public void onClick(View v) {
doSave();
}
});
}
// When radio group "Difficulty Level" checked change.
private void doOnDifficultyLevelChanged(RadioGroup group, int checkedId) {
int checkedRadioId = group.getCheckedRadioButtonId();
if(checkedRadioId== R.id.radioButton_easy) {
Toast.makeText(this,"You choose the level of difficulty: Easy",Toast.LENGTH_SHORT).show();
} else if(checkedRadioId== R.id.radioButton_medium ) {
Toast.makeText(this,"You choose the level of difficulty: Medium",Toast.LENGTH_SHORT).show();
} else if(checkedRadioId== R.id.radioButton_hard) {
Toast.makeText(this,"You choose the level of difficulty: Hard",Toast.LENGTH_SHORT).show();
}
}
// When radio button checked change.
private void doOnGameCharacterChanged(CompoundButton buttonView, boolean isChecked) {
RadioButton radio =(RadioButton) buttonView;
Log.i(LOGTAG, "RadioButton "+ radio.getText()+" : "+ isChecked);
}
// When button "Save" clicked.
private void doSave() {
int difficultyLevel = this.radioGroupDiffLevel.getCheckedRadioButtonId();
int gameCharacter = this.radioGroupCharacter.getCheckedRadioButtonId();
RadioButton radioButtonDiffLevel = (RadioButton) this.findViewById(difficultyLevel);
RadioButton radioButtonGameCharacter = (RadioButton) this.findViewById(gameCharacter);
String message ="Difficulty Level: "+ radioButtonDiffLevel.getText()
+", Game Character: " + radioButtonGameCharacter.getText() ;
Toast.makeText(this,message,Toast.LENGTH_LONG).show();
}
}
Android Programming Tutorials
- Configure Android Emulator in Android Studio
- Android ToggleButton Tutorial with Examples
- Create a simple File Finder Dialog in Android
- Android TimePickerDialog Tutorial with Examples
- Android DatePickerDialog Tutorial with Examples
- What is needed to get started with Android?
- Install Android Studio on Windows
- Install Intel® HAXM for Android Studio
- Android AsyncTask Tutorial with Examples
- Android AsyncTaskLoader Tutorial with Examples
- Android Tutorial for Beginners - Basic examples
- How to know the phone number of Android Emulator and change it
- Android TextInputLayout Tutorial with Examples
- Android CardView Tutorial with Examples
- Android ViewPager2 Tutorial with Examples
- Get Phone Number in Android using TelephonyManager
- Android Phone Call Tutorial with Examples
- Android Wifi Scanning Tutorial with Examples
- Android 2D Game Tutorial for Beginners
- Android DialogFragment Tutorial with Examples
- Android CharacterPickerDialog Tutorial with Examples
- Android Tutorial for Beginners - Hello Android
- Using Android Device File Explorer
- Enable USB Debugging on Android Device
- Android UI Layouts Tutorial with Examples
- Android SMS Tutorial with Examples
- Android SQLite Database Tutorial with Examples
- Google Maps Android API Tutorial with Examples
- Android Text to Speech Tutorial with Examples
- Android Space Tutorial with Examples
- Android Toast Tutorial with Examples
- Create a custom Android Toast
- Android SnackBar Tutorial with Examples
- Android TextView Tutorial with Examples
- Android TextClock Tutorial with Examples
- Android EditText Tutorial with Examples
- Android TextWatcher Tutorial with Examples
- Format Credit Card Number with Android TextWatcher
- Android Clipboard Tutorial with Examples
- Create a simple File Chooser in Android
- Android AutoCompleteTextView and MultiAutoCompleteTextView Tutorial with Examples
- Android ImageView Tutorial with Examples
- Android ImageSwitcher Tutorial with Examples
- Android ScrollView and HorizontalScrollView Tutorial with Examples
- Android WebView Tutorial with Examples
- Android SeekBar Tutorial with Examples
- Android Dialog Tutorial with Examples
- Android AlertDialog Tutorial with Examples
- Android RatingBar Tutorial with Examples
- Android ProgressBar Tutorial with Examples
- Android Spinner Tutorial with Examples
- Android Button Tutorial with Examples
- Android Switch Tutorial with Examples
- Android ImageButton Tutorial with Examples
- Android FloatingActionButton Tutorial with Examples
- Android CheckBox Tutorial with Examples
- Android RadioGroup and RadioButton Tutorial with Examples
- Android Chip and ChipGroup Tutorial with Examples
- Using image assets and icon assets of Android Studio
- Setting SD Card for Android Emulator
- ChipGroup and Chip Entry Example
- How to add external libraries to Android Project in Android Studio?
- How to disable the permissions already granted to the Android application?
- How to remove applications from Android Emulator?
- Android LinearLayout Tutorial with Examples
- Android TableLayout Tutorial with Examples
- Android FrameLayout Tutorial with Examples
- Android QuickContactBadge Tutorial with Examples
- Android StackView Tutorial with Examples
- Android Camera Tutorial with Examples
- Android MediaPlayer Tutorial with Examples
- Android VideoView Tutorial with Examples
- Playing Sound effects in Android with SoundPool
- Android Networking Tutorial with Examples
- Android JSON Parser Tutorial with Examples
- Android SharedPreferences Tutorial with Examples
- Android Internal Storage Tutorial with Examples
- Android External Storage Tutorial with Examples
- Android Intents Tutorial with Examples
- Example of an explicit Android Intent, calling another Intent
- Example of implicit Android Intent, open a URL, send an email
- Android Services Tutorial with Examples
- Android Notifications Tutorial with Examples
- Android DatePicker Tutorial with Examples
- Android TimePicker Tutorial with Examples
- Android Chronometer Tutorial with Examples
- Android OptionMenu Tutorial with Examples
- Android ContextMenu Tutorial with Examples
- Android PopupMenu Tutorial with Examples
- Android Fragments Tutorial with Examples
- Android ListView Tutorial with Examples
- Android ListView with Checkbox using ArrayAdapter
- Android GridView Tutorial with Examples
Show More