Android ScrollView and HorizontalScrollView Tutorial with Examples
1. ScrollView and HorizontalScrollView
In Android, ScrollView is a Layout type, which is a rectangular container with a vertical scroll bar. It is able to contain another component larger-sized than itself. Similar to ScrollView, HorizontalScrollView is a container with a horizontal scroll bar.
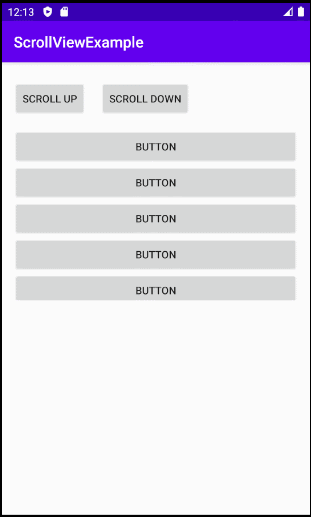
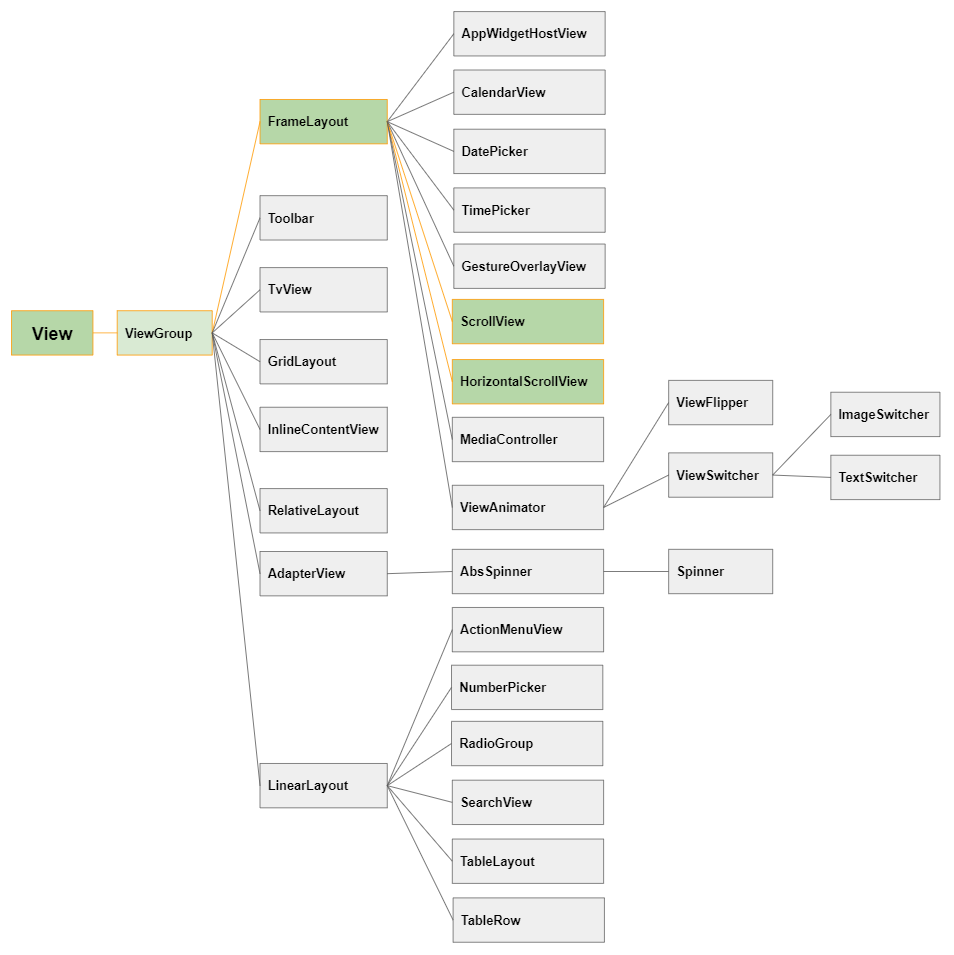
Generally speaking, ScrollView and HorizontalScrollView are useful. Both of them are used to contain a large-sized content, and the user needs to use scroll bars to be able to view the content completely.
ScrollView and HorizontalScrollView can only contain one direct child component, so its child component is normally another container that can contain one or more child components.
On Android Studio, if you drag and drop a ScrollView (Or HorizontalScrollView) into the interface, a child component called LinearLayout will automatically be added. You can definitely delete it to insert another child component.
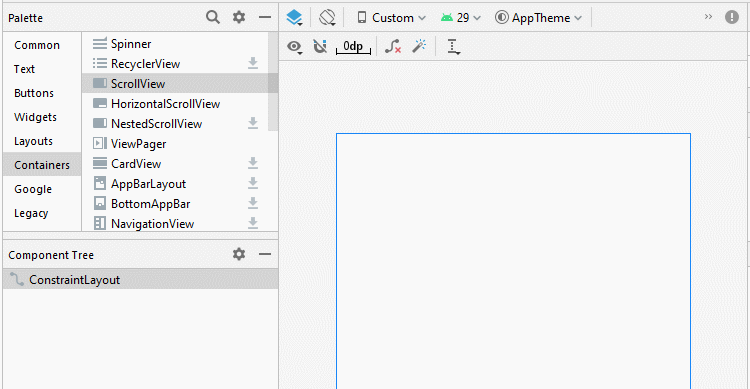
You had better not combine the ScrollView with ListView or GridView components, because both of them already have their own vertical scroll bars.
2. An example of the ScrollView
In this example, a vertical LinearLayout has multiple child components. As clearly seen, it needs a space with sufficient height. However, the screen size is limited, so it is essential to be placed in a ScrollView.
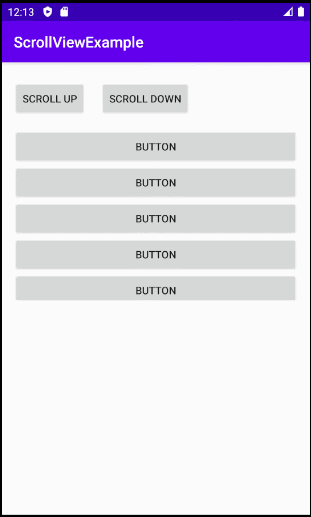
Some steps to design the interface of the app are shown in this example:
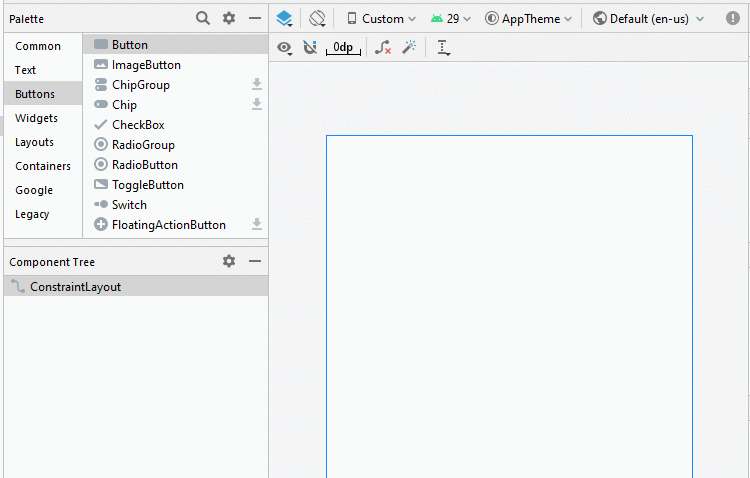
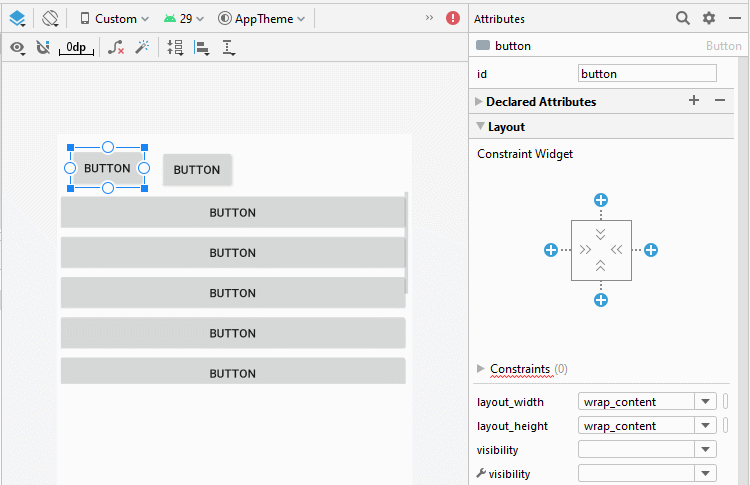
Set ID, Text for the components in the interface:
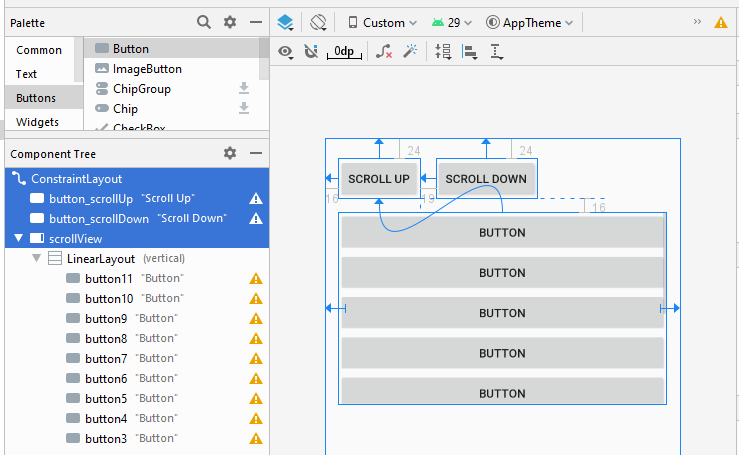
main_activity.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<Button
android:id="@+id/button_scrollUp"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="24dp"
android:text="Scroll Up"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/button_scrollDown"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="19dp"
android:layout_marginLeft="19dp"
android:layout_marginTop="24dp"
android:text="Scroll Down"
app:layout_constraintStart_toEndOf="@+id/button_scrollUp"
app:layout_constraintTop_toTopOf="parent" />
<ScrollView
android:id="@+id/scrollView"
android:layout_width="0dp"
android:layout_height="229dp"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/button_scrollUp">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<Button
android:id="@+id/button11"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Button" />
<Button
android:id="@+id/button10"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Button" />
<Button
android:id="@+id/button9"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Button" />
<Button
android:id="@+id/button8"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Button" />
<Button
android:id="@+id/button7"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Button" />
<Button
android:id="@+id/button6"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Button" />
<Button
android:id="@+id/button5"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Button" />
<Button
android:id="@+id/button4"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Button" />
<Button
android:id="@+id/button3"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Button" />
</LinearLayout>
</ScrollView>
</androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.java
package com.example.scrollviewexample;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.ScrollView;
public class MainActivity extends AppCompatActivity {
private ScrollView scrollView;
private Button buttonScrollUp;
private Button buttonScrollDown;
public static final int SCROLL_DELTA = 15; // Pixel.
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.scrollView = (ScrollView) this.findViewById(R.id.scrollView);
this.buttonScrollUp = (Button) this.findViewById(R.id.button_scrollUp);
this.buttonScrollDown = (Button) this.findViewById(R.id.button_scrollDown);
this.buttonScrollUp.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
doScrollUp();
}
});
this.buttonScrollDown.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
doScrollDown();
}
});
}
private void doScrollUp() {
int x = this.scrollView.getScrollX();
int y = this.scrollView.getScrollY();
if(y - SCROLL_DELTA >= 0) {
this.scrollView.scrollTo(x, y-SCROLL_DELTA);
}
}
private void doScrollDown() {
int maxAmount = scrollView.getMaxScrollAmount();
int x = this.scrollView.getScrollX();
int y = this.scrollView.getScrollY();
if(y + SCROLL_DELTA <= maxAmount) {
this.scrollView.scrollTo(x, y + SCROLL_DELTA);
}
}
}
3. An example of the HorizontalScrollView
In this example, a horizontal LinearLayout has a lot of child components. It definitely needs a space with sufficient width. As you can see, the user's device screen size is limited, so it is necessary to be placed in a HorizontalScrollView.
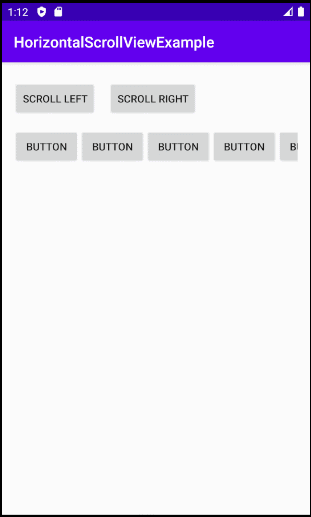
Now let's design your interface:
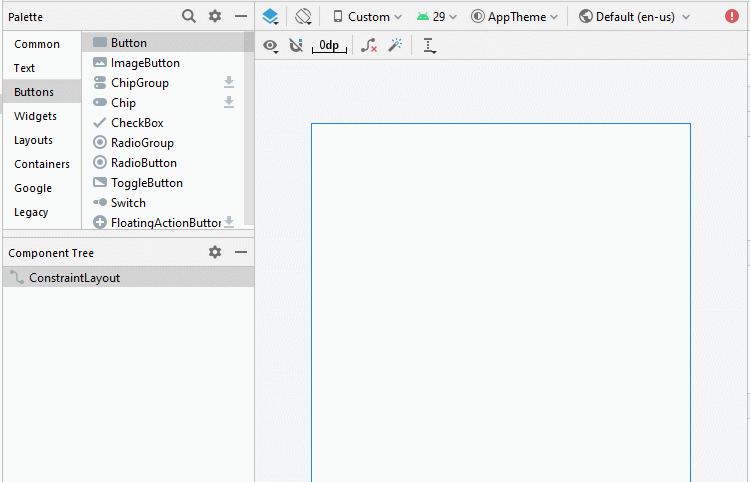
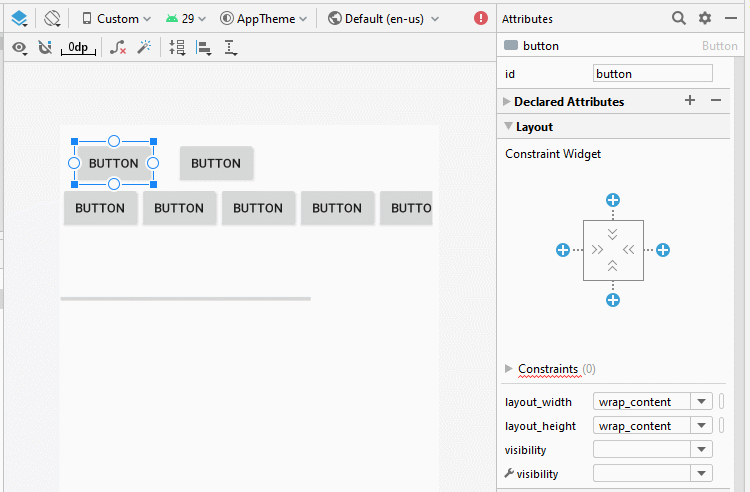
And set ID, Text for the components in the interface:
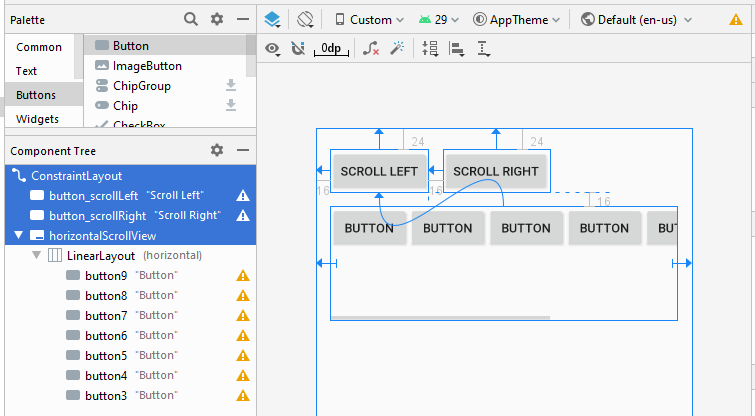
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<Button
android:id="@+id/button_scrollLeft"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="24dp"
android:text="Scroll Left"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/button_scrollRight"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="24dp"
android:text="Scroll Right"
app:layout_constraintStart_toEndOf="@+id/button_scrollLeft"
app:layout_constraintTop_toTopOf="parent" />
<HorizontalScrollView
android:id="@+id/horizontalScrollView"
android:layout_width="0dp"
android:layout_height="128dp"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/button_scrollLeft">
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:orientation="horizontal">
<Button
android:id="@+id/button9"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="Button" />
<Button
android:id="@+id/button8"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="Button" />
<Button
android:id="@+id/button7"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="Button" />
<Button
android:id="@+id/button6"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="Button" />
<Button
android:id="@+id/button5"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="Button" />
<Button
android:id="@+id/button4"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="Button" />
<Button
android:id="@+id/button3"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="Button" />
</LinearLayout>
</HorizontalScrollView>
</androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.java
package com.example.horizontalscrollviewexample;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.HorizontalScrollView;
public class MainActivity extends AppCompatActivity {
private HorizontalScrollView horizontalScrollView;
private Button buttonScrollLeft;
private Button buttonScrollRight;
public static final int SCROLL_DELTA = 15; // Pixel.
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.horizontalScrollView = (HorizontalScrollView) this.findViewById(R.id.horizontalScrollView);
this.buttonScrollLeft = (Button) this.findViewById(R.id.button_scrollLeft);
this.buttonScrollRight = (Button) this.findViewById(R.id.button_scrollRight);
this.buttonScrollLeft.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
doScrollLeft();
}
});
this.buttonScrollRight.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
doScrollRight();
}
});
}
private void doScrollLeft() {
int x = this.horizontalScrollView.getScrollX();
int y = this.horizontalScrollView.getScrollY();
if(x - SCROLL_DELTA >= 0) {
this.horizontalScrollView.scrollTo(x - SCROLL_DELTA, y);
}
}
private void doScrollRight() {
int maxAmount = horizontalScrollView.getMaxScrollAmount();
int x = this.horizontalScrollView.getScrollX();
int y = this.horizontalScrollView.getScrollY();
if(x + SCROLL_DELTA <= maxAmount) {
this.horizontalScrollView.scrollTo(x + SCROLL_DELTA, y);
}
}
}
Android Programming Tutorials
- Configure Android Emulator in Android Studio
- Android ToggleButton Tutorial with Examples
- Create a simple File Finder Dialog in Android
- Android TimePickerDialog Tutorial with Examples
- Android DatePickerDialog Tutorial with Examples
- What is needed to get started with Android?
- Install Android Studio on Windows
- Install Intel® HAXM for Android Studio
- Android AsyncTask Tutorial with Examples
- Android AsyncTaskLoader Tutorial with Examples
- Android Tutorial for Beginners - Basic examples
- How to know the phone number of Android Emulator and change it
- Android TextInputLayout Tutorial with Examples
- Android CardView Tutorial with Examples
- Android ViewPager2 Tutorial with Examples
- Get Phone Number in Android using TelephonyManager
- Android Phone Call Tutorial with Examples
- Android Wifi Scanning Tutorial with Examples
- Android 2D Game Tutorial for Beginners
- Android DialogFragment Tutorial with Examples
- Android CharacterPickerDialog Tutorial with Examples
- Android Tutorial for Beginners - Hello Android
- Using Android Device File Explorer
- Enable USB Debugging on Android Device
- Android UI Layouts Tutorial with Examples
- Android SMS Tutorial with Examples
- Android SQLite Database Tutorial with Examples
- Google Maps Android API Tutorial with Examples
- Android Text to Speech Tutorial with Examples
- Android Space Tutorial with Examples
- Android Toast Tutorial with Examples
- Create a custom Android Toast
- Android SnackBar Tutorial with Examples
- Android TextView Tutorial with Examples
- Android TextClock Tutorial with Examples
- Android EditText Tutorial with Examples
- Android TextWatcher Tutorial with Examples
- Format Credit Card Number with Android TextWatcher
- Android Clipboard Tutorial with Examples
- Create a simple File Chooser in Android
- Android AutoCompleteTextView and MultiAutoCompleteTextView Tutorial with Examples
- Android ImageView Tutorial with Examples
- Android ImageSwitcher Tutorial with Examples
- Android ScrollView and HorizontalScrollView Tutorial with Examples
- Android WebView Tutorial with Examples
- Android SeekBar Tutorial with Examples
- Android Dialog Tutorial with Examples
- Android AlertDialog Tutorial with Examples
- Android RatingBar Tutorial with Examples
- Android ProgressBar Tutorial with Examples
- Android Spinner Tutorial with Examples
- Android Button Tutorial with Examples
- Android Switch Tutorial with Examples
- Android ImageButton Tutorial with Examples
- Android FloatingActionButton Tutorial with Examples
- Android CheckBox Tutorial with Examples
- Android RadioGroup and RadioButton Tutorial with Examples
- Android Chip and ChipGroup Tutorial with Examples
- Using image assets and icon assets of Android Studio
- Setting SD Card for Android Emulator
- ChipGroup and Chip Entry Example
- How to add external libraries to Android Project in Android Studio?
- How to disable the permissions already granted to the Android application?
- How to remove applications from Android Emulator?
- Android LinearLayout Tutorial with Examples
- Android TableLayout Tutorial with Examples
- Android FrameLayout Tutorial with Examples
- Android QuickContactBadge Tutorial with Examples
- Android StackView Tutorial with Examples
- Android Camera Tutorial with Examples
- Android MediaPlayer Tutorial with Examples
- Android VideoView Tutorial with Examples
- Playing Sound effects in Android with SoundPool
- Android Networking Tutorial with Examples
- Android JSON Parser Tutorial with Examples
- Android SharedPreferences Tutorial with Examples
- Android Internal Storage Tutorial with Examples
- Android External Storage Tutorial with Examples
- Android Intents Tutorial with Examples
- Example of an explicit Android Intent, calling another Intent
- Example of implicit Android Intent, open a URL, send an email
- Android Services Tutorial with Examples
- Android Notifications Tutorial with Examples
- Android DatePicker Tutorial with Examples
- Android TimePicker Tutorial with Examples
- Android Chronometer Tutorial with Examples
- Android OptionMenu Tutorial with Examples
- Android ContextMenu Tutorial with Examples
- Android PopupMenu Tutorial with Examples
- Android Fragments Tutorial with Examples
- Android ListView Tutorial with Examples
- Android ListView with Checkbox using ArrayAdapter
- Android GridView Tutorial with Examples
Show More