Android RatingBar Tutorial with Examples
1. Android RatingBar
In Android, RatingBar is a user interface control used to receive reviews from users. RatingBar is a descendant of ProgressBar,so it has features inherited from the ProgressBar. It is also used to display an aggregate rating (average) from all users. In terms of the RatingBar interface, it consists of stars, and users will select a star to give a rating, the first star corresponds to the lowest rating, and the final star corresponds to the highest rating.
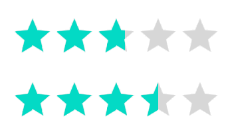
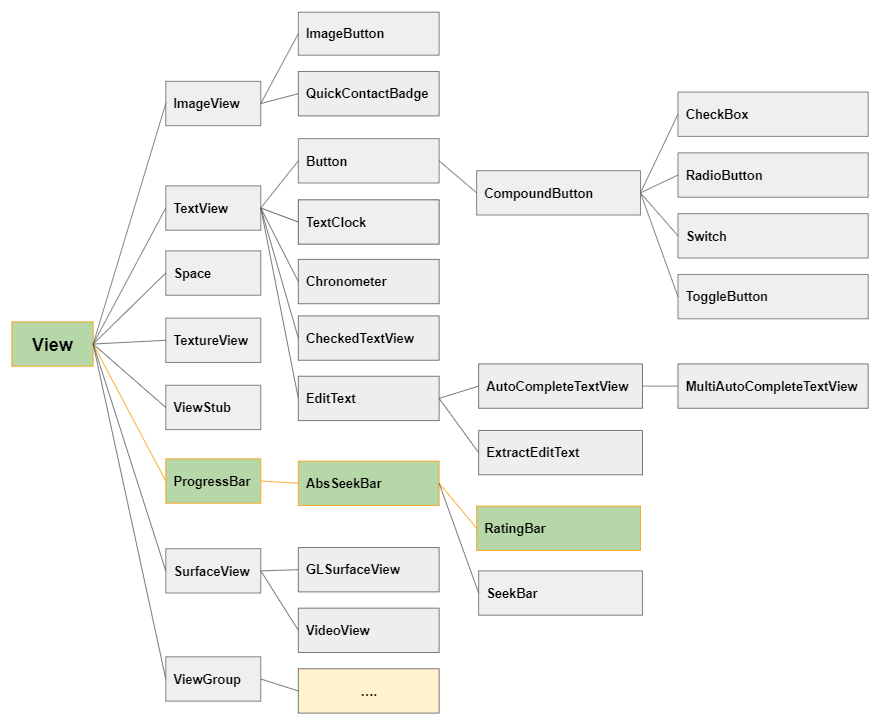
Below is the structure of a RatingBar:
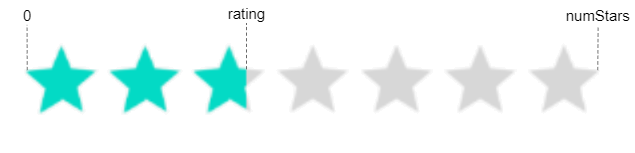
android:numStars
The android:numStars attribute allows you to set the number of stars to be displayed on the RatingBar. Its default value is 5.
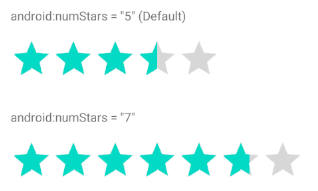
android:numStars
<!-- numStars default is 5 -->
<RatingBar
android:id="@+id/ratingBar21"
android:rating="3.5"
android:stepSize="0.1" ... />
<!-- numStars = 7 -->
<RatingBar
android:id="@+id/ratingBar22"
android:numStars="7"
android:rating="5.7"
android:stepSize="0.1" ... />
android:rating, android:stepSize
android:rating: A value indicating the rating of the user, or the average value from all ratings of all users. It is in the range of (0,numStars].
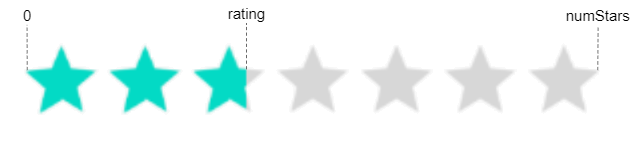
The default value of the android:stepSize attribute is 0.5, which means that the RatingBar will only display the ratings (or the user reviews) with the values of 0, 0.5, 1, 1.5, 2, etc. When the value of the android:stepSite attribute is changed to 0.1, RatingBar can display the ratings with the values of 0, 0.1, 0.2, 0.3, 0.4, 0.5, etc.
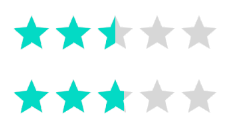
android:stepSize
<!-- android.stepSize = "0.5" (Default) -->
<RatingBar
android:id="@+id/ratingBar31"
android:rating="2.7" ... />
<!-- android.stepSize = "0.1" -->
<RatingBar
android:id="@+id/ratingBar32"
android:rating="2.7"
android:stepSize="0.1" ... />
android:isIndicator
The android:isIndicator = "true" attribute is used when you do not want users to leave a rating with this RatingBar. For example, you expect to have a RatingBar only to show an aggregate rating (average) from all users. By default the value of the attribute is false.
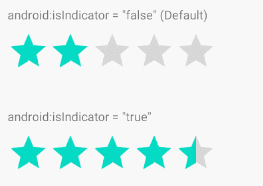
<!-- android:isIndicator = "false" (Default) -->
<RatingBar
android:id="@+id/ratingBar51"
android:rating="2" ... />
<!-- android:isIndicator = "true" (Readonly RatingBar) -->
<RatingBar
android:id="@+id/ratingBar52"
android:isIndicator="true"
android:rating="4.5" ... />
2. RatingBar Styles
The style attribute is an option of the RatingBar, which allows you to set the style for RatingBar. There are several styles available in Android library that may be ready to use.
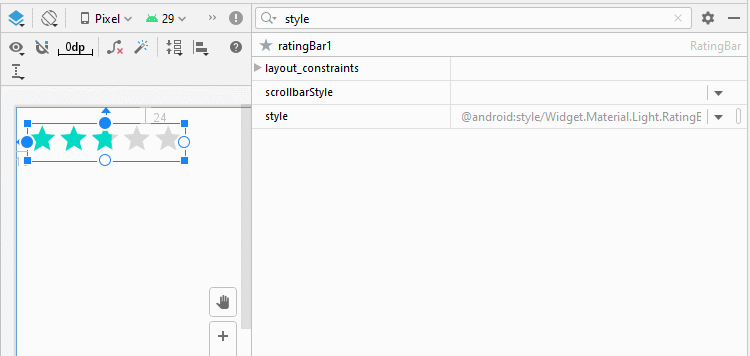
The Android library offers quite a few styles for RatingBar; however, a remarkable number of them look so much alike. Thus, it is hard for you to tell the difference. Here is an example with some nice designs:
- style="@style/Widget.AppCompat.RatingBar.Indicator"
- style="@android:style/Widget.DeviceDefault.Light.RatingBar.Small"
- style="@android:style/Widget.RatingBar"
- style="@android:style/Widget.Holo.RatingBar"
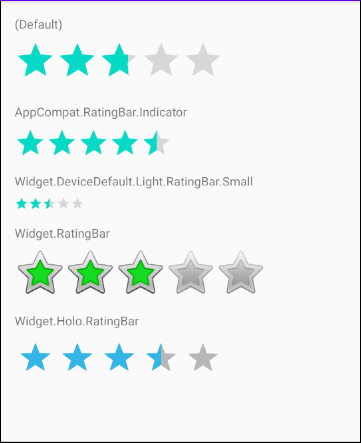
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity" >
<TextView
android:id="@+id/textView41"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="(Default)"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<RatingBar
android:id="@+id/ratingBar41"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
android:rating="2.7"
android:stepSize="0.1"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView41" />
<TextView
android:id="@+id/textView42"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="AppCompat.RatingBar.Indicator"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/ratingBar41" />
<RatingBar
android:id="@+id/ratingBar42"
style="@style/Widget.AppCompat.RatingBar.Indicator"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
android:rating="4.5"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView42" />
<TextView
android:id="@+id/textView43"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Widget.DeviceDefault.Light.RatingBar.Small"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/ratingBar42" />
<RatingBar
android:id="@+id/ratingBar43"
style="@android:style/Widget.DeviceDefault.Light.RatingBar.Small"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
android:rating="2.5"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView43" />
<TextView
android:id="@+id/textView44"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Widget.RatingBar"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/ratingBar43" />
<RatingBar
android:id="@+id/ratingBar44"
style="@android:style/Widget.RatingBar"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
android:rating="3"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView44" />
<TextView
android:id="@+id/textView45"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Widget.Holo.RatingBar"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/ratingBar44" />
<RatingBar
android:id="@+id/ratingBa45"
style="@android:style/Widget.Holo.RatingBar"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
android:rating="3.5"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView45" />
</androidx.constraintlayout.widget.ConstraintLayout>
3. RatingBar Events
There are quite a few events related to a RatingBar, but the following events are most frequently used:
- setOnClickListener(View.OnClickListener);
- setOnRatingBarChangeListener(RatingBar.OnRatingBarChangeListener);
On Click Event:
The event happens when the user clicks the RatingBar. It is the same as the action of the user clicking a Button.
ratingBar.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
RatingBar rb = (RatingBar) v;
float rating = rb.getRating();
// Your code ...
}
});
On RatingBar Changed Event
The event happens when the rating changes due to the user's action or the effect of calling the ratingBar.setRating(newRating) method, etc.
ratingBar.setOnRatingBarChangeListener(new RatingBar.OnRatingBarChangeListener() {
@Override
public void onRatingChanged(RatingBar ratingBar, float newRating, boolean fromUser) {
// Your code
}
});
4. Example of RatingBar
OK, now we are going to set a simple example using RatingBar. In this example, the first RatingBar receives user reviews. The second RatingBar displays an aggregate rating (average) from all users.
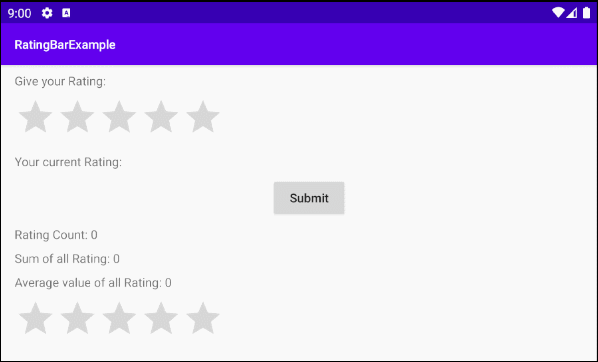
On Android Studio, create a project:
- File > New > New Project > Empty Activity
- Name: RatingBarExample
- Package name: org.o7planning.ratingbarexample
- Language: Java
Here is the application interface:
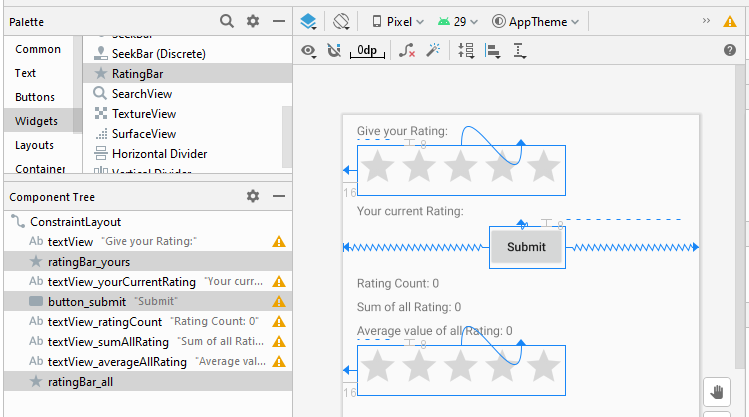
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity" >
<TextView
android:id="@+id/textView"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Give your Rating:"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<RatingBar
android:id="@+id/ratingBar_yours"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView" />
<TextView
android:id="@+id/textView_yourCurrentRating"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Your current Rating:"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.0"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/ratingBar_yours" />
<Button
android:id="@+id/button_submit"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="8dp"
android:text="Submit"
android:textAllCaps="false"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.52"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView_yourCurrentRating" />
<TextView
android:id="@+id/textView_ratingCount"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Rating Count: 0"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/button_submit" />
<TextView
android:id="@+id/textView_sumAllRating"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Sum of all Rating: 0"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView_ratingCount" />
<TextView
android:id="@+id/textView_averageAllRating"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Average value of all Rating: 0"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView_sumAllRating" />
<RatingBar
android:id="@+id/ratingBar_all"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
android:isIndicator="true"
android:stepSize="0.1"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView_averageAllRating" />
</androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.java
package com.example.ratingbarexample;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.RatingBar;
import android.widget.TextView;
import java.util.ArrayList;
import java.util.List;
public class MainActivity extends AppCompatActivity {
private RatingBar ratingBarYours;
private RatingBar ratingBarAll;
private Button buttonSubmit;
private TextView textViewYourCurrentRating;
private TextView textViewRatingCount;
private TextView textViewSumAllRating;
private TextView textViewAverageAllRating;
private List<Float> allRatings = new ArrayList<Float>();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//
this.buttonSubmit = (Button) this.findViewById(R.id.button_submit);
this.ratingBarYours = (RatingBar) this.findViewById(R.id.ratingBar_yours);
this.ratingBarAll = (RatingBar) this.findViewById(R.id.ratingBar_all);
this.textViewYourCurrentRating = (TextView) this.findViewById(R.id.textView_yourCurrentRating);
this.textViewRatingCount= (TextView) this.findViewById(R.id.textView_ratingCount);
this.textViewSumAllRating= (TextView) this.findViewById(R.id.textView_sumAllRating);
this.textViewAverageAllRating= (TextView) this.findViewById(R.id.textView_averageAllRating);
this.ratingBarYours.setOnRatingBarChangeListener(new RatingBar.OnRatingBarChangeListener() {
@Override
public void onRatingChanged(RatingBar ratingBar, float rating, boolean fromUser) {
textViewYourCurrentRating.setText("Your current Rating: " + rating);
}
});
this.buttonSubmit.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
doSubmit();
}
});
}
private void doSubmit() {
float rating = this.ratingBarYours.getRating();
this.allRatings.add(rating);
int ratingCount = this.allRatings.size();
float ratingSum = 0f;
for(Float r: this.allRatings) {
ratingSum += r;
}
float averageRating = ratingSum / ratingCount;
this.textViewRatingCount.setText("Rating Count: " + ratingCount);
this.textViewSumAllRating.setText("Sum off all Rating: " + ratingSum);
this.textViewAverageAllRating.setText("Average value off all Rating: " + averageRating);
float ratingAll = this.ratingBarAll.getNumStars() * averageRating / this.ratingBarYours.getNumStars() ;
this.ratingBarAll.setRating(ratingAll);
}
}
Android Programming Tutorials
- Configure Android Emulator in Android Studio
- Android ToggleButton Tutorial with Examples
- Create a simple File Finder Dialog in Android
- Android TimePickerDialog Tutorial with Examples
- Android DatePickerDialog Tutorial with Examples
- What is needed to get started with Android?
- Install Android Studio on Windows
- Install Intel® HAXM for Android Studio
- Android AsyncTask Tutorial with Examples
- Android AsyncTaskLoader Tutorial with Examples
- Android Tutorial for Beginners - Basic examples
- How to know the phone number of Android Emulator and change it
- Android TextInputLayout Tutorial with Examples
- Android CardView Tutorial with Examples
- Android ViewPager2 Tutorial with Examples
- Get Phone Number in Android using TelephonyManager
- Android Phone Call Tutorial with Examples
- Android Wifi Scanning Tutorial with Examples
- Android 2D Game Tutorial for Beginners
- Android DialogFragment Tutorial with Examples
- Android CharacterPickerDialog Tutorial with Examples
- Android Tutorial for Beginners - Hello Android
- Using Android Device File Explorer
- Enable USB Debugging on Android Device
- Android UI Layouts Tutorial with Examples
- Android SMS Tutorial with Examples
- Android SQLite Database Tutorial with Examples
- Google Maps Android API Tutorial with Examples
- Android Text to Speech Tutorial with Examples
- Android Space Tutorial with Examples
- Android Toast Tutorial with Examples
- Create a custom Android Toast
- Android SnackBar Tutorial with Examples
- Android TextView Tutorial with Examples
- Android TextClock Tutorial with Examples
- Android EditText Tutorial with Examples
- Android TextWatcher Tutorial with Examples
- Format Credit Card Number with Android TextWatcher
- Android Clipboard Tutorial with Examples
- Create a simple File Chooser in Android
- Android AutoCompleteTextView and MultiAutoCompleteTextView Tutorial with Examples
- Android ImageView Tutorial with Examples
- Android ImageSwitcher Tutorial with Examples
- Android ScrollView and HorizontalScrollView Tutorial with Examples
- Android WebView Tutorial with Examples
- Android SeekBar Tutorial with Examples
- Android Dialog Tutorial with Examples
- Android AlertDialog Tutorial with Examples
- Android RatingBar Tutorial with Examples
- Android ProgressBar Tutorial with Examples
- Android Spinner Tutorial with Examples
- Android Button Tutorial with Examples
- Android Switch Tutorial with Examples
- Android ImageButton Tutorial with Examples
- Android FloatingActionButton Tutorial with Examples
- Android CheckBox Tutorial with Examples
- Android RadioGroup and RadioButton Tutorial with Examples
- Android Chip and ChipGroup Tutorial with Examples
- Using image assets and icon assets of Android Studio
- Setting SD Card for Android Emulator
- ChipGroup and Chip Entry Example
- How to add external libraries to Android Project in Android Studio?
- How to disable the permissions already granted to the Android application?
- How to remove applications from Android Emulator?
- Android LinearLayout Tutorial with Examples
- Android TableLayout Tutorial with Examples
- Android FrameLayout Tutorial with Examples
- Android QuickContactBadge Tutorial with Examples
- Android StackView Tutorial with Examples
- Android Camera Tutorial with Examples
- Android MediaPlayer Tutorial with Examples
- Android VideoView Tutorial with Examples
- Playing Sound effects in Android with SoundPool
- Android Networking Tutorial with Examples
- Android JSON Parser Tutorial with Examples
- Android SharedPreferences Tutorial with Examples
- Android Internal Storage Tutorial with Examples
- Android External Storage Tutorial with Examples
- Android Intents Tutorial with Examples
- Example of an explicit Android Intent, calling another Intent
- Example of implicit Android Intent, open a URL, send an email
- Android Services Tutorial with Examples
- Android Notifications Tutorial with Examples
- Android DatePicker Tutorial with Examples
- Android TimePicker Tutorial with Examples
- Android Chronometer Tutorial with Examples
- Android OptionMenu Tutorial with Examples
- Android ContextMenu Tutorial with Examples
- Android PopupMenu Tutorial with Examples
- Android Fragments Tutorial with Examples
- Android ListView Tutorial with Examples
- Android ListView with Checkbox using ArrayAdapter
- Android GridView Tutorial with Examples
Show More