Android QuickContactBadge Tutorial with Examples
1. Android QuickContactBadge
Android QuickContactBadge is a subclass of ImageView. It displays as a small badge which the user clicks on to quickly create a contact, such as adding a phone number to the contacts, adding an email to the contacts, etc.
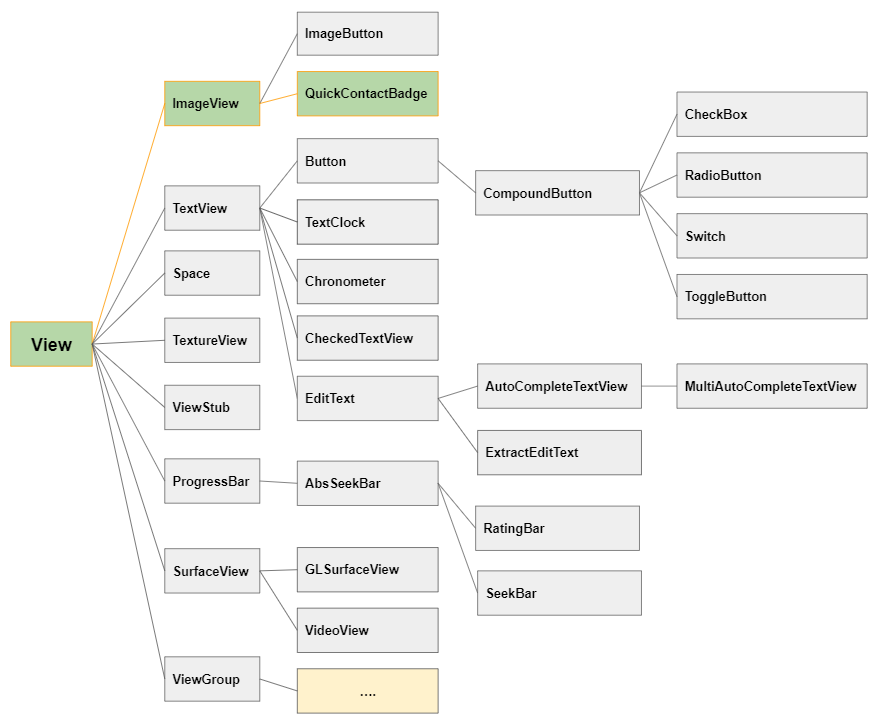
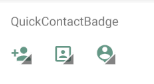
Note: QuickContactBadge is not available in the Palette of the design window, so you need to use the following XML code to add QuickContactBadge into the interface.
QuickContactBadge
<QuickContactBadge
android:id="@+id/quickContactBadge11"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@drawable/icon_contact_add" ...>
</QuickContactBadge>
True to its name of "Quick Contact Badge", with just a couple of short lines of code, you can easily create a small "Badge" that allows the user to click on in order to add a contact.
For example: Set the parameters for a QuickContactBadge to add a specific phone number to the contacts. You don't even need to write the OnClick event handler for the QuickContactBadge.
this.quickContactBadgePhone = (QuickContactBadge) findViewById(R.id.quickContactBadge_phone);
this.quickContactBadgePhone.assignContactFromPhone("+1-555-521-5554", true);
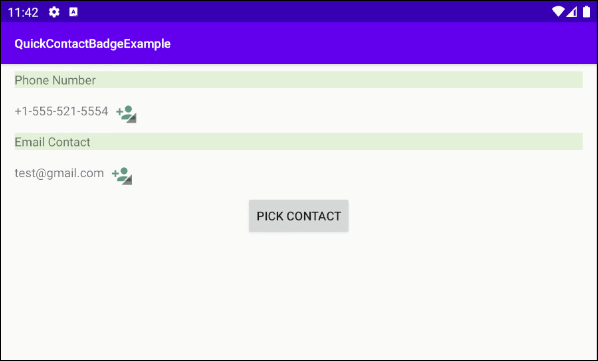
You can see the newly added phone number to the contacts:
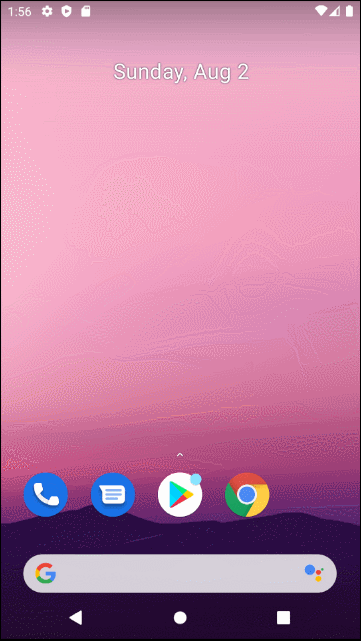
2. Some important methods
Some important methods of QuickContactBadge are:
- assignContactFromPhone(String phoneNumber, boolean lazyLookup, Bundle extras)
- assignContactFromPhone(String phoneNumber, boolean lazyLookup)
- assignContactFromEmail(String emailAddress, boolean lazyLookup, Bundle extras)
- assignContactFromEmail(String emailAddress, boolean lazyLookup)
- assignContactUri(Uri contactUri)
3. Example of QuickContactBadge
In this example, we have two QuickContactBadge(s). The first QuickContactBadge allows the user to quickly add a phone number to the contacts, and the second QuickContactBadge allows the user to quickly add an Email to the contacts.
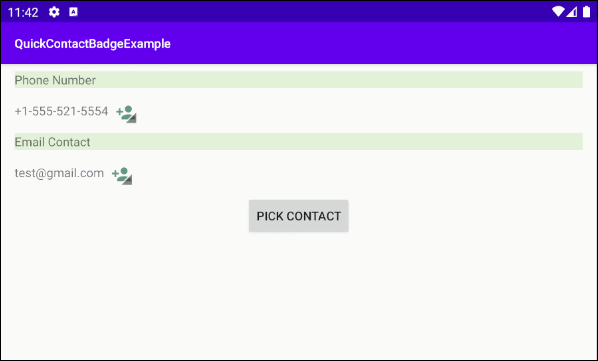
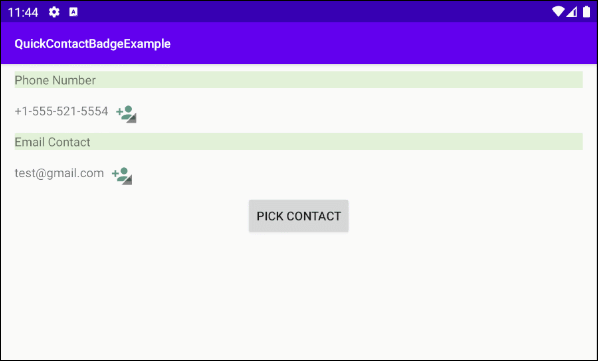
The "Pick Contact" button permits the user to select an existing contact in the contacts.
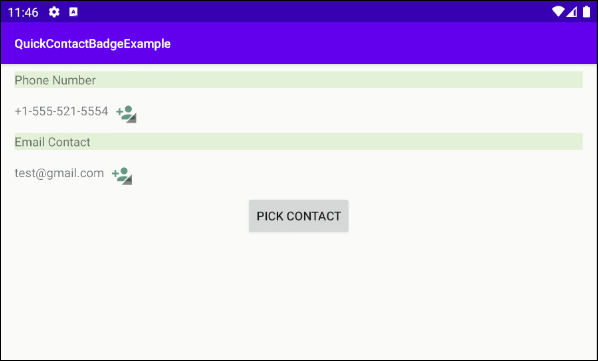
OK. Now on Android Studio,create a project:
- File > New > New Project > Empty Activity
- Name: QuickContactBadgeExample
- Package name: com.example.quickcontactbadgeexample
- Language: Java
The interface of the example looks like this:
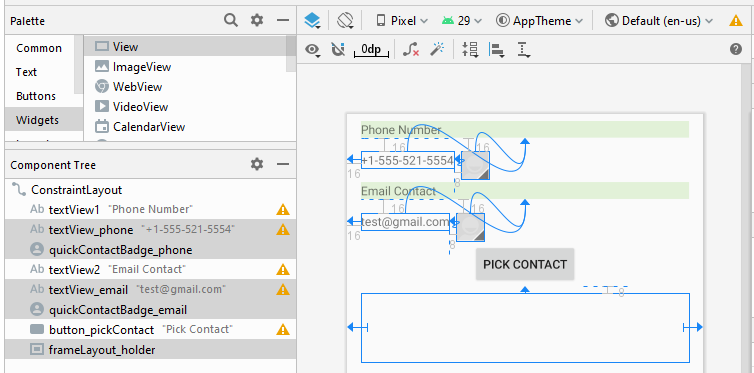
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView1"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="16dp"
android:background="#E2F1D8"
android:text="Phone Number"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.0"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<TextView
android:id="@+id/textView_phone"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginTop="16dp"
android:inputType="textEmailAddress"
android:text="+1-555-521-5554"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView1" />
<QuickContactBadge
android:id="@+id/quickContactBadge_phone"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginTop="16dp"
android:scaleType="centerCrop"
android:src="@drawable/icon_contact_add"
app:layout_constraintStart_toEndOf="@+id/textView_phone"
app:layout_constraintTop_toBottomOf="@+id/textView1"></QuickContactBadge>
<TextView
android:id="@+id/textView2"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:background="#E2F1D8"
android:text="Email Contact"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.0"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView_phone" />
<TextView
android:id="@+id/textView_email"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginTop="16dp"
android:inputType="textEmailAddress"
android:text="test@gmail.com"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView2" />
<QuickContactBadge
android:id="@+id/quickContactBadge_email"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginTop="16dp"
android:scaleType="centerCrop"
android:src="@drawable/icon_contact_add"
app:layout_constraintStart_toEndOf="@+id/textView_email"
app:layout_constraintTop_toBottomOf="@+id/textView2"></QuickContactBadge>
<Button
android:id="@+id/button_pickContact"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="16dp"
android:text="Pick Contact"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView_email"></Button>
<FrameLayout
android:id="@+id/frameLayout_holder"
android:layout_width="0dp"
android:layout_height="80dp"
android:layout_marginStart="16dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="16dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.0"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/button_pickContact"></FrameLayout>
</androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.java
package com.example.quickcontactbadgeexample;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Intent;
import android.net.Uri;
import android.os.Bundle;
import android.provider.ContactsContract;
import android.view.View;
import android.widget.Button;
import android.widget.FrameLayout;
import android.widget.QuickContactBadge;
import android.widget.TextView;
public class MainActivity extends AppCompatActivity {
public static final int CONTACT_PICKER_RESULT = 100;
private TextView textViewEmail;
private TextView textViewPhone;
private Button buttonPickContact;
private FrameLayout frameLayoutHolder;
private QuickContactBadge quickContactBadgeEmail;
private QuickContactBadge quickContactBadgePhone;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.textViewEmail = (TextView) this.findViewById(R.id.textView_email);
this.textViewPhone = (TextView) this.findViewById(R.id.textView_phone);
this.buttonPickContact = (Button) this.findViewById(R.id.button_pickContact);
this.frameLayoutHolder = (FrameLayout) this.findViewById(R.id.frameLayout_holder);
this.quickContactBadgeEmail = (QuickContactBadge) findViewById(R.id.quickContactBadge_email);
this.quickContactBadgePhone = (QuickContactBadge) findViewById(R.id.quickContactBadge_phone);
int textViewHeight = this.textViewEmail.getLayoutParams().height;
quickContactBadgeEmail.setMaxHeight(textViewHeight);
String email = this.textViewEmail.getText().toString(); // test@gmail.com
String phoneNumber = this.textViewPhone.getText().toString(); // +1-555-521-5554
// Set Parameters for quickContactBadgeEmail:
this.quickContactBadgeEmail.assignContactFromEmail(email, true);
this.quickContactBadgeEmail.setMode(ContactsContract.QuickContact.MODE_SMALL);
// Set Parameters for quickContactBadgePhone:
this.quickContactBadgePhone.assignContactFromPhone(phoneNumber, true);
this.buttonPickContact.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
pickContactHandler( );
}
});
}
public void pickContactHandler( ) {
Intent contactPickerIntent = new Intent(Intent.ACTION_PICK,
ContactsContract.Contacts.CONTENT_URI);
startActivityForResult(contactPickerIntent, CONTACT_PICKER_RESULT);
}
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (resultCode == RESULT_OK) {
switch (requestCode) {
case CONTACT_PICKER_RESULT:
Uri contactUri = data.getData();
QuickContactBadge badgeLarge = new QuickContactBadge(this);
badgeLarge.assignContactUri(contactUri);
badgeLarge.setMode(ContactsContract.QuickContact.MODE_LARGE);
badgeLarge.setImageResource(R.drawable.icon_contact1);
this.frameLayoutHolder.addView(badgeLarge);
break;
}
}
}
}
Android Programming Tutorials
- Configure Android Emulator in Android Studio
- Android ToggleButton Tutorial with Examples
- Create a simple File Finder Dialog in Android
- Android TimePickerDialog Tutorial with Examples
- Android DatePickerDialog Tutorial with Examples
- What is needed to get started with Android?
- Install Android Studio on Windows
- Install Intel® HAXM for Android Studio
- Android AsyncTask Tutorial with Examples
- Android AsyncTaskLoader Tutorial with Examples
- Android Tutorial for Beginners - Basic examples
- How to know the phone number of Android Emulator and change it
- Android TextInputLayout Tutorial with Examples
- Android CardView Tutorial with Examples
- Android ViewPager2 Tutorial with Examples
- Get Phone Number in Android using TelephonyManager
- Android Phone Call Tutorial with Examples
- Android Wifi Scanning Tutorial with Examples
- Android 2D Game Tutorial for Beginners
- Android DialogFragment Tutorial with Examples
- Android CharacterPickerDialog Tutorial with Examples
- Android Tutorial for Beginners - Hello Android
- Using Android Device File Explorer
- Enable USB Debugging on Android Device
- Android UI Layouts Tutorial with Examples
- Android SMS Tutorial with Examples
- Android SQLite Database Tutorial with Examples
- Google Maps Android API Tutorial with Examples
- Android Text to Speech Tutorial with Examples
- Android Space Tutorial with Examples
- Android Toast Tutorial with Examples
- Create a custom Android Toast
- Android SnackBar Tutorial with Examples
- Android TextView Tutorial with Examples
- Android TextClock Tutorial with Examples
- Android EditText Tutorial with Examples
- Android TextWatcher Tutorial with Examples
- Format Credit Card Number with Android TextWatcher
- Android Clipboard Tutorial with Examples
- Create a simple File Chooser in Android
- Android AutoCompleteTextView and MultiAutoCompleteTextView Tutorial with Examples
- Android ImageView Tutorial with Examples
- Android ImageSwitcher Tutorial with Examples
- Android ScrollView and HorizontalScrollView Tutorial with Examples
- Android WebView Tutorial with Examples
- Android SeekBar Tutorial with Examples
- Android Dialog Tutorial with Examples
- Android AlertDialog Tutorial with Examples
- Android RatingBar Tutorial with Examples
- Android ProgressBar Tutorial with Examples
- Android Spinner Tutorial with Examples
- Android Button Tutorial with Examples
- Android Switch Tutorial with Examples
- Android ImageButton Tutorial with Examples
- Android FloatingActionButton Tutorial with Examples
- Android CheckBox Tutorial with Examples
- Android RadioGroup and RadioButton Tutorial with Examples
- Android Chip and ChipGroup Tutorial with Examples
- Using image assets and icon assets of Android Studio
- Setting SD Card for Android Emulator
- ChipGroup and Chip Entry Example
- How to add external libraries to Android Project in Android Studio?
- How to disable the permissions already granted to the Android application?
- How to remove applications from Android Emulator?
- Android LinearLayout Tutorial with Examples
- Android TableLayout Tutorial with Examples
- Android FrameLayout Tutorial with Examples
- Android QuickContactBadge Tutorial with Examples
- Android StackView Tutorial with Examples
- Android Camera Tutorial with Examples
- Android MediaPlayer Tutorial with Examples
- Android VideoView Tutorial with Examples
- Playing Sound effects in Android with SoundPool
- Android Networking Tutorial with Examples
- Android JSON Parser Tutorial with Examples
- Android SharedPreferences Tutorial with Examples
- Android Internal Storage Tutorial with Examples
- Android External Storage Tutorial with Examples
- Android Intents Tutorial with Examples
- Example of an explicit Android Intent, calling another Intent
- Example of implicit Android Intent, open a URL, send an email
- Android Services Tutorial with Examples
- Android Notifications Tutorial with Examples
- Android DatePicker Tutorial with Examples
- Android TimePicker Tutorial with Examples
- Android Chronometer Tutorial with Examples
- Android OptionMenu Tutorial with Examples
- Android ContextMenu Tutorial with Examples
- Android PopupMenu Tutorial with Examples
- Android Fragments Tutorial with Examples
- Android ListView Tutorial with Examples
- Android ListView with Checkbox using ArrayAdapter
- Android GridView Tutorial with Examples
Show More