Android Chip and ChipGroup Tutorial with Examples
1. Chip
Chip are new component first introduced in Android SDK version 28. Which is basically an interface component consisting of icon and text, placed in a rectangular background with rounded corners.
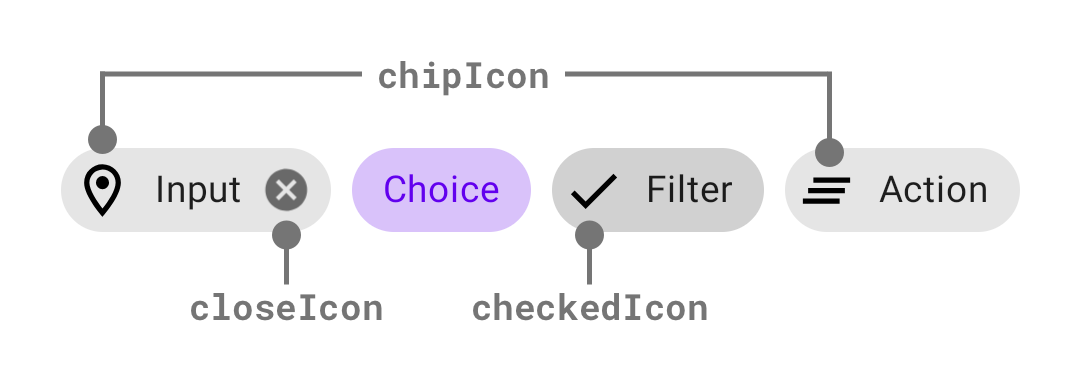
In order to use the Chip object in the project you need to install it.
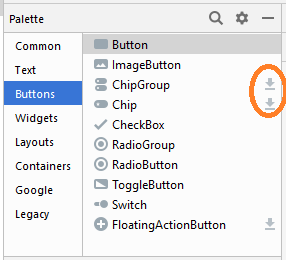
After it has been successfully installed, a library including Chip will be declared in the build.gradle (Module app) file.
implementation 'com.google.android.material:material:1.1.0'
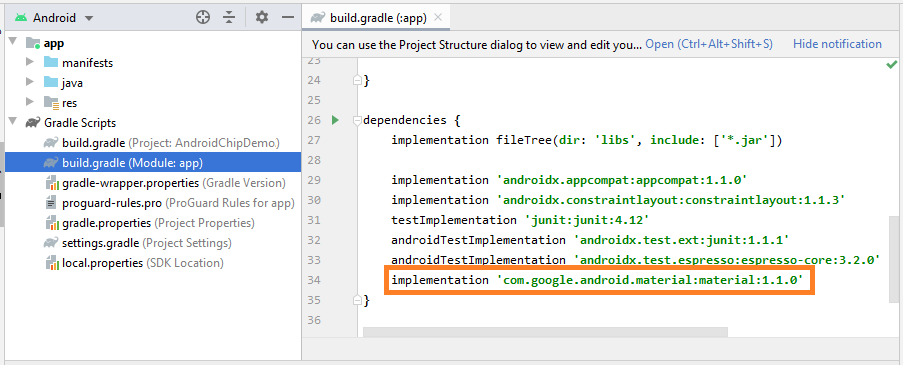
Here is an XML code of a simple Chip:
Chip Sample
<!-- IMPORTANT: android:theme is required -->
<com.google.android.material.chip.Chip
android:id="@+id/chip1"
style="@style/Widget.MaterialComponents.Chip.Action"
android:theme="@style/Theme.MaterialComponents.Light"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Chip Action" />
theme attribute
The android:theme attribute is required for a Chip. Without this attribute, your entire application will not work, and it also will not notify you of that. I was surprised because of that when I first used a Chip.
- android:theme="@style/Theme.MaterialComponents"
- android:theme="@style/Theme.MaterialComponents.Light"
- android:theme="@style/Theme.MaterialComponents.***"
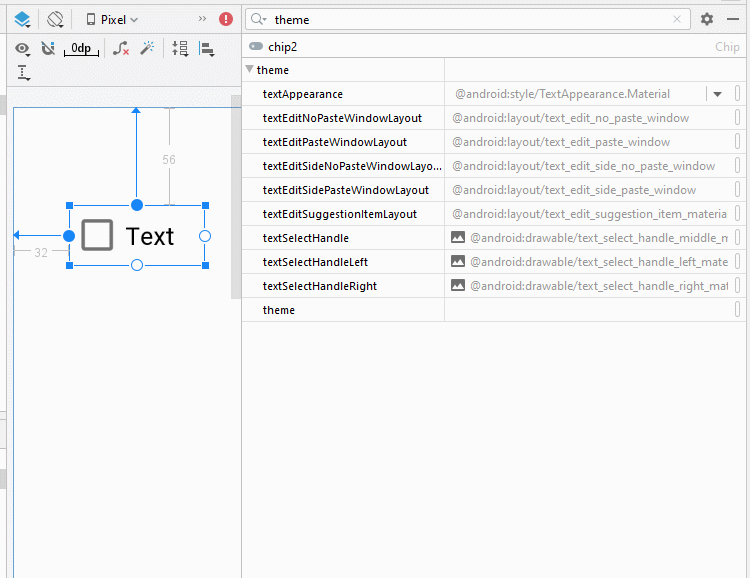
style attribute:
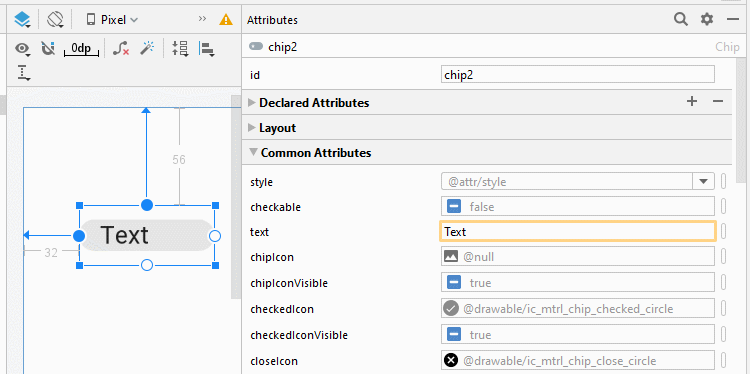
The style attribute has 4 values which helps you to specify the type of Chips:
- @style/Widget.MaterialComponents.Chip.Action (Default)
- @style/Widget.MaterialComponents.Chip.Entry
- @style/Widget.MaterialComponents.Chip.Choice
- @style/Widget.MaterialComponents.Chip.Filter
Style Default
The style attribute is optional and its default value is known as "@style/Widget.MaterialComponents.Chip.Action".
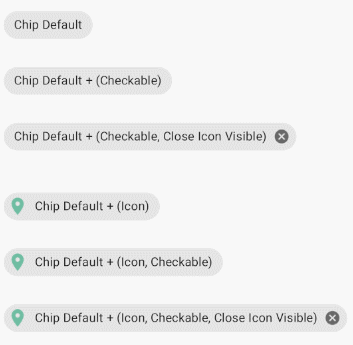
Chip Default Sample
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<com.google.android.material.chip.Chip
android:id="@+id/chip1"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="50dp"
android:text="Chip Default"
android:theme="@style/Theme.MaterialComponents.Light"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<com.google.android.material.chip.Chip
android:id="@+id/chip2"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="16dp"
android:checkable="true"
android:text="Chip Default + (Checkable)"
android:theme="@style/Theme.MaterialComponents.Light"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip1" />
<com.google.android.material.chip.Chip
android:id="@+id/chip3"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="16dp"
android:checkable="true"
android:text="Chip Default + (Checkable, Close Icon Visible)"
android:theme="@style/Theme.MaterialComponents.Light"
app:closeIconVisible="true"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip2" />
<com.google.android.material.chip.Chip
android:id="@+id/chip4"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="32dp"
android:checkable="true"
android:text="Chip Default + (Icon)"
android:theme="@style/Theme.MaterialComponents.Light"
app:chipIcon="@drawable/my_icon"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip3" />
<com.google.android.material.chip.Chip
android:id="@+id/chip5"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="16dp"
android:checkable="true"
android:text="Chip Default + (Icon, Checkable)"
android:theme="@style/Theme.MaterialComponents.Light"
app:chipIcon="@drawable/my_icon"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip4" />
<com.google.android.material.chip.Chip
android:id="@+id/chip6"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="16dp"
android:checkable="true"
android:text="Chip Default + (Icon, Checkable, Close Icon Visible)"
android:theme="@style/Theme.MaterialComponents.Light"
app:chipIcon="@drawable/my_icon"
app:closeIconVisible="true"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip5" />
</androidx.constraintlayout.widget.ConstraintLayout>
style="@style/Widget.MaterialComponents.Chip.Action"
This is the default value of style.
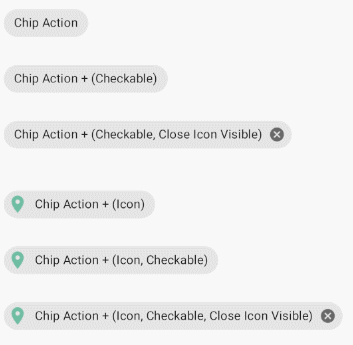
style="@style/Widget.MaterialComponents.Chip.Entry"
Chip Entry = Chip Default + Close Icon + Checkable
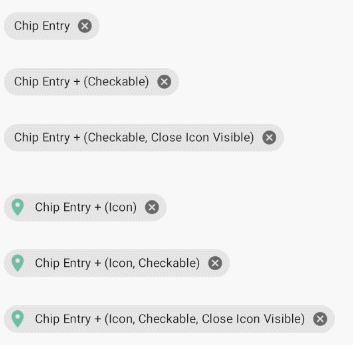
Chip Entry Sample
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<com.google.android.material.chip.Chip
android:id="@+id/chip1"
style="@style/Widget.MaterialComponents.Chip.Entry"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="50dp"
android:text="Chip Entry"
android:theme="@style/Theme.MaterialComponents.Light"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<com.google.android.material.chip.Chip
android:id="@+id/chip2"
style="@style/Widget.MaterialComponents.Chip.Entry"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="16dp"
android:checkable="true"
android:text="Chip Entry + (Checkable)"
android:theme="@style/Theme.MaterialComponents.Light"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip1" />
<com.google.android.material.chip.Chip
android:id="@+id/chip3"
style="@style/Widget.MaterialComponents.Chip.Entry"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="16dp"
android:checkable="true"
android:text="Chip Entry + (Checkable, Close Icon Visible)"
android:theme="@style/Theme.MaterialComponents.Light"
app:closeIconVisible="true"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip2" />
<com.google.android.material.chip.Chip
android:id="@+id/chip4"
style="@style/Widget.MaterialComponents.Chip.Entry"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="32dp"
android:checkable="true"
android:text="Chip Entry + (Icon)"
android:theme="@style/Theme.MaterialComponents.Light"
app:chipIcon="@drawable/my_icon"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip3" />
<com.google.android.material.chip.Chip
android:id="@+id/chip5"
style="@style/Widget.MaterialComponents.Chip.Entry"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="16dp"
android:checkable="true"
android:text="Chip Entry + (Icon, Checkable)"
android:theme="@style/Theme.MaterialComponents.Light"
app:chipIcon="@drawable/my_icon"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip4" />
<com.google.android.material.chip.Chip
android:id="@+id/chip6"
style="@style/Widget.MaterialComponents.Chip.Entry"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="16dp"
android:checkable="true"
android:text="Chip Entry + (Icon, Checkable, Close Icon Visible)"
android:theme="@style/Theme.MaterialComponents.Light"
app:chipIcon="@drawable/my_icon"
app:closeIconVisible="true"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip5" />
</androidx.constraintlayout.widget.ConstraintLayout>
style="@style/Widget.MaterialComponents.Chip.Choice"
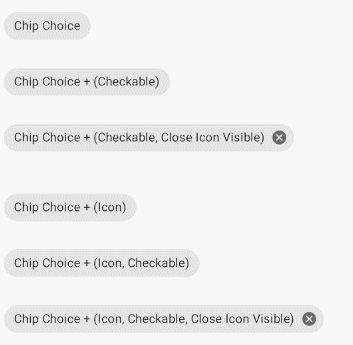
Here are some characteristics of Chip Choice:
- When Chip Choice is selected, its background color will look darker.
- There is no check icon on the left, even if you set checkable=true.
- Icon - Not displayed even if you set the value for it.
Chip Choice Sample
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<com.google.android.material.chip.Chip
android:id="@+id/chip1"
style="@style/Widget.MaterialComponents.Chip.Choice"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="50dp"
android:text="Chip Choice"
android:theme="@style/Theme.MaterialComponents.Light"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<com.google.android.material.chip.Chip
android:id="@+id/chip2"
style="@style/Widget.MaterialComponents.Chip.Choice"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="16dp"
android:checkable="true"
android:text="Chip Choice + (Checkable)"
android:theme="@style/Theme.MaterialComponents.Light"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip1" />
<com.google.android.material.chip.Chip
android:id="@+id/chip3"
style="@style/Widget.MaterialComponents.Chip.Choice"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="16dp"
android:checkable="true"
android:text="Chip Choice + (Checkable, Close Icon Visible)"
android:theme="@style/Theme.MaterialComponents.Light"
app:closeIconVisible="true"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip2" />
<com.google.android.material.chip.Chip
android:id="@+id/chip4"
style="@style/Widget.MaterialComponents.Chip.Choice"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="32dp"
android:checkable="true"
android:text="Chip Choice + (Icon)"
android:theme="@style/Theme.MaterialComponents.Light"
app:chipIcon="@drawable/my_icon"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip3" />
<com.google.android.material.chip.Chip
android:id="@+id/chip5"
style="@style/Widget.MaterialComponents.Chip.Choice"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="16dp"
android:checkable="true"
android:text="Chip Choice + (Icon, Checkable)"
android:theme="@style/Theme.MaterialComponents.Light"
app:chipIcon="@drawable/my_icon"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip4" />
<com.google.android.material.chip.Chip
android:id="@+id/chip6"
style="@style/Widget.MaterialComponents.Chip.Choice"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="16dp"
android:checkable="true"
android:text="Chip Choice + (Icon, Checkable, Close Icon Visible)"
android:theme="@style/Theme.MaterialComponents.Light"
app:chipIcon="@drawable/my_icon"
app:closeIconVisible="true"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip5" />
</androidx.constraintlayout.widget.ConstraintLayout>
style="@style/Widget.MaterialComponents.Chip.Filter"
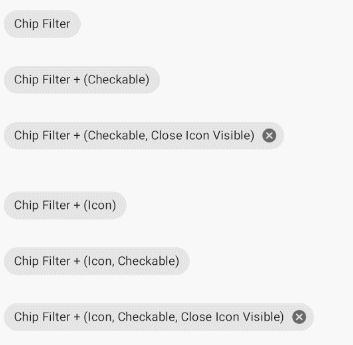
Characteristics of Chip Filter are:
- Chip Filter = Chip Default + Checkable
- Icon - Not displayed even if you set the value for it.
Chip Filter Sample
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<com.google.android.material.chip.Chip
android:id="@+id/chip1"
style="@style/Widget.MaterialComponents.Chip.Filter"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="50dp"
android:text="Chip Filter"
android:theme="@style/Theme.MaterialComponents.Light"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<com.google.android.material.chip.Chip
android:id="@+id/chip2"
style="@style/Widget.MaterialComponents.Chip.Filter"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="16dp"
android:checkable="true"
android:text="Chip Filter + (Checkable)"
android:theme="@style/Theme.MaterialComponents.Light"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip1" />
<com.google.android.material.chip.Chip
android:id="@+id/chip3"
style="@style/Widget.MaterialComponents.Chip.Filter"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="16dp"
android:checkable="true"
android:text="Chip Filter + (Checkable, Close Icon Visible)"
android:theme="@style/Theme.MaterialComponents.Light"
app:closeIconVisible="true"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip2" />
<com.google.android.material.chip.Chip
android:id="@+id/chip4"
style="@style/Widget.MaterialComponents.Chip.Filter"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="32dp"
android:checkable="true"
android:text="Chip Filter + (Icon)"
android:theme="@style/Theme.MaterialComponents.Light"
app:chipIcon="@drawable/my_icon"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip3" />
<com.google.android.material.chip.Chip
android:id="@+id/chip5"
style="@style/Widget.MaterialComponents.Chip.Filter"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="16dp"
android:checkable="true"
android:text="Chip Filter + (Icon, Checkable)"
android:theme="@style/Theme.MaterialComponents.Light"
app:chipIcon="@drawable/my_icon"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip4" />
<com.google.android.material.chip.Chip
android:id="@+id/chip6"
style="@style/Widget.MaterialComponents.Chip.Filter"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="16dp"
android:checkable="true"
android:text="Chip Filter + (Icon, Checkable, Close Icon Visible)"
android:theme="@style/Theme.MaterialComponents.Light"
app:chipIcon="@drawable/my_icon"
app:closeIconVisible="true"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip5" />
</androidx.constraintlayout.widget.ConstraintLayout>
2. Chip Theme
The android:theme attribute is required for a Chip. Your application may not work if Chips do not have this attribute, or if you set an invalid value for them.
Some possible values are:
- android:theme="@style/Theme.MaterialComponents.Light"
- android:theme="@style/Theme.MaterialComponents.Bridge"
- android:theme="@style/Theme.MaterialComponents.DayNight"
- .....
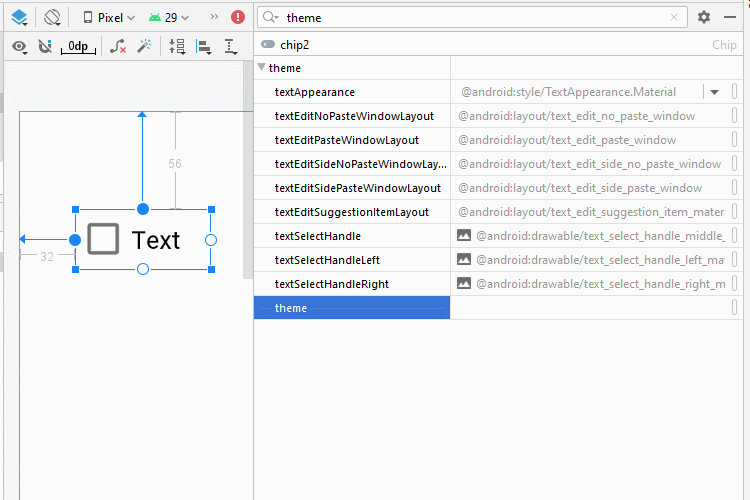
android:theme="@style/Theme.MaterialComponents.Light"
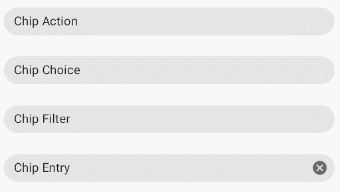
android:theme="@style/Theme.MaterialComponents.Bridge"
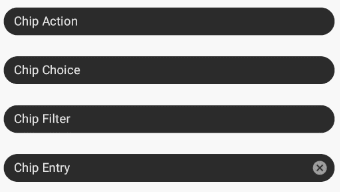
3. Chip Listener
There are many events related to Chips, but basically there are 3 common events as follows:
- chip.setOnClickListener(View.OnClickListener)
- chip.setOnCloseIconClickListener(View.OnClickListener)
- chip.setOnCheckedChangeListener(CompoundButton.OnCheckedChangeListener)
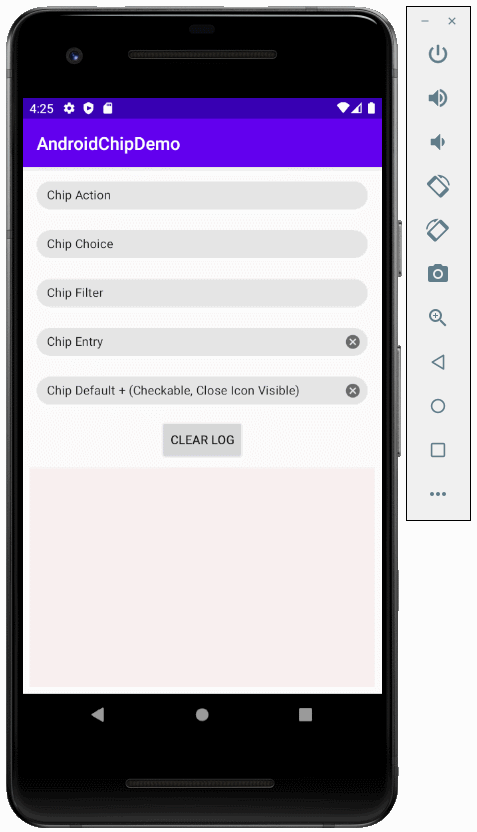
main_activity.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<com.google.android.material.chip.Chip
android:id="@+id/chip_action"
style="@style/Widget.MaterialComponents.Chip.Action"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:clickable="true"
android:text="Chip Action"
android:theme="@style/Theme.MaterialComponents.Light"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<com.google.android.material.chip.Chip
android:id="@+id/chip_choice"
style="@style/Widget.MaterialComponents.Chip.Choice"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Chip Choice"
android:theme="@style/Theme.MaterialComponents.Light"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip_action" />
<com.google.android.material.chip.Chip
android:id="@+id/chip_filter"
style="@style/Widget.MaterialComponents.Chip.Filter"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Chip Filter"
android:theme="@style/Theme.MaterialComponents.Light"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip_choice" />
<com.google.android.material.chip.Chip
android:id="@+id/chip_entry"
style="@style/Widget.MaterialComponents.Chip.Entry"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Chip Entry"
android:theme="@style/Theme.MaterialComponents.Light"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip_filter" />
<com.google.android.material.chip.Chip
android:id="@+id/chip_custom"
android:layout_width="0dp"
android:layout_height="48dp"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:checkable="true"
android:text="Chip Default + (Checkable, Close Icon Visible)"
android:theme="@style/Theme.MaterialComponents.Light"
app:closeIconVisible="true"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip_entry" />
<Button
android:id="@+id/button_clear"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="8dp"
android:text="Clear Log"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip_custom" />
<EditText
android:id="@+id/editText_log"
android:layout_width="0dp"
android:layout_height="0dp"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="8dp"
android:layout_marginRight="8dp"
android:layout_marginBottom="8dp"
android:background="#F8EFEF"
android:ems="10"
android:gravity="top|left"
android:inputType="textMultiLine"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/button_clear" />
</androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.java
package org.o7planning.androidchipdemo;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.widget.Button;
import android.widget.CompoundButton;
import android.widget.EditText;
import com.google.android.material.chip.Chip;
public class MainActivity extends AppCompatActivity {
private Chip chipAction;
private Chip chipChoice;
private Chip chipEntry;
private Chip chipFilter;
private Chip chipCustom;
private EditText editTextLog;
private Button buttonClear;
private View.OnClickListener clickListener;
private View.OnClickListener closeIconClickListener;
private CompoundButton.OnCheckedChangeListener checkedChangeListener;
private static final String LOG_TAG = "AndroidChipDemo";
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.chipAction = (Chip) this.findViewById(R.id.chip_action);
this.chipChoice = (Chip) this.findViewById(R.id.chip_choice);
this.chipEntry = (Chip) this.findViewById(R.id.chip_entry);
this.chipFilter = (Chip) this.findViewById(R.id.chip_filter);
this.chipCustom = (Chip) this.findViewById(R.id.chip_custom);
this.editTextLog = (EditText) this.findViewById(R.id.editText_log);
this.buttonClear = (Button) this.findViewById(R.id.button_clear);
//
this.clickListener= new ClickListenerImpl();
this.closeIconClickListener = new CloseIconClickListenerImpl();
this.checkedChangeListener = new CheckedChangeListenerImpl();
//
this.chipAction.setOnClickListener( clickListener);
this.chipAction.setOnCloseIconClickListener(closeIconClickListener);
this.chipAction.setOnCheckedChangeListener(checkedChangeListener);
//
this.chipChoice.setOnClickListener( clickListener);
this.chipChoice.setOnCloseIconClickListener(closeIconClickListener);
this.chipChoice.setOnCheckedChangeListener(checkedChangeListener);
//
this.chipEntry.setOnClickListener( clickListener);
this.chipEntry.setOnCloseIconClickListener(closeIconClickListener);
this.chipEntry.setOnCheckedChangeListener(checkedChangeListener);
//
this.chipFilter.setOnClickListener( clickListener);
this.chipFilter.setOnCloseIconClickListener(closeIconClickListener);
this.chipFilter.setOnCheckedChangeListener(checkedChangeListener);
//
this.chipCustom.setOnClickListener( clickListener);
this.chipCustom.setOnCloseIconClickListener(closeIconClickListener);
this.chipCustom.setOnCheckedChangeListener(checkedChangeListener);
//
this.buttonClear.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
editTextLog.setText("");
}
});
}
private void appendLog(String text) {
this.editTextLog.append(text) ;
this.editTextLog.append("\n");
Log.i(LOG_TAG, text);
}
class ClickListenerImpl implements View.OnClickListener {
@Override
public void onClick(View v) {
appendLog("Clicked");
}
}
class CloseIconClickListenerImpl implements View.OnClickListener {
@Override
public void onClick(View v) {
appendLog("Close Icon Clicked");
}
}
class CheckedChangeListenerImpl implements CompoundButton.OnCheckedChangeListener {
@Override
public void onCheckedChanged(CompoundButton buttonView, boolean isChecked) {
appendLog("Checked Changed! isChecked? " + isChecked);
}
}
}
4. ChipGroup
ChipGroup is used to contain multiple Chips. By default, Chips will be sorted on many of lines if one line cannot contain all the Chips.
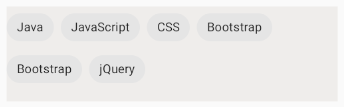
However, you can set it to ensure that the Chips will be displayed on a single line.
<com.google.android.material.chip.ChipGroup
app:singleLine="true"
...>
</com.google.android.material.chip.ChipGroup>
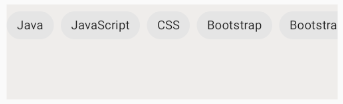
ChipGroup can also work as a RadioGroup, which means that if one of the Chips is set to be checked, the rest of the Chips will be unchecked.
<com.google.android.material.chip.ChipGroup
app:singleSelection="true"
...>
</com.google.android.material.chip.ChipGroup>
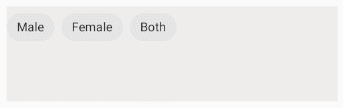
Android Programming Tutorials
- Configure Android Emulator in Android Studio
- Android ToggleButton Tutorial with Examples
- Create a simple File Finder Dialog in Android
- Android TimePickerDialog Tutorial with Examples
- Android DatePickerDialog Tutorial with Examples
- What is needed to get started with Android?
- Install Android Studio on Windows
- Install Intel® HAXM for Android Studio
- Android AsyncTask Tutorial with Examples
- Android AsyncTaskLoader Tutorial with Examples
- Android Tutorial for Beginners - Basic examples
- How to know the phone number of Android Emulator and change it
- Android TextInputLayout Tutorial with Examples
- Android CardView Tutorial with Examples
- Android ViewPager2 Tutorial with Examples
- Get Phone Number in Android using TelephonyManager
- Android Phone Call Tutorial with Examples
- Android Wifi Scanning Tutorial with Examples
- Android 2D Game Tutorial for Beginners
- Android DialogFragment Tutorial with Examples
- Android CharacterPickerDialog Tutorial with Examples
- Android Tutorial for Beginners - Hello Android
- Using Android Device File Explorer
- Enable USB Debugging on Android Device
- Android UI Layouts Tutorial with Examples
- Android SMS Tutorial with Examples
- Android SQLite Database Tutorial with Examples
- Google Maps Android API Tutorial with Examples
- Android Text to Speech Tutorial with Examples
- Android Space Tutorial with Examples
- Android Toast Tutorial with Examples
- Create a custom Android Toast
- Android SnackBar Tutorial with Examples
- Android TextView Tutorial with Examples
- Android TextClock Tutorial with Examples
- Android EditText Tutorial with Examples
- Android TextWatcher Tutorial with Examples
- Format Credit Card Number with Android TextWatcher
- Android Clipboard Tutorial with Examples
- Create a simple File Chooser in Android
- Android AutoCompleteTextView and MultiAutoCompleteTextView Tutorial with Examples
- Android ImageView Tutorial with Examples
- Android ImageSwitcher Tutorial with Examples
- Android ScrollView and HorizontalScrollView Tutorial with Examples
- Android WebView Tutorial with Examples
- Android SeekBar Tutorial with Examples
- Android Dialog Tutorial with Examples
- Android AlertDialog Tutorial with Examples
- Android RatingBar Tutorial with Examples
- Android ProgressBar Tutorial with Examples
- Android Spinner Tutorial with Examples
- Android Button Tutorial with Examples
- Android Switch Tutorial with Examples
- Android ImageButton Tutorial with Examples
- Android FloatingActionButton Tutorial with Examples
- Android CheckBox Tutorial with Examples
- Android RadioGroup and RadioButton Tutorial with Examples
- Android Chip and ChipGroup Tutorial with Examples
- Using image assets and icon assets of Android Studio
- Setting SD Card for Android Emulator
- ChipGroup and Chip Entry Example
- How to add external libraries to Android Project in Android Studio?
- How to disable the permissions already granted to the Android application?
- How to remove applications from Android Emulator?
- Android LinearLayout Tutorial with Examples
- Android TableLayout Tutorial with Examples
- Android FrameLayout Tutorial with Examples
- Android QuickContactBadge Tutorial with Examples
- Android StackView Tutorial with Examples
- Android Camera Tutorial with Examples
- Android MediaPlayer Tutorial with Examples
- Android VideoView Tutorial with Examples
- Playing Sound effects in Android with SoundPool
- Android Networking Tutorial with Examples
- Android JSON Parser Tutorial with Examples
- Android SharedPreferences Tutorial with Examples
- Android Internal Storage Tutorial with Examples
- Android External Storage Tutorial with Examples
- Android Intents Tutorial with Examples
- Example of an explicit Android Intent, calling another Intent
- Example of implicit Android Intent, open a URL, send an email
- Android Services Tutorial with Examples
- Android Notifications Tutorial with Examples
- Android DatePicker Tutorial with Examples
- Android TimePicker Tutorial with Examples
- Android Chronometer Tutorial with Examples
- Android OptionMenu Tutorial with Examples
- Android ContextMenu Tutorial with Examples
- Android PopupMenu Tutorial with Examples
- Android Fragments Tutorial with Examples
- Android ListView Tutorial with Examples
- Android ListView with Checkbox using ArrayAdapter
- Android GridView Tutorial with Examples
Show More