Android CheckBox Tutorial with Examples
1. Android CheckBox
In Android, CheckBox is a button with two states, checked and unchecked. It is a basic component and frequently used in Android applications.
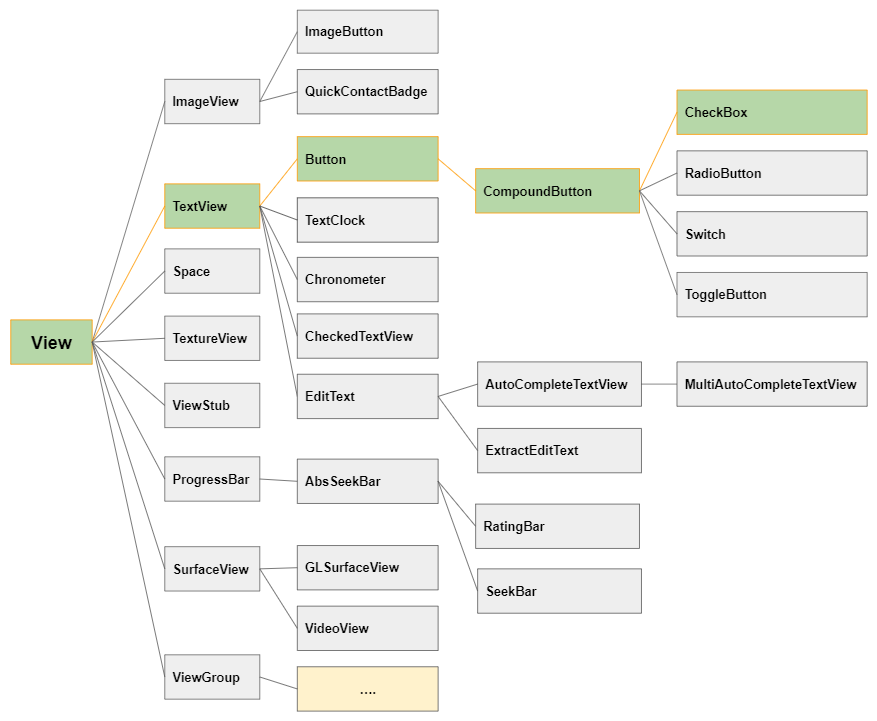
Basically, you can use multiple CheckBoxes in the application to allow users to select one or more options in a set of values.
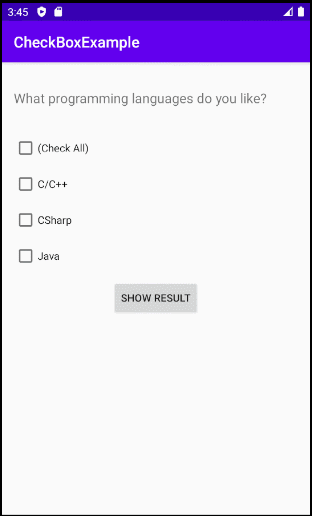
By default, the CheckBoxes have the unchecked state, so you can change its state via the android:checked attribute.
<CheckBox
android:id="@+id/someId"
android:checked="true"
... />
Some important attributes of CheckBox:
android:checked | Specify the current status for the CheckBox. |
android:gravity | Align the text of the CheckBox. The possible values are left, right, center, top, ... |
android:text | Set text content for CheckBox. |
android:textColor | Set the color of the text. |
android:textSize | Set font size of text. |
android:textStyle | Set text style (bold, italic, bolditalic). |
android:background | Set the background color for the CheckBox. |
android:padding | Set padding for CheckBox. |
android:onClick | The name of the method will be called when the user clicks on CheckBox. |
toggle()
All 4 classes of ToggleButton, CheckBox, RadioButton, Switch are subclasses of CompoundButton, so they inherit the toggle() method, which is the commonly used method to change their state from Checked (ON) to Unchecked (OFF), and vice versa.
CompoundButton button = (CheckBox) findViewById(R.id.checkBox);
button.toggle();
2. CheckBox Events
There are quite a few events related to a CheckBox, but here are two events used most often:
- checkBox.setOnClickListener(View.OnClickListener)
- checkBox.setOnCheckedChangeListener(CompoundButton.OnCheckedChangeListener)
On Click Event:
The event occurs when the user clicks on the CheckBox, similarly when the user clicks on a Button.
CheckBox chk = (CheckBox) findViewById(R.id.chk1);
chk.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
boolean checked = ((CheckBox) v).isChecked();
// Check which checkbox was clicked
if (checked){
// Your code
}
else{
// Your code
}
}
});
On Checked Change Event:
The event occurs when the CheckBox changes its state, due to the user's action or the effect of calling the checkBox.setChecked(newState) method.
CheckBox chk = (CheckBox) findViewById(R.id.chk1);
chk.setOnCheckedChangeListener(new CompoundButton.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(CompoundButton buttonView, boolean isChecked) {
if(isChecked) {
// Your code
} else {
// Your code
}
}
});
3. An example of the CheckBox
Now let's take a quick look before we start:
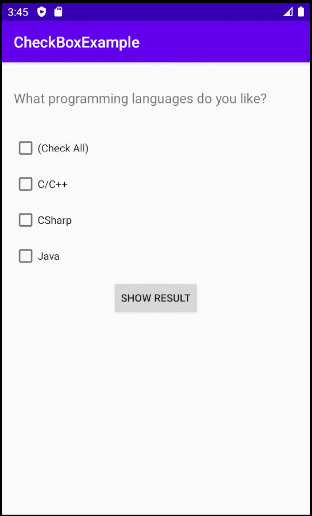
The interface of the example application will look like this:
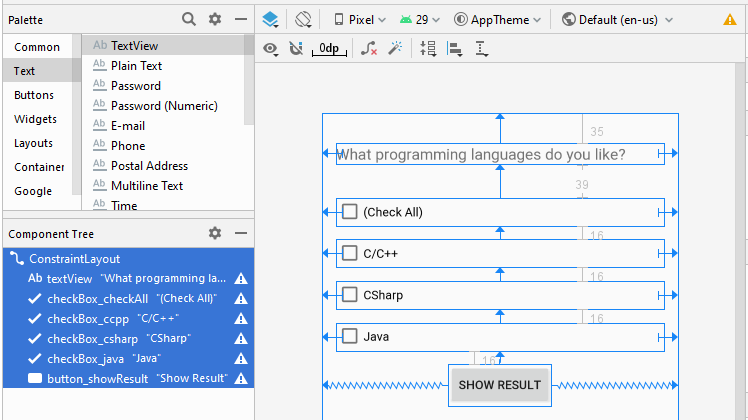
Note: If you are interested in the steps to design the interface of this application, please check out the appendix at the end of the article.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="35dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="What programming languages do you like?"
android:textSize="18sp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<CheckBox
android:id="@+id/checkBox_checkAll"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="39dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="(Check All)"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView" />
<CheckBox
android:id="@+id/checkBox_ccpp"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="C/C++"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/checkBox_checkAll" />
<CheckBox
android:id="@+id/checkBox_csharp"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="CSharp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/checkBox_ccpp" />
<CheckBox
android:id="@+id/checkBox_java"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Java"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/checkBox_csharp" />
<Button
android:id="@+id/button_showResult"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="16dp"
android:text="Show Result"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/checkBox_java" />
</androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.java
package com.example.checkboxexample;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.CheckBox;
import android.widget.CompoundButton;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
private CheckBox checkBoxCheckAll;
private CheckBox checkBoxCcpp;
private CheckBox checkBoxCsharp;
private CheckBox checkBoxJava;
private Button buttonShowResult;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.checkBoxCheckAll = (CheckBox) this.findViewById(R.id.checkBox_checkAll);
this.checkBoxCcpp = (CheckBox) this.findViewById(R.id.checkBox_ccpp);
this.checkBoxCsharp = (CheckBox) this.findViewById(R.id.checkBox_csharp);
this.checkBoxJava = (CheckBox) this.findViewById(R.id.checkBox_java);
this.buttonShowResult = (Button) this.findViewById(R.id.button_showResult);
this.buttonShowResult.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
showResult();
}
});
this.checkBoxCheckAll.setOnCheckedChangeListener(new CompoundButton.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(CompoundButton buttonView, boolean isChecked) {
checkAllCheckedChange(isChecked);
}
});
}
private void showResult() {
String message = null;
if(this.checkBoxCcpp.isChecked()) {
message = this.checkBoxCcpp.getText().toString();
}
if(this.checkBoxCsharp.isChecked()) {
if(message== null) {
message = this.checkBoxCsharp.getText().toString();
} else {
message += ", " + this.checkBoxCsharp.getText().toString();
}
}
if(this.checkBoxJava.isChecked()) {
if(message== null) {
message = this.checkBoxJava.getText().toString();
} else {
message += ", " + this.checkBoxJava.getText().toString();
}
}
message = message == null? "You select nothing": "You select: " + message;
Toast.makeText(this, message, Toast.LENGTH_LONG).show();
}
// When "Check All" change state.
private void checkAllCheckedChange(boolean isChecked) {
this.checkBoxCsharp.setChecked(isChecked);
this.checkBoxCcpp.setChecked(isChecked);
this.checkBoxJava.setChecked(isChecked);
}
}
Android Programming Tutorials
- Configure Android Emulator in Android Studio
- Android ToggleButton Tutorial with Examples
- Create a simple File Finder Dialog in Android
- Android TimePickerDialog Tutorial with Examples
- Android DatePickerDialog Tutorial with Examples
- What is needed to get started with Android?
- Install Android Studio on Windows
- Install Intel® HAXM for Android Studio
- Android AsyncTask Tutorial with Examples
- Android AsyncTaskLoader Tutorial with Examples
- Android Tutorial for Beginners - Basic examples
- How to know the phone number of Android Emulator and change it
- Android TextInputLayout Tutorial with Examples
- Android CardView Tutorial with Examples
- Android ViewPager2 Tutorial with Examples
- Get Phone Number in Android using TelephonyManager
- Android Phone Call Tutorial with Examples
- Android Wifi Scanning Tutorial with Examples
- Android 2D Game Tutorial for Beginners
- Android DialogFragment Tutorial with Examples
- Android CharacterPickerDialog Tutorial with Examples
- Android Tutorial for Beginners - Hello Android
- Using Android Device File Explorer
- Enable USB Debugging on Android Device
- Android UI Layouts Tutorial with Examples
- Android SMS Tutorial with Examples
- Android SQLite Database Tutorial with Examples
- Google Maps Android API Tutorial with Examples
- Android Text to Speech Tutorial with Examples
- Android Space Tutorial with Examples
- Android Toast Tutorial with Examples
- Create a custom Android Toast
- Android SnackBar Tutorial with Examples
- Android TextView Tutorial with Examples
- Android TextClock Tutorial with Examples
- Android EditText Tutorial with Examples
- Android TextWatcher Tutorial with Examples
- Format Credit Card Number with Android TextWatcher
- Android Clipboard Tutorial with Examples
- Create a simple File Chooser in Android
- Android AutoCompleteTextView and MultiAutoCompleteTextView Tutorial with Examples
- Android ImageView Tutorial with Examples
- Android ImageSwitcher Tutorial with Examples
- Android ScrollView and HorizontalScrollView Tutorial with Examples
- Android WebView Tutorial with Examples
- Android SeekBar Tutorial with Examples
- Android Dialog Tutorial with Examples
- Android AlertDialog Tutorial with Examples
- Android RatingBar Tutorial with Examples
- Android ProgressBar Tutorial with Examples
- Android Spinner Tutorial with Examples
- Android Button Tutorial with Examples
- Android Switch Tutorial with Examples
- Android ImageButton Tutorial with Examples
- Android FloatingActionButton Tutorial with Examples
- Android CheckBox Tutorial with Examples
- Android RadioGroup and RadioButton Tutorial with Examples
- Android Chip and ChipGroup Tutorial with Examples
- Using image assets and icon assets of Android Studio
- Setting SD Card for Android Emulator
- ChipGroup and Chip Entry Example
- How to add external libraries to Android Project in Android Studio?
- How to disable the permissions already granted to the Android application?
- How to remove applications from Android Emulator?
- Android LinearLayout Tutorial with Examples
- Android TableLayout Tutorial with Examples
- Android FrameLayout Tutorial with Examples
- Android QuickContactBadge Tutorial with Examples
- Android StackView Tutorial with Examples
- Android Camera Tutorial with Examples
- Android MediaPlayer Tutorial with Examples
- Android VideoView Tutorial with Examples
- Playing Sound effects in Android with SoundPool
- Android Networking Tutorial with Examples
- Android JSON Parser Tutorial with Examples
- Android SharedPreferences Tutorial with Examples
- Android Internal Storage Tutorial with Examples
- Android External Storage Tutorial with Examples
- Android Intents Tutorial with Examples
- Example of an explicit Android Intent, calling another Intent
- Example of implicit Android Intent, open a URL, send an email
- Android Services Tutorial with Examples
- Android Notifications Tutorial with Examples
- Android DatePicker Tutorial with Examples
- Android TimePicker Tutorial with Examples
- Android Chronometer Tutorial with Examples
- Android OptionMenu Tutorial with Examples
- Android ContextMenu Tutorial with Examples
- Android PopupMenu Tutorial with Examples
- Android Fragments Tutorial with Examples
- Android ListView Tutorial with Examples
- Android ListView with Checkbox using ArrayAdapter
- Android GridView Tutorial with Examples
Show More