Android FloatingActionButton Tutorial with Examples
1. Android FloatingActionButton
FloatingActionButton is a special button which is usually displayed as a circular shape with an Icon in the center. It floats on the surface of the interface allowing the user to click on it to perform an action.
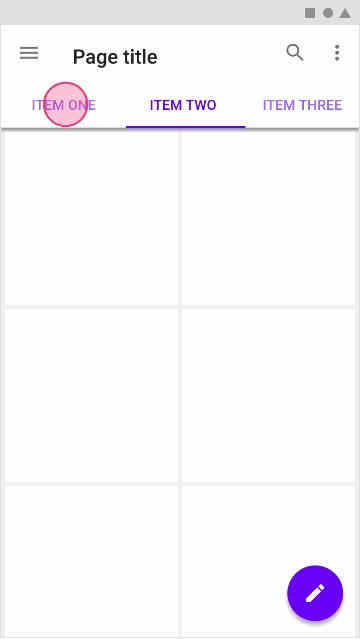
The FloatingActionButton is suitably displayed for different contexts.
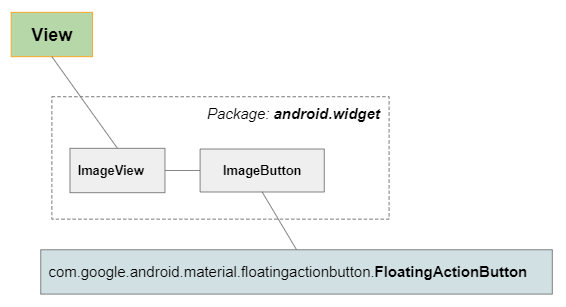
FloatingActionButton is a subclass of ImageButton, and is a descendant of ImageView, so you can set the Icon for it through the android:srcCompat attribute.
<com.google.android.material.floatingactionbutton.FloatingActionButton
android:id="@+id/fab"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:clickable="true"
app:srcCompat="@drawable/icon_new" ... />
The FloatingActionButton library is not available in the Android SDK, so you need to install it in your project from Palette of the design window, or add the library to the project manually.
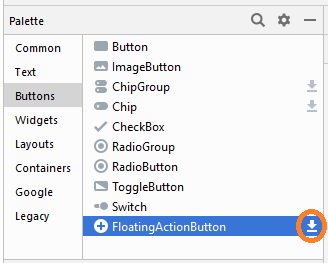
After being installed from Palette, the library has been declared in build.gradle (Module: app).
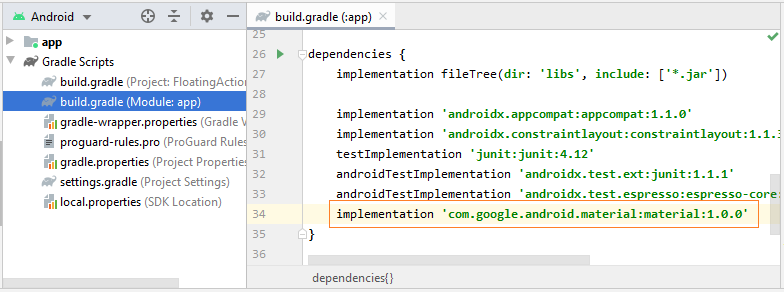
implementation 'com.google.android.material:material:1.0.0'
Check out the latest version of this library on mvnrepository.com:
2. FAB and CoordinatorLayout
FloatingActionButton (FAB) is commonly placed in a CoordinatorLayout where theFloatingActionButton(s) will hide/show in accordance with the context, and the transition of the View(s) is inside the CoordinatorLayout. This enhances the user's experience.
In this article I only mention the features of FAB instead of its relation with CoordinatorLayout. When understanding the features of FAB,you can combine them with a CoordinatorLayout. And the following article will be useful to you:
- Android CoordinatorLayout
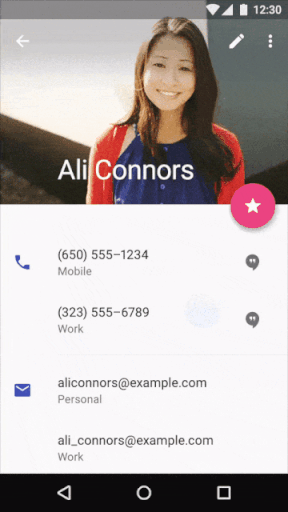
3. Các tình huống sử dụng FloatingActionButton
Normally, it depends on the context to use a FloatingActionButton (FAB), and FAB is the primary action given to the user. For example, you are on the screen to view an email list. An FAB can be displayedto allow the user to create a new Email (If it is thought that this action is commonly used by the user).
4. Example of FAB and ConstraintLayout
As mentioned above, FloatingActionButton (FAB) should be placed in a CoordinatorLayout in order to enhance the user's experience. However, in a few simple applications, CoordinatorLayout is not necessary. In this example, I will be putting a FloatingActionButton (fab) in a ConstraintLayout, and anchor it to the bottom right corner of the Layout. When the user clicks on this button, three other buttons (fab1, fab2, fab3) will show up next to the fab, or be pushed out of the user's sight.
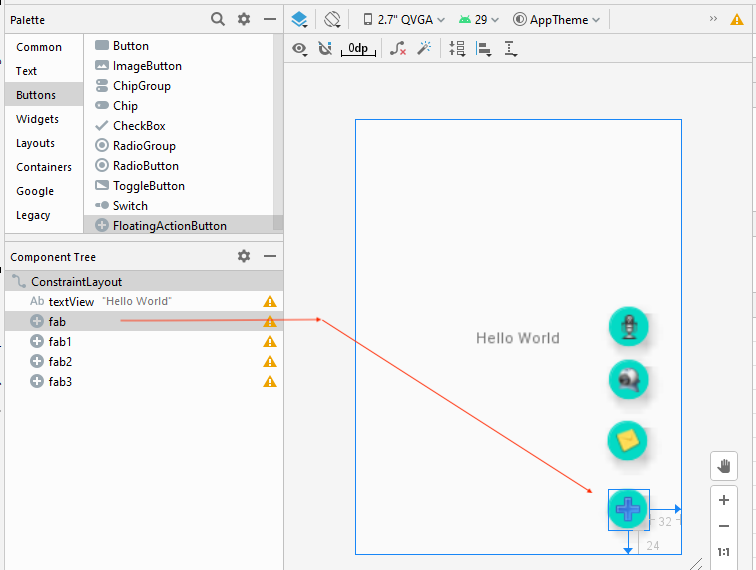
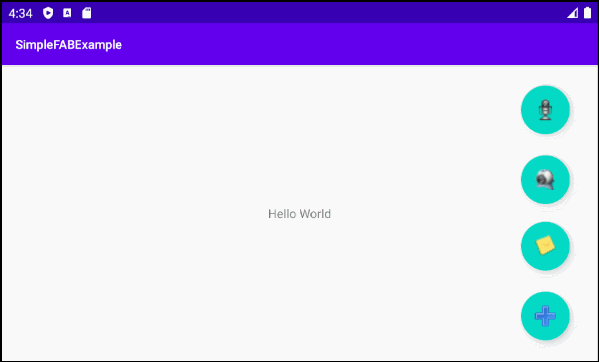
OK, now on Android Studio create a project:
- File > New > New Project > Empty Activity
- Name: SimpleFABExample
- Package name: org.o7planning.simplefabexample
- Language: Java
The FloatingActionButton component is not available in SDK of Android. In order to use the component, you need to install it into your project.
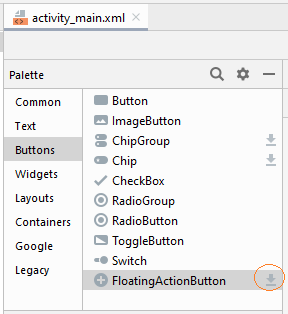
Or declare the library consisting of the FAB in build.gradle (Module: app) file.
....
dependencies {
...
implementation 'com.google.android.material:material:1.0.0
}
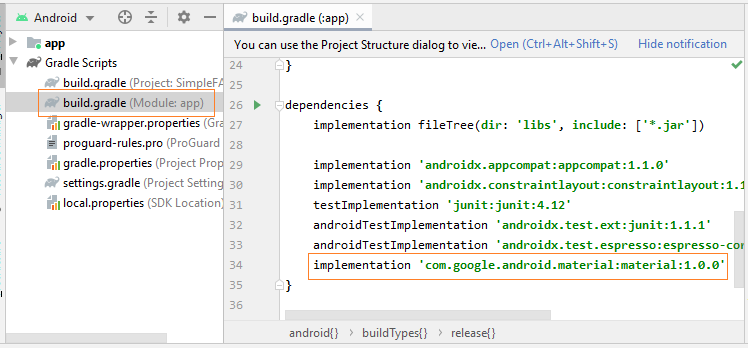
Copy several image files into the "drawable" folder.
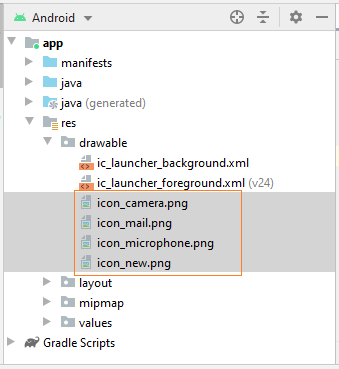
icon_new.png | icon_mail.png | icon_camera.png | icon_microphone.png |
Next, design the application interface:
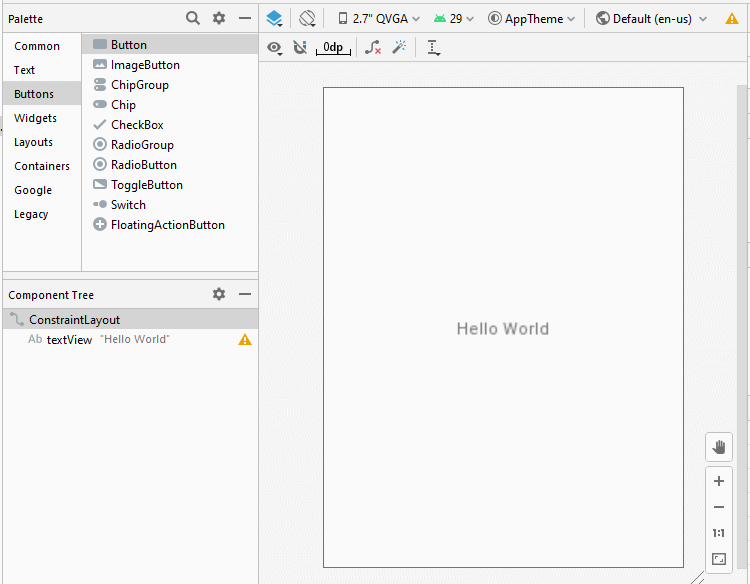
Then align the position of the FAB(s) in the interface:
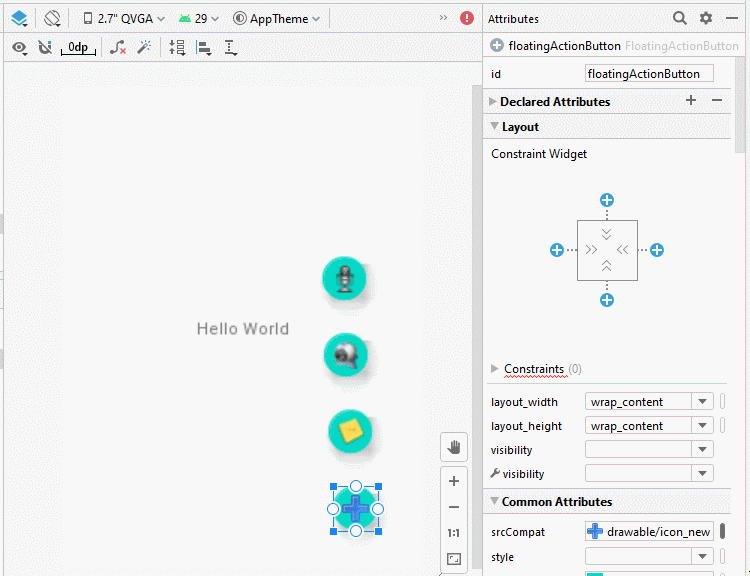
Finally, set ID to FAB(s):
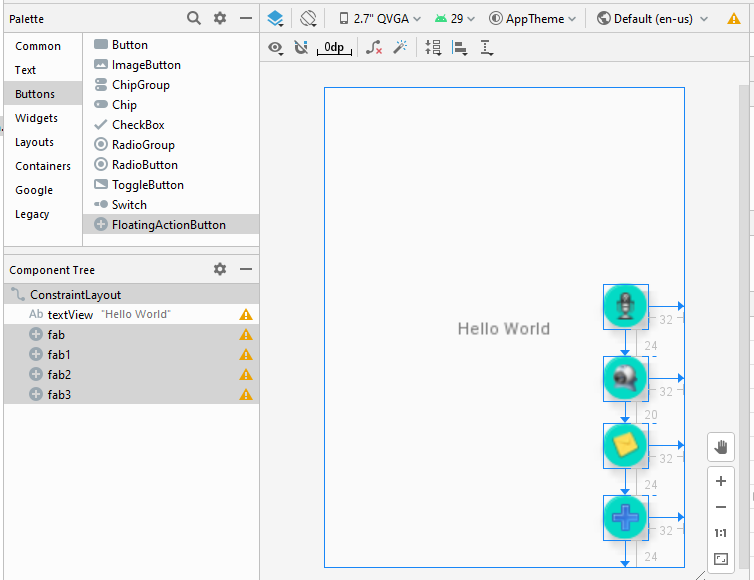
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello World"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<com.google.android.material.floatingactionbutton.FloatingActionButton
android:id="@+id/fab"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginEnd="32dp"
android:layout_marginRight="32dp"
android:layout_marginBottom="24dp"
android:clickable="true"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:srcCompat="@drawable/icon_new" />
<com.google.android.material.floatingactionbutton.FloatingActionButton
android:id="@+id/fab1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginEnd="32dp"
android:layout_marginRight="32dp"
android:layout_marginBottom="24dp"
android:clickable="true"
app:layout_constraintBottom_toTopOf="@+id/fab"
app:layout_constraintEnd_toEndOf="parent"
app:srcCompat="@drawable/icon_mail" />
<com.google.android.material.floatingactionbutton.FloatingActionButton
android:id="@+id/fab2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginEnd="32dp"
android:layout_marginRight="32dp"
android:layout_marginBottom="20dp"
android:clickable="true"
app:layout_constraintBottom_toTopOf="@+id/fab1"
app:layout_constraintEnd_toEndOf="parent"
app:srcCompat="@drawable/icon_camera" />
<com.google.android.material.floatingactionbutton.FloatingActionButton
android:id="@+id/fab3"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginEnd="32dp"
android:layout_marginRight="32dp"
android:layout_marginBottom="24dp"
android:clickable="true"
app:layout_constraintBottom_toTopOf="@+id/fab2"
app:layout_constraintEnd_toEndOf="parent"
app:srcCompat="@drawable/icon_microphone" />
</androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.java
package org.o7planning.simplefabexample;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import com.google.android.material.floatingactionbutton.FloatingActionButton;
public class MainActivity extends AppCompatActivity {
private FloatingActionButton fab;
private FloatingActionButton fab1;
private FloatingActionButton fab2;
private FloatingActionButton fab3;
private boolean isFABOpen;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.fab = (FloatingActionButton) findViewById(R.id.fab);
this.fab1 = (FloatingActionButton) findViewById(R.id.fab1);
this.fab2 = (FloatingActionButton) findViewById(R.id.fab2);
this.fab3 = (FloatingActionButton) findViewById(R.id.fab3);
this.fab.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
if(!isFABOpen){
showFABMenu();
} else{
closeFABMenu();
}
}
});
}
private void showFABMenu(){
isFABOpen=true;
fab1.animate().translationY(-getResources().getDimension(R.dimen.standard_55));
fab2.animate().translationY(-getResources().getDimension(R.dimen.standard_105));
fab3.animate().translationY(-getResources().getDimension(R.dimen.standard_155));
}
private void closeFABMenu(){
isFABOpen=false;
fab1.animate().translationY(0);
fab2.animate().translationY(0);
fab3.animate().translationY(0);
}
}
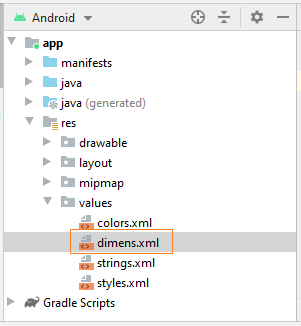
dimens.xml
<?xml version="1.0" encoding="utf-8"?>
<resources>
<dimen name="standard_55">255dp</dimen>
<dimen name="standard_105">2105dp</dimen>
<dimen name="standard_155">2155dp</dimen>
</resources>
Android Programming Tutorials
- Configure Android Emulator in Android Studio
- Android ToggleButton Tutorial with Examples
- Create a simple File Finder Dialog in Android
- Android TimePickerDialog Tutorial with Examples
- Android DatePickerDialog Tutorial with Examples
- What is needed to get started with Android?
- Install Android Studio on Windows
- Install Intel® HAXM for Android Studio
- Android AsyncTask Tutorial with Examples
- Android AsyncTaskLoader Tutorial with Examples
- Android Tutorial for Beginners - Basic examples
- How to know the phone number of Android Emulator and change it
- Android TextInputLayout Tutorial with Examples
- Android CardView Tutorial with Examples
- Android ViewPager2 Tutorial with Examples
- Get Phone Number in Android using TelephonyManager
- Android Phone Call Tutorial with Examples
- Android Wifi Scanning Tutorial with Examples
- Android 2D Game Tutorial for Beginners
- Android DialogFragment Tutorial with Examples
- Android CharacterPickerDialog Tutorial with Examples
- Android Tutorial for Beginners - Hello Android
- Using Android Device File Explorer
- Enable USB Debugging on Android Device
- Android UI Layouts Tutorial with Examples
- Android SMS Tutorial with Examples
- Android SQLite Database Tutorial with Examples
- Google Maps Android API Tutorial with Examples
- Android Text to Speech Tutorial with Examples
- Android Space Tutorial with Examples
- Android Toast Tutorial with Examples
- Create a custom Android Toast
- Android SnackBar Tutorial with Examples
- Android TextView Tutorial with Examples
- Android TextClock Tutorial with Examples
- Android EditText Tutorial with Examples
- Android TextWatcher Tutorial with Examples
- Format Credit Card Number with Android TextWatcher
- Android Clipboard Tutorial with Examples
- Create a simple File Chooser in Android
- Android AutoCompleteTextView and MultiAutoCompleteTextView Tutorial with Examples
- Android ImageView Tutorial with Examples
- Android ImageSwitcher Tutorial with Examples
- Android ScrollView and HorizontalScrollView Tutorial with Examples
- Android WebView Tutorial with Examples
- Android SeekBar Tutorial with Examples
- Android Dialog Tutorial with Examples
- Android AlertDialog Tutorial with Examples
- Android RatingBar Tutorial with Examples
- Android ProgressBar Tutorial with Examples
- Android Spinner Tutorial with Examples
- Android Button Tutorial with Examples
- Android Switch Tutorial with Examples
- Android ImageButton Tutorial with Examples
- Android FloatingActionButton Tutorial with Examples
- Android CheckBox Tutorial with Examples
- Android RadioGroup and RadioButton Tutorial with Examples
- Android Chip and ChipGroup Tutorial with Examples
- Using image assets and icon assets of Android Studio
- Setting SD Card for Android Emulator
- ChipGroup and Chip Entry Example
- How to add external libraries to Android Project in Android Studio?
- How to disable the permissions already granted to the Android application?
- How to remove applications from Android Emulator?
- Android LinearLayout Tutorial with Examples
- Android TableLayout Tutorial with Examples
- Android FrameLayout Tutorial with Examples
- Android QuickContactBadge Tutorial with Examples
- Android StackView Tutorial with Examples
- Android Camera Tutorial with Examples
- Android MediaPlayer Tutorial with Examples
- Android VideoView Tutorial with Examples
- Playing Sound effects in Android with SoundPool
- Android Networking Tutorial with Examples
- Android JSON Parser Tutorial with Examples
- Android SharedPreferences Tutorial with Examples
- Android Internal Storage Tutorial with Examples
- Android External Storage Tutorial with Examples
- Android Intents Tutorial with Examples
- Example of an explicit Android Intent, calling another Intent
- Example of implicit Android Intent, open a URL, send an email
- Android Services Tutorial with Examples
- Android Notifications Tutorial with Examples
- Android DatePicker Tutorial with Examples
- Android TimePicker Tutorial with Examples
- Android Chronometer Tutorial with Examples
- Android OptionMenu Tutorial with Examples
- Android ContextMenu Tutorial with Examples
- Android PopupMenu Tutorial with Examples
- Android Fragments Tutorial with Examples
- Android ListView Tutorial with Examples
- Android ListView with Checkbox using ArrayAdapter
- Android GridView Tutorial with Examples
Show More