What are polyfills in programming science?
1. Polyfill
In layman’s terms, one can imagine a polyfill as a common paste which can be used to fill holes and cracks to smooth out any defects in a wall. In the UK there is a popular brand name for this paste which is called “Polyfilla”.
For the web, the term "Polyfill" has a similar meaning. A Polyfill is a small piece of code or a missing browser function that needs to be added or filled in.
For easy understanding, take a look at a small ES6 JavaScript code snippet below:
// ES6 Code
var s = 'Tom and Jerry';
if (s.startsWith('Tom')) {
console.log('Hello Tom');
}
The startsWith method is part of the ES6 specification published in 2015. The code above works perfectly on new browsers, such as Chrome 41+. Note: Chrome version 41 only partially supports ES6 features. It was only when Chrome version 51 (May 2016) was released that ES6 was fully supported by Chrome.
Browser | Version Supports startsWith | Version Fully Support ES6 | Date |
Chrome | 41 | 51 | May 2016 |
Edge | 12 | 14 | Aug 2016 |
Firefox | 17 | 54 | Jun 2017 |
Internet Explorer | No | No | |
Safari | 9 | 10 | Sep 2016 |
Opera | 28 | 38 | Jun 2016 |
To support old browsers you must patch functions that are missing. In this case, make sure to check if the browser supports the startsWith method. If it doesn't, you need to simulate it with equivalent feature:
// ES5 Code
if (!String.prototype.startsWith) {
String.prototype.startsWith = function (searchString, position) {
position = position || 0;
return this.substr(position, searchString.length) === searchString;
};
}
var s = 'Tom and Jerry';
if (s.startsWith('Tom')) {
console.log('Hello Tom');
}
Another example of a polyfill. The static method Math.trunc(number) is used to "truncate" the decimal part of a number and return an integer. For example Math.trunc(1.23) returns 1. Old browsers do not support the method, therefore we need to simulate this method to add to it.
if (!Math.trunc) { // if no such method
// implement it
Math.trunc = function (number) {
// Math.ceil and Math.floor exist even in ancient JavaScript engines
// they are covered later in the tutorial
return number < 0 ? Math.ceil(number) : Math.floor(number);
};
}
By the time this article is released (2021), we can basically be sure that most users have switched to using a browser that supports JavaScript ES5+. So creating polyfill(s) for "too old" browsers (ES4-) is not really necessary anymore.
caniuse.com is a website that helps you test which browsers support a certain feature. For example, check browser support for the startsWith method
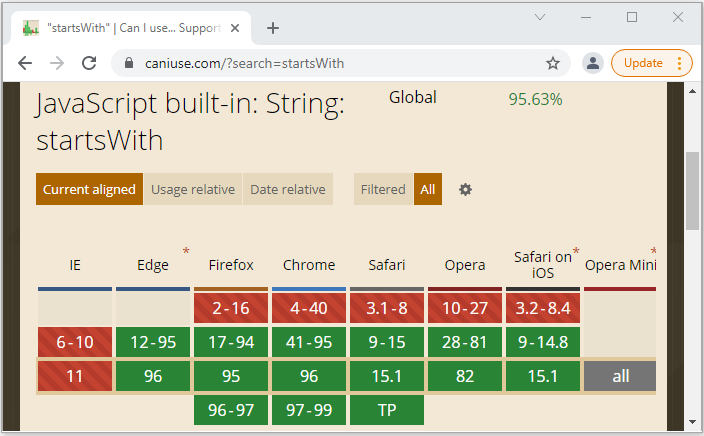
2. Ways to use Polyfill(s)
Basically, there are 2 ways to use Polyfill:
Polyfilling manually
Manually write and use your Polyfill(s) like the examples above.
Polyfill library
Many Polyfill libraries are created by the community and are freely shared. They are often used in conjunction with Transpiler(s).
For example, JavaScript Polyfill libraries like babel-polyfill, Es6-shim, polyfill-library,... can be imported into your projects, such as NodeJS, React,...
A few examples using the Polyfill libraries, which you may be interested in:
- NodeJS + babel-polyfill
ECMAScript, Javascript Tutorials
- Introduction to Javascript and ECMAScript
- Quickstart with Javascript
- Alert, Confirm, Prompt Dialog Box in Javascript
- Quickstart with JavaScript
- JavaScript Variables Tutorial with Examples
- Bitwise Operations
- JavaScript Arrays Tutorial with Examples
- JavaScript Loops Tutorial with Examples
- JavaScript Functions Tutorial with Examples
- JavaScript Number Tutorial with Examples
- JavaScript Boolean Tutorial with Examples
- JavaScript Strings Tutorial with Examples
- JavaScript if else Statement Tutorial with Examples
- JavaScript Switch Statement
- JavaScript Error Handling Tutorial with Examples
- JavaScript Date Tutorial with Examples
- JavaScript Modules Tutorial with Examples
- The History of Modules in JavaScript
- JavaScript setTimeout and setInterval Function
- Javascript Form Validation Tutorial with Examples
- JavaScript Web Cookies Tutorial with Examples
- JavaScript void Keyword Tutorial with Examples
- Classes and Objects in JavaScript
- Class and inheritance simulation techniques in JavaScript
- Inheritance and polymorphism in JavaScript
- Undertanding Duck Typing in JavaScript
- JavaScript Symbols Tutorial with Examples
- JavaScript Set Collection Tutorial with Examples
- JavaScript Map Collection Tutorial with Examples
- Undertanding JavaScript Iterables and Iterators
- JavaScript Regular Expressions Tutorial with Examples
- JavaScript Promise, Async/Await Tutorial with Examples
- Javascript Window Tutorial with Examples
- Javascript Console Tutorial with Examples
- Javascript Screen Tutorial with Examples
- Javascript Navigator Tutorial with Examples
- Javascript Geolocation API Tutorial with Examples
- Javascript Location Tutorial with Examples
- Javascript History API Tutorial with Examples
- Javascript Statusbar Tutorial with Examples
- Javascript Locationbar Tutorial with Examples
- Javascript Scrollbars Tutorial with Examples
- Javascript Menubar Tutorial with Examples
- JavaScript JSON Tutorial with Examples
- JavaScript Event Handling Tutorial with Examples
- Javascript MouseEvent Tutorial with Examples
- Javascript WheelEvent Tutorial with Examples
- Javascript KeyboardEvent Tutorial with Examples
- Javascript FocusEvent Tutorial with Examples
- Javascript InputEvent Tutorial with Examples
- Javascript ChangeEvent Tutorial with Examples
- Javascript DragEvent Tutorial with Examples
- Javascript HashChangeEvent Tutorial with Examples
- Javascript URL Encoding Tutorial with Examples
- Javascript FileReader Tutorial with Examples
- Javascript XMLHttpRequest Tutorial with Examples
- Javascript Fetch API Tutorial with Examples
- Parsing XML in Javascript with DOMParser
- Introduction to Javascript HTML5 Canvas API
- Highlighting code with SyntaxHighlighter Javascript library
- What are polyfills in programming science?
Show More