JavaScript Switch Statement
1. The structure of switch statement
Syntax
// variable_to_test: A varible to test.
switch ( variable_to_test ) {
case value1:
// Do something here ...
break;
case value2:
// Do something here ...
break;
default:
// Do something here ...
}
The characteristics of switch statement:
The switch will check the value of a variable and compare that variable with each different value from top to bottom. Each value to be compared is called a case. When a case is true, the statement block of that case will be executed.
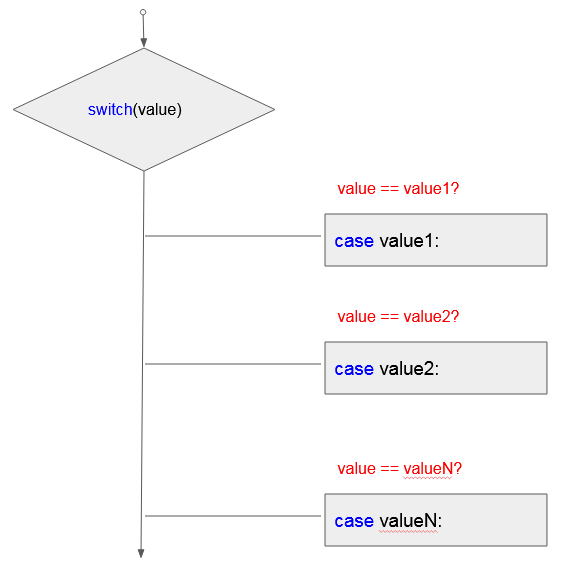
If all cases are false, the default statement block will be executed. Note: in the structure of the switch statement, there is probably or not a default statement block.
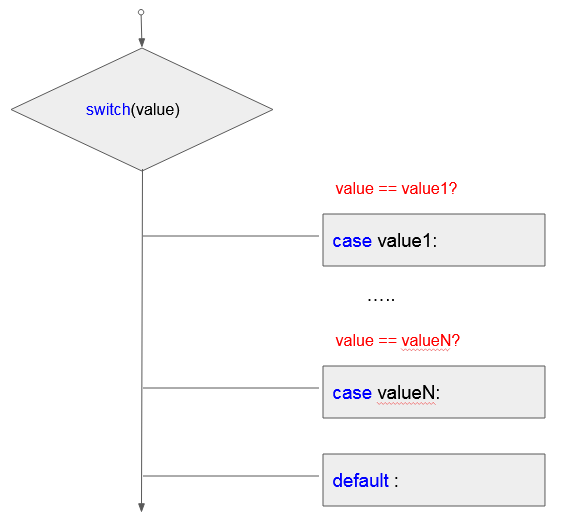
When finding a true case, the statement block of such case will be executed. If not meeting the break statement in this block, the program will continue performing the blocks below until it meets the break statement or no statement block is executed.
The break statement makes the program exit the switch. (See the illustration below).
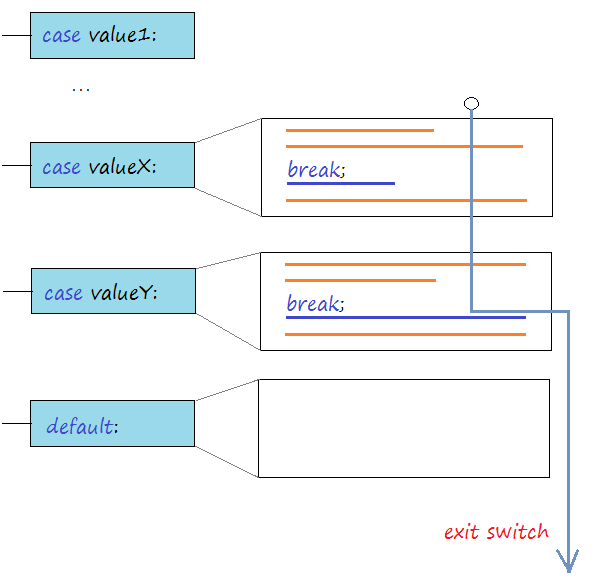
Note that the case statement is a specific value you can not do the following:
// This is not allowed !!
case (age < 18) :
// case only accept a specific value, eg:
case 18:
// Do something here ..
break;
2. switch Example
switch-example.js
// Declare a variable age
let age = 20;
// Check the value of age
switch (age) {
// Case age = 18
case 18:
console.log("You are 18 year old");
break;
// Case age = 20
case 20:
console.log("You are 20 year old");
break;
// Remaining cases
default:
console.log("You are not 18, 20 year old");
}
Output:
You are 20 year old
3. break statement in switch
break means a statement to be able to appear in the case block, or default block of switch. When meeting the break statement, the program will exit the switch.
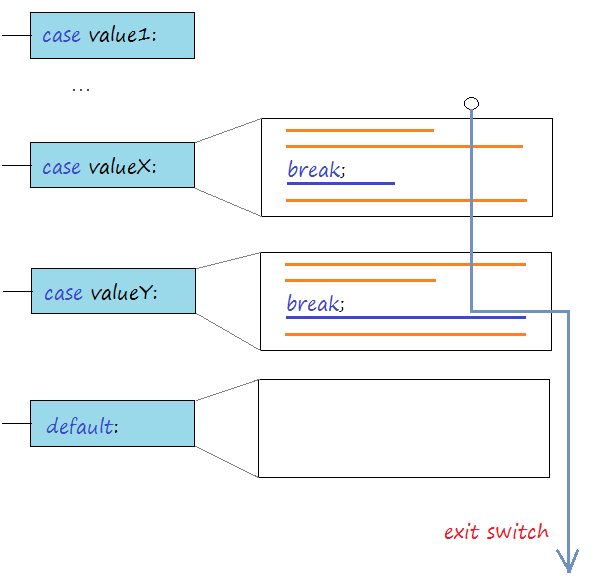
When the program meets a switch statement, it will check cases from top to bottom. When finding a true case, the block of that case will be executed. If it doesn't meet the break statement in this block, it will continue to execute the blocks below until it meets the break statement or there is no longer block to execute.
Example:
switch-example2.js
// Declare a variable age.
let age = 30;
// Check the value of age.
switch (age) {
// Case age = 18
case 18:
console.log("You are 18 year old");
break;
// Case age in 20, 30, 40
case 20:
case 30:
case 40:
console.log("You are " + age);
break;
// Remaining case:
default:
console.log("Other age");
}
Output:
You are 30
ECMAScript, Javascript Tutorials
- Introduction to Javascript and ECMAScript
- Quickstart with Javascript
- Alert, Confirm, Prompt Dialog Box in Javascript
- Quickstart with JavaScript
- JavaScript Variables Tutorial with Examples
- Bitwise Operations
- JavaScript Arrays Tutorial with Examples
- JavaScript Loops Tutorial with Examples
- JavaScript Functions Tutorial with Examples
- JavaScript Number Tutorial with Examples
- JavaScript Boolean Tutorial with Examples
- JavaScript Strings Tutorial with Examples
- JavaScript if else Statement Tutorial with Examples
- JavaScript Switch Statement
- JavaScript Error Handling Tutorial with Examples
- JavaScript Date Tutorial with Examples
- JavaScript Modules Tutorial with Examples
- The History of Modules in JavaScript
- JavaScript setTimeout and setInterval Function
- Javascript Form Validation Tutorial with Examples
- JavaScript Web Cookies Tutorial with Examples
- JavaScript void Keyword Tutorial with Examples
- Classes and Objects in JavaScript
- Class and inheritance simulation techniques in JavaScript
- Inheritance and polymorphism in JavaScript
- Undertanding Duck Typing in JavaScript
- JavaScript Symbols Tutorial with Examples
- JavaScript Set Collection Tutorial with Examples
- JavaScript Map Collection Tutorial with Examples
- Undertanding JavaScript Iterables and Iterators
- JavaScript Regular Expressions Tutorial with Examples
- JavaScript Promise, Async/Await Tutorial with Examples
- Javascript Window Tutorial with Examples
- Javascript Console Tutorial with Examples
- Javascript Screen Tutorial with Examples
- Javascript Navigator Tutorial with Examples
- Javascript Geolocation API Tutorial with Examples
- Javascript Location Tutorial with Examples
- Javascript History API Tutorial with Examples
- Javascript Statusbar Tutorial with Examples
- Javascript Locationbar Tutorial with Examples
- Javascript Scrollbars Tutorial with Examples
- Javascript Menubar Tutorial with Examples
- JavaScript JSON Tutorial with Examples
- JavaScript Event Handling Tutorial with Examples
- Javascript MouseEvent Tutorial with Examples
- Javascript WheelEvent Tutorial with Examples
- Javascript KeyboardEvent Tutorial with Examples
- Javascript FocusEvent Tutorial with Examples
- Javascript InputEvent Tutorial with Examples
- Javascript ChangeEvent Tutorial with Examples
- Javascript DragEvent Tutorial with Examples
- Javascript HashChangeEvent Tutorial with Examples
- Javascript URL Encoding Tutorial with Examples
- Javascript FileReader Tutorial with Examples
- Javascript XMLHttpRequest Tutorial with Examples
- Javascript Fetch API Tutorial with Examples
- Parsing XML in Javascript with DOMParser
- Introduction to Javascript HTML5 Canvas API
- Highlighting code with SyntaxHighlighter Javascript library
- What are polyfills in programming science?
Show More