Javascript InputEvent Tutorial with Examples
1. InputEvent
InputEvent is an interface that represents events occurring when a user enters data on the editable elements such as <input>, <textarea> or the elements with contenteditable="true" attributes.
InputEvent is a sub-interface of UIEvent.
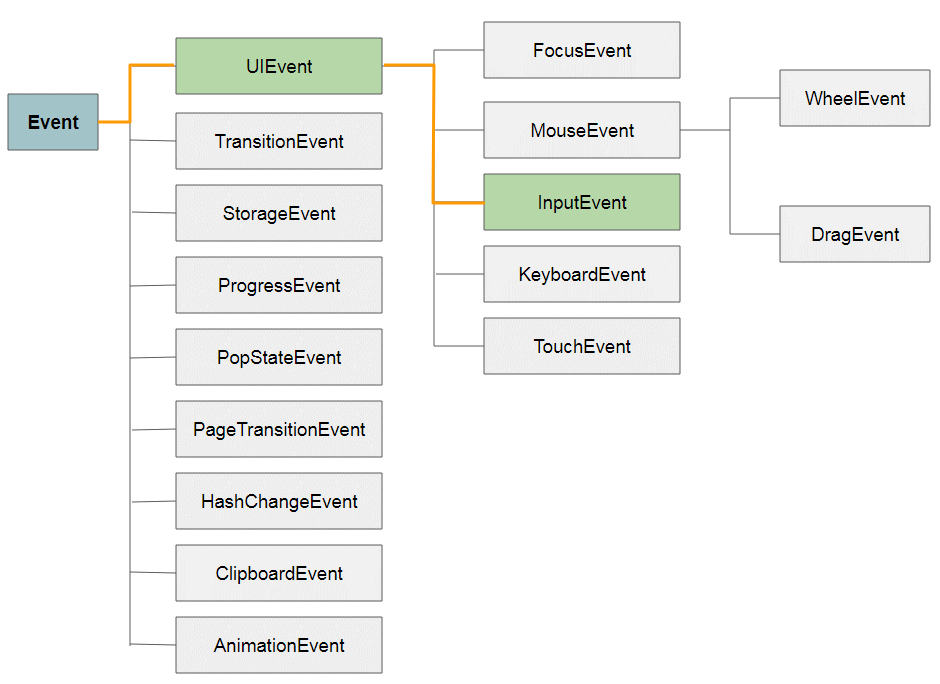
Events:
Event | Description |
input | The event occurs when an element gets user input |
The browsers that support InputEvent:

Example of elements that support the input event:
inputevents-elements-example.html
<!DOCTYPE html>
<html>
<head>
<title>InputEvent Example</title>
<meta charset="UTF-8">
<style>
*[contenteditable='true'] {
height:30px;padding:5px;border:1px solid #ccd;
}
</style>
<script>
// Note: event.data is not supported in Firefox, IE
function inputHandler(evt) {
showLog(evt.data);
}
function showLog(text) {
var logDiv = document.getElementById("log-div");
logDiv.innerHTML = logDiv.innerHTML + " .. " + text;
}
</script>
</head>
<body>
<h3>InputEvent example</h3>
<p style="color:red">Note: event.data is not supported in Firefox, IE</p>
<h3>Input element</h3>
<input type="text" oninput ="inputHandler(event)"/>
<h3>Textarea element</h3>
<textarea oninput ="inputHandler(event)" rows="5" cols="30"></textarea>
<h3>Elements with contenteditable='true'</h3>
<div oninput ="inputHandler(event)" contenteditable="true"></div>
<p id="log-div"></p>
</body>
</html>
Events: input vs change
You also can consider using the change event because it is quite similar to the input event:
- The change event supports the <select> element. It occurs when users change the option on <select>.
- For the <input> (text, number, email, password, tel, time, week, month, date, datetime-local, search, url), <textarea> elements: The input event occurs immediately after the value of element changes. While the change event occurs when the value of element changes and the element loses focus.
2. Properties, Methods
InputEvent is the sub-interface of UIEvent so it inherits all methods and properties from UIEvent. In addition, it has its own methods and properties.
Properties:
Property | Description |
data | Returns the inserted characters. This may be an empty string if the change doesn't insert text (such as when deleting characters, for example). |
dataTransfer | Returns a DataTransfer object containing information about richtext or plaintext data being added to or removed from editable content. |
inputType | Returns the type of change for editable content such as, for example, 'inserting', 'deleting', 'formatting', ... |
isComposing | Returns true/false indicating if the event is fired after compositionstart and before compositionend. |
The possible values of inputType:
- insertText
- insertReplacementText
- insertLineBreak
- insertParagraph
- insertOrderedList
- insertUnorderedList
- insertHorizontalRule
- insertFromYank
- insertFromDrop
- insertFromPaste
- insertTranspose
- insertCompositionText
- insertFromComposition
- insertLink
- deleteByComposition
- deleteCompositionText
- deleteWordBackward
- deleteWordForward
- deleteSoftLineBackward
- deleteSoftLineForward
- deleteEntireSoftLine
- deleteHardLineBackward
- deleteHardLineForward
- deleteByDrag
- deleteByCut
- deleteByContent
- deleteContentBackward
- deleteContentForward
- historyUndo
- historyRedo
- formatBold
- formatItalic
- formatUnderline
- formatStrikethrough
- formatSuperscript
- formatSubscript
- formatJustifyFull
- formatJustifyCenter
- formatJustifyRight
- formatJustifyLeft
- formatIndent
- formatOutdent
- formatRemove
- formatSetBlockTextDirection
- formatSetInlineTextDirection
- formatBackColor
- formatFontColor
- formatFontName
Methods:
Method | Description |
getTargetRanges() | Returns an array containing target ranges that will be affected by this event. |
Note: Although InputEvent is supported by all browsers, some properties and methods of InputEvent are not supported in some browsers, so you should consider using them.
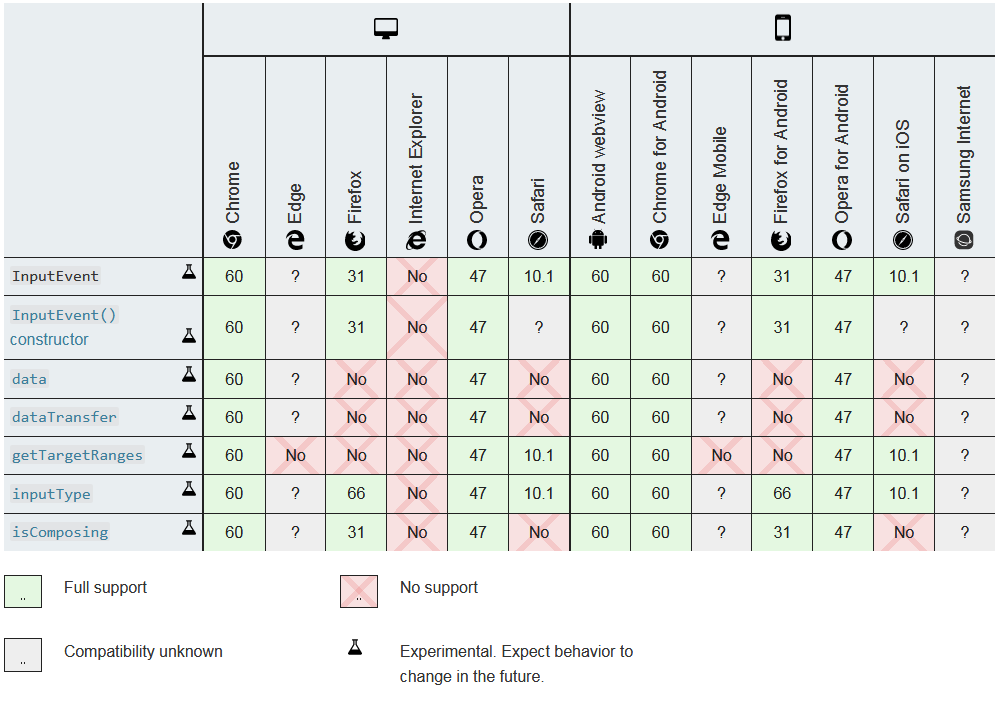
Example:
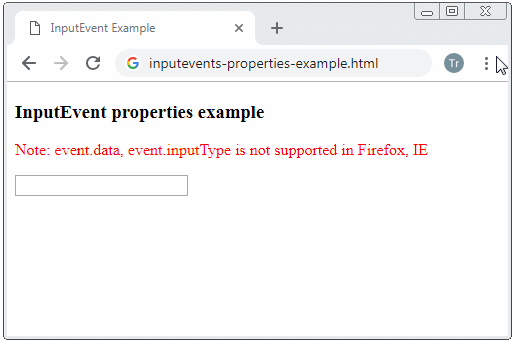
inputevents-properties-example.html
<!DOCTYPE html>
<html>
<head>
<title>InputEvent Example</title>
<meta charset="UTF-8">
<script>
// Note: event.data, event.inputType is not supported in Firefox, IE
function inputHandler(evt) {
showLog(evt.data +" - " + evt.inputType);
}
function showLog(text) {
var logDiv = document.getElementById("log-div");
logDiv.innerHTML = text;
}
</script>
</head>
<body>
<h3>InputEvent properties example</h3>
<p style="color:red">Note: event.data, event.inputType is not supported in Firefox, IE</p>
<input type="text" oninput ="inputHandler(event)"/>
<p id="log-div"></p>
</body>
</html>
ECMAScript, Javascript Tutorials
- Introduction to Javascript and ECMAScript
- Quickstart with Javascript
- Alert, Confirm, Prompt Dialog Box in Javascript
- Quickstart with JavaScript
- JavaScript Variables Tutorial with Examples
- Bitwise Operations
- JavaScript Arrays Tutorial with Examples
- JavaScript Loops Tutorial with Examples
- JavaScript Functions Tutorial with Examples
- JavaScript Number Tutorial with Examples
- JavaScript Boolean Tutorial with Examples
- JavaScript Strings Tutorial with Examples
- JavaScript if else Statement Tutorial with Examples
- JavaScript Switch Statement
- JavaScript Error Handling Tutorial with Examples
- JavaScript Date Tutorial with Examples
- JavaScript Modules Tutorial with Examples
- The History of Modules in JavaScript
- JavaScript setTimeout and setInterval Function
- Javascript Form Validation Tutorial with Examples
- JavaScript Web Cookies Tutorial with Examples
- JavaScript void Keyword Tutorial with Examples
- Classes and Objects in JavaScript
- Class and inheritance simulation techniques in JavaScript
- Inheritance and polymorphism in JavaScript
- Undertanding Duck Typing in JavaScript
- JavaScript Symbols Tutorial with Examples
- JavaScript Set Collection Tutorial with Examples
- JavaScript Map Collection Tutorial with Examples
- Undertanding JavaScript Iterables and Iterators
- JavaScript Regular Expressions Tutorial with Examples
- JavaScript Promise, Async/Await Tutorial with Examples
- Javascript Window Tutorial with Examples
- Javascript Console Tutorial with Examples
- Javascript Screen Tutorial with Examples
- Javascript Navigator Tutorial with Examples
- Javascript Geolocation API Tutorial with Examples
- Javascript Location Tutorial with Examples
- Javascript History API Tutorial with Examples
- Javascript Statusbar Tutorial with Examples
- Javascript Locationbar Tutorial with Examples
- Javascript Scrollbars Tutorial with Examples
- Javascript Menubar Tutorial with Examples
- JavaScript JSON Tutorial with Examples
- JavaScript Event Handling Tutorial with Examples
- Javascript MouseEvent Tutorial with Examples
- Javascript WheelEvent Tutorial with Examples
- Javascript KeyboardEvent Tutorial with Examples
- Javascript FocusEvent Tutorial with Examples
- Javascript InputEvent Tutorial with Examples
- Javascript ChangeEvent Tutorial with Examples
- Javascript DragEvent Tutorial with Examples
- Javascript HashChangeEvent Tutorial with Examples
- Javascript URL Encoding Tutorial with Examples
- Javascript FileReader Tutorial with Examples
- Javascript XMLHttpRequest Tutorial with Examples
- Javascript Fetch API Tutorial with Examples
- Parsing XML in Javascript with DOMParser
- Introduction to Javascript HTML5 Canvas API
- Highlighting code with SyntaxHighlighter Javascript library
- What are polyfills in programming science?
Show More