Javascript Geolocation API Tutorial with Examples
1. Geolocation API
Geolocation API includes interfaces helping you determine the position of the user who is using the browser and you can interact with these interfaces through Javascript.This allows a website or an application to be able to provide a customization content based on the user's position.
Basically, Geolocation API includes the following interfaces:
- Geolocation
- PositionOptions
- Position
- Coordinates
- PositionError
When Geolocation API tries to take information on the user's position, the browser will display a dialog box to ask the user to allow. It is noted that each browser has its own policy and method to request for this right.
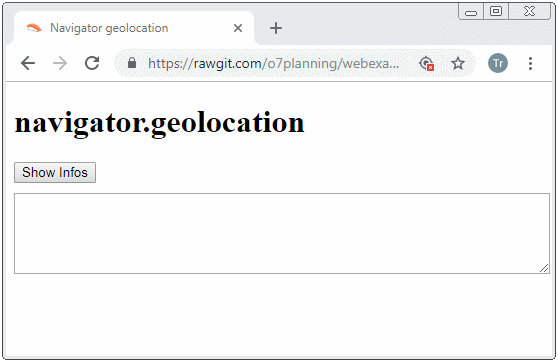
geolocation-example.js
// Success Handler
function successHandler(position) {
var logArea = document.getElementById("log-area");
logArea.value = "";
logArea.value += "Latitude: " + position.coords.latitude + "\n";
logArea.value += "Longitude: " + position.coords.longitude + "\n";
}
// Error Handler
function errorHandler(positionError) {
if(positionError.code == 1) { // PERMISSION_DENIED
alert("Error: Permission Denied! " + positionError.message);
} else if(positionError.code == 2) { // POSITION_UNAVAILABLE
alert("Error: Position Unavailable! " + positionError.message);
} else if(positionError.code == 3) { // TIMEOUT
alert("Error: Timeout!" + positionError.message);
}
}
function showInfos() {
navigator.geolocation.getCurrentPosition(successHandler, errorHandler);
}
geolocation-example.html
<!DOCTYPE html>
<html>
<head>
<title>Geolocation</title>
<meta charset="UTF-8">
<style>textarea {width:100%;margin-top:10px;}</style>
<script src="geolocation-example.js"></script>
</head>
<body>
<h1>Geolocation</h1>
<button onClick="showInfos()">Show Infos</button>
<textarea name="name" rows="5" id="log-area"></textarea>
</body>
</html>
2. PositionOptions interface
PositionOptions is the interface describing an object with optional properties. This object is passed in as a parameter for Geolocation.getCurrentPosition() method or Geolocation.watchPosition() method.
var options = {
maximumAge : 1000,
timeout: 5000,
enableHighAccuracy : true
};
PositionOptions has 3 properties:
- maximumAge
- timeout
- enableHighAccuracy
maximumAge
User's latest position information will be stored in the cache memory for the maximumAge milliseconds. The program will take the value in the cache to return to the user instead of searching real time position. Default is 0, which means that the program always searches position in real time.
timeout
Time is in maximum milisecond which the program waits for the result of locating users. If in such period of time, the user is not located, it is considered as failure and the Callback-error function will be called.
enableHighAccuracy
The value of this parameter is true/false. The true value means that the program tries to seek the best position, for example, telephone has GPS chip, it will be used to determine a position more exact. This takes more time and energy. Default value is false.
3. Geolocation interface
Geolocation is the most important interface in Geolocation API. You can access it through the navigator.geolocation property.
navigator.geolocation
// Geolocation object:
var geo = navigator.geolocation;
// Or:
var geo = window.navigator.geolocation;
The methods of Geolocation:
- getCurrentPosition()
- watchPosition()
- clearWatch()
getCurrentPosition(success[,error[, [options]])
The getCurrentPosition() method is used to get the current position of the device.
// success: A Callback function
// error: A Callback function (Optional)
// options: PositionOptions object (Optional)
getCurrentPosition(success[, error[, [options]])
// position: Position object.
var success = function(position) {
}
// positionError: PositionError object.
var error = function( positionError ) {
}
Parameters:
success
A Callback function has a parameter - position (The object of Position interface ). It is called when averything is successful, including the fact that users allow Geolocation API determine their positions and this API can determine users' position.
error
A Callback function has a parameter - positionError (the object of PositionError interface). It is called when the users do not allow Geolocation API to view their position, or cannot determine the user location, or timeout.
options
Being an object of the PositionOptions interface includes the options:
- maximumAge
- timeout
- enableHighAccuracy
** See more the specification of the PositionOptions interface in this document.
watchPosition(success[, error[, options]])
The watchPosition() method is used to register a handler function that will be called automatically each time the position of the device changes. This method returns an integer, which is the code of the task.
// success: A Callback function
// error: A Callback function (Optional)
// options: PositionOptions object (Optional)
// watchID: An Integer Number.
var watchID = watchPosition(success[, error[, [options]])
// position: Position object.
var success = function(position) {
}
// positionError: PositionError object.
var error = function( positionError ) {
}
Parameters:
success
A Callback function has a parameter -position (the Object of Position interface). It is called when everything succeeds, including the fact that users allow Geolocation API to determine their position, and this API determines users' position successfully.
error
A Callback function with a parameter - positionError (An object of the PositionError interface) is called when users don't allow Geolocation API view their position or users' position, or unidentified user location, or timeout.
options
Being an object of the PositionOptions interface includes the options:
- maximumAge
- timeout
- enableHighAccuracy
** See more the specification of the PositionOptions interface in this document.
clearWatch(id)
The clearWatch(ID) method removes a handler by ID provided by the parameter, handler created before by use by using the watchPosition() method.
4. Position interface
The Position Interface contains the information such as position and time of fetching the position of equipment.
Properties:
position.coords
Return the Coordinates object storing the information on longitude, latitude, altitude of equipment relative to Earth's sea level.
position.timestamp
Returns a DOMTimeStamp representing the time at which the location was retrieved.
5. Coordinates interface
The Coordinates interface represents the position and altitude of the device relative to Earth's sea level, as well as the accuracy with which these properties are calculated.
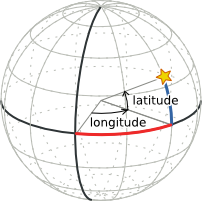
- latitude
Return a number that describes latitude in "decimal degrees" . Its values range [-90,90]
- longitude
Return a number that describes longitude in "decimal degrees". Its values range [-180,180].
- altitude
Returns a number representing the position's altitude in meters, relative to sea level. This value can be null if the implementation cannot provide the data.
- accuracy
Returns a number representing the accuracy of the latitude and longitude properties, expressed in meters.
- altitudeAccuracy
Returns a number representing the accuracy of the altitude expressed in meters. This value can be null.
- heading
Returns a number representing the direction in which the device is traveling. This value, specified in degrees, indicates how far off from heading true north the device is. Value 0 degrees represents true north, and the direction is determined clockwise (which means that east is 90 degrees and west is 270 degrees). If speed is 0, then value of heading is NaN. If the device is unable to provide heading information, this value is null.
- speed
Returns a number representing the velocity of the device in meters per second. This value can be null.
6. PositionError interface
The PositionError interface represents the reason of an error occurring when using the geolocating device.
Value | Constants | Description |
1 | PERMISSION_DENIED | Getting the Geolocation information fails because users do not allow access to their position. |
2 | POSITION_UNAVAILABLE | Getting the Geolocation information fails because an internal error occurs at the geographic information provider side. |
3 | TIMEOUT | The request for getting position information has been sent, and exceeding the allowable time, the results have not yet been received. Therefore, the request is canceled, and is considered as a failure. PositionOptions.timeout allows you to configure maximum time of waiting for a response. |
Properties:
- message
Return a string describing the cause of the error occurring when the application tries to get equipment position information.
ECMAScript, Javascript Tutorials
- Introduction to Javascript and ECMAScript
- Quickstart with Javascript
- Alert, Confirm, Prompt Dialog Box in Javascript
- Quickstart with JavaScript
- JavaScript Variables Tutorial with Examples
- Bitwise Operations
- JavaScript Arrays Tutorial with Examples
- JavaScript Loops Tutorial with Examples
- JavaScript Functions Tutorial with Examples
- JavaScript Number Tutorial with Examples
- JavaScript Boolean Tutorial with Examples
- JavaScript Strings Tutorial with Examples
- JavaScript if else Statement Tutorial with Examples
- JavaScript Switch Statement
- JavaScript Error Handling Tutorial with Examples
- JavaScript Date Tutorial with Examples
- JavaScript Modules Tutorial with Examples
- The History of Modules in JavaScript
- JavaScript setTimeout and setInterval Function
- Javascript Form Validation Tutorial with Examples
- JavaScript Web Cookies Tutorial with Examples
- JavaScript void Keyword Tutorial with Examples
- Classes and Objects in JavaScript
- Class and inheritance simulation techniques in JavaScript
- Inheritance and polymorphism in JavaScript
- Undertanding Duck Typing in JavaScript
- JavaScript Symbols Tutorial with Examples
- JavaScript Set Collection Tutorial with Examples
- JavaScript Map Collection Tutorial with Examples
- Undertanding JavaScript Iterables and Iterators
- JavaScript Regular Expressions Tutorial with Examples
- JavaScript Promise, Async/Await Tutorial with Examples
- Javascript Window Tutorial with Examples
- Javascript Console Tutorial with Examples
- Javascript Screen Tutorial with Examples
- Javascript Navigator Tutorial with Examples
- Javascript Geolocation API Tutorial with Examples
- Javascript Location Tutorial with Examples
- Javascript History API Tutorial with Examples
- Javascript Statusbar Tutorial with Examples
- Javascript Locationbar Tutorial with Examples
- Javascript Scrollbars Tutorial with Examples
- Javascript Menubar Tutorial with Examples
- JavaScript JSON Tutorial with Examples
- JavaScript Event Handling Tutorial with Examples
- Javascript MouseEvent Tutorial with Examples
- Javascript WheelEvent Tutorial with Examples
- Javascript KeyboardEvent Tutorial with Examples
- Javascript FocusEvent Tutorial with Examples
- Javascript InputEvent Tutorial with Examples
- Javascript ChangeEvent Tutorial with Examples
- Javascript DragEvent Tutorial with Examples
- Javascript HashChangeEvent Tutorial with Examples
- Javascript URL Encoding Tutorial with Examples
- Javascript FileReader Tutorial with Examples
- Javascript XMLHttpRequest Tutorial with Examples
- Javascript Fetch API Tutorial with Examples
- Parsing XML in Javascript with DOMParser
- Introduction to Javascript HTML5 Canvas API
- Highlighting code with SyntaxHighlighter Javascript library
- What are polyfills in programming science?
Show More