Quickstart with Javascript
1. The objective of the lesson
Javascript vs ECMAScriptJavascript and ECMAScript are two different names, but they have a very close relationship with each other. Javascript itself is a language developed according to the standards defined by ECMAScript. That's reason why the topics of JavaScript and ECMAScript are put alternately by us.-If you are a beginner with Javascript, I recommend that you read the following post to understand this relationship more:
Javascript is a programming language. Javascript code snippets embedded in HTML help the website be able to interact with users.
The following example is an HTML page with a <img src = "light-off.gif"> tag showing a light that is off. Javascript can change the value of the src (<img src="light-on.gif">) attribute to replace the old image with a new image- the image of the light which is on.
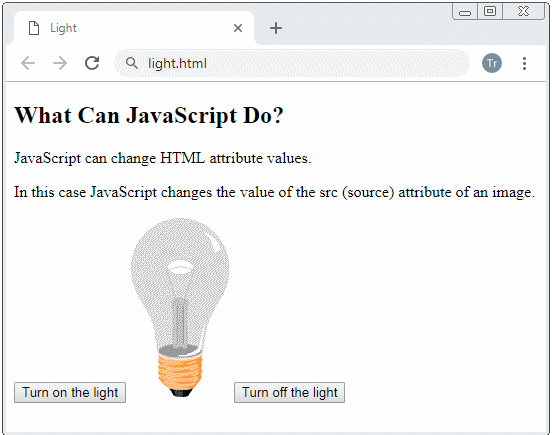
The objective of this lesson is to help you understand how to embed JavaScript into HTML and practise with a few simple examples. All these examples aim at only helping you get familiar with Javascript before going to more detailed topics.
2. Write Javascript in HTML
Javascript code can be written inside HTML, specifically it is written inside the <script></script> tag:
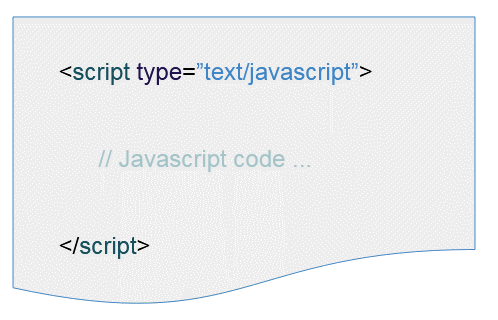
The <script> tags can be placed into the <head> tag or into the <body> tag. First of all, let's look at a simple following example:
Create a helloworld.html file with the content:
helloworld.html
<!DOCTYPE html>
<html>
<head>
<title>Hello Javascript</title>
<script type = "text/javascript">
function sayHello() {
alert("Hello Everyone!");
}
</script>
</head>
<body>
<button onclick="sayHello()">Click me!</button>
</body>
</html>
Run the helloworld.html file on browser and see the result:
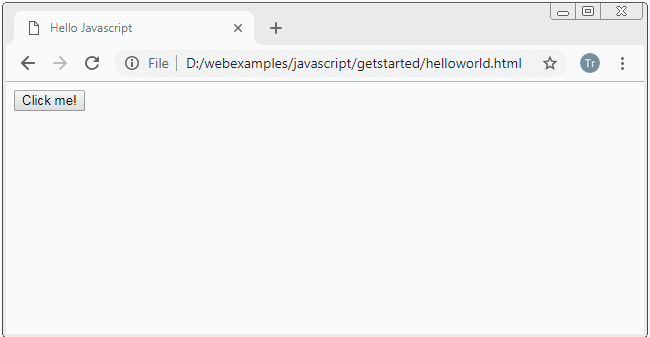
In the above example, in the <script></script> tag, I create the sayHello() function. When the user clicks Button, the sayHello() function is called, and it displays a dialog with "Hello Everyone!" content. Don't worry if you don't know what the function is, temporarily ignore that.
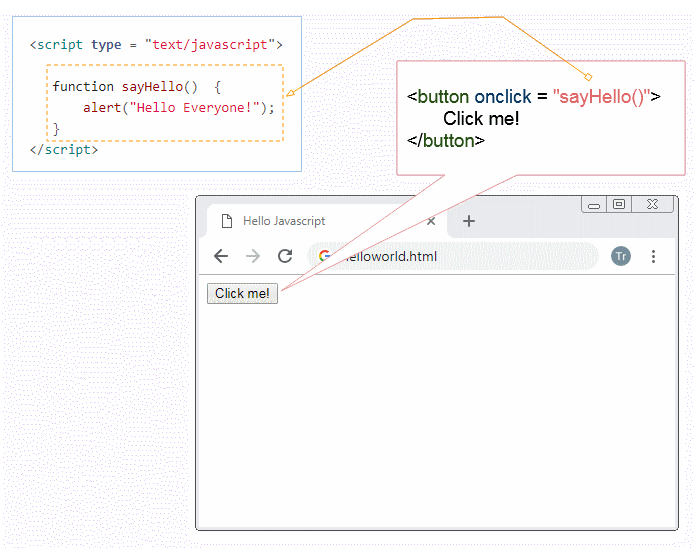
3. Write Javascript in a separate file
Write Javascript code in a separate file, and then HTML will import this file to use. This is the way that helps you manage your Javascript code better, and Javascript file can be shared for many HTML files.
Javascript code is written in a file with extension such as js. The HTML files will import the aforesaid Javascript file to use.
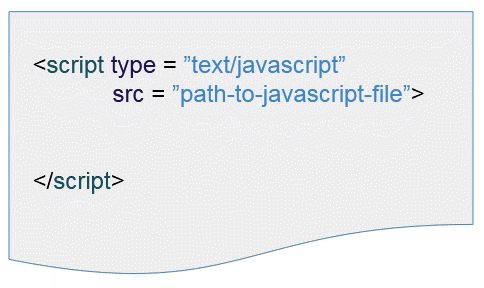
Next is an example with the light. In this example we need 4 files:
- light.js
- light.html
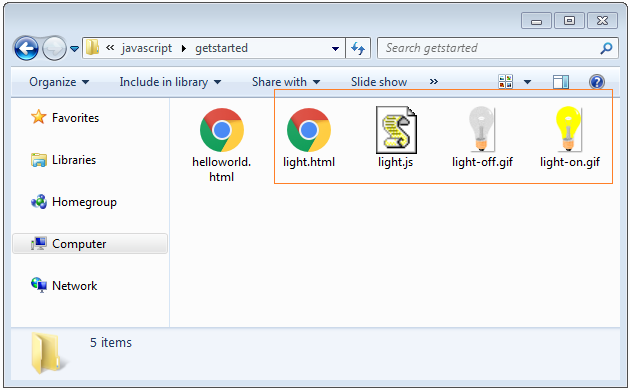
light.js
function turnLightOn() {
var img = document.getElementById("myImage");
img.src = "light-on.gif";
}
function turnLightOff() {
var img = document.getElementById("myImage");
img.src = "light-off.gif";
}
light.html
<!DOCTYPE html>
<html>
<head>
<title>Light</title>
<script type="text/javascript" src="light.js"></script>
</head>
<body>
<h2>What Can JavaScript Do?</h2>
<p>JavaScript can change HTML attribute values.</p>
<p>In this case JavaScript changes the value of the src (source) attribute of an image.</p>
<button onclick="turnLightOn()">Turn on the light</button>
<img id="myImage" src="light-off.gif" style="width:100px">
<button onclick="turnLightOff()">Turn off the light</button>
</body>
</html>
ECMAScript, Javascript Tutorials
- Introduction to Javascript and ECMAScript
- Quickstart with Javascript
- Alert, Confirm, Prompt Dialog Box in Javascript
- Quickstart with JavaScript
- JavaScript Variables Tutorial with Examples
- Bitwise Operations
- JavaScript Arrays Tutorial with Examples
- JavaScript Loops Tutorial with Examples
- JavaScript Functions Tutorial with Examples
- JavaScript Number Tutorial with Examples
- JavaScript Boolean Tutorial with Examples
- JavaScript Strings Tutorial with Examples
- JavaScript if else Statement Tutorial with Examples
- JavaScript Switch Statement
- JavaScript Error Handling Tutorial with Examples
- JavaScript Date Tutorial with Examples
- JavaScript Modules Tutorial with Examples
- The History of Modules in JavaScript
- JavaScript setTimeout and setInterval Function
- Javascript Form Validation Tutorial with Examples
- JavaScript Web Cookies Tutorial with Examples
- JavaScript void Keyword Tutorial with Examples
- Classes and Objects in JavaScript
- Class and inheritance simulation techniques in JavaScript
- Inheritance and polymorphism in JavaScript
- Undertanding Duck Typing in JavaScript
- JavaScript Symbols Tutorial with Examples
- JavaScript Set Collection Tutorial with Examples
- JavaScript Map Collection Tutorial with Examples
- Undertanding JavaScript Iterables and Iterators
- JavaScript Regular Expressions Tutorial with Examples
- JavaScript Promise, Async/Await Tutorial with Examples
- Javascript Window Tutorial with Examples
- Javascript Console Tutorial with Examples
- Javascript Screen Tutorial with Examples
- Javascript Navigator Tutorial with Examples
- Javascript Geolocation API Tutorial with Examples
- Javascript Location Tutorial with Examples
- Javascript History API Tutorial with Examples
- Javascript Statusbar Tutorial with Examples
- Javascript Locationbar Tutorial with Examples
- Javascript Scrollbars Tutorial with Examples
- Javascript Menubar Tutorial with Examples
- JavaScript JSON Tutorial with Examples
- JavaScript Event Handling Tutorial with Examples
- Javascript MouseEvent Tutorial with Examples
- Javascript WheelEvent Tutorial with Examples
- Javascript KeyboardEvent Tutorial with Examples
- Javascript FocusEvent Tutorial with Examples
- Javascript InputEvent Tutorial with Examples
- Javascript ChangeEvent Tutorial with Examples
- Javascript DragEvent Tutorial with Examples
- Javascript HashChangeEvent Tutorial with Examples
- Javascript URL Encoding Tutorial with Examples
- Javascript FileReader Tutorial with Examples
- Javascript XMLHttpRequest Tutorial with Examples
- Javascript Fetch API Tutorial with Examples
- Parsing XML in Javascript with DOMParser
- Introduction to Javascript HTML5 Canvas API
- Highlighting code with SyntaxHighlighter Javascript library
- What are polyfills in programming science?
Show More