Javascript Console Tutorial with Examples
1. Console
The console object provides access to the debugging console of thebrowser (for example, Web Console in Firefox). Console is not useful for end users but useful for programmers. It helps them know how their Javascript codes work in browser, and assists in detection of the location of errors for handling.
Syntax for access to the console object:
window.console
// Or Simple:
console
Open the Console window in the Chrome browser:
/ More Tools / Developer Tools
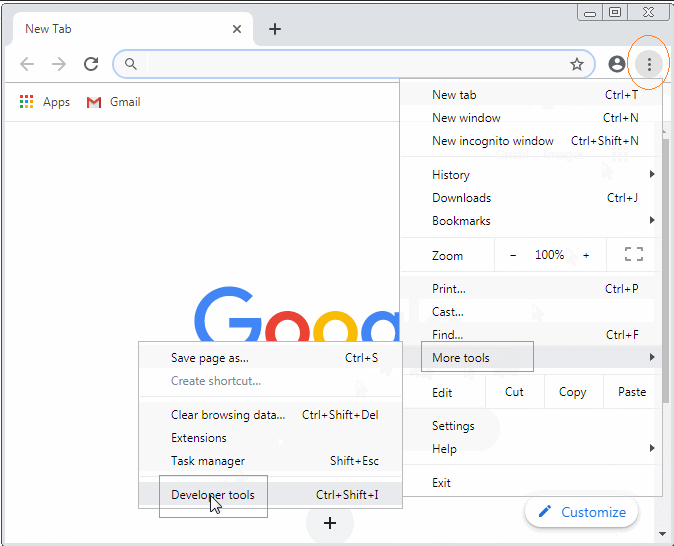
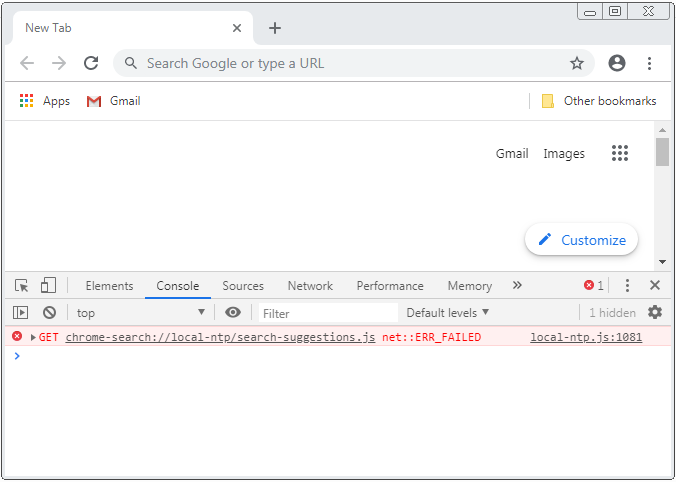
Open the Console window in the Firefox browser:
/ Web Developer / Web Console
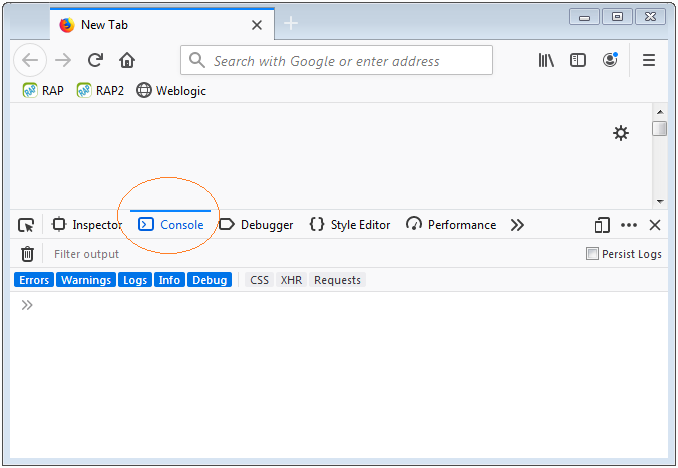
2. Common methods
The console object has quite many methods but its 5 methods used most include:
- console.debug(..)
- console.log(..)
- console.info(..)
- console.warn(..)
- console.error(..)
// (Important!!) Read more notes for debug() method.
console.debug(obj1 [, obj2, ..., objN]);
console.debug(message [, subst1, ..., substN]);
console.log(obj1 [, obj2, ..., objN]);
console.log(message [, subst1, ..., substN]);
console.info(obj1 [, obj2, ..., objN]);
console.info(message [, subst1, ..., substN]);
console.warn(obj1 [, obj2, ..., objN]);
console.warn(message [, subst1, ..., substN]);
console.error(obj1 [, obj2, ..., objN]);
console.error(message [, subst1, ..., substN]);
The above methods log an object (Or many objects), or a String to the console window. The contents printed in the Console window may have different colors and styles depending on the method used.
Parameters:
obj1 ... objN
List of objects to be printed in the Console window.
message
A String to be printed in the Console window.
subst1 ... substN
Objects are used to replace Substitution strings in the message string, which allows you to control output format additions
Substitution string | Description |
%o | Outputs a JavaScript object. Clicking the object name opens more information about it in the inspector. |
%O | Outputs a JavaScript object. Clicking the object name opens more information about it in the inspector. |
%d | Outputs an integer. Number formatting is supported, for example console.log("Foo %.2d", 1.1) will output the number as two significant figures with a leading 0: Foo 01 |
%i | Outputs an integer. Number formatting is supported, for example console.log("Foo %.2d", 1.1) will output the number as two significant figures with a leading 0: Foo 01 |
%s | Outputs a string. |
%f | Outputs a floating-point value. Formatting is supported, for example console.log("Foo %.2f", 1.1) will output the number to 2 decimal places: Foo 1.10 |
console.debug(..)You may see only the contents printed by the console.debug(..) method if your Console is configured Log-Level="debug", by default, Log-Level="log".
For example, use the console.log(), console.info(), console.warn(), console.error() methods to log an object in the Console window.
var myObject = {id : 123, text : 'Some Text'};
console.log(myObject);
console.info(myObject);
console.warn(myObject);
console.error(myObject);
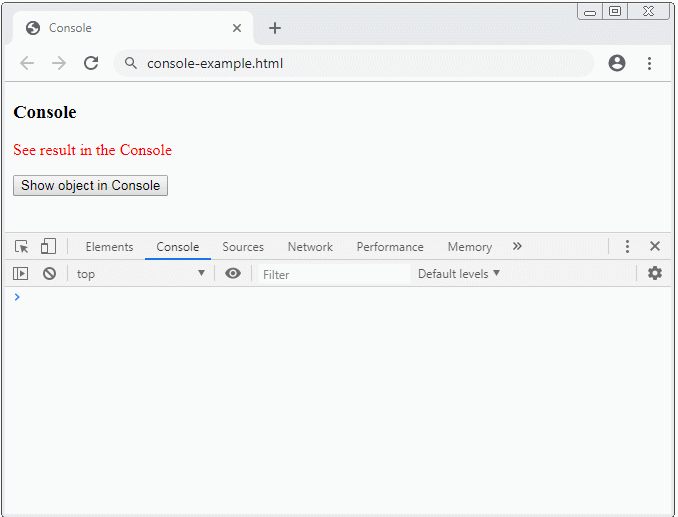
console-example.html
<!DOCTYPE html>
<html>
<head>
<title>Console</title>
<meta charset="UTF-8">
<script>
function test() {
var myObject = {id : 123, text : 'Some Text'};
console.log(myObject);
console.info(myObject);
console.warn(myObject);
console.error(myObject);
}
</script>
</head>
<body>
<h3>Console</h3>
<p style="color:red;">See result in the Console</p>
<button onclick="test()">Show object in Console</button>
</body>
</html>
Example of using console.log(message, obj1, obj2,.. , objN):
var message = "Hello, %s. You've called me %d times.";
console.log(message, "Tom", 5);
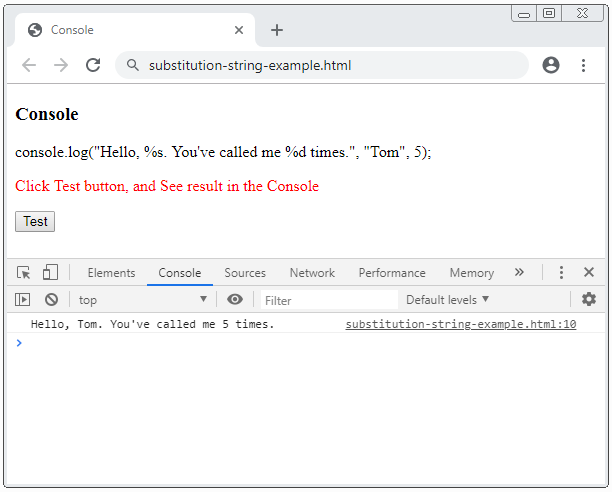
substitution-string-example.html
<!DOCTYPE html>
<html>
<head>
<title>Console</title>
<meta charset="UTF-8">
<script>
function test() {
var message = "Hello, %s. You've called me %d times.";
console.log(message, "Tom", 5);
}
</script>
</head>
<body>
<h3>Console</h3>
<p>console.log("Hello, %s. You've called me %d times.", "Tom", 5);</p>
<p style="color:red;">Click Test button, and See result in the Console</p>
<button onclick="test()">Test</button>
</body>
</html>
3. Customize output style
You may use only %c directive to apply a SS style for the output of Console.
function test() {
var style = "color: yellow; font-style: italic; background-color: blue;padding: 2px";
console.log("This is %cMy stylish message", style);
}
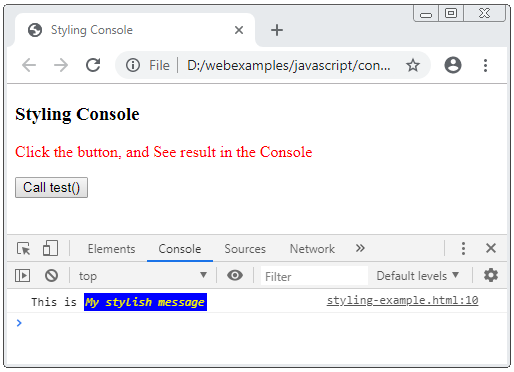
styling-example.html
<!DOCTYPE html>
<html>
<head>
<title>Styling Console</title>
<meta charset="UTF-8">
<script>
function test() {
var style = "color: yellow; font-style: italic; background-color: blue;padding: 2px";
console.log("This is %cMy stylish message", style);
}
</script>
</head>
<body>
<h3>Styling Console</h3>
<p style="color:red;">
Click the button, and See result in the Console
</p>
<button onclick="test()">Call test()</button>
</body>
</html>
4. Console assert(..)
console.assert(assertion, obj1 [, obj2, ..., objN]);
console.assert(assertion, message [, subst1, ..., substN]);
The console.assert(..) method is similar to the console.error(..) method. The different thing is that contents are displayed only in the Console window if the assertion parameter is evaluated to be false.
Parameters:
assertion
If any expression is evaluated to be false, other parameters will be used to display in Console window, like you use the console.error(..) method. On the contrary, if it is evaluated to be true then this method will do nothing.
obj1 ... objN
List of objects to be printed in the Console window.
message
A String to be printed in the Console window.
subst1 ... substN
Objects are used to replace Substitution strings in the message string, which allows you to control output format additions
Example:
const errorMsg = 'the # is not even';
for (let number = 2; number <= 5; number += 1) {
console.log('the # is ' + number);
console.assert(number % 2 === 0, {
number: number,
errorMsg: errorMsg
});
}
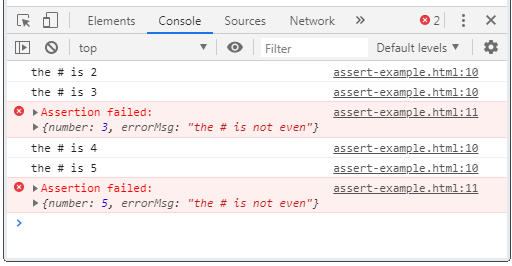
The full Code of the example:
assert-example.html
<!DOCTYPE html>
<html>
<head>
<title>Console assert()</title>
<meta charset="UTF-8">
<script>
function test() {
const errorMsg = 'the # is not even';
for (let number = 2; number <= 5; number += 1) {
console.log('the # is ' + number);
console.assert(number % 2 === 0, {number: number, errorMsg: errorMsg});
}
}
</script>
</head>
<body>
<h3>Console assert()</h3>
<p style="color:red;">Click the button, and See result in the Console</p>
<button onclick="test()">Test</button>
</body>
</html>
5. Console clear()
The console.clear() method is used to clear all contents logged in the console window.
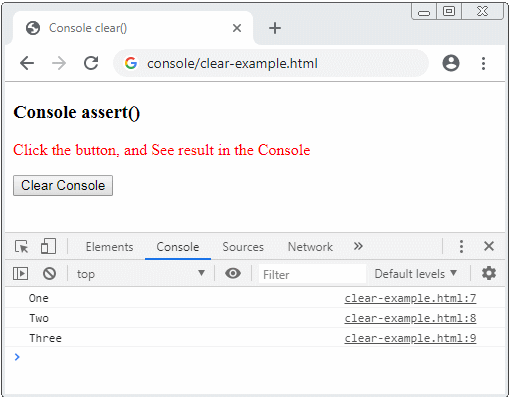
clear-example.html
<!DOCTYPE html>
<html>
<head>
<title>Console clear()</title>
<meta charset="UTF-8">
<script>
console.log("One");
console.log("Two");
console.log("Three");
function clearConsole() {
console.clear();
}
</script>
</head>
<body>
<h3>Console assert()</h3>
<p style="color:red;">Click the button, and See result in the Console</p>
<button onclick="clearConsole()">Clear Console</button>
</body>
</html>
6. Console Group
Sometimes, the content printed on the Console window is quite difficult to see, therefore, you want them to display in blocks in an organized way. There are some methods that help you do that.
- console.group()
- console.groupCollapsed()
- console.groupEnd()
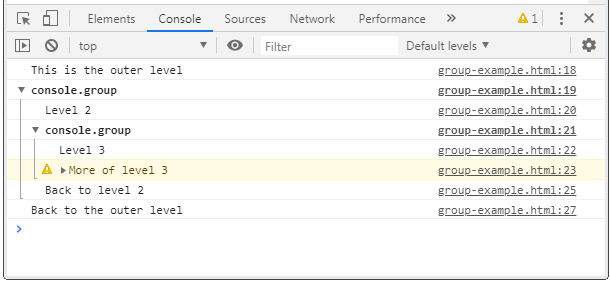
The console.group() method helps you create a block in the Console window. You can create nested blocks and use console.groupEnd() to exit the current block to return to the parent block.
The console.groupCollapsed() method is also for creating a block but this block will be in the Collapsed status while the console.group() method creates a block in expanded status.
See a simple example:
console.log("This is the outer level");
console.group();
console.log("Level 2");
console.group();
console.log("Level 3");
console.warn("More of level 3");
console.groupEnd();
console.log("Back to level 2");
console.groupEnd();
console.log("Back to the outer level");
The result seen by you in the Console window (of the Chrome browser):
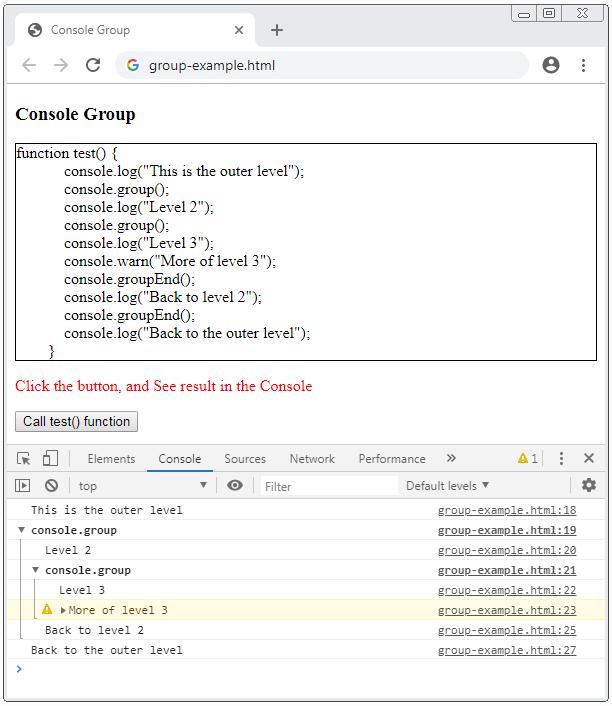
The full Code of the example:
group-example.html
<!DOCTYPE html>
<html>
<head>
<title>Console Group</title>
<meta charset="UTF-8">
<style>
#div-code {
border: 1px solid;
padding: 5px 5px 5px -15px;
display: block;
unicode-bidi: embed;
white-space: pre;
}
</style>
<script>
function test() {
console.log("This is the outer level");
console.group();
console.log("Level 2");
console.group();
console.log("Level 3");
console.warn("More of level 3");
console.groupEnd();
console.log("Back to level 2");
console.groupEnd();
console.log("Back to the outer level");
}
function showCode_of_test_function() {
document.getElementById("div-code").innerHTML = test.toString();
}
</script>
</head>
<body onload="showCode_of_test_function()">
<h3>Console Group</h3>
<div id="div-code"></div>
<p style="color:red;">Click the button, and See result in the Console</p>
<button onclick="test()">Call test() function</button>
</body>
</html>
7. Console Timer
Sometimes, you want to check how long a code snippet finishes being executed. There are some methods of Console that help you to do this.
- console.time(label)
- console.timeEnd(label)
- console.timeLog(label)
console.time(label)
The console.time(label) method starts a Timer in Console. The label parameters can be considered as the name of timer. It helps you to distinguish the active timer in the Console.
console.timeEnd(label)
The console.timeEnd(label) method is used to end a timer and display the result in the Console window.
console.timeLog(label)
The console.timeLog(label) method log ethe current value of Timer created previously with the console.time(label) method by you.
Example: use the Console Timer to evaluate user's response time.
console.time("Answer time");
alert("Click to continue");
console.timeLog("Answer time");
alert("Do a bunch of other stuff...");
console.timeEnd("Answer time");
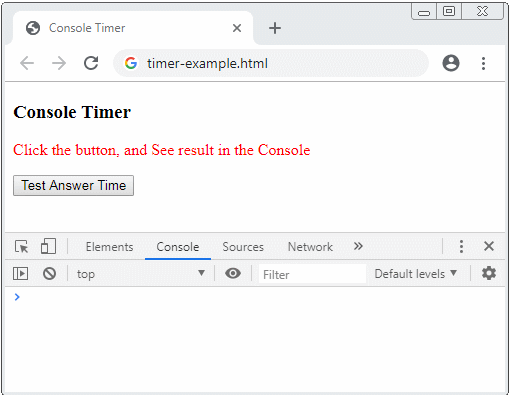
See the full code of the example:
timer-example.html
<!DOCTYPE html>
<html>
<head>
<title>Console Timer</title>
<meta charset="UTF-8">
<script>
function testAnswerTime() {
console.time("Answer time");
alert("Click to continue");
console.timeLog("Answer time");
alert("Do a bunch of other stuff...");
console.timeEnd("Answer time");
}
</script>
</head>
<body>
<h3>Console Timer</h3>
<p style="color:red;">Click the button, and See result in the Console</p>
<button onclick="testAnswerTime()">Test Answer Time</button>
</body>
</html>
8. Console Stack Trace
Sometimes, you want to log all functions having been called before meeting the function containing the console.trace() code line. This helps you know the order of functions called in the program.
For simplification, let's see an example:
function donald() {
}
function tom() {
jerry();
}
function jerry() {
donald();
console.trace();
}
// Start Here!!
function testTrace() {
tom();
}
Below is the illustration of order of functions called until the console.trace() is encountered.
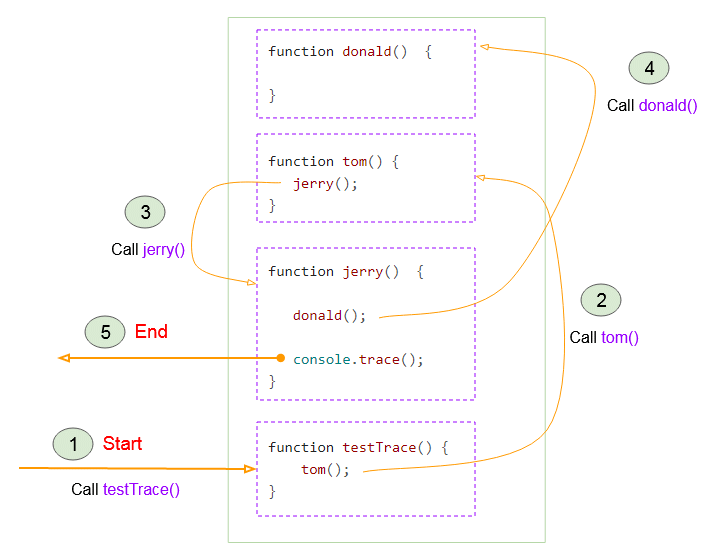
The result is received by you when running the example:
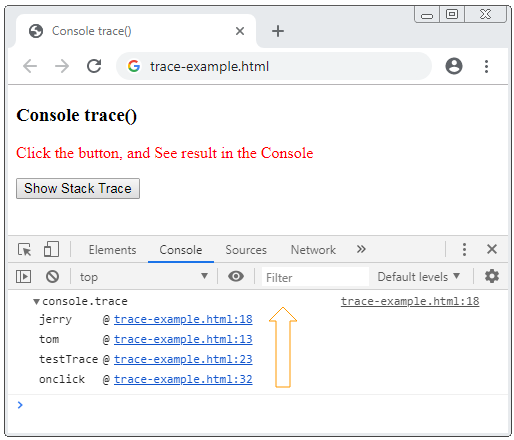
See the full code of the example:
trace-example.html
<!DOCTYPE html>
<html>
<head>
<title>Console trace()</title>
<meta charset="UTF-8">
<script>
function donald() {
}
function tom() {
jerry();
}
function jerry() {
donald();
console.trace();
}
// Start Here!!
function testTrace() {
tom();
}
</script>
</head>
<body>
<h3>Console trace()</h3>
<p style="color:red;">Click the button, and See result in the Console</p>
<button onclick="testTrace()">Show Stack Trace</button>
</body>
</html>
You can call console.trace() at many positions in the program. See the example:
trace-example2
function donald() {
console.trace();
}
function tom() {
jerry();
}
function jerry() {
console.trace();
donald();
}
// Start Here!!
function testTrace() {
tom();
}
And the result received:
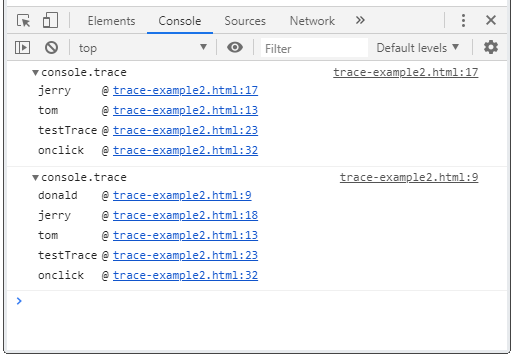
trace-example2.html
<!DOCTYPE html>
<html>
<head>
<title>Console trace()</title>
<meta charset="UTF-8">
<script>
function donald() {
console.trace();
}
function tom() {
jerry();
}
function jerry() {
console.trace();
donald();
}
// Start Here!!
function testTrace() {
tom();
}
</script>
</head>
<body>
<h3>Console trace()</h3>
<p style="color:red;">Click the button, and See result in the Console</p>
<button onclick="testTrace()">Show Stack Trace</button>
</body>
</html>
9. Console Table
console.table(data [,columns])
The console.table(data[,columns]) method displays a data in a table format.
data - Object:
If data parameter is an object, the table will consist of 2 columns. The first column (index column) contains properties and the second column (value column) contains property values .
var person = {
firstName: 'John',
lastName: 'Smith',
age: 40
}
function test() {
console.table(person);
}
The result received by you:
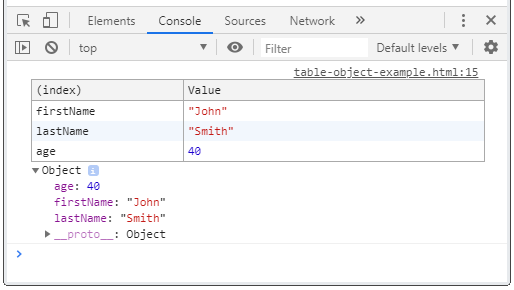
data - Array of primitives
If data parameter is an array of primitive values, the table will include 2 columns. The first column (index column) contains the array indexes. The second column (value column) contains the array elements.
var flowers = ["Lily", "Tulip", "Freesia"];
function test() {
console.table(flowers);
}
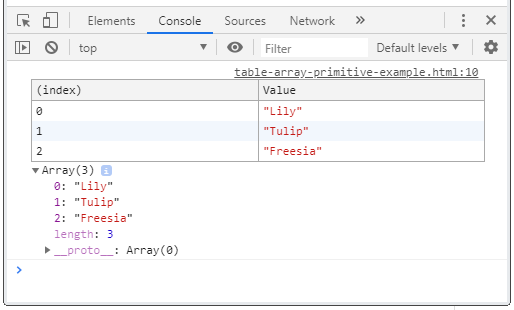
data - Array of Objects
If the data parameter is an array of objects. The will be at least two columns in the table. The first column (index column) contains array indexes.
var person = {
firstName: 'John',
lastName: 'Smith'
};
var array = ["Lily", "Tulip", person];
function test() {
console.table(array);
}
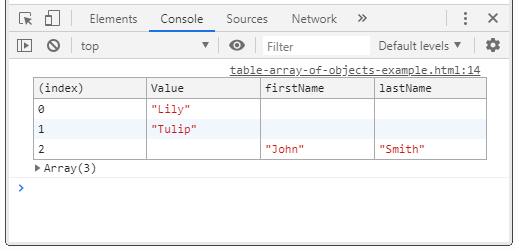
data - Array of arrays
If the data parameter is an array of arrays, there will be at least 2 columns in the table. The first column (index column) contains array indexes.
var array = [
["Value-0-1", "Value-0-2"],
["Value-1-1", "Value-1-2", "Value-1-3"],
["Value-2-1", "Value-2-2"],
];
function test() {
console.table(array);
}
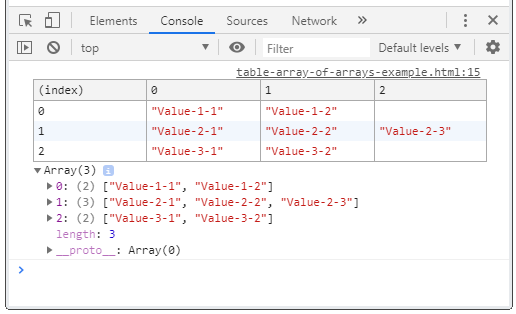
data, columns
Use the columns parameter to specify the columns to be included in the table.
var john = {firstName: "John", lastName: "Smith"};
var jane = {firstName: "Jane", lastName: "Doe"};
var array = [john, jane];
function test() {
var columns = ["firstName"];
// All columns
console.table(array);
// Custome Columns
console.table(array, columns);
}
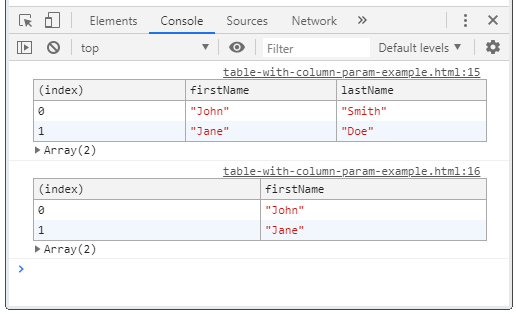
10. Console count(), countReset()
count([label])
Log times of calling the console.count([label]) method,
console.count( [label] );
Example:
function greet(userName) {
console.count(userName);
if (userName != null) {
return "Hi " + userName;
}
return "Hi!";
}
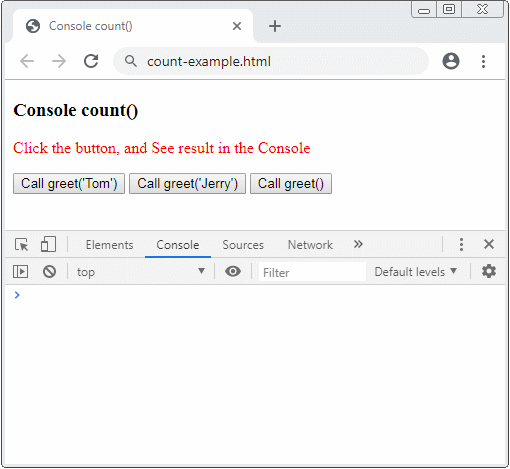
count-example.html
<!DOCTYPE html>
<html>
<head>
<title>Console count()</title>
<meta charset="UTF-8">
<script>
function greet(userName) {
console.count(userName);
if(userName != null) {
return "Hi " + userName;
}
return "Hi!";
}
</script>
</head>
<body>
<h3>Console count()</h3>
<p style="color:red;">
Click the button, and See result in the Console
</p>
<button onclick="greet('Tom')">Call greet('Tom')</button>
<button onclick="greet('Jerry')">Call greet('Jerry')</button>
<button onclick="greet()">Call greet()</button>
</body>
</html>
console.countReset([label])
The console.countReset([label]) method reset the counter of console.count([label]) to 0.
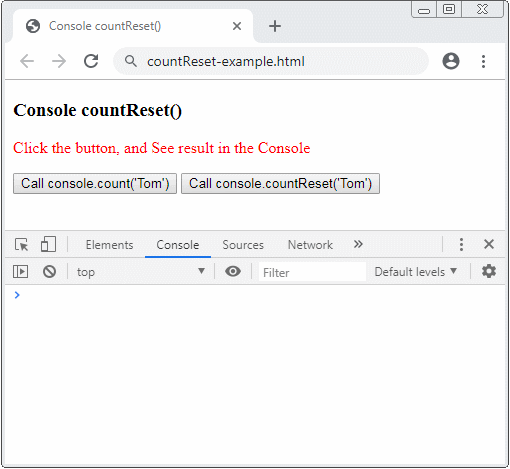
countReset-example.html
<!DOCTYPE html>
<html>
<head>
<title>Console countReset()</title>
<meta charset="UTF-8">
</head>
<body>
<h3>Console countReset()</h3>
<p style="color:red;">
Click the button, and See result in the Console
</p>
<button onclick="console.count('Tom')">Call console.count('Tom')</button>
<button onclick="console.countReset('Tom')">Call console.countReset('Tom')</button>
</body>
</html>
ECMAScript, Javascript Tutorials
- Introduction to Javascript and ECMAScript
- Quickstart with Javascript
- Alert, Confirm, Prompt Dialog Box in Javascript
- Quickstart with JavaScript
- JavaScript Variables Tutorial with Examples
- Bitwise Operations
- JavaScript Arrays Tutorial with Examples
- JavaScript Loops Tutorial with Examples
- JavaScript Functions Tutorial with Examples
- JavaScript Number Tutorial with Examples
- JavaScript Boolean Tutorial with Examples
- JavaScript Strings Tutorial with Examples
- JavaScript if else Statement Tutorial with Examples
- JavaScript Switch Statement
- JavaScript Error Handling Tutorial with Examples
- JavaScript Date Tutorial with Examples
- JavaScript Modules Tutorial with Examples
- The History of Modules in JavaScript
- JavaScript setTimeout and setInterval Function
- Javascript Form Validation Tutorial with Examples
- JavaScript Web Cookies Tutorial with Examples
- JavaScript void Keyword Tutorial with Examples
- Classes and Objects in JavaScript
- Class and inheritance simulation techniques in JavaScript
- Inheritance and polymorphism in JavaScript
- Undertanding Duck Typing in JavaScript
- JavaScript Symbols Tutorial with Examples
- JavaScript Set Collection Tutorial with Examples
- JavaScript Map Collection Tutorial with Examples
- Undertanding JavaScript Iterables and Iterators
- JavaScript Regular Expressions Tutorial with Examples
- JavaScript Promise, Async/Await Tutorial with Examples
- Javascript Window Tutorial with Examples
- Javascript Console Tutorial with Examples
- Javascript Screen Tutorial with Examples
- Javascript Navigator Tutorial with Examples
- Javascript Geolocation API Tutorial with Examples
- Javascript Location Tutorial with Examples
- Javascript History API Tutorial with Examples
- Javascript Statusbar Tutorial with Examples
- Javascript Locationbar Tutorial with Examples
- Javascript Scrollbars Tutorial with Examples
- Javascript Menubar Tutorial with Examples
- JavaScript JSON Tutorial with Examples
- JavaScript Event Handling Tutorial with Examples
- Javascript MouseEvent Tutorial with Examples
- Javascript WheelEvent Tutorial with Examples
- Javascript KeyboardEvent Tutorial with Examples
- Javascript FocusEvent Tutorial with Examples
- Javascript InputEvent Tutorial with Examples
- Javascript ChangeEvent Tutorial with Examples
- Javascript DragEvent Tutorial with Examples
- Javascript HashChangeEvent Tutorial with Examples
- Javascript URL Encoding Tutorial with Examples
- Javascript FileReader Tutorial with Examples
- Javascript XMLHttpRequest Tutorial with Examples
- Javascript Fetch API Tutorial with Examples
- Parsing XML in Javascript with DOMParser
- Introduction to Javascript HTML5 Canvas API
- Highlighting code with SyntaxHighlighter Javascript library
- What are polyfills in programming science?
Show More