Javascript Location Tutorial with Examples
1. window.location
window.location returns a Location object with the information about URL of current document object.
window.location (As a String)
Note: Javascript 1.0 designs window.location as a String. Next Javascript versions design it as an object. The legacy from version 1.0 is still accepted by all browsers, therefore, you can assign a URL String value to window.location to change the URL of the document object. However, I recommend that you assign the URL String to window.location.href instead of window.location.
legacy-location-example.html
<!DOCTYPE html>
<html>
<head>
<title>window.location Example</title>
<meta charset="UTF-8">
</head>
<body>
<h1>window.location (Legacy)</h1>
<p style="color:red;">
Note: Javascript 1.0, window.location as a String,
NOW, It is still accepted by all browsers
</p>
<button onclick="alert(window.location)">alert(window.location)</button>
<br><br>
<button onclick="window.location='https://google.com'">
Set window.location='https://google.com';
</button>
</body>
</html>
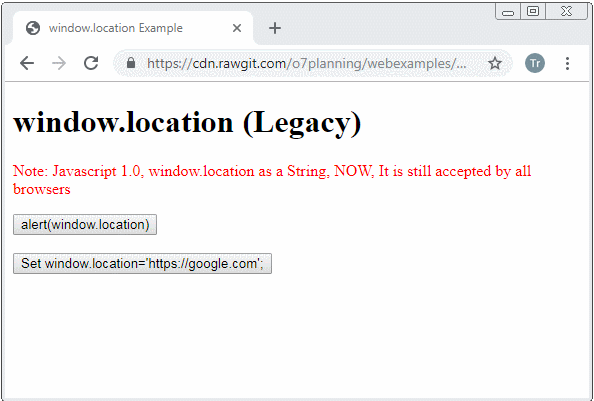
window.location (As a Object)
window.location is recommended to be used as an object, it has useful properties and methods.
2. Properties
The Location object includes properties:
- href
- protocol
- host
- hostname
- port
- pathname
- search
- hash
- username
- password
- origin (Read only)
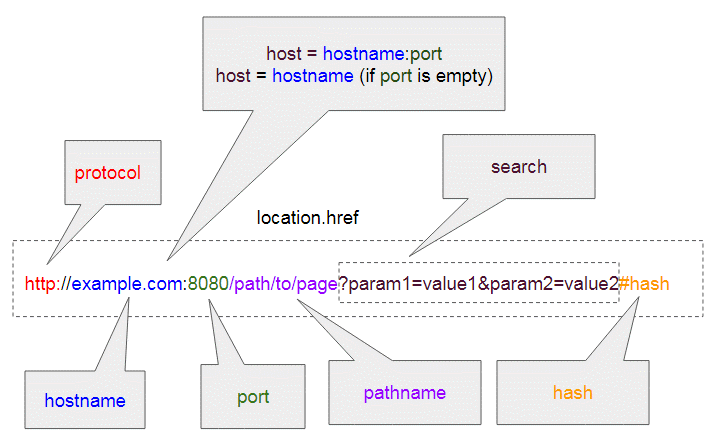
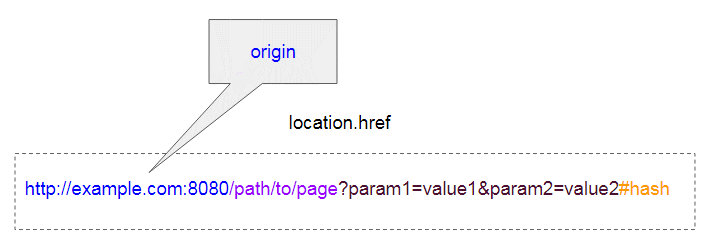
Property | Description |
href | A String, which is the URL of document. |
protocol | A String, which is the protocol scheme of URL. The character ( : ) at the end is included. |
hostname | A String, which is the domain name of URL. |
port | A String, which is the port of URL. It may be empty. |
host | A String, which is hostname:port or hostname if port is empty. |
pathname | A String, which is a path to the file name of URL, including the ( / ) character at the beginning of a string. |
search | A String, which is the "querystring" of URL, including the ( ? ) character at the beginning of a string. |
hash | A String, also known as hash string, including the ( # ) character at the beginning of a string. |
username | A String, in which user name is specified in front of domain name. |
password | A String, in which the password of the user is specified in front of domain name. |
origin (Read only) | A String, which is protocol//hostname:port or protocal//hostname if port is empty. |
Example:
- http://example.com:8080/path/to/page?param1=value1¶m2=value2#hash
Property | Value |
protocol | http: |
hostname | example.com |
port | 8080 |
host | example.com:8080 |
pathname | /path/to/page |
search | ?param1=value1¶m2=value2 |
hash | #hash |
origin | http://example.com:8080 |
location-example.html
<!DOCTYPE html>
<html>
<head>
<title>Location Example</title>
<meta charset="UTF-8">
<style>textarea {width:100%;margin-top:10px;}</style>
</head>
<body>
<h1>window.location</h1>
<button onClick="showInfos()">Show Infos</button>
<br>
<textarea name="name" rows="15" id="log-area"></textarea>
<script>
function showInfos() {
var logArea = document.getElementById("log-area");
logArea.value = "";
logArea.value += "location.href= " + location.href +"\n";
logArea.value += "location.protocol= " + location.protocol +"\n";
logArea.value += "location.host= " + location.host +"\n";
logArea.value += "location.hostname= " + location.hostname +"\n";
logArea.value += "location.port= " + location.port +"\n";
logArea.value += "location.pathname= " + location.pathname +"\n";
logArea.value += "location.search= " + location.search +"\n";
logArea.value += "location.hash= " + location.hash +"\n";
logArea.value += "location.username= " + location.username +"\n";
logArea.value += "location.password= " + location.password +"\n";
logArea.value += "location.origin= " + location.origin +"\n";
}
showInfos();
</script>
</body>
</html>
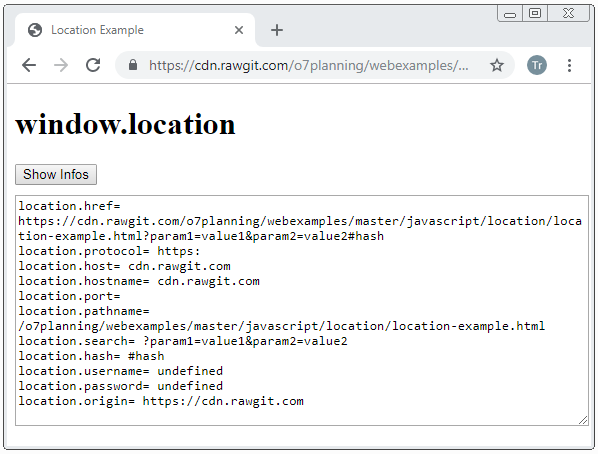
For example, get the current URL of page or set a new URL for current page.
location-href-example.html
<!DOCTYPE html>
<html>
<head>
<title>window.location Example</title>
<meta charset="UTF-8">
</head>
<body>
<h1>window.location.href</h1>
<button onclick="alert(window.location.href)">alert(window.location.href)</button>
<br><br>
<button onclick="window.location.href='https://google.com'">
Set window.location.href='https://google.com';
</button>
</body>
</html>
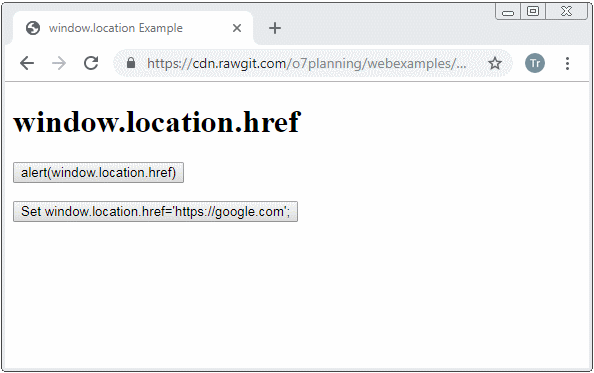
3. Methods
The Location object includes methods:
- assign(url)
- replace(url)
- reload(forcedReload)
- toString()
location.assign(url)
Load page with the url provided by the parameter. This method creates a new history record, which means that you can use the Go-Back function to come back to the previous page.
location-assign-example.html
<!DOCTYPE html>
<html>
<head>
<title>window.location Example</title>
<meta charset="UTF-8">
</head>
<body>
<h1>window.location.assign(url)</h1>
<button onclick="location.assign('https://google.com')">
location.assign('https://google.com')
</button>
</body>
</html>
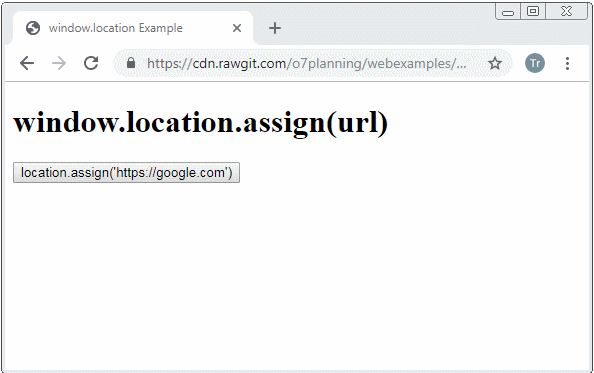
location.replace(url)
Load a page with the url provided by the method parameter. This method replaces current history record with a new history record, i.e. you can not use the Go-Back function to returt to the previous page.
location-replace-example.html
<!DOCTYPE html>
<html>
<head>
<title>window.location Example</title>
<meta charset="UTF-8">
</head>
<body>
<h1>window.location.replace(url)</h1>
<button onclick="location.replace('https://google.com')">
location.replace('https://google.com')
</button>
</body>
</html>
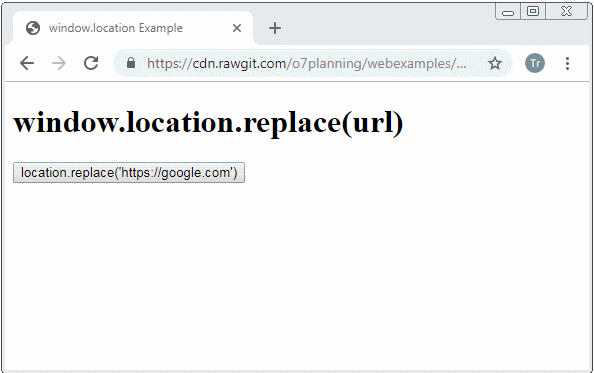
location.reload(forcedReload)
The location.reload(forcedReload) method is used to reload current page. It is like you click on Refresh. The forcedReload optional parameter has two true/false values. If forcedReload = true, that means the content of page will be loaded from a server, vice versa, if forcedReload = false, the page contents can be taken from cache if the browser finds that it is not necessary to get from the server. The default value is false.
After calling the location.reload(forcedReload) method, the scroll position of scrollbars may be changed depending on forcedReload. Specifically, if forceReload = false the scroll position doesn't change. For some browsers, if forcedReload = true, the scroll position will return to 0 (window.scrollY = 0).
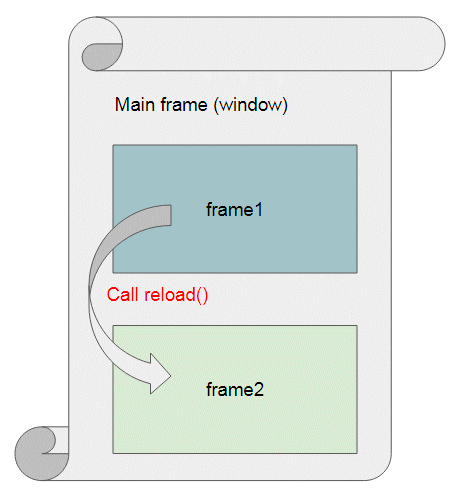
You can call the reload() method from a Frame to reload the contents of another Frame , but it can be blocked and you will receive an error if such two Frames have different origin. Learn more about Same-Origin Policy:
Example of using the location.reload() method:
location-reload-example.html
<!DOCTYPE html>
<html>
<head>
<title>window.location Example</title>
<meta charset="UTF-8">
<script>
function showCurrentTime() {
document.getElementById("showtime").innerHTML ="Now is " + new Date();
}
</script>
</head>
<body onload="showCurrentTime()">
<h2>window.location.reload(true/false)</h2>
<p id="showtime"></p>
<button onclick="location.reload(true)">
location.reload(true)
</button>
</body>
</html>
location.toString()
This method returns the URL of document. It exactly returns window.location.href.
4. Frames
A page can contain Frames, and a Frame can contain other Fames. They form a hierarchy of Frames.
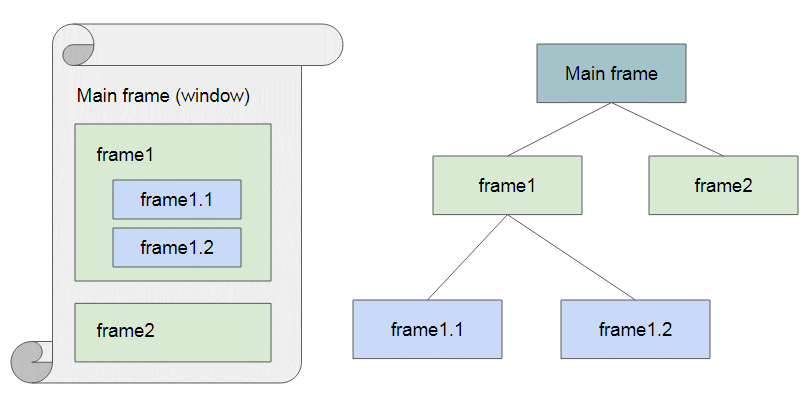
Property | Read Only | Description |
window.name | Gets/sets the name of the window. | |
window.frameElement | Y | Returns the element in which the window is embedded, or null if the window is not embedded. |
window.frames | Y | Returns an array of the frames in the current window. |
window.length | Y | Returns the number of frames in the window. See also window.frames. |
window.parent | Y | Returns a reference to the parent of the current window or subframe. |
window.self | Y | Returns an object reference to the window object itself. |
window.opener | Y | Returns a reference to the window that opened this current window. |
window[0],
window[1], etc. | Y | Returns a reference to the window object in the frames. See window.frames for more details. |
window.top | Y | Returns a reference to the topmost window in the window hierarchy. This property is read only. |
For example, use Javascript to change the URL of a Frame form other Frame.
frame-main.html
<!DOCTYPE html>
<html>
<head>
<title>Location example</title>
<meta charset="UTF-8">
<style>iframe {height:155px; width:100%}</style>
</head>
<body>
<h1 id="my-h1">Main Frame</h1>
<p>Frame 1</p>
<iframe src="frame-a.html"></iframe>
<p>Frame 2</p>
<iframe src="frame-b.html"></iframe>
</body>
</html>
frame-a.html
<!DOCTYPE html>
<html>
<head>
<title>frame-a.html</title>
<meta charset="UTF-8">
<script>
function changeSrcOfFrame2(newUrl) {
var frame2 = window.parent.frames[1];
frame2.location.href = newUrl;
}
</script>
</head>
<body>
<h2>frame-a.html</h2>
<button onclick="changeSrcOfFrame2('frame-c.html')">
Change Location of Frame 2 --> frame-c.html
</button>
<br/><br/>
<button onclick="changeSrcOfFrame2('frame-b.html')">
Change Location of Frame 2 --> frame-b.html
</button>
</body>
</html>
frame-b.html
<!DOCTYPE html>
<html>
<head>
<title>frame-b.html</title>
<meta charset="UTF-8">
<script>
function showCurrentTime() {
document.getElementById("curr-date").innerHTML= new Date();
}
</script>
</head>
<body onload="showCurrentTime()">
<h2 id="my-h2" style="color:blue;">frame-b.html</h2>
<p id="curr-date">...</p>
</body>
</html>
frame-c.html
<!DOCTYPE html>
<html>
<head>
<title>frame-c.html</title>
<meta charset="UTF-8">
<script>
function showCurrentTime() {
document.getElementById("curr-date").innerHTML= new Date();
}
</script>
</head>
<body onload="showCurrentTime()">
<h2 id="my-h2" style="color:red;">frame-c.html</h2>
<p id="curr-date">...</p>
</body>
</html>
ECMAScript, Javascript Tutorials
- Introduction to Javascript and ECMAScript
- Quickstart with Javascript
- Alert, Confirm, Prompt Dialog Box in Javascript
- Quickstart with JavaScript
- JavaScript Variables Tutorial with Examples
- Bitwise Operations
- JavaScript Arrays Tutorial with Examples
- JavaScript Loops Tutorial with Examples
- JavaScript Functions Tutorial with Examples
- JavaScript Number Tutorial with Examples
- JavaScript Boolean Tutorial with Examples
- JavaScript Strings Tutorial with Examples
- JavaScript if else Statement Tutorial with Examples
- JavaScript Switch Statement
- JavaScript Error Handling Tutorial with Examples
- JavaScript Date Tutorial with Examples
- JavaScript Modules Tutorial with Examples
- The History of Modules in JavaScript
- JavaScript setTimeout and setInterval Function
- Javascript Form Validation Tutorial with Examples
- JavaScript Web Cookies Tutorial with Examples
- JavaScript void Keyword Tutorial with Examples
- Classes and Objects in JavaScript
- Class and inheritance simulation techniques in JavaScript
- Inheritance and polymorphism in JavaScript
- Undertanding Duck Typing in JavaScript
- JavaScript Symbols Tutorial with Examples
- JavaScript Set Collection Tutorial with Examples
- JavaScript Map Collection Tutorial with Examples
- Undertanding JavaScript Iterables and Iterators
- JavaScript Regular Expressions Tutorial with Examples
- JavaScript Promise, Async/Await Tutorial with Examples
- Javascript Window Tutorial with Examples
- Javascript Console Tutorial with Examples
- Javascript Screen Tutorial with Examples
- Javascript Navigator Tutorial with Examples
- Javascript Geolocation API Tutorial with Examples
- Javascript Location Tutorial with Examples
- Javascript History API Tutorial with Examples
- Javascript Statusbar Tutorial with Examples
- Javascript Locationbar Tutorial with Examples
- Javascript Scrollbars Tutorial with Examples
- Javascript Menubar Tutorial with Examples
- JavaScript JSON Tutorial with Examples
- JavaScript Event Handling Tutorial with Examples
- Javascript MouseEvent Tutorial with Examples
- Javascript WheelEvent Tutorial with Examples
- Javascript KeyboardEvent Tutorial with Examples
- Javascript FocusEvent Tutorial with Examples
- Javascript InputEvent Tutorial with Examples
- Javascript ChangeEvent Tutorial with Examples
- Javascript DragEvent Tutorial with Examples
- Javascript HashChangeEvent Tutorial with Examples
- Javascript URL Encoding Tutorial with Examples
- Javascript FileReader Tutorial with Examples
- Javascript XMLHttpRequest Tutorial with Examples
- Javascript Fetch API Tutorial with Examples
- Parsing XML in Javascript with DOMParser
- Introduction to Javascript HTML5 Canvas API
- Highlighting code with SyntaxHighlighter Javascript library
- What are polyfills in programming science?
Show More