Javascript History API Tutorial with Examples
1. window.history
Each Tab or Frame has its own history object, and each history object contains a reference to a special object called "Joint Session History". This special object manages all browser history objects.
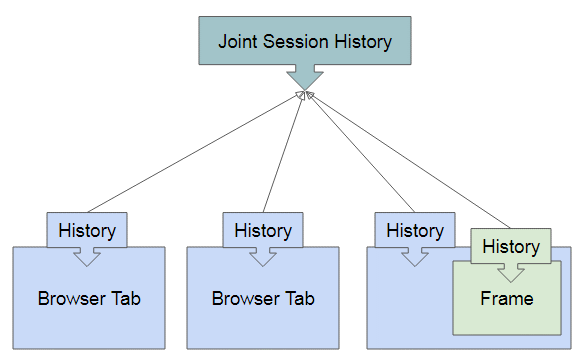
Use the window.history syntax (or simply, history) to access the history of the current Tab or Frame.
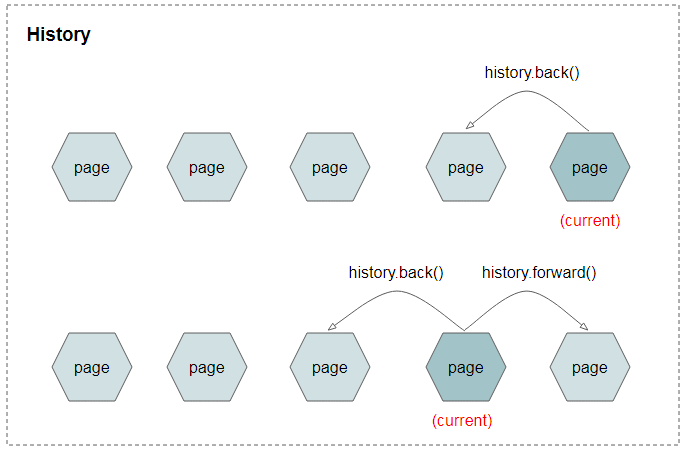
How the list of pages in History will change if you stand on a page in history and go to another page that does not belong to history.
Note: Going to another page that does not belong to history means that you don't use BACK, FORWARD button and call one of the methods: history.back(), history.forward(), history.go(). You can go to a new page that doesn't belong to history by clicking a link with a link with URL different from current URL or enter a new URL and click on Enter,..
Before going to a new page:
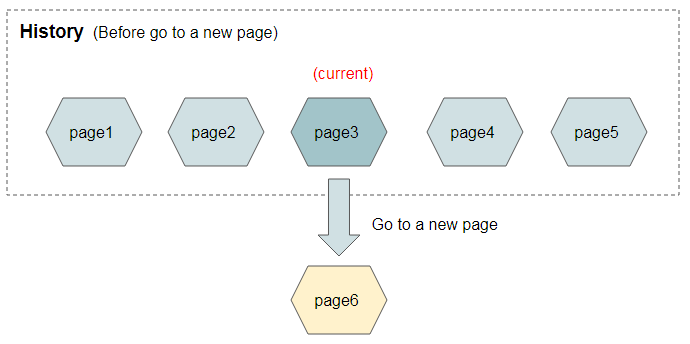
After going to a new page:
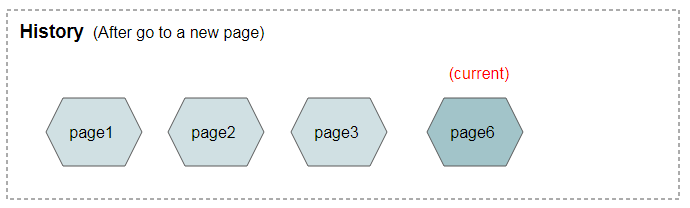
2. Properties
history.length
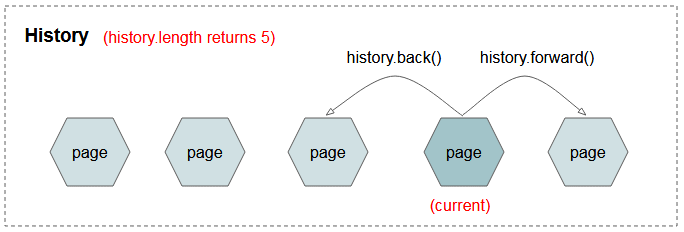
Returns the number of entities being managed in the history object of the current Tab (or Frame).
history.scrollRestoration
When you call one of back(), forward(), go() functions or use the BACK, FORWARD buttons to go to a history page. After the history page is displayed, the browser tries to restore the scroll position of scrollbars for this page. Restoring way depends on the history.scrollRestoration value.
history.scrollRestoration has the 2 values - "auto" or "manual". By default, "auto".
history.scrollRestoration = "auto"
Browser will do the duty of restoring the scroll position of scrollbar.
history.scrollRestoration = "manual"
You have to restore the position of scrollbars by yourself. To do this, the scroll position information of the scroll bar needs to be stored in each History entity. See more history.pushState (..) method.
The following Video shows you difference of "auto" and "manual" values:
3. Methods
The methods of history:
- go([delta])
- back()
- forward()
- pushState(data,title[,url])
- replaceState(data,title[,url])
history.go([delta])
Back or forward delta steps in History. If delta = 0 or is not provided, the browser will reload current page. If delta is located outside allowable values, the browser does nothing.
history.back()
Back a step in History. It is equivalent to calling the history.go(-1) method. If there is no previous page in History, the browser does nothing.
history.forward()
Forward a step in History. It is equivalent to calling the history.go(1) method. If there is no next page in History, the browser does nothing.
pushState(data,title[,url])
Create an entity (a page) in history.
replaceState(data,title[,url])
Update current entity in history.
4. history.pushState()
Using hash, you can create entities in history. This way helps you to obtain entities in history when the browser doesn't need to reload page.
Let's see an example of history with hash:
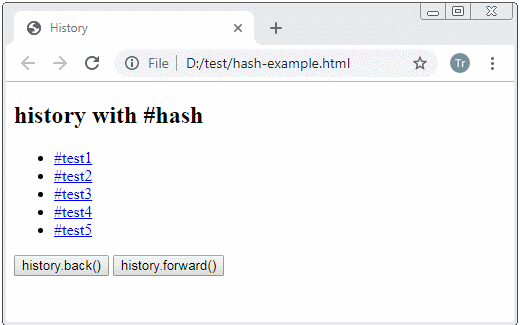
history.pushState(dataState, title [,url])
The history.pushState(..) method allows you to create an entity in history (URL doesn't probably need chagning), This is useful in Single Page applications.
When a user goes to an entity (a page) in history and this entity is created by using the history.pushState() method, it will create the popstate event.
Parameters:
dataState
An object stores the state of an entity. There are a lot of things you can store in this object, such as the scroll position of scrollbars. This helps you to restore the scrollbar position manually, ...
title
The title parameter means a suggestion. Browsers do not usually use this parameter to establish the title of document.
url
The url parameter is not mandatory. If provided, it must have the same origin as that of current URL of page.
Example:
pushState-example.js
function showHistory() {
console.log(window.history);
var log = document.getElementById("log-area");
log.value="";
log.value +="history.length="+ history.length +"\n";
log.value +="history.scrollRestoration="+history.scrollRestoration+"\n";
log.value +="history.state="+ JSON.stringify(history.state)+"\n";
}
function popstateHandler(popstateEvent) {
console.log(popstateEvent );
document.title = popstateEvent.state.title;
showHistory();
}
// popstate event handler:
window.onpopstate = popstateHandler;
var number = 0;
function call_pushState() {
number = number + 1;
var title = "State "+ number;
var dataState = {
empId : number,
showProfile: true,
title: title
};
window.history.pushState(dataState, title);
document.title = title;
// Show current History:
showHistory();
}
pushState-example.html
<!DOCTYPE html>
<html>
<head>
<title>history.pushState()</title>
<meta charset="UTF-8">
<meta http-equiv="pragma" content="no-cache">
<style>textarea {width:100%;margin-top:10px;}</style>
<script src="pushState-example.js"></script>
</head>
<body onpageshow="showHistory()">
<h2 id="my-h2">history.pushState(..)</h2>
<button onclick="call_pushState()">
Call history.pushState(..)
</button>
<br/><br/>
<button onclick="history.back()">history.back()</button>
<button onclick="history.forward()">history.forward()</button>
<br/>
<textarea rows="8" id="log-area"></textarea>
</body>
</html>
5. history.replaceState()
history.replaceState(dataState, title [,url])
The history.replaceState(..) method updates state, title, and URL for current entity in History.
Parameters:
dataState
An object stores the state of an entity.
title
The title parameter means a suggestion. Browsers don't usually use this parameter to establish title for document.
url
The url parameter is not mandatory, if offered it must have the same origin with the current URL of the page.
6. Popstate event
The popstate event occurs when a user goes to a page (an entity) in history created by using the history.pushState(..) method or history.replaceState(..) method.
You can listen to the popstate event by adding an event listener to the window object.
window.addEventListener(‘popstate’. function(popstateEvent) {
// Do something here!
)};
Or use the window.onpopstate property:
window.onpopstate = function(popstateEvent). {
// Do something here!
};
- Hướng dẫn và ví dụ Javascript PopStateEvent
ECMAScript, Javascript Tutorials
- Introduction to Javascript and ECMAScript
- Quickstart with Javascript
- Alert, Confirm, Prompt Dialog Box in Javascript
- Quickstart with JavaScript
- JavaScript Variables Tutorial with Examples
- Bitwise Operations
- JavaScript Arrays Tutorial with Examples
- JavaScript Loops Tutorial with Examples
- JavaScript Functions Tutorial with Examples
- JavaScript Number Tutorial with Examples
- JavaScript Boolean Tutorial with Examples
- JavaScript Strings Tutorial with Examples
- JavaScript if else Statement Tutorial with Examples
- JavaScript Switch Statement
- JavaScript Error Handling Tutorial with Examples
- JavaScript Date Tutorial with Examples
- JavaScript Modules Tutorial with Examples
- The History of Modules in JavaScript
- JavaScript setTimeout and setInterval Function
- Javascript Form Validation Tutorial with Examples
- JavaScript Web Cookies Tutorial with Examples
- JavaScript void Keyword Tutorial with Examples
- Classes and Objects in JavaScript
- Class and inheritance simulation techniques in JavaScript
- Inheritance and polymorphism in JavaScript
- Undertanding Duck Typing in JavaScript
- JavaScript Symbols Tutorial with Examples
- JavaScript Set Collection Tutorial with Examples
- JavaScript Map Collection Tutorial with Examples
- Undertanding JavaScript Iterables and Iterators
- JavaScript Regular Expressions Tutorial with Examples
- JavaScript Promise, Async/Await Tutorial with Examples
- Javascript Window Tutorial with Examples
- Javascript Console Tutorial with Examples
- Javascript Screen Tutorial with Examples
- Javascript Navigator Tutorial with Examples
- Javascript Geolocation API Tutorial with Examples
- Javascript Location Tutorial with Examples
- Javascript History API Tutorial with Examples
- Javascript Statusbar Tutorial with Examples
- Javascript Locationbar Tutorial with Examples
- Javascript Scrollbars Tutorial with Examples
- Javascript Menubar Tutorial with Examples
- JavaScript JSON Tutorial with Examples
- JavaScript Event Handling Tutorial with Examples
- Javascript MouseEvent Tutorial with Examples
- Javascript WheelEvent Tutorial with Examples
- Javascript KeyboardEvent Tutorial with Examples
- Javascript FocusEvent Tutorial with Examples
- Javascript InputEvent Tutorial with Examples
- Javascript ChangeEvent Tutorial with Examples
- Javascript DragEvent Tutorial with Examples
- Javascript HashChangeEvent Tutorial with Examples
- Javascript URL Encoding Tutorial with Examples
- Javascript FileReader Tutorial with Examples
- Javascript XMLHttpRequest Tutorial with Examples
- Javascript Fetch API Tutorial with Examples
- Parsing XML in Javascript with DOMParser
- Introduction to Javascript HTML5 Canvas API
- Highlighting code with SyntaxHighlighter Javascript library
- What are polyfills in programming science?
Show More