Undertanding JavaScript Iterables and Iterators
1. Iteration
ECMAScript 6 provides you with a new way to interact with data structure, which is Iteration. Now, we will clarify it.
There are two concepts that you need to distinguish:
- Iterator
- Iterable
The concepts of Iterator, Iterables apply to the Array, Set, and Map classes.
2. Concept of Iterator
Iterator: An object called Iterator if it contains a pointer to next element in Iteration.
Technically, an object in ECMAScript is called an Iterator if it has a method with the next() name, and this method returns an object with the form {value:SomeValue,done:booleanValue}. done has true value if the Iteration has been completed, otherwise it has false value.
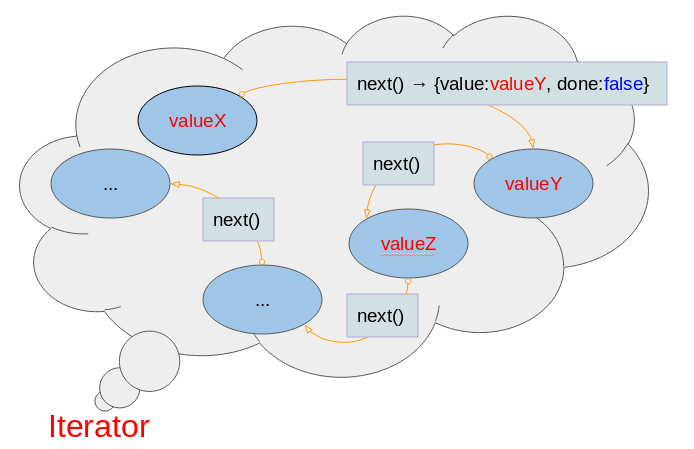
iterator-object-example.js
// An Iterator Object:
let myIterator = {
someProp: "Some Prop",
//
next : function() {
return {
value: "Tom",
done: false
}
}
}
// ----------- TEST -------------------
let entry = myIterator.next();
console.log(entry); // { value: 'Tom', done: false }
console.log(entry.value); // Tom
3. Concept of Iterable
Iterable - In terms of language, an object is called Iterable if it contains a collection of data, and it provides a way to publicize its data. For example, an array can be called Iterable because it contains a collection of data, and you can access its elements.
Technically, in the ECMAScript, an object that is called Iterable must have a method with the key of Symbol.iterator and this method returns an Iterator object
Symbol.iterator is a value of the Symbol data type, like 100 is a value of Integer type. You can see also Symbol in the following post:
Below is a simple example, an object with properties:
- myProp1
- myProp2
- 100
- myProp3
- Symbol.iterator
object-with-properties.js
// An Object:
let myObject = {
// A property
myProp1 : "Some Value 1",
// A property
'myProp2' : "Some Value 2",
// A property
100 : "One hundred",
// A property (Method)
myProp3 : function() {
console.log("I'm a method");
},
// A property (Method)
[Symbol.iterator] : function() {
console.log("I'm a [Symbol.iterator] method");
}
}
// ----------- TEST --------------
console.log( myObject["myProp1"] ); // Some Value 1
console.log( myObject["myProp2"] ); // Some Value 2
console.log( myObject[100] ); // One hundred
myObject["myProp3"](); // I'm a method
myObject[Symbol.iterator]();// I'm a [Symbol.iterator] method
Output:
Some Value 1
Some Value 2
One hundred
I'm a method
I'm a [Symbol.iterator] method
Iterable?
To sum up, an object is called Iterable if it has a method with a Symbol.iterable key, and this method returns an Iterator object.
iterable-object-example.js
// An Iterator Object
let myIterator = {
next : function() {
return {value: Math.random(), done: false};
}
}
// A Iterable object:
let myIterable = {
myProp : "Some value",
// A Method returns an Iterator object.
[Symbol.iterator] : function() {
return myIterator;
}
}
// ------ TEST -----------
// An iterator object.
let it = myIterable[Symbol.iterator]();
let entry = it.next();
console.log(entry);
entry = it.next();
console.log(entry);
Output:
{ value: 0.6026736348993575, done: false }
{ value: 0.1790056262472559, done: false }
A class is called Iterable if it has a method with the [Symbol.iterator] name. The objects created from this class will be Iterable objects.
class-iterable-example.js
// An Iterable class.
class MyClass {
constructor() {
}
someMethod() {
console.log("Some method");
}
[Symbol.iterator]() {
// An Iterator object.
var myIterator = {
next: function() {
return {value: Math.random(),done: false};
}
};
return myIterator;
}
}
// ----------- TEST -----------------
let myObject = new MyClass();
myObject.someMethod();
// An Iterator Object.
let it = myObject[Symbol.iterator]();
let entry;
let i = 0;
while ( (entry = it.next()).done == false ) {
console.log( entry);
i++;
if(i > 5) {
break;
}
}
Note: In the ECMAScript, the Array, Set, Map classes are Iterable.
ECMAScript, Javascript Tutorials
- Introduction to Javascript and ECMAScript
- Quickstart with Javascript
- Alert, Confirm, Prompt Dialog Box in Javascript
- Quickstart with JavaScript
- JavaScript Variables Tutorial with Examples
- Bitwise Operations
- JavaScript Arrays Tutorial with Examples
- JavaScript Loops Tutorial with Examples
- JavaScript Functions Tutorial with Examples
- JavaScript Number Tutorial with Examples
- JavaScript Boolean Tutorial with Examples
- JavaScript Strings Tutorial with Examples
- JavaScript if else Statement Tutorial with Examples
- JavaScript Switch Statement
- JavaScript Error Handling Tutorial with Examples
- JavaScript Date Tutorial with Examples
- JavaScript Modules Tutorial with Examples
- The History of Modules in JavaScript
- JavaScript setTimeout and setInterval Function
- Javascript Form Validation Tutorial with Examples
- JavaScript Web Cookies Tutorial with Examples
- JavaScript void Keyword Tutorial with Examples
- Classes and Objects in JavaScript
- Class and inheritance simulation techniques in JavaScript
- Inheritance and polymorphism in JavaScript
- Undertanding Duck Typing in JavaScript
- JavaScript Symbols Tutorial with Examples
- JavaScript Set Collection Tutorial with Examples
- JavaScript Map Collection Tutorial with Examples
- Undertanding JavaScript Iterables and Iterators
- JavaScript Regular Expressions Tutorial with Examples
- JavaScript Promise, Async/Await Tutorial with Examples
- Javascript Window Tutorial with Examples
- Javascript Console Tutorial with Examples
- Javascript Screen Tutorial with Examples
- Javascript Navigator Tutorial with Examples
- Javascript Geolocation API Tutorial with Examples
- Javascript Location Tutorial with Examples
- Javascript History API Tutorial with Examples
- Javascript Statusbar Tutorial with Examples
- Javascript Locationbar Tutorial with Examples
- Javascript Scrollbars Tutorial with Examples
- Javascript Menubar Tutorial with Examples
- JavaScript JSON Tutorial with Examples
- JavaScript Event Handling Tutorial with Examples
- Javascript MouseEvent Tutorial with Examples
- Javascript WheelEvent Tutorial with Examples
- Javascript KeyboardEvent Tutorial with Examples
- Javascript FocusEvent Tutorial with Examples
- Javascript InputEvent Tutorial with Examples
- Javascript ChangeEvent Tutorial with Examples
- Javascript DragEvent Tutorial with Examples
- Javascript HashChangeEvent Tutorial with Examples
- Javascript URL Encoding Tutorial with Examples
- Javascript FileReader Tutorial with Examples
- Javascript XMLHttpRequest Tutorial with Examples
- Javascript Fetch API Tutorial with Examples
- Parsing XML in Javascript with DOMParser
- Introduction to Javascript HTML5 Canvas API
- Highlighting code with SyntaxHighlighter Javascript library
- What are polyfills in programming science?
Show More