Javascript HashChangeEvent Tutorial with Examples
1. HashChangeEvent
Hash is "Anchor part" of an URL. It tells the browser to scroll the browser scrollbar to display the content area corresponding to Hash.
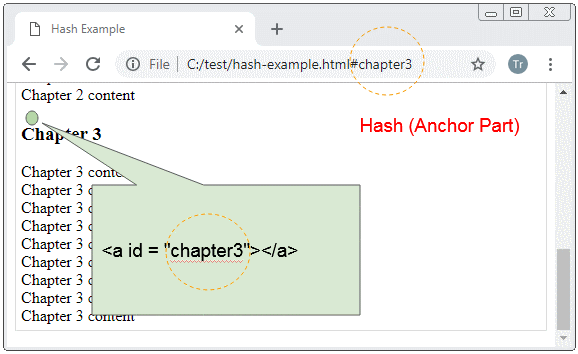
Ví dụ với Hash:
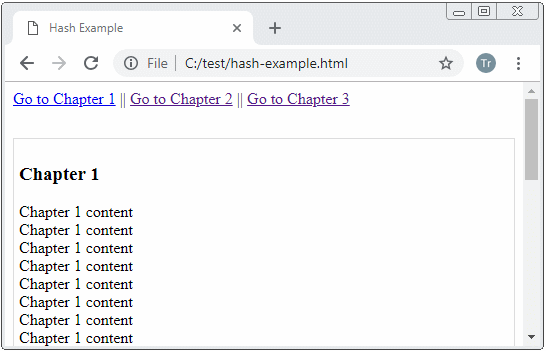
hash-example.html
<!DOCTYPE html>
<html>
<head>
<title>Hash Example</title>
<meta charset="UTF-8">
<style>
.content {
margin-top: 30px;
padding: 5px;
border: 1px solid #ddd;
}
</style>
</head>
<body>
<a href="#chapter1">Go to Chapter 1</a>
||
<a href="#chapter2">Go to Chapter 2</a>
||
<a href="#chapter3">Go to Chapter 3</a>
<div class="content">
<!-- Anchor 1 -->
<a id="chapter1"></a>
<h3>Chapter 1</h3>
Chapter 1 content <br/>
Chapter 1 content <br/>
Chapter 1 content <br/>
Chapter 1 content <br/>
Chapter 1 content <br/>
Chapter 1 content <br/>
Chapter 1 content <br/>
Chapter 1 content <br/>
Chapter 1 content <br/>
<!-- Anchor 2 -->
<a id="chapter2"></a>
<h3>Chapter 2</h3>
Chapter 2 content <br/>
Chapter 2 content <br/>
Chapter 2 content <br/>
Chapter 2 content <br/>
Chapter 2 content <br/>
Chapter 2 content <br/>
Chapter 2 content <br/>
Chapter 2 content <br/>
Chapter 2 content <br/>
<!-- Anchor 3 -->
<a id="chapter3"></a>
<h3>Chapter 3</h3>
Chapter 3 content <br/>
Chapter 3 content <br/>
Chapter 3 content <br/>
Chapter 3 content <br/>
Chapter 3 content <br/>
Chapter 3 content <br/>
Chapter 3 content <br/>
Chapter 3 content <br/>
Chapter 3 content <br/>
</div>
<p id="log-div"></p>
</body>
</html>
HashChangeEvent
HashChangeEvent is a sub- interface of interface Event. It represents the event that occurs when the "Anchor part" on the URL is changed.
Events:
Event | Description |
hashchange | Event occurs when the "Anchor part" on the URL is changed. |
Properties:
Property | Description |
newURL | Returns the URL of the document, after the Hash has been changed |
oldURL | Returns the URL of the document, before the Hash was changed |
Basically, the newURL, oldURL properties of HashChangeEvent are supported by all browsers excluding IE.
Example with HashChangeEvent:
hashchangeevents-example.html
<!DOCTYPE html>
<html>
<head>
<title>HashChangeEvent Example</title>
<meta charset="UTF-8">
<style>
.content {
margin-top: 30px;
padding: 5px;
border: 1px solid #ddd;
}
</style>
<script>
function hashchangeHandler(evt) {
var msg = "Hash Change! \n"
+ "event.newURL= "+ evt.newURL +"\n"
+ "event.oldURL= "+ evt.oldURL ;
alert(msg);
}
</script>
</head>
<body onhashchange="hashchangeHandler(event)">
<a href="#chapter1">Go to Chapter 1</a>
||
<a href="#chapter2">Go to Chapter 2</a>
||
<a href="#chapter3">Go to Chapter 3</a>
<div class="content">
<!-- Anchor 1 -->
<a id="chapter1"></a>
<h3>Chapter 1</h3>
Chapter 1 content <br/>
Chapter 1 content <br/>
Chapter 1 content <br/>
Chapter 1 content <br/>
Chapter 1 content <br/>
Chapter 1 content <br/>
Chapter 1 content <br/>
Chapter 1 content <br/>
Chapter 1 content <br/>
<!-- Anchor 2 -->
<a id="chapter2"></a>
<h3>Chapter 2</h3>
Chapter 2 content <br/>
Chapter 2 content <br/>
Chapter 2 content <br/>
Chapter 2 content <br/>
Chapter 2 content <br/>
Chapter 2 content <br/>
Chapter 2 content <br/>
Chapter 2 content <br/>
Chapter 2 content <br/>
<!-- Anchor 3 -->
<a id="chapter3"></a>
<h3>Chapter 3</h3>
Chapter 3 content <br/>
Chapter 3 content <br/>
Chapter 3 content <br/>
Chapter 3 content <br/>
Chapter 3 content <br/>
Chapter 3 content <br/>
Chapter 3 content <br/>
Chapter 3 content <br/>
Chapter 3 content <br/>
</div>
</body>
</html>
ECMAScript, Javascript Tutorials
- Introduction to Javascript and ECMAScript
- Quickstart with Javascript
- Alert, Confirm, Prompt Dialog Box in Javascript
- Quickstart with JavaScript
- JavaScript Variables Tutorial with Examples
- Bitwise Operations
- JavaScript Arrays Tutorial with Examples
- JavaScript Loops Tutorial with Examples
- JavaScript Functions Tutorial with Examples
- JavaScript Number Tutorial with Examples
- JavaScript Boolean Tutorial with Examples
- JavaScript Strings Tutorial with Examples
- JavaScript if else Statement Tutorial with Examples
- JavaScript Switch Statement
- JavaScript Error Handling Tutorial with Examples
- JavaScript Date Tutorial with Examples
- JavaScript Modules Tutorial with Examples
- The History of Modules in JavaScript
- JavaScript setTimeout and setInterval Function
- Javascript Form Validation Tutorial with Examples
- JavaScript Web Cookies Tutorial with Examples
- JavaScript void Keyword Tutorial with Examples
- Classes and Objects in JavaScript
- Class and inheritance simulation techniques in JavaScript
- Inheritance and polymorphism in JavaScript
- Undertanding Duck Typing in JavaScript
- JavaScript Symbols Tutorial with Examples
- JavaScript Set Collection Tutorial with Examples
- JavaScript Map Collection Tutorial with Examples
- Undertanding JavaScript Iterables and Iterators
- JavaScript Regular Expressions Tutorial with Examples
- JavaScript Promise, Async/Await Tutorial with Examples
- Javascript Window Tutorial with Examples
- Javascript Console Tutorial with Examples
- Javascript Screen Tutorial with Examples
- Javascript Navigator Tutorial with Examples
- Javascript Geolocation API Tutorial with Examples
- Javascript Location Tutorial with Examples
- Javascript History API Tutorial with Examples
- Javascript Statusbar Tutorial with Examples
- Javascript Locationbar Tutorial with Examples
- Javascript Scrollbars Tutorial with Examples
- Javascript Menubar Tutorial with Examples
- JavaScript JSON Tutorial with Examples
- JavaScript Event Handling Tutorial with Examples
- Javascript MouseEvent Tutorial with Examples
- Javascript WheelEvent Tutorial with Examples
- Javascript KeyboardEvent Tutorial with Examples
- Javascript FocusEvent Tutorial with Examples
- Javascript InputEvent Tutorial with Examples
- Javascript ChangeEvent Tutorial with Examples
- Javascript DragEvent Tutorial with Examples
- Javascript HashChangeEvent Tutorial with Examples
- Javascript URL Encoding Tutorial with Examples
- Javascript FileReader Tutorial with Examples
- Javascript XMLHttpRequest Tutorial with Examples
- Javascript Fetch API Tutorial with Examples
- Parsing XML in Javascript with DOMParser
- Introduction to Javascript HTML5 Canvas API
- Highlighting code with SyntaxHighlighter Javascript library
- What are polyfills in programming science?
Show More