Javascript MouseEvent Tutorial with Examples
1. MouseEvent
MouseEvent is an interface that represents for the events occurring when users interact with pointing devices, such as mouse, common events such as click, dbclick, mousedown, mouseup,...
MouseEvent is an sub-interface of UIEvent.
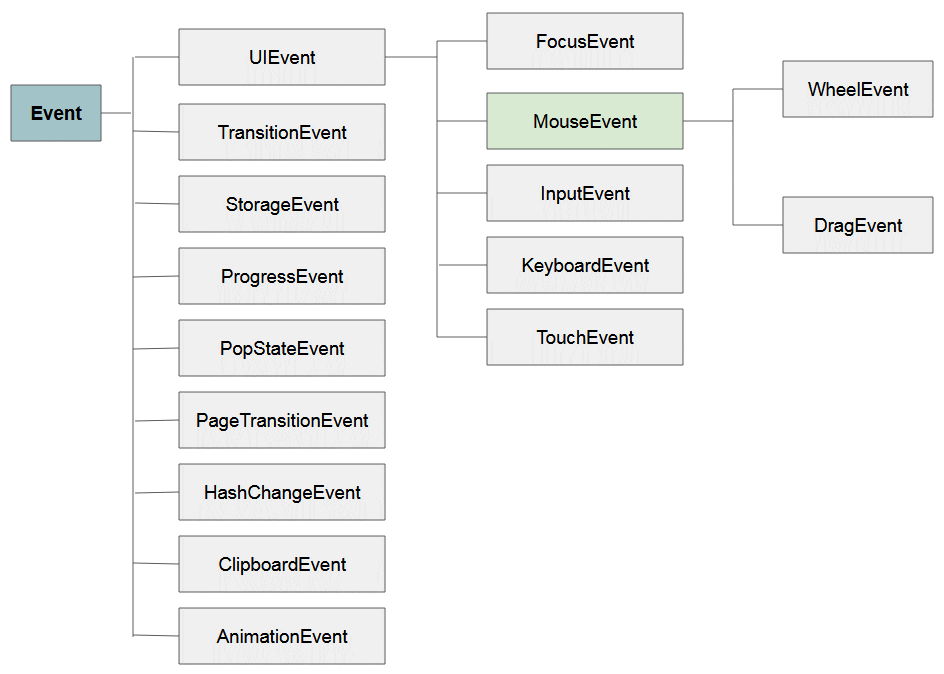
Event types of MouseEvent:
Event | Description |
click | The event occurs when the user clicks on an element |
contextmenu | The event occurs when the user right-clicks on an element to open a context menu |
dblclick | The event occurs when the user double-clicks on an element |
mousedown | The event occurs when the user presses a mouse button over an element |
mouseup | The event occurs when a user releases a mouse button over an element |
mouseenter | The event occurs when the pointer is moved onto an element |
mouseleave | The event occurs when the pointer is moved out of an element |
mousemove | The event occurs when the pointer is moving while it is over an element or its children. |
mouseout | The event occurs when a user moves the mouse pointer out of an element, or out of one of its children |
mouseover | The event occurs when the pointer is moved onto an element, or onto one of its children |
mousedown, mouseup, click
Let's start with the most common event out of the mouse events that is click. It is formed from pressing down and releasing. The click event is illustrated as below:
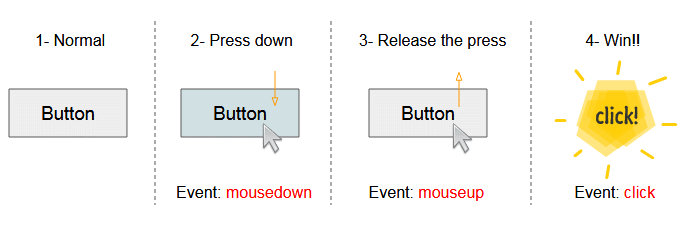
dbclick
If you click 2 times quickly, it will create a dbclick event.
mouseover vs mouseenter
The mouseenter event occurs when the cursor enters an element. The mouseover event occurs when the cursor enters an element or one of its child elements.
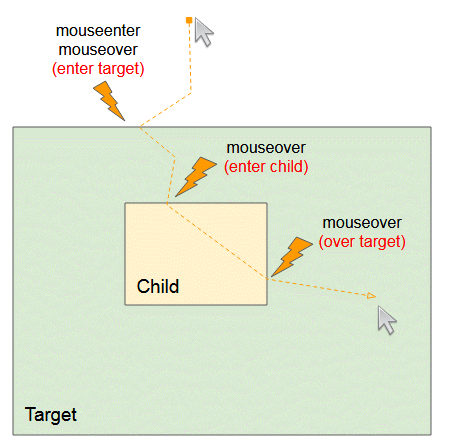
mouseleave vs mouseout
The mouseleave element occurs when the cursor moves out of an element. The mouseout event happens when the cursor moves out of an element or one of its child elements.
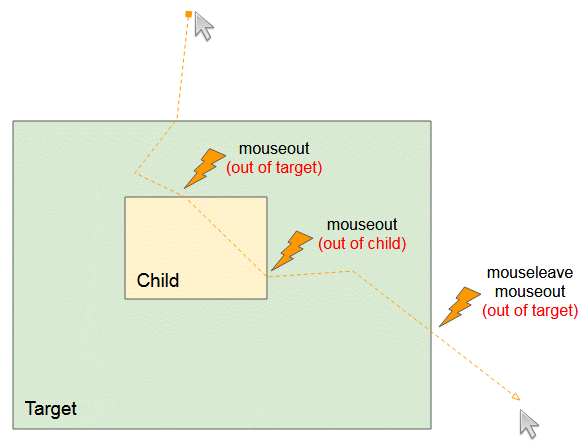
The following example helps you compare mouseenter vs mouseover, mouseleave vs mouseout:
enter-vs-over-leave-vs-out.html
<!DOCTYPE html>
<html>
<head>
<title>Javascript Mouse Events</title>
<script src="enter-vs-over-leave-vs-out.js"></script>
<style>
#target-div {
width: 320px;
height: 150px;
background: blue;
}
#target-div > div {
width: 50px;
height: 80px;
margin: 40px 0 0 40px;
padding: 5px 0 0 5px;
float: left;
background: yellow;
}
</style>
</head>
<body>
<h3>mouseenter vs mouseover</h3>
<h3>mouseleave vs mouseout</h3>
<div id="target-div"
onmouseenter="mouseenterHandler(event)"
onmouseover="mouseoverHandler(event)"
onmouseleave="mouseleaveHandler(event)"
onmouseout="mouseoutHandler(event)"
>
<div>Child</div>
<div>Child</div>
<div>Child</div>
</div>
<h3>Statistics:</h3>
<div style="color:red;" id="statistics-div">
</div>
</body>
</html>
enter-vs-over-leave-vs-out.js
var enterCount = 0;
var overCount = 0;
var leaveCount = 0;
var outCount = 0;
function mouseenterHandler(evt) {
enterCount += 1;
showStatistics();
}
function mouseoverHandler(evt) {
overCount += 1;
showStatistics();
}
function mouseleaveHandler(evt) {
leaveCount += 1;
showStatistics();
}
function mouseoutHandler(evt) {
outCount += 1;
showStatistics();
}
function showStatistics() {
var html =
"enterCount:" + enterCount + "<br/>" //
+
"overCount:" + overCount + "<hr/>" //
+
"leaveCount:" + leaveCount + "<br/>" //
+
"outCount:" + outCount ;
document.getElementById("statistics-div").innerHTML = html;
}
mousemove
mousemove-example.html
<!DOCTYPE html>
<html>
<head>
<title>Javascript Mouse Events</title>
<script src="mousemove-example.js"></script>
<style>
#target-div {
width: 320px;
height: 150px;
background: blue;
margin-bottom: 50px;
}
#target-div > div {
width: 50px;
height: 150px;
margin: 40px 0 0 40px;
padding: 5px 0 0 5px;
float: left;
background: yellow;
}
</style>
</head>
<body>
<h3>mousemove example</h3>
<div id="target-div" onmousemove="mousemoveHandler(event)">
<div>Child</div>
<div>Child</div>
<div>Child</div>
</div>
<h3>Statistics:</h3>
<div style="color:red;" id="statistics-div">
</div>
</body>
</html>
mousemove-example.js
var moveCount = 0;
function mousemoveHandler(evt) {
moveCount += 1;
showStatistics();
}
function showStatistics() {
var html = "moveCount:" + moveCount;
document.getElementById("statistics-div").innerHTML = html;
}
2. Properties & Methods
MouseEvent is the sub- interface of UIEvent, therefore, it inherits the properties and methods from this interface.
- Javascript UiEvent
key
Property | Description |
altKey | Returns true if "ALT" key was pressed when the mouse event was triggered, otherwise returns false. |
ctrlKey | Returns true if "CTRL" key was pressed when the mouse event was triggered, otherwise returns false. |
shiftKey | Returns true if "SHIFT" key was pressed when an event was triggered, otherwise returns false. |
metaKey | Returns true if "META" key was pressed when an event was triggered, otherwise returns false. |
Example with the ctrlKey property:
prop-ctrlKey-example.html
<!DOCTYPE html>
<html>
<head>
<title>Javascript Mouse Events</title>
<style>
#target-div {
width: 320px;
height: 150px;
background: blue;
margin-bottom: 10px;
}
</style>
<script>
function clickHandler(evt) {
var html = "evt.ctrlKey=" + evt.ctrlKey;
document.getElementById("log-div").innerHTML = html;
}
</script>
</head>
<body>
<h3>Press Ctrl key and Click</h3>
<div id="target-div" onclick="clickHandler(event)" >
</div>
<div style="color:red;" id="log-div">
</div>
</body>
</html>
Position:
Property | Description |
clientX | Returns the horizontal coordinate of the mouse pointer, relative to the current window. |
clientY | Returns the vertical coordinate of the mouse pointer, relative to the current window. |
offsetX | Returns the horizontal coordinate of the mouse pointer relative to the position of the edge of the target element |
offsetY | Returns the vertical coordinate of the mouse pointer relative to the position of the edge of the target element |
pageX | Returns the horizontal coordinate of the mouse pointer, relative to the document. |
pageY | Returns the vertical coordinate of the mouse pointer, relative to the document. |
screenX | Returns the horizontal coordinate of the mouse pointer, relative to the screen (Computer or device) |
screenY | Returns the vertical coordinate of the mouse pointer, relative to the screen (Computer or device) |
movementX | Returns the horizontal coordinate of the mouse pointer relative to the position of the last mousemove event |
movementY | Returns the vertical coordinate of the mouse pointer relative to the position of the last mousemove event |
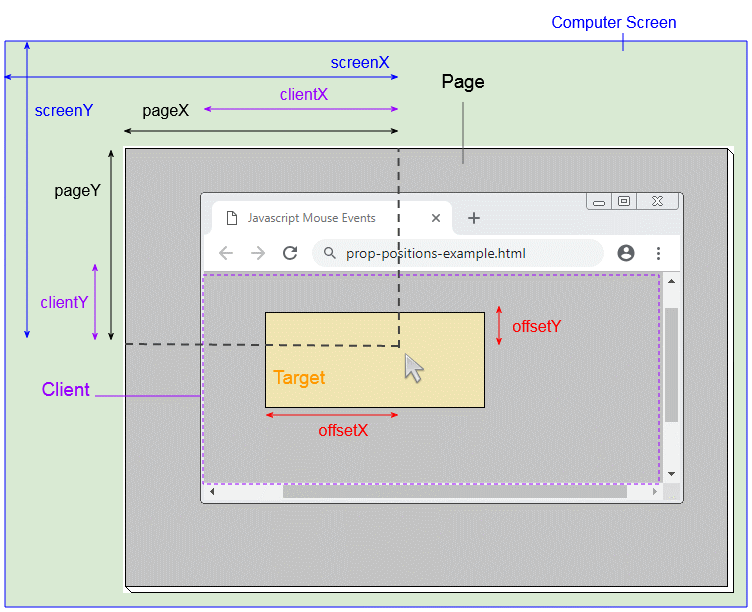
prop-positions-example.html
<!DOCTYPE html>
<html>
<head>
<title>Javascript Mouse Events</title>
<script src="prop-positions-example.js"></script>
<style>
#target-div {
width: 300px;
height: 210px;
background: #ccc;
margin: 0px 0px 10px 20px;
padding: 5px;
}
</style>
</head>
<body style="height:450px; width:1000px;">
<h3>Positions</h3>
<div id="target-div" onmousemove="mousemoveHandler(event)" >
</div>
</body>
</html>
prop-positions-example.js
function mousemoveHandler(evt) {
var html = "offsetX = " + evt.offsetX +"<br/>"
+ "offsetY = " + evt.offsetY +"<br/>"
+ "clientX = " + evt.clientX +"<br/>"
+ "clientY = " + evt.clientY +"<br/>"
+ "pageX = " + evt.pageX +"<br/>"
+ "pageY = " + evt.pageY +"<br/>"
+ "screenX = " + evt.screenX +"<br/>"
+ "screenY = " + evt.screenY +"<br/>"
+ "movementX = " + evt.movementX +"<br/>"
+ "movementY = " + evt.movementY;
document.getElementById("target-div").innerHTML = html;
}
button
Property | Description |
button | Returns a number that describes which mouse button was pressed when the mouse event was triggered |
buttons | Returns a number that describes which mouse buttons were pressed when the mouse event was triggered |
(Not a standard property, should not be used). |
event.button:
- 0: Main button, usually the left button or the un-initialized state
- 1: Auxiliary button, usually the wheel button or the middle button (if present)
- 2: Secondary button, usually the right button
- 3: Fourth button, typically the Browser Back button
- 4: Fifth button, typically the Browser Forward button
event.buttons:
- 0 : No button or un-initialized
- 1 : Primary button, usually the left button.
- 2 : Secondary button, usually the right button.
- 4 : Auxilary button, usually the mouse wheel button or middle button.
- 8 : 4th button, typically the "Browser Back" button.
- 16 : 5th button, typically the "Browser Forward" button.
event.buttons may return a different value other than the above values. For example, event.buttons returns 3 if you press both the left mouse and right mouse at the same time.
prop-button-buttons-example.html
<!DOCTYPE html>
<html>
<head>
<title>MouseEvent Example</title>
<script>
function mousedownHandler(evt) {
var logDiv = document.getElementById("log-div");
logDiv.innerHTML = "event.button= " + evt.button +"<br/>"
+ "event.buttons= "+ evt.buttons;
}
</script>
</head>
<body>
<h3>event.button, event.buttons</h3>
<button onmousedown="mousedownHandler(event)">Click Me!</button>
<br/><br/>
<div id="log-div" style="color:red;"></div>
</body>
</html>
Other properties
Property | Description |
relatedTarget | Returns the element related to the element that triggered the mouse event |
ECMAScript, Javascript Tutorials
- Introduction to Javascript and ECMAScript
- Quickstart with Javascript
- Alert, Confirm, Prompt Dialog Box in Javascript
- Quickstart with JavaScript
- JavaScript Variables Tutorial with Examples
- Bitwise Operations
- JavaScript Arrays Tutorial with Examples
- JavaScript Loops Tutorial with Examples
- JavaScript Functions Tutorial with Examples
- JavaScript Number Tutorial with Examples
- JavaScript Boolean Tutorial with Examples
- JavaScript Strings Tutorial with Examples
- JavaScript if else Statement Tutorial with Examples
- JavaScript Switch Statement
- JavaScript Error Handling Tutorial with Examples
- JavaScript Date Tutorial with Examples
- JavaScript Modules Tutorial with Examples
- The History of Modules in JavaScript
- JavaScript setTimeout and setInterval Function
- Javascript Form Validation Tutorial with Examples
- JavaScript Web Cookies Tutorial with Examples
- JavaScript void Keyword Tutorial with Examples
- Classes and Objects in JavaScript
- Class and inheritance simulation techniques in JavaScript
- Inheritance and polymorphism in JavaScript
- Undertanding Duck Typing in JavaScript
- JavaScript Symbols Tutorial with Examples
- JavaScript Set Collection Tutorial with Examples
- JavaScript Map Collection Tutorial with Examples
- Undertanding JavaScript Iterables and Iterators
- JavaScript Regular Expressions Tutorial with Examples
- JavaScript Promise, Async/Await Tutorial with Examples
- Javascript Window Tutorial with Examples
- Javascript Console Tutorial with Examples
- Javascript Screen Tutorial with Examples
- Javascript Navigator Tutorial with Examples
- Javascript Geolocation API Tutorial with Examples
- Javascript Location Tutorial with Examples
- Javascript History API Tutorial with Examples
- Javascript Statusbar Tutorial with Examples
- Javascript Locationbar Tutorial with Examples
- Javascript Scrollbars Tutorial with Examples
- Javascript Menubar Tutorial with Examples
- JavaScript JSON Tutorial with Examples
- JavaScript Event Handling Tutorial with Examples
- Javascript MouseEvent Tutorial with Examples
- Javascript WheelEvent Tutorial with Examples
- Javascript KeyboardEvent Tutorial with Examples
- Javascript FocusEvent Tutorial with Examples
- Javascript InputEvent Tutorial with Examples
- Javascript ChangeEvent Tutorial with Examples
- Javascript DragEvent Tutorial with Examples
- Javascript HashChangeEvent Tutorial with Examples
- Javascript URL Encoding Tutorial with Examples
- Javascript FileReader Tutorial with Examples
- Javascript XMLHttpRequest Tutorial with Examples
- Javascript Fetch API Tutorial with Examples
- Parsing XML in Javascript with DOMParser
- Introduction to Javascript HTML5 Canvas API
- Highlighting code with SyntaxHighlighter Javascript library
- What are polyfills in programming science?
Show More