Javascript FocusEvent Tutorial with Examples
1. FocusEvent
FocusEvent is an interface that represents the events related to focus, namely, the 4 events such as focus, focusin, blur, focusout.
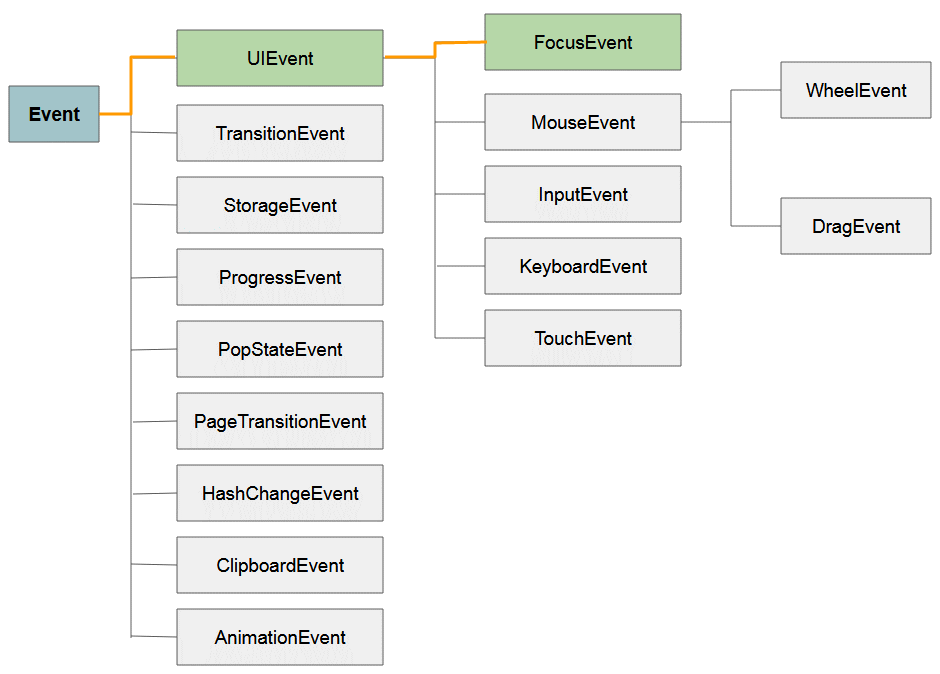
- Javascript UiEvent
Properties:
Property | Description |
relatedTarget | Returns the element related to the element that triggered the event |
If you jump to an element and then jump outside (focus to another element), there will be 4 events that occur in sequence respectively:
- focus
- focusin
- blue
- focusout
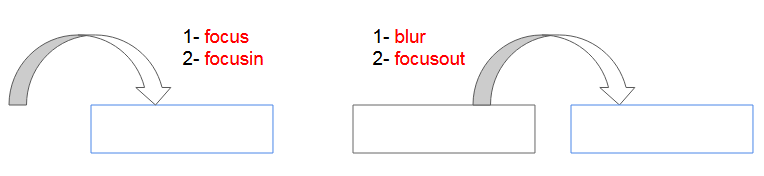
The focus event occurs before focusin. In fact, they happen very close to each other, which can be considered as occurring at the same time. Similarly, the blur event occurs before focusout, iIn fact, they happen very close to each other, which can be considered as occurring at the same time.
focus
The focus event occurs when you are in other place and jumps into an element, such as the <input> element to prepare to enter content for this element.
blur
The blur event occurs when you jump out of an element (focus on another element).
focusevent-example.html
<!DOCTYPE html>
<html>
<head>
<title>FocusEvent</title>
<script>
function focusHandler(evt) {
showLog("focus");
}
function blurHandler(evt) {
showLog("blur");
}
function showLog(msg) {
var oldHtml= document.getElementById("log-div").innerHTML;
document.getElementById("log-div").innerHTML=oldHtml + " .. "+ msg;
}
</script>
</head>
<body>
<h3>Test events: focus, blur</h3>
<input type="text"
onfocus="focusHandler(event)"
onblur="blurHandler(event)" />
<br><br>
<div id="log-div" style="padding:10px;border:1px solid #ccd;"></div>
</body>
</html>
2. focusin, focusout
The focus, blur events are designed for the elements are "focusable", for example, <input>, <textarea>,.. While the focusin, focusout events are designed for the elements that can contain other elements for example, <div>, <span>, <form>,..
focus vs focusin
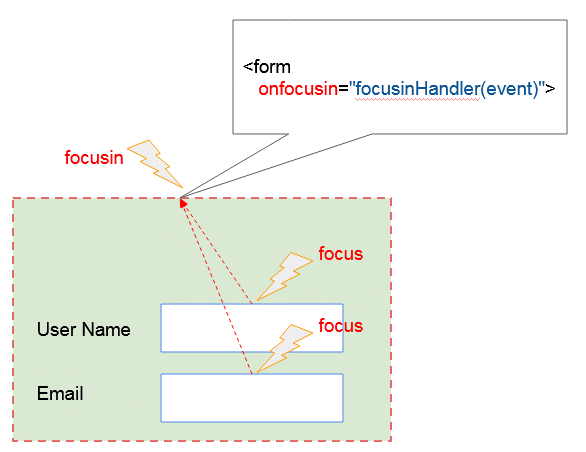
Suppose you have a <form> with 2 <input> child elements. When an user jumps into one of the <input> elements then the focus event will occur. The focus event at the child element will create the focusin event at the parent elements. Thus this focusin event will occur at the <form> element. Note: Although the focusin event occurs on the parent element, but event.target returns the child element (The element that fires the focus event).
blur vs focusout
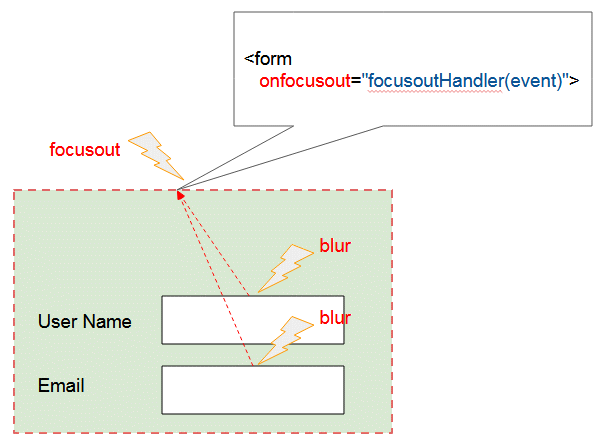
Suppose you have a <form> with 2 <input> child elements. When an user jumps out of the <input> element, the blur event will happen.The blur event at the child element will create the focusout event at the parent elements. Thus this focusout event will occur at the <form> element. Note: Although the focusout event occurs on the parent element, but event.target returns the child element (The element that fires the blur event).
Example: focusin & focusout
focusin-focusout-example.html
<!DOCTYPE html>
<html>
<head>
<title>FocusEvent</title>
<meta charset="utf-8" />
<script src="focusin-focusout-example.js"></script>
<style>
form {
border: 1px solid #ccc;
padding: 10px;
display: inline-block;
}
</style>
</head>
<body>
<h3>Events: focusin & focusout</h3>
<p>Focus to 'input' and Blur to it</p>
<form onfocusin="focusinHandler(event)" onfocusout="focusoutHandler(event)">
User Name
<input type="text" name="username" />
<br>
<br> Email
<input type="text" name="email" />
</form>
</body>
</html>
focusin-focusout-example.js
function focusinHandler(evt) {
// <input>
evt.target.style.border="1px solid blue";
// <form>
evt.target.parentElement.style.border="1px solid red";
}
function focusoutHandler(evt) {
// <input>
evt.target.style.border="1px solid black";
// <form>
evt.target.parentElement.style.border="1px solid black";
}
Do you have problem with onfocusout (???)
For the <input>, <textarea>,.. elements, you need only to handle the focus, blur events. The onfocusout attribute doesn't work with this element on some browsers (I have checked this problem on the Firefox, Chrome browsers).
<input type="text"
onfocus="focusHandler(event)"
onblur="blurHandler(event)"
onfocusin="focusinHandler(event)"onforcusout="focusoutHandler(event)"
/>
attr-onfocusout-not-working.html
<!DOCTYPE html>
<html>
<head>
<title>FocusEvent</title>
<script>
function focusHandler(evt) {
showLog("focus");
}
function focusinHandler(evt) {
showLog("focusin");
}
function focusoutHandler(evt) {
showLog("focusout");
}
function blurHandler(evt) {
showLog("blur");
}
function showLog(msg) {
var oldHtml= document.getElementById("log-div").innerHTML;
document.getElementById("log-div").innerHTML=oldHtml + " .. "+ msg;
}
</script>
</head>
<body>
<h3>Why does the 'onfocusout' attribute not work with 'input'?</h3>
<input type="text"
onfocus="focusHandler(event)"
onblur="blurHandler(event)"
onfocusin="focusinHandler(event)"
onforcusout="focusoutHandler(event)" />
<br><br>
<div id="log-div" style="padding:10px;border:1px solid #ccd;"></div>
</body>
</html>
Althought the onfocusout attribute doesn't work with the <input>, <textarea>,.. elements on some browsers. But, if desiring, you can still register the focusout event for them in the following way:
// Javascript Code:
var myInput = document.getElementById("my-input");
myInput.addEventListener("focus", focusHandler);
myInput.addEventListener("focusin", focusinHandler);
myInput.addEventListener("focusout", focusoutHandler);
myInput.addEventListener("blur", blurHandler);
See full example:
focusevents-addEventListener-example.html
<!DOCTYPE html>
<html>
<head>
<title>FocusEvent</title>
<meta charset="utf-8"/>
</head>
<body>
<h3>Test events: focus, focusin, focusout, blur</h3>
<input id="my-input" type="text" />
<br><br>
<div id="log-div" style="padding:10px;border:1px solid #ccd;"></div>
<script src="focusevents-addEventListener-example.js"></script>
</body>
</html>
focusevents-addEventListener-example.js
function focusHandler(evt) {
showLog("focus");
}
function focusinHandler(evt) {
showLog("focusin");
}
function focusoutHandler(evt) {
showLog("focusout");
}
function blurHandler(evt) {
showLog("blur");
}
function showLog(msg) {
var oldHtml= document.getElementById("log-div").innerHTML;
document.getElementById("log-div").innerHTML=oldHtml + " .. "+ msg;
}
// Javascript Code:
var myInput = document.getElementById("my-input");
myInput.addEventListener("focus", focusHandler);
myInput.addEventListener("focusin", focusinHandler);
myInput.addEventListener("focusout", focusoutHandler);
myInput.addEventListener("blur", blurHandler);
ECMAScript, Javascript Tutorials
- Introduction to Javascript and ECMAScript
- Quickstart with Javascript
- Alert, Confirm, Prompt Dialog Box in Javascript
- Quickstart with JavaScript
- JavaScript Variables Tutorial with Examples
- Bitwise Operations
- JavaScript Arrays Tutorial with Examples
- JavaScript Loops Tutorial with Examples
- JavaScript Functions Tutorial with Examples
- JavaScript Number Tutorial with Examples
- JavaScript Boolean Tutorial with Examples
- JavaScript Strings Tutorial with Examples
- JavaScript if else Statement Tutorial with Examples
- JavaScript Switch Statement
- JavaScript Error Handling Tutorial with Examples
- JavaScript Date Tutorial with Examples
- JavaScript Modules Tutorial with Examples
- The History of Modules in JavaScript
- JavaScript setTimeout and setInterval Function
- Javascript Form Validation Tutorial with Examples
- JavaScript Web Cookies Tutorial with Examples
- JavaScript void Keyword Tutorial with Examples
- Classes and Objects in JavaScript
- Class and inheritance simulation techniques in JavaScript
- Inheritance and polymorphism in JavaScript
- Undertanding Duck Typing in JavaScript
- JavaScript Symbols Tutorial with Examples
- JavaScript Set Collection Tutorial with Examples
- JavaScript Map Collection Tutorial with Examples
- Undertanding JavaScript Iterables and Iterators
- JavaScript Regular Expressions Tutorial with Examples
- JavaScript Promise, Async/Await Tutorial with Examples
- Javascript Window Tutorial with Examples
- Javascript Console Tutorial with Examples
- Javascript Screen Tutorial with Examples
- Javascript Navigator Tutorial with Examples
- Javascript Geolocation API Tutorial with Examples
- Javascript Location Tutorial with Examples
- Javascript History API Tutorial with Examples
- Javascript Statusbar Tutorial with Examples
- Javascript Locationbar Tutorial with Examples
- Javascript Scrollbars Tutorial with Examples
- Javascript Menubar Tutorial with Examples
- JavaScript JSON Tutorial with Examples
- JavaScript Event Handling Tutorial with Examples
- Javascript MouseEvent Tutorial with Examples
- Javascript WheelEvent Tutorial with Examples
- Javascript KeyboardEvent Tutorial with Examples
- Javascript FocusEvent Tutorial with Examples
- Javascript InputEvent Tutorial with Examples
- Javascript ChangeEvent Tutorial with Examples
- Javascript DragEvent Tutorial with Examples
- Javascript HashChangeEvent Tutorial with Examples
- Javascript URL Encoding Tutorial with Examples
- Javascript FileReader Tutorial with Examples
- Javascript XMLHttpRequest Tutorial with Examples
- Javascript Fetch API Tutorial with Examples
- Parsing XML in Javascript with DOMParser
- Introduction to Javascript HTML5 Canvas API
- Highlighting code with SyntaxHighlighter Javascript library
- What are polyfills in programming science?
Show More