JavaScript Modules Tutorial with Examples
1. ES Module
ECMAScript 6 introduces ES6 Module Syntax to help developers modularize their code. Simply put, you can write your code on separate files. On this file, you can export necessary things in modules. Other files can import the modules of such file to use.
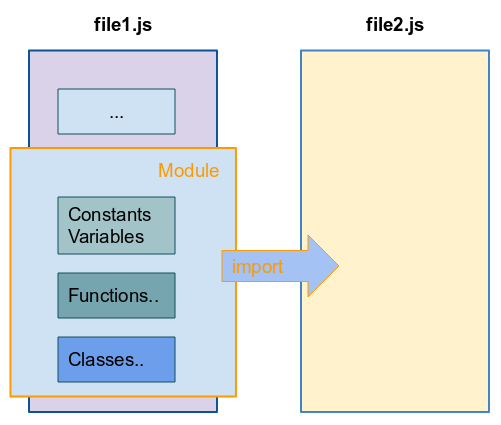
Module and import/export are a great idea to help you easily manage and maintain code in large applications. The code that you write in a protected file can be accessed from another file only when it is exported in a module form.
To be simple, here, I create the two files such as es6-module-file.js & es6-file1.js.
- es6-module-file.js: This file defines constants, functions, classes, ... Some of them are packaged in the same module and export this module.
- es6-file1.js: a file that imports some modules of the es6-module-file.js file for use.
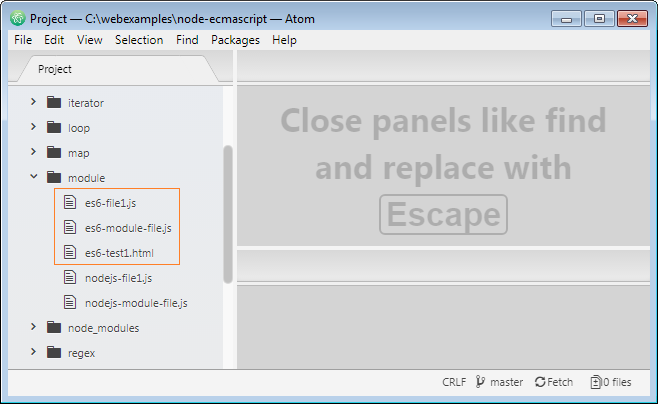
se6-module-file.js
// Constants
const HELLO = "Hello Everybody";
const BYE = "Goodbye!";
// Private function (Do not export this function)
function doSomething() {
console.log("Do Something");
}
// A Function
let sayHello = function(name) {
if(name) {
console.log("Hello " + name);
} else {
console.log(HELLO);
}
}
// A Function
let sayGoodbye = function(name) {
if(name) {
console.log("Goodbye " + name);
} else {
console.log(BYE);
}
}
// Export a Module
export {HELLO, BYE, sayHello, sayGoodbye};
// Export as default Module.
// IMPORTANT!!: Allow at most one 'default'
export default {sayHello, sayGoodbye};
The es6-file1.js file imports some modules of the es6-module-file.js file:
es6-file1.js
// Import *
import * as myModule from './es6-module-file.js';
console.log( myModule.HELLO ); // Hello Everybody
console.log( myModule.BYE ); // Goodbye!
myModule.sayHello("Tom") ; // Hello Tom
myModule.sayGoodbye("Tom") ;// Goodbye Tom
For testing the example, the simplest way is to create a HTML file, for example, test1.html:
Note: You have to use <script type="module"> instead of <script type="text/javascript">.
es6-test1.html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Import/Export</title>
<!-- IMPORTANT!! type = "module" -->
<script type="module" src="es6-file1.js"></script>
</head>
<body>
Show informations in the Console.
</body>
</html>
You need to run the es6-test1.html file on an HTTP Server, and you can see the result in the browser's Console window. Note: The ES6 Module will not work if you run the HTML file directly on the browser with the schema file: ///.
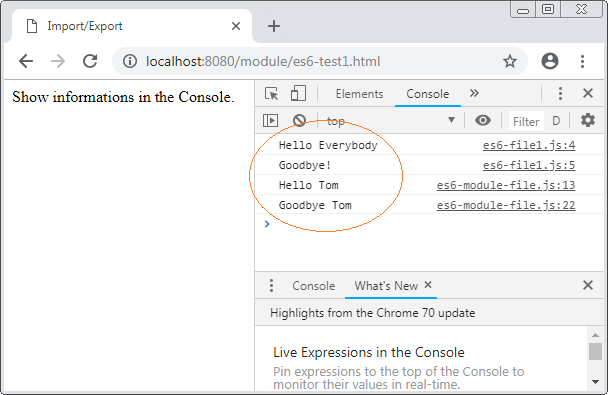
NodeJS!
If you receive the following error while running the es6-file1.js file on NodeJS. You can see the explanation at the end of this post.C:\webexamples\node-ecmascript\module\es6-file1.js:2 import * as myModule from './es6-module-file.js'; ^^^^^^ SyntaxError: Unexpected token import at createScript (vm.js:80:10) at Object.runInThisContext (vm.js:139:10) at Module._compile (module.js:617:28) at Object.Module._extensions..js (module.js:664:10) at Module.load (module.js:566:32) at tryModuleLoad (module.js:506:12) at Function.Module._load (module.js:498:3) at Function.Module.runMain (module.js:694:10) at startup (bootstrap_node.js:204:16) at bootstrap_node.js:625:3
2. ES Import Syntaxes
Import Syntaxes
import defaultExport from "module-name";
import * as name from "module-name";
import { export } from "module-name";
import { export as alias } from "module-name";
import { export1 , export2 } from "module-name";
import { foo , bar } from "module-name/path/to/specific/un-exported/file";
import { export1 , export2 as alias2 , [...] } from "module-name";
import defaultExport, { export [ , [...] ] } from "module-name";
import defaultExport, * as name from "module-name";
import "module-name";
var promise = import("module-name");
Example:
es6-file1.js
// Syntax: import * as name from "Module-name or File-path";
import * as myModule from './es6-module-file.js';
console.log( myModule.HELLO ); // Hello Everybody
console.log( myModule.BYE ); // Goodbye!
myModule.sayHello("Tom") ; // Hello Tom
myModule.sayGoodbye("Tom") ;// Goodbye Tom
Example:
es6-file2.js
// Syntax: import { export1 , export2 } from "Module-name or File-path";
import {sayHello, HELLO} from './es6-module-file.js';
console.log( HELLO ); // Hello Everybody
sayHello("Tom") ; // Hello Tom
Example:
es6-file3.js
// Syntax: import { export as alias } from "Module-name or File-path";
import {sayHello as mySayHello, HELLO} from './es6-module-file.js';
console.log( HELLO ); // Hello Everybody
mySayHello("Tom") ; // Hello Tom
Example:
es6-file4.js
// Syntax: import { export as alias } from "Module-name or File-path";
import {sayHello as mySayHello, HELLO} from './es6-module-file.js';
console.log( HELLO ); // Hello Everybody
mySayHello("Tom") ; // Hello Tom
Example:
es6-file5.js
// Syntax: import defaultExport, * as name from "Module-name or File-path";
import myModule, {sayHello, HELLO} from './es6-module-file.js';
console.log( HELLO ); // Hello Everybody
myModule.sayHello("Tom") ; // Hello Tom
3. NodeJS - CommonJS Module
NodeJS uses its own syntax to export and import a module. This syntaxis usually known as the "CommonJS Module Syntax". Let's look at an example with this syntax:
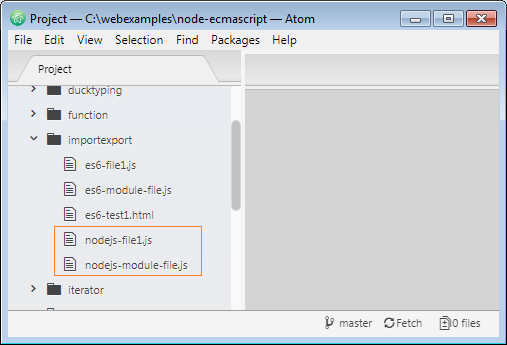
nodejs-module-file.js
// Constants
const HELLO = "Hello Everybody";
const BYE = "Goodbye!";
// Private function (Do not export this function)
function doSomething() {
console.log("Do Something");
}
// A Function
let sayHello = function(name) {
if(name) {
console.log("Hello " + name);
} else {
console.log(HELLO);
}
}
// A Function
let sayGoodbye = function(name) {
if(name) {
console.log("Goodbye " + name);
} else {
console.log(BYE);
}
}
// Export a Module (CommonJS Module Syntax)
module.exports = {HELLO, BYE, sayHello, sayGoodbye};
The nodejs-file1.js file imports some modules of the nodejs-module-file.js file:
nodejs-file1.js
// Import
var myModule = require("./nodejs-module-file.js");
console.log( myModule.HELLO ); // Hello Everybody
console.log( myModule.BYE ); // Goodbye!
myModule.sayHello("Tom") ; // Hello Tom
myModule.sayGoodbye("Tom") ;// Goodbye Tom
Run the nodejs-file1.js file directly in the NodeJS environment:
Hello Everybody
Goodbye!
Hello Tom
Goodbye Tom
4. ES6 Module in NodeJS
Many Module syntaxes are used at the same time inJavascript, for example CommonJS Module Syntax, ES6 Module Syntax,..
- NodeJS uses CommonJS Module Syntax to export and import a module. Specifically, it uses the module.exports/required() keyword instead of export/import.
- ES6 Module Syntax uses the export/import keyword to export and import one module.
Although the ES6 Module Syntax was introduced in the ECMAScript6 version (Released in 2015), NodeJS version 11 (released in October 2018 ) still does not support this syntax by default, therefore, when executing the file, Javascript uses ES Module Syntax on NodeJS. You may encounter a similar error below:
C:\webexamples\node-ecmascript\module\es6-file1.js:1
(function (exports, require, module, __filename, __dirname) { import { sayHello } from './es6-file1.js';
^^^^^^
SyntaxError: Unexpected token import
The solution here is that you need to rename the *.js file to *.mjs (Module JS). For example, I create 2 mjs files:
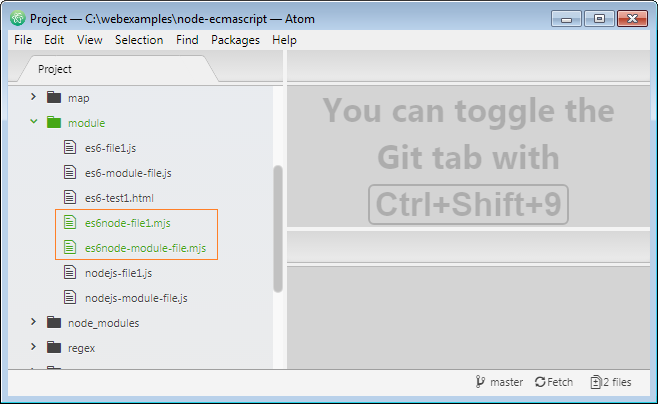
es6node-module-file.mjs
// Constants
const HELLO = "Hello Everybody";
const BYE = "Goodbye!";
// Private function (Do not export this function)
function doSomething() {
console.log("Do Something");
}
// A Function
let sayHello = function(name) {
if(name) {
console.log("Hello " + name);
} else {
console.log(HELLO);
}
}
// A Function
let sayGoodbye = function(name) {
if(name) {
console.log("Goodbye " + name);
} else {
console.log(BYE);
}
}
// Export a Module
export {HELLO, BYE, sayHello, sayGoodbye};
es6node-file1.mjs
// Import *
import * as myModule from './es6node-module-file.mjs';
console.log( myModule.HELLO ); // Hello Everybody
console.log( myModule.BYE ); // Goodbye!
myModule.sayHello("Tom") ; // Hello Tom
myModule.sayGoodbye("Tom") ;// Goodbye Tom
Open the CMD and CD windows into the directory containing the two above files and execute the following command:
node --experimental-modules es6node-file1.mjs
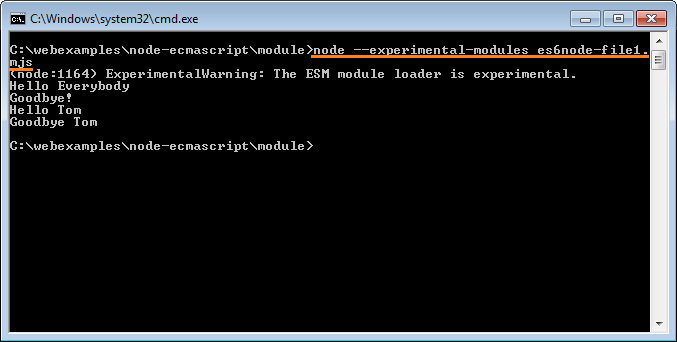
ECMAScript, Javascript Tutorials
- Introduction to Javascript and ECMAScript
- Quickstart with Javascript
- Alert, Confirm, Prompt Dialog Box in Javascript
- Quickstart with JavaScript
- JavaScript Variables Tutorial with Examples
- Bitwise Operations
- JavaScript Arrays Tutorial with Examples
- JavaScript Loops Tutorial with Examples
- JavaScript Functions Tutorial with Examples
- JavaScript Number Tutorial with Examples
- JavaScript Boolean Tutorial with Examples
- JavaScript Strings Tutorial with Examples
- JavaScript if else Statement Tutorial with Examples
- JavaScript Switch Statement
- JavaScript Error Handling Tutorial with Examples
- JavaScript Date Tutorial with Examples
- JavaScript Modules Tutorial with Examples
- The History of Modules in JavaScript
- JavaScript setTimeout and setInterval Function
- Javascript Form Validation Tutorial with Examples
- JavaScript Web Cookies Tutorial with Examples
- JavaScript void Keyword Tutorial with Examples
- Classes and Objects in JavaScript
- Class and inheritance simulation techniques in JavaScript
- Inheritance and polymorphism in JavaScript
- Undertanding Duck Typing in JavaScript
- JavaScript Symbols Tutorial with Examples
- JavaScript Set Collection Tutorial with Examples
- JavaScript Map Collection Tutorial with Examples
- Undertanding JavaScript Iterables and Iterators
- JavaScript Regular Expressions Tutorial with Examples
- JavaScript Promise, Async/Await Tutorial with Examples
- Javascript Window Tutorial with Examples
- Javascript Console Tutorial with Examples
- Javascript Screen Tutorial with Examples
- Javascript Navigator Tutorial with Examples
- Javascript Geolocation API Tutorial with Examples
- Javascript Location Tutorial with Examples
- Javascript History API Tutorial with Examples
- Javascript Statusbar Tutorial with Examples
- Javascript Locationbar Tutorial with Examples
- Javascript Scrollbars Tutorial with Examples
- Javascript Menubar Tutorial with Examples
- JavaScript JSON Tutorial with Examples
- JavaScript Event Handling Tutorial with Examples
- Javascript MouseEvent Tutorial with Examples
- Javascript WheelEvent Tutorial with Examples
- Javascript KeyboardEvent Tutorial with Examples
- Javascript FocusEvent Tutorial with Examples
- Javascript InputEvent Tutorial with Examples
- Javascript ChangeEvent Tutorial with Examples
- Javascript DragEvent Tutorial with Examples
- Javascript HashChangeEvent Tutorial with Examples
- Javascript URL Encoding Tutorial with Examples
- Javascript FileReader Tutorial with Examples
- Javascript XMLHttpRequest Tutorial with Examples
- Javascript Fetch API Tutorial with Examples
- Parsing XML in Javascript with DOMParser
- Introduction to Javascript HTML5 Canvas API
- Highlighting code with SyntaxHighlighter Javascript library
- What are polyfills in programming science?
Show More