JavaScript Map Collection Tutorial with Examples
1. Collections - Map
ECMAScript 6 introduces the 2 new data structures such as Map & Set. They are part of ECMAScript Collections Framework .
- Maps - This data structure allows you to store "Key/Value" pairs. And you can access the value through the key, or update a new value corresponding to a key.
- Sets - This data structure stores a list of elements not allowing duplicate and not indexing elements.
In this post, I will introduce the Map to you.
Map:
A Map object stores "Key/Value", of which the Key and the Value can be Primitive types or objects.
- In the Map object, keys are not iterated.
- The Map is an ordered data type, which means that the "Key/Value" pair which is added first, will come first. the second fair which will come second.
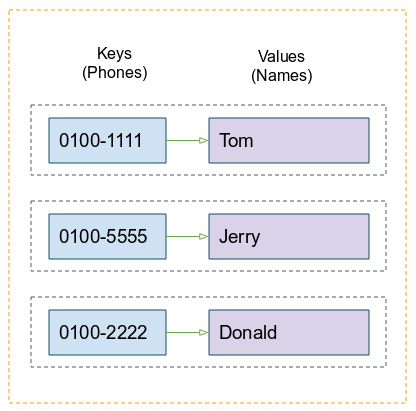
Create a Map object through the constructor of Map class:
new Map( [iteratorObject] )
Parameters:
- iteratorObject - any object which is iterable.
Properties:
Property | Description |
size | This property returns the number of "Key/Value" pairs in the Map object. |
map-size-example.js
var myContacts = new Map();
myContacts.set("0100-1111", "Tom");
myContacts.set("0100-5555", "Jerry");
myContacts.set("0100-2222", "Donald");
console.log(myContacts.size); // 3
for..of
You can use the for..of loop to loop through Map's key/value pairs.
map-for-of-loop-example.js
// Create a Map object.
var myContacts = new Map();
myContacts.set("0100-1111", "Tom");
myContacts.set("0100-5555", "Jerry");
myContacts.set("0100-2222", "Donald");
for( let arr of myContacts) {
console.log(arr);
console.log(" - Phone: " + arr[0]);
console.log(" - Name: " + arr[1]);
}
Output:
[ '0100-1111', 'Tom' ]
- Phone: 0100-1111
- Name: Tom
[ '0100-5555', 'Jerry' ]
- Phone: 0100-5555
- Name: Jerry
[ '0100-2222', 'Donald' ]
- Phone: 0100-2222
- Name: Donald
2. Map Methods
set(key, value)
The set(key, newValue) method will add a key/newValue pair to Map object if no pair with such key exists, on the contrary, it will update a new value for the key/value pair found in the Map.
map-set-example.js
var data = [
["0100-1111", "Tom"],
["0100-5555", "Jerry"],
["0100-2222", "Donald"]
];
var myContacts = new Map(data);
console.log(myContacts);
// Add new Key/Value pair to Map
myContacts.set("0100-9999", "Mickey");
console.log(myContacts);
// Update
myContacts.set("0100-5555", "Bugs Bunny");
console.log(myContacts);
Output:
Map {
'0100-1111' => 'Tom',
'0100-5555' => 'Jerry',
'0100-2222' => 'Donald' }
Map {
'0100-1111' => 'Tom',
'0100-5555' => 'Jerry',
'0100-2222' => 'Donald',
'0100-9999' => 'Mickey' }
Map {
'0100-1111' => 'Tom',
'0100-5555' => 'Bugs Bunny',
'0100-2222' => 'Donald',
'0100-9999' => 'Mickey' }
has(key)
This method tests whether a key exists in the Map or not. It returns true if existing, on the contrary, it returns false.
map-has-example.js
var data = [
["0100-1111", "Tom"],
["0100-5555", "Jerry"],
["0100-2222", "Donald"]
];
var myContacts = new Map(data);
var has = myContacts.has("0100-5555");
console.log("Has key 0100-5555? " + has); // true
clear()
Remove all "Key/Value" pairs out of the Map object.
map-clear-example.js
var data = [
["0100-1111", "Tom"],
["0100-5555", "Jerry"],
["0100-2222", "Donald"]
];
var myContacts = new Map(data);
console.log("Size: " + myContacts.size); // 3
myContacts.clear();
console.log("Size after clearing: " + myContacts.size); // 0
delete(key)
Remove a "Key/Value" pair out of the Map object, return true if a pair is removed out of the Map, return false if this key doesn't exist in the Map.
map-delete-example.js
var data = [
["0100-1111", "Tom"],
["0100-5555", "Jerry"],
["0100-2222", "Donald"]
];
var myContacts = new Map(data);
console.log("Size: " + myContacts.size); // 3
var deleted = myContacts.delete("0100-5555");
console.log("Deleted? " + deleted); // true
console.log("Size after delete: " + myContacts.size); // 2
entries()
Return the Iterator, of which each entry contains an array with the 2 elements such as [key, value]. The order of entries is still kept unchanged, like the one of the Key/Value pairs in the Map object. (See the following illustration)
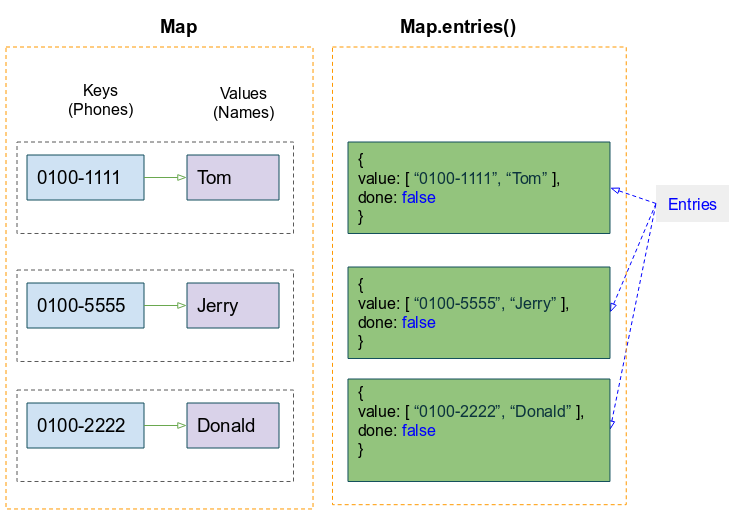
map-entries-example.js
var myContacts = new Map();
myContacts.set("0100-1111", "Tom");
myContacts.set("0100-5555", "Jerry");
myContacts.set("0100-2222", "Donald");
var entries = myContacts.entries();
var entry;
while( !(entry = entries.next()).done ) {
var array = entry.value;
console.log(array); // [ '0100-1111', 'Tom' ]
}
Output:
[ '0100-1111', 'Tom' ]
[ '0100-5555', 'Jerry' ]
[ '0100-2222', 'Donald' ]
keys()
This method returns a new Iterator object helping you access Map keys.
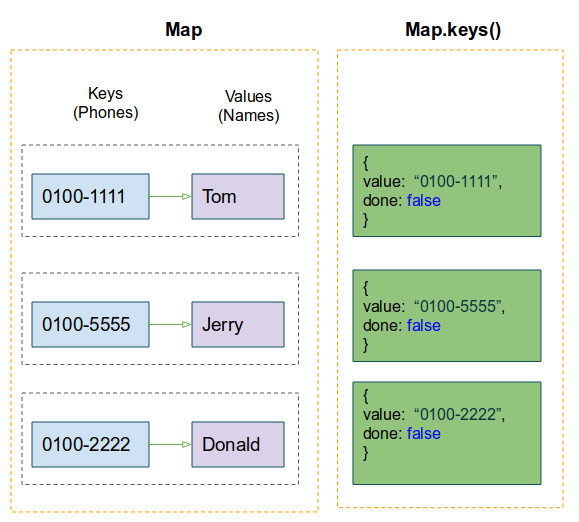
map-keys-example.js
var data = [
["0100-1111", "Tom"],
["0100-5555", "Jerry"],
["0100-2222", "Donald"]
];
var myContacts = new Map(data);
var iteratorPhones= myContacts.keys();
var entry;
while( !(entry = iteratorPhones.next()).done ) {
var phone = entry.value;
console.log(phone); // 0100-1111
}
Output:
0100-1111
0100-5555
0100-2222
values()
This method returns a new Iterator object helping you access Map values.
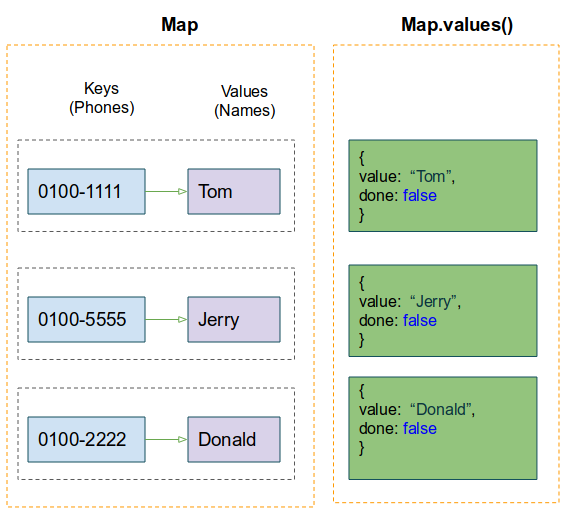
map-values-example.js
var data = [
["0100-1111", "Tom"],
["0100-5555", "Jerry"],
["0100-2222", "Donald"]
];
var myContacts = new Map(data);
var iteratorNames = myContacts.values();
var entry;
while( !(entry = iteratorNames.next()).done ) {
var name = entry.value;
console.log(name); // Tom
}
forEach(callbackFn [, thisArg])
This method will call the callbackFn function one time corresponding to each "Key/Value" pair of the Map object.
myMap.forEach(callback[, thisArg])
Parameters:
- callbackFn -This function will be called one time corresponding to each "Key/Value" pair of the Map object.
- thisArg - The parameter is used like this when executingcallbackFn.
map-forEach-example.js
var showContact = function(key, value, thisMap) {
console.log("Phone: " + key +". Name: " + value);
}
var data = [
["0100-1111", "Tom"],
["0100-5555", "Jerry"],
["0100-2222", "Donald"]
];
var myContacts = new Map(data);
// or call: myContacts.forEach(showContact)
myContacts.forEach(showContact, myContacts);
Output:
Phone: 0100-1111. Name: Tom
Phone: 0100-5555. Name: Jerry
Phone: 0100-2222. Name: Donald
3. WeakMap
Basically, the WeakMap is quite the same as Map, but it has the following differences:
- Its keys have to be objects
- The keys of WeakMap can be eliminated in the garbage collection process, which is an independent process to remove objects that are no longer used in the program.
- WeakMap doesn't support property: size, therefore, you can not how many elements it has.
- Many methods may be available in the Map class but there is no WeakMap class, for example,values(), keys(), entries(), clear(),..
Note: You can not use the for..of loop for the WeakMap, and there is no way for you to iterate over the key/value fairs of the WeakMap.
Create a WeakMap object.
var map2 = new WeakMap( [iteratorObject] )
Parameters:
- iteratorObject - any object which is iterable.
Methods:
The quantity of WeakMap methods is less than the quantity of the Map methods:
- WeakMap.delete(key)
- WeakMap.get(key)
- WeakMap.has(key)
- WeakMap.set(key, value)
The keys in the WeakMap have to be an object. It can not be a Primitive type.
let w = new WeakMap();
w.set('a', 'b'); // Uncaught TypeError: Invalid value used as weak map key
let m = new Map();
m.set('a', 'b'); // Works
weakmap-example.js
var key1 = {}; // An Object
var key2 = {foo: "bar"};
var key3 = {bar: "foo"};
var data = [
[key1, "Tom"],
[key2, "Jerry"],
[key3, "Donald"]
];
var myWeakMap = new WeakMap(data);
console.log(myWeakMap.get(key1));
ECMAScript, Javascript Tutorials
- Introduction to Javascript and ECMAScript
- Quickstart with Javascript
- Alert, Confirm, Prompt Dialog Box in Javascript
- Quickstart with JavaScript
- JavaScript Variables Tutorial with Examples
- Bitwise Operations
- JavaScript Arrays Tutorial with Examples
- JavaScript Loops Tutorial with Examples
- JavaScript Functions Tutorial with Examples
- JavaScript Number Tutorial with Examples
- JavaScript Boolean Tutorial with Examples
- JavaScript Strings Tutorial with Examples
- JavaScript if else Statement Tutorial with Examples
- JavaScript Switch Statement
- JavaScript Error Handling Tutorial with Examples
- JavaScript Date Tutorial with Examples
- JavaScript Modules Tutorial with Examples
- The History of Modules in JavaScript
- JavaScript setTimeout and setInterval Function
- Javascript Form Validation Tutorial with Examples
- JavaScript Web Cookies Tutorial with Examples
- JavaScript void Keyword Tutorial with Examples
- Classes and Objects in JavaScript
- Class and inheritance simulation techniques in JavaScript
- Inheritance and polymorphism in JavaScript
- Undertanding Duck Typing in JavaScript
- JavaScript Symbols Tutorial with Examples
- JavaScript Set Collection Tutorial with Examples
- JavaScript Map Collection Tutorial with Examples
- Undertanding JavaScript Iterables and Iterators
- JavaScript Regular Expressions Tutorial with Examples
- JavaScript Promise, Async/Await Tutorial with Examples
- Javascript Window Tutorial with Examples
- Javascript Console Tutorial with Examples
- Javascript Screen Tutorial with Examples
- Javascript Navigator Tutorial with Examples
- Javascript Geolocation API Tutorial with Examples
- Javascript Location Tutorial with Examples
- Javascript History API Tutorial with Examples
- Javascript Statusbar Tutorial with Examples
- Javascript Locationbar Tutorial with Examples
- Javascript Scrollbars Tutorial with Examples
- Javascript Menubar Tutorial with Examples
- JavaScript JSON Tutorial with Examples
- JavaScript Event Handling Tutorial with Examples
- Javascript MouseEvent Tutorial with Examples
- Javascript WheelEvent Tutorial with Examples
- Javascript KeyboardEvent Tutorial with Examples
- Javascript FocusEvent Tutorial with Examples
- Javascript InputEvent Tutorial with Examples
- Javascript ChangeEvent Tutorial with Examples
- Javascript DragEvent Tutorial with Examples
- Javascript HashChangeEvent Tutorial with Examples
- Javascript URL Encoding Tutorial with Examples
- Javascript FileReader Tutorial with Examples
- Javascript XMLHttpRequest Tutorial with Examples
- Javascript Fetch API Tutorial with Examples
- Parsing XML in Javascript with DOMParser
- Introduction to Javascript HTML5 Canvas API
- Highlighting code with SyntaxHighlighter Javascript library
- What are polyfills in programming science?
Show More