Javascript Navigator Tutorial with Examples
1. window.navigator
The window.navigator object contains information on user's browser, for example, browser name, version, .... You can access the navigator object without using the window prefix.
- navigator.appName
- navigator.appCodeName
- navigator.platform
- ...
2. navigator.product
navigator.product always returns "Gecko" for all browsers
var prodName = navigator.product; // Gecko
3. navigator.appName
navigator.appName returns the browser application name. But in fact it doesn't make much sense because in most cases it returns "Netscape", specifically, to different browsers:
- IE11+, Firefox, Chrome and Safari returns "Netscape".
- IE 10 and earlier versions return "Microsoft Internet Explorer".
- Opera returns "Opera".
var appName = navigator.appName;
4. navigator.appCodeName
navigator.appCodeName returns the browser code name. But it doesn't make much sense because all browsers return the "Mozilla" value, which implies that this browser is compatible with Mozilla.
var codeName = navigator.appCodeName; // Mozilla
5. navigator.appVersion
navigator.appVersion returns browser version information.
appVersion-example.html
<!DOCTYPE html>
<html>
<head>
<title>Navigator appVersion</title>
<meta charset="UTF-8">
<style>textarea {width:100%;margin-top:10px;}</style>
</head>
<body>
<h1>navigator.appVersion</h1>
<button onClick="showInfos()">Show Infos</button>
<textarea name="name" rows="5" id="log-area"></textarea>
<script>
function showInfos() {
var logArea = document.getElementById("log-area");
logArea.value = navigator.appVersion;
}
showInfos();
</script>
</body>
</html>
Run the example on the Chrome (Windows 7) browser and receive the result:
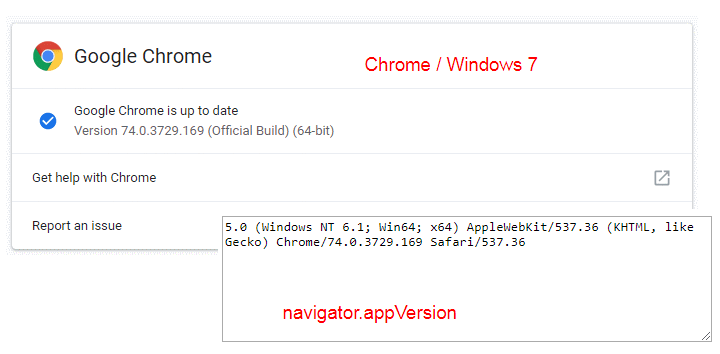
Run the example on Firefox (Windows 7) browser and receive the result:
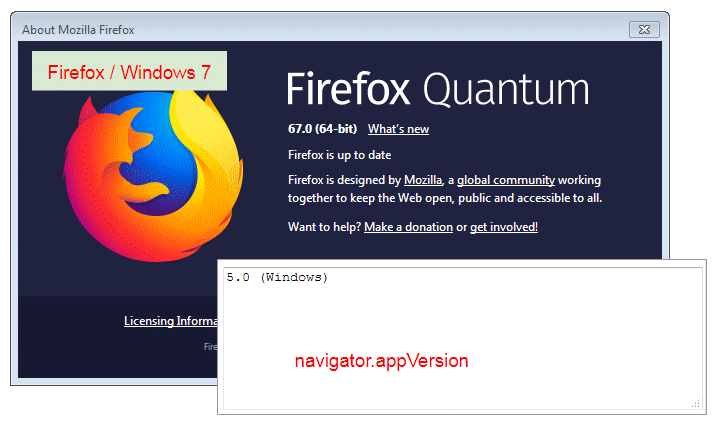
6. navigator.platform
navigator.platform returns platform name on which the browser is compiled. It can return a empty string if the browser refuses or cannot identify the platform. Values can be:
- HP-UX
- Linux i686
- Linux armv7l
- Mac68K
- MacPPC
- MacIntel
- SunOS
- Win16
- Win32
- WinCE
- Etc..
Most browsers, including Chrome, Edge, and Firefox 63 and later, return "Win32" even if running on a 64-bit version of Windows, Internet Explorer and versions of Firefox prior to version 63 still report "Win64".
7. navigator.cookieEnabled
navigator.cookieEnabled returns true if the browser enables Cookie, vice versa, return false.
Configure Chrome browser to turn on or turn off Cookie:
Configure Firefox browser to turn on or turn off Cookie:
See Also:
8. navigator.geolocation
navigator.geolocation returns a Geolocation object, containing user's position information such as longitude, latitude,... .This allows a website or application to offer customized results based on the user's location
Note: For security reasons, when a web page tries to access location information, the user is notified and asked to grant permission. Be aware that each browser has its own policies and methods for requesting this permission.
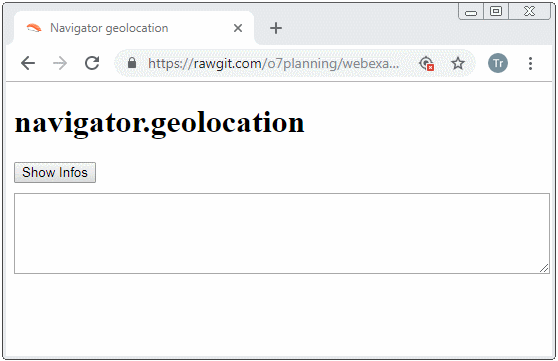
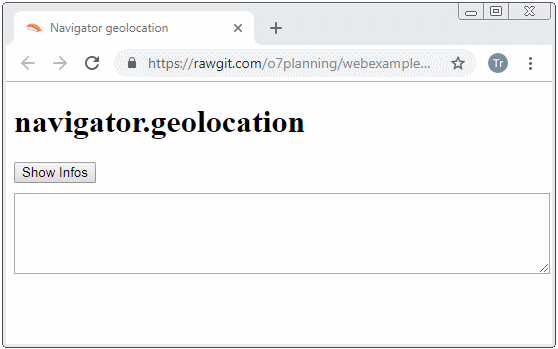
Example:
geolocation-example.js
// Success Handler
function successHandler(position) {
var logArea = document.getElementById("log-area");
logArea.value = "";
logArea.value += "Latitude: " + position.coords.latitude + "\n";
logArea.value += "Longitude: " + position.coords.longitude + "\n";
}
// Error Handler
function errorHandler(positionError) {
if(positionError.code == PositionError.PERMISSION_DENIED) {
alert("Error: Permission Denied!");
} else if(positionError.code == PositionError.POSITION_UNAVAILABLE) {
alert("Error: Position Unavailable!");
} else if(positionError.code == PositionError.TIMEOUT) {
alert("Error: Timeout!");
}
}
function showInfos() {
navigator.geolocation.getCurrentPosition(successHandler, errorHandler);
}
geolocation-example.html
<!DOCTYPE html>
<html>
<head>
<title>Navigator geolocation</title>
<meta charset="UTF-8">
<style>textarea {width:100%;margin-top:10px;}</style>
<script src="geolocation-example.js"></script>
</head>
<body>
<h1>navigator.geolocation</h1>
<button onClick="showInfos()">Show Infos</button>
<textarea name="name" rows="5" id="log-area"></textarea>
</body>
</html>
Geolocation is a pretty large API, so it needs to be introduced in a separate post. If you are interested, you can see the following post:
9. navigator.language
navigator.language returns a string representing the user's preferred language, also known as the "Browser UI" language.
var lang = navigator.language
Values can be "en", "en-US", "fr", "fr-FR", "es-ES", ... It is noted that the Safari browser on MacOS and iOS before version 10.2, country code is lowercase "en-us", "fr-fr", "es-es", ...
10. navigator.onLine
navigator.onLine returns the online status of the browser. It returns true, that is, the browser is online, on the contrary, it returns false. Its value is updated when a user accesses a link or a request is sent to the server by JavaScript.
var online = navigator.onLine;
Different browsers may have slightly different online concepts.
In Chrome and Safari, If a browser cannot connect to a local area network (LAN) or Router, it will be offline, on the contrary, it is considered online. This means that your getting true value does not mean that you can access the Internet.
There are some situations causing you to make false judgments. For example, your computer is installed a Virtual Network. If this virtual network is always connected, navigator.onLine will return true value. Therefore, if you really want to determine browser's online status, you need to develop additional means to test. To learn more you can refer to the following post:
In Firefox (version <= 41), Internet Explorer if you change the mode of browser to offline status, navigator.onLine returns false value; on the contrary, it always returns true. For Firefox (version > 41) act is the same as Chrome and Safari.
You can see changes in network status by listening to events on window.ononline and window.onoffline.
onLine-example.html
<!DOCTYPE html>
<html>
<head>
<title>Navigator onLine</title>
<meta charset="UTF-8">
<style>textarea {width:100%;margin-top:10px;}</style>
<script>
function updateOnlineStatus(msg) {
var logArea = document.getElementById("log-area");
logArea.value += msg +"\n";
logArea.value += "navigator.onLine = " + navigator.onLine +"\n";
logArea.value += "---------------------\n";
}
function loaded() {
updateOnlineStatus("Load Event");
document.body.addEventListener("offline", function () {
updateOnlineStatus("Offline Event")
}, false);
document.body.addEventListener("online", function () {
updateOnlineStatus("Online Event")
}, false);
}
</script>
</head>
<body onload = "loaded()">
<h1>navigator.onLine</h1>
<textarea rows="8" id="log-area"></textarea>
</body>
</html>
11. navigator.userAgent
Everywhen a browser sends a request to a server, it attaches a piece of information on Header which isuser-agent. The User Agent provides the server a short information on the user's browser and operating system.
var userAgent = navigator.userAgent;
General structure of the User Agent string:
appCodeName/appVersion number (Platform; Security; OS-or-CPU; Localization; rv: revision-version-number) product/productSub Application-Name Application-Name-version
For example, this is User Agent string sent to the server from the Firefox browser which is running on the Windows 7 operating system:
Firefox/Windows 7
Mozilla/5.0 (Windows NT 6.1; WOW64; rv:12.0) Gecko/20100101 Firefox/12.0
The User Agent of the Internet Explorer 9 browser runs on the Windows 7 operating system:
IE9/Windows 7
Mozilla/5.0 (compatible; MSIE 9.0; Windows NT 6.1; WOW64; Trident/5.0)
The User Agent string of the Chrome browser runs on the Windows 7 operating system:
Chrome/Windows 7
Mozilla/5.0 (Windows NT 6.1; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/74.0.3729.169 Safari/537.36
You also can visit the following address to view the User Agent of your browser:
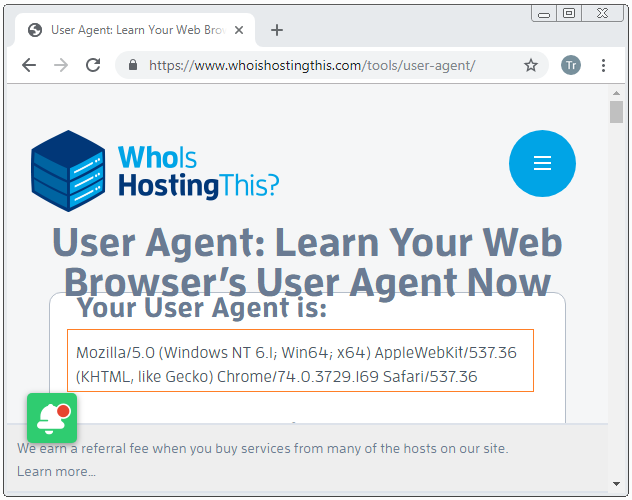
Browser identification based on the detection of the User Agent string is unreliable and not encouraged because users can customize it. Example:
- For Firefox browser, you can customize User Agent by amending general.useragent.override in about:config.
- For Opera 6+ you can set browser identification string through Menu.
- For Internet Explorer you can change User Agent via Windows Registry.
- ....
Using JavaScript, you also can customize the User Agent given to server.
JavaScript
navigator.__defineGetter__('userAgent', function() {
return "Mozilla/5.0 (Windows NT 6.2; WOW64; rv:28.0) Gecko/20100101 Firefox/28.0)"
});
jQuery
$.ajaxSetup({
beforeSend: function(request) {
request.setRequestHeader("User-Agent",
"Mozilla/5.0 (Windows NT 6.2; WOW64; rv:28.0) Gecko/20100101 Firefox/28.0)");
}
});
12. navigator.javaEnabled()
navigator.javaEnabled() returns true value, that means this browser enables to allow Java applications to operate, for example, Java Applet. You can enable or disable it through browser options.
Basically when the browser finds out that a Java application prepares to be run, it will request a permission from a user.
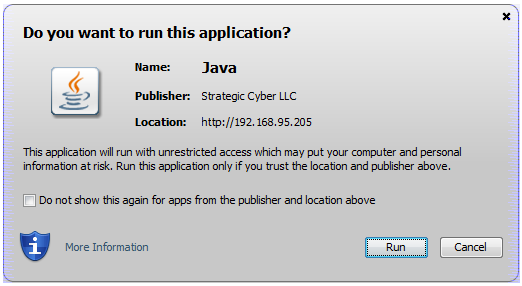
ECMAScript, Javascript Tutorials
- Introduction to Javascript and ECMAScript
- Quickstart with Javascript
- Alert, Confirm, Prompt Dialog Box in Javascript
- Quickstart with JavaScript
- JavaScript Variables Tutorial with Examples
- Bitwise Operations
- JavaScript Arrays Tutorial with Examples
- JavaScript Loops Tutorial with Examples
- JavaScript Functions Tutorial with Examples
- JavaScript Number Tutorial with Examples
- JavaScript Boolean Tutorial with Examples
- JavaScript Strings Tutorial with Examples
- JavaScript if else Statement Tutorial with Examples
- JavaScript Switch Statement
- JavaScript Error Handling Tutorial with Examples
- JavaScript Date Tutorial with Examples
- JavaScript Modules Tutorial with Examples
- The History of Modules in JavaScript
- JavaScript setTimeout and setInterval Function
- Javascript Form Validation Tutorial with Examples
- JavaScript Web Cookies Tutorial with Examples
- JavaScript void Keyword Tutorial with Examples
- Classes and Objects in JavaScript
- Class and inheritance simulation techniques in JavaScript
- Inheritance and polymorphism in JavaScript
- Undertanding Duck Typing in JavaScript
- JavaScript Symbols Tutorial with Examples
- JavaScript Set Collection Tutorial with Examples
- JavaScript Map Collection Tutorial with Examples
- Undertanding JavaScript Iterables and Iterators
- JavaScript Regular Expressions Tutorial with Examples
- JavaScript Promise, Async/Await Tutorial with Examples
- Javascript Window Tutorial with Examples
- Javascript Console Tutorial with Examples
- Javascript Screen Tutorial with Examples
- Javascript Navigator Tutorial with Examples
- Javascript Geolocation API Tutorial with Examples
- Javascript Location Tutorial with Examples
- Javascript History API Tutorial with Examples
- Javascript Statusbar Tutorial with Examples
- Javascript Locationbar Tutorial with Examples
- Javascript Scrollbars Tutorial with Examples
- Javascript Menubar Tutorial with Examples
- JavaScript JSON Tutorial with Examples
- JavaScript Event Handling Tutorial with Examples
- Javascript MouseEvent Tutorial with Examples
- Javascript WheelEvent Tutorial with Examples
- Javascript KeyboardEvent Tutorial with Examples
- Javascript FocusEvent Tutorial with Examples
- Javascript InputEvent Tutorial with Examples
- Javascript ChangeEvent Tutorial with Examples
- Javascript DragEvent Tutorial with Examples
- Javascript HashChangeEvent Tutorial with Examples
- Javascript URL Encoding Tutorial with Examples
- Javascript FileReader Tutorial with Examples
- Javascript XMLHttpRequest Tutorial with Examples
- Javascript Fetch API Tutorial with Examples
- Parsing XML in Javascript with DOMParser
- Introduction to Javascript HTML5 Canvas API
- Highlighting code with SyntaxHighlighter Javascript library
- What are polyfills in programming science?
Show More