JavaScript Number Tutorial with Examples
1. ECMAScript Number
In ECMAScript, the Number data type represents for a number, which can be an Integer, or a Floating-point Number.
Based on memory-based management way, Number is divided into the 2 kinds: Number Object and Number Primitive:
Number Primitive:
Normally, to create a number, you should use the Primitive syntax:
// Primitive Syntax:
var myNumber1 = 100;
var myNumber2 = 55.1;
Number primitives are managed in Common Pool, which means when you declare 2 or more Number primitives with the same value. They will point to the same memory location in the Common Pool.
The === operator is used to check if two variables point to the same address on memory or not.
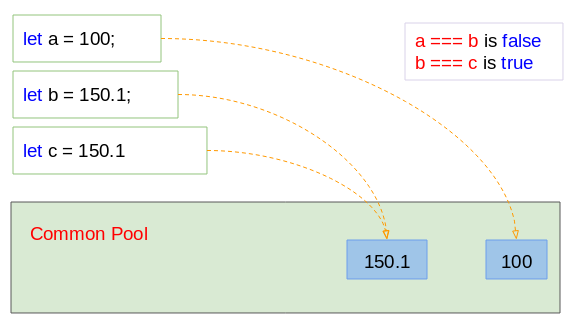
number-primitive-example1.js
let a = 100;
let b = 150.1;
let c = 150.1;
// a, b: Same address?
console.log( a === b); // false
// b, c: Same address?
console.log( b === c); // true
You can also create a number by using the Number(value) function. The result received by you is a Number primitive:
number-primitive-example2.js
let a = 100;
let b = 150.1;
// Using Number() function
let c = Number(150.1);
// a, b: Same address?
console.log( a === b); // false
// b, c: Same address?
console.log( b === c); // true
Number object:
You can create a number through the constructor of Number class. In this case, you will receive a Number object.
// Syntax:
var aNumber = new Number(number);
// Example:
var myNumber1 = new Number(100);
var myNumber2 = new Number(150.1);
The new operator always creates a new entity on the Heap memory.
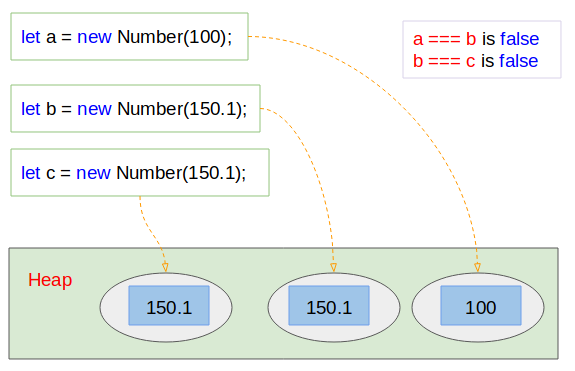
When you assign the new value which is variable aa to the variable bb . The variable bb will point to the address on memory to which the variable aa is pointing. No entity will be created on memory in this case.
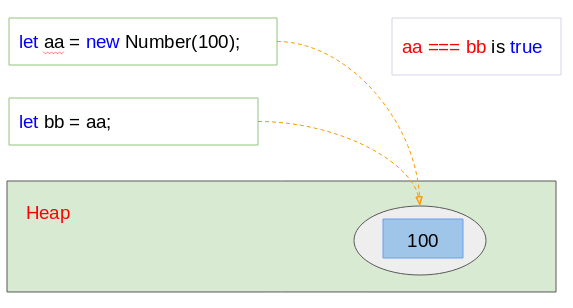
number-object-example2.js
let aa = new Number(100);
let bb = aa;
// aa, bb: Same address?
var sameAddress = aa === bb;
console.log( sameAddress); // true
Number object vs Number primitive
Number primitives are stored on the Stack (Common Pool). While Number objects are created and stored on the Heap memory, it requires a complex memory management and wastes storage space. Therefore, you should use Number primitive instead of Number object as anytime as possible.
typeof
The typeof(Number_object) operator will return the 'object' string, while the typeof(Number_primitive) will return the 'number' string:
typeof-example.js
let myNumberObject = new Number(200);
console.log(myNumberObject); // [Number: 200]
console.log( typeof(myNumberObject) ); // object
let myNumberPrimitive = 500;
console.log(myNumberPrimitive); // 500
console.log( typeof(myNumberPrimitive) ); // number
2. Compare numbers
The === operator is used to compare the addresses on memory to which two variables are pointing.
comparing-example.js
var a = new Number(100);
var b = new Number(100);
var c = 100; // Stored in Common Pool.
var d = 100; // Stored in Common Pool.
console.log( a === b); // false
console.log( c === d); // true
console.log( a === c); // false
The == operator is used to compare the values of two variables. It works perfectly for primitive data types such as Boolean (primitive), Number (primitive), String (Literal), null, undefined, NaN. But it may work not in the way you are thinking for Object data types. The Number data type in ECMAScript can be Primitive or Object, therefore, you need to be careful when using this operator.
Example: The == operator works perfectly when comparing Number Primitives:
comparing-example2.js
let a = 100;
let b = 100;
let c = 120;
console.log( a == b); // true
console.log( a == c); // false
Example: The == operator works not in the way you are think of which comparing 2 Number objects:
comparing-example3.js
let a = new Number(100);
let b = new Number(100);
let c = new Number(120);
// Are you expecting true value?
console.log( a == b); // false
console.log( a == c); // false
If you are not sure that your variable is Number object or Number primitive, you should use Number(value) function to convert it to Number primitive before using the == operator.
comparing-example3b.js
let a = new Number(100);
let b = new Number(100);
let c = new Number(120);
console.log( a == b); // false
// Use Number() function to convert Number-object to Number-literal
console.log( Number(a) == Number(b) ); // true
console.log( a == c); // false
Example: Use the == operator to compare the value of a Number primitive and the value of a Number object:
comparing-example4.js
let a = 100;
let b = new Number(100);
let c = new Number(120);
console.log( a == b); // true
console.log( a == c); // false
3. Constants
The Number class predefines a few important Constants:
- Number.EPSILON
- Number.MAX_SAFE_INTEGER
- Number.MAX_VALUE
- Number.MIN_SAFE_INTEGER
- Number.MIN_VALUE
- Number.NEGATIVE_INFINITY
- Number.NaN
- Number.POSITIVE_INFINITY
Number.EPSILON
The smallest interval between two representable numbers.
epsilon-example.js
var interval = Number.EPSILON;
console.log(interval); // 2.220446049250313e-16
Number.MAX_SAFE_INTEGER
The largest integer that the ECMAScript can represent (
2^53-1
).max-safe-integer-exampl
// 2^53 - 1
console.log( Number.MAX_SAFE_INTEGER); // 9007199254740991
console.log( Number.MAX_SAFE_INTEGER.toExponential() ); // 9.007199254740991e+15
Number.MIN_SAFE_INTEGER
The minimum interger that the ECMAScript can represent (
-(2^53-1)
).min-safe-integer-example.js
// - (2^53 - 1)
console.log( Number.MIN_SAFE_INTEGER); // -9007199254740991
console.log( Number.MIN_SAFE_INTEGER.toExponential() ); // -9.007199254740991e+15
Number.MAX_VALUE
The maximum number can be represented by the ECMAScript .
max-value-example.js
console.log( Number.MAX_VALUE); // 1.7976931348623157e+308
Number.MIN_VALUE
The minimum number can be represented by the ECMAScript .
min-value-example.js
console.log( Number.MIN_VALUE); // 5e-324
Number.NaN
Specify the "Not a number" value.
Example:
If the value of the parameter passed to the constructor of the Number by you is not a number, you will get the NaN (Not-a-Number) object. Or in other situation, if you divide a string by a nuumber, the received value is also NaN.
NaN-example.js
let a = new Number("A String");
console.log(a); // [Number: NaN]
console.log( typeof a ); // object
let b = "A String" / 100;
console.log(b); // NaN
console.log( typeof b ); // number
Number.NEGATIVE_INFINITY
Value represents a Negative infinity.
negative-infinity-example.js
let a = -1 / 0;
console.log(a); // -Infinity
console.log( Number.NEGATIVE_INFINITY ); // -Infinity
console.log( a == Number.NEGATIVE_INFINITY); // true
Number.POSITIVE_INFINITY
Value represents a Positive infinity.
positive-infinity-example.js
let a = 1 / 0;
console.log(a); // Infinity
console.log( Number.POSITIVE_INFINITY ); // Infinity
console.log( a == Number.POSITIVE_INFINITY); // true
4. Static methods
The Number class offers you some very useful static methods:
- Number.isNaN(number)
- Number.isFinite(number)
- Number.isInteger(number)
- Number.isSafeInteger(number)
- Number.parseFloat(string)
- Number.parseInt(string)
This is a static method, which helps check if the a value is NaN (Not a Number).
isNaN-example.js
console.log( Number.isNaN(10) ); // false
let a = "A String" / 100;
console.log(a); // NaN
console.log( typeof a ); // number
console.log( Number.isNaN(a) ); // true
let b = new Number("A String");
console.log(b); // [Number: NaN]
console.log( typeof b ); // object
// b is an Object, wrap NaN value!
console.log( Number.isNaN(b) ); // false (!!!)
// Convert to Number-literal:
let b2 = Number(b);
console.log( Number.isNaN( b2 )); // true
Number.isFinite(number)
This is a static method. It helps check if a value is a finite number. The method returns true/false.
isFinite-example.js
let a = 100;
console.log( Number.isFinite(a)); // true
let b = 1 / 0;
console.log( b ); // Infinite
console.log( Number.isFinite(b)); // false
let c = new Number(100);
// c is an Object
console.log( Number.isFinite(c)); // false (!!!)
// Convert c to Number-literal:
let c2 = Number(c);
console.log( Number.isFinite(c2)); // true
Number.isInteger(number)
This is a static method, that helps check if a value is an integer. The method returns true/false.
isInteger-example.js
console.log(Number.isInteger(0)); // true
console.log(Number.isInteger(1)); // true
console.log(Number.isInteger(-100000)); // true
console.log(Number.isInteger(0.1)); // false
console.log(Number.isInteger(Infinity)); // false
console.log(Number.isInteger("10")); // false
console.log(Number.isInteger(true)); // false
console.log(Number.isInteger(false)); // false
// A Number-object
let a = new Number(100);
console.log(Number.isInteger( a )); // false (!!!)
// Convert Number-object to Number-literal
let a2 = Number(a);
console.log(Number.isInteger( a2 )); // true
Number.isSafeInteger(number)
This is a static method, which helps check if a value is an integer and is in the safe range [-(2^53-1), 2^53 -1]. The method returns true/false.
isSafeInteger-example.js
let a = 100;
console.log( Number.isSafeInteger(a) ); // true
let b = Math.pow(2, 54); // 2^54
console.log( Number.isSafeInteger(b) ); // false
Number.parseFloat(string)
This is a static method, parse a string in parameters and returning a floating point number.
parseFloat-example.js
let aString1 = "100.2";
let a1 = Number.parseFloat(aString1);
console.log(a1); // 100.2
let aString2 = "Hello 100.2";
let a2 = Number.parseFloat(aString2);
console.log(a2); // NaN
Number.parseInt(string)
This is a static method, parse a string in parameters and returning an Integer.
parseInt-example.js
let aString1 = "100.2";
let a1 = Number.parseInt(aString1);
console.log(a1); // 100
let aString2 = "Hello 100.2";
let a2 = Number.parseInt(aString2);
console.log(a2); // NaN
5. Methods
- toExponential()
- toFixed(digits)
- toLocaleString()
- toPrecision(precision)
- toString(radix)
- valueOf()
toExponential()
Returns a string representing the number in exponential notation.
toExponential-example.js
var a = 510000;
console.log( a.toExponential() ); // 5.1e+5
var b = 123456789;
console.log( b.toExponential() ); // 1.23456789e+8
toFixed(digits)
This method formats a number with a specific number of digits to the right of the decimal. Method returns a String.
Parameter:
- digits: The number of digits to appear after the decimal point.
toFixed-example.js
let a = 1.234;
console.log ( a.toFixed() ); // 1
console.log ( a.toFixed(2) ); // 1.23
console.log ( a.toFixed(3) ); // 1.234
console.log ( a.toFixed(5) ); // 1.23400
toLocaleString()
This method converts a number object into a human readable string representing the number using the Locale of the environment.
toLocaleString-example.js
// This example tested on Locale VN.
let num = new Number(177.1234);
console.log( num.toLocaleString()); // 177.123
toPrecision(precision)
This method returns a string representing the number object to the specified precision.
Parameter:
- precision − An integer specifying the number of significant digits.
toPrecision-example.js
let num = 7.12345;
console.log( num.toPrecision() ); // 7.12345
console.log( num.toPrecision(1) ); // 7
console.log( num.toPrecision(2) ); // 7.1
let num2 = 7.98765;
console.log( num2.toPrecision() ); // 7.98765
console.log( num2.toPrecision(1) ); // 8
console.log( num2.toPrecision(2) ); // 8.0
The toString(radix) method returns a string representing the current number in the radix/base system specified by the parameter.
Parameter:
- radix (base): An integer between 2 and 36 specifying the base to use for representing numeric values. Default is 10.
toString-example.js
var num = new Number(10);
console.log(num.toString()); // 10
console.log(num.toString(2)); // 1010
console.log(num.toString(8)); // 12
valueOf()
This method returns the primitive value of the specified number object.
valueOf-example.js
// Number object:
let num = new Number(10);
console.log(num.valueOf()); // 10
ECMAScript, Javascript Tutorials
- Introduction to Javascript and ECMAScript
- Quickstart with Javascript
- Alert, Confirm, Prompt Dialog Box in Javascript
- Quickstart with JavaScript
- JavaScript Variables Tutorial with Examples
- Bitwise Operations
- JavaScript Arrays Tutorial with Examples
- JavaScript Loops Tutorial with Examples
- JavaScript Functions Tutorial with Examples
- JavaScript Number Tutorial with Examples
- JavaScript Boolean Tutorial with Examples
- JavaScript Strings Tutorial with Examples
- JavaScript if else Statement Tutorial with Examples
- JavaScript Switch Statement
- JavaScript Error Handling Tutorial with Examples
- JavaScript Date Tutorial with Examples
- JavaScript Modules Tutorial with Examples
- The History of Modules in JavaScript
- JavaScript setTimeout and setInterval Function
- Javascript Form Validation Tutorial with Examples
- JavaScript Web Cookies Tutorial with Examples
- JavaScript void Keyword Tutorial with Examples
- Classes and Objects in JavaScript
- Class and inheritance simulation techniques in JavaScript
- Inheritance and polymorphism in JavaScript
- Undertanding Duck Typing in JavaScript
- JavaScript Symbols Tutorial with Examples
- JavaScript Set Collection Tutorial with Examples
- JavaScript Map Collection Tutorial with Examples
- Undertanding JavaScript Iterables and Iterators
- JavaScript Regular Expressions Tutorial with Examples
- JavaScript Promise, Async/Await Tutorial with Examples
- Javascript Window Tutorial with Examples
- Javascript Console Tutorial with Examples
- Javascript Screen Tutorial with Examples
- Javascript Navigator Tutorial with Examples
- Javascript Geolocation API Tutorial with Examples
- Javascript Location Tutorial with Examples
- Javascript History API Tutorial with Examples
- Javascript Statusbar Tutorial with Examples
- Javascript Locationbar Tutorial with Examples
- Javascript Scrollbars Tutorial with Examples
- Javascript Menubar Tutorial with Examples
- JavaScript JSON Tutorial with Examples
- JavaScript Event Handling Tutorial with Examples
- Javascript MouseEvent Tutorial with Examples
- Javascript WheelEvent Tutorial with Examples
- Javascript KeyboardEvent Tutorial with Examples
- Javascript FocusEvent Tutorial with Examples
- Javascript InputEvent Tutorial with Examples
- Javascript ChangeEvent Tutorial with Examples
- Javascript DragEvent Tutorial with Examples
- Javascript HashChangeEvent Tutorial with Examples
- Javascript URL Encoding Tutorial with Examples
- Javascript FileReader Tutorial with Examples
- Javascript XMLHttpRequest Tutorial with Examples
- Javascript Fetch API Tutorial with Examples
- Parsing XML in Javascript with DOMParser
- Introduction to Javascript HTML5 Canvas API
- Highlighting code with SyntaxHighlighter Javascript library
- What are polyfills in programming science?
Show More