JavaScript Boolean Tutorial with Examples
1. ECMAScript Boolean
Boolean is the simplest data type in ECMAScript. It only has two primitive values: true and false.
Boolean-primitive
Below is the primitive syntax which is the simplest one for you to create a variable with the data type of Boolean:
boolean-primitive-example.js
let a = true;
let b = false;
console.log( a ); // true
console.log( b ); // false
Boolean Common Pool is a pool. It has only two values: true, false. When you create a variable based on the primitive syntax, it will point to the address of true or false in the pool.
The === operator is used to compare 2 addresses that two variables are pointing to.
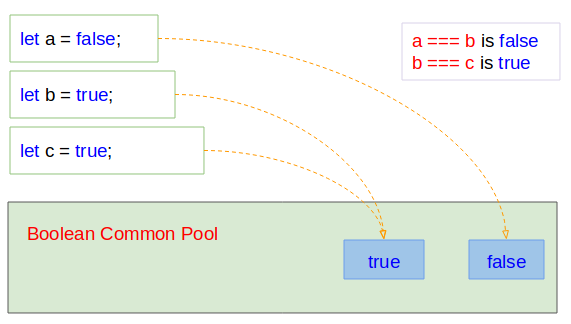
Boolean-object:
You can also create a Boolean object through the constructor of Boolean class. In essence you will create a object wrapping a primitive value: true or false .
var myObj = new Boolean(value);
Parameter:
- value: If this parameter has the value such as false, 0, "", null, undefined, NaN, Number.Infinite , it will create a Boolean {false} object. In other cases it will create Boolean {true} object.
boolean-object-example.js
console.log( new Boolean(false) ); // [Boolean: false]
console.log( new Boolean("") ); // [Boolean: false]
console.log( new Boolean(null) ); // [Boolean: false]
console.log( new Boolean() ); // [Boolean: false]
console.log( new Boolean(undefined) ); // [Boolean: false]
console.log( new Boolean(0) ); // [Boolean: false]
console.log( new Boolean(NaN) ); // [Boolean: false]
console.log( new Boolean(Number.Infinite) ); // [Boolean: false]
console.log( new Boolean(-Number.Infinite) );// [Boolean: false]
console.log(" ------------------- ");
console.log( new Boolean("0") ); // [Boolean: true]
console.log( new Boolean("false") ); // [Boolean: true]
console.log( new Boolean(1) ); // [Boolean: true]
console.log( new Boolean(100) ); // [Boolean: true]
console.log( new Boolean( {} ) ); // [Boolean: true]
Everytime you use the new operator, a new object will be created on the Heap memory. So, creating a Boolean object through the new operator will cost more to store them.
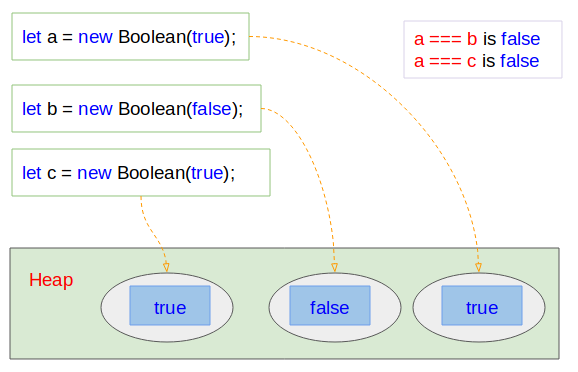
When you assign the new value which is variable aa to the variable bb. The bb variable will point to the address on memory to which the variable aa is pointing. No entity will be created on memory in this case.
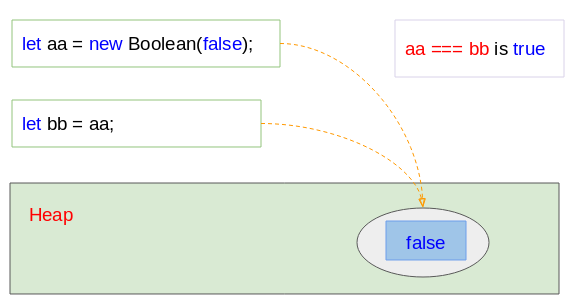
typeof
The typeof operator helps you check the type of a variable. It returns the "object" string if the variable is a Boolean object, and returns the "boolean" string if the variable is a Boolean primitive.
typeof-example.js
let a = new Boolean(true);
console.log( typeof a); // object
let b = false;
console.log( typeof b); // boolean
Boolean(..) Method
Boolean(value) function helps you to convert anything to a primitive true or false value.
- value: If this parameter has the value such as false, 0, "", null, undefined, NaN, Number.Infinite, this function will return false. In other cases, this function will return true.
Boolean-function-example.js
console.log( Boolean(false) ); // false
console.log( Boolean("") ); // false
console.log( Boolean(null) ); // false
console.log( Boolean() ); // false
console.log( Boolean(undefined) ); // false
console.log( Boolean(0) ); // false
console.log( Boolean(NaN) ); // false
console.log( Boolean(Number.Infinite) ); // false
console.log( Boolean(-Number.Infinite) );// false
console.log(" ------------------- ");
console.log( Boolean("0") ); // true
console.log( Boolean("false") ); // true
console.log( Boolean(1) ); // true
console.log( Boolean(100) ); // true
console.log( Boolean( {} ) ); // true
2. Comparison of Booleans
The === operator is used to compare the addresses pointed to of the two variables.
comparing-example.js
var a = new Boolean(true);
var b = new Boolean(true);
var c = false; // Stored in Common Pool.
var d = false; // Stored in Common Pool.
console.log( a === b); // false
console.log( c === d); // true
console.log( a === c); // false
The == operator is used to compare the values of two variables. It works perfectly for primitive data types such as Boolean (primitive), Number (primitive), String (Literal), null, undefined, NaN. But it may work not in the way like you are thinking for Object data types. Boolean data type in ECMAScript can be Primitive or Object, therefore, you need to be careful when using this operator.
Example: The == operator works perfectly when comparing Boolean primitives:
comparing-example2.js
let a = true;
let b = true;
let c = false;
console.log( a == b); // true
console.log( a == c); // false
Example: The == operator works not in the way you think when comparing 2 Boolean objects:
comparing-example3.js
let a = new Boolean(true);
let b = new Boolean(true);
let c = new Boolean(false);
// Are you expecting true value?
console.log( a == b); // false
console.log( a == c); // false
If you are not sure that your variable is a Boolean object or Boolean primitive, you should use the Boolean() function to convert it to Boolean primitive before using the == operator.
comparing-example3b.js
let a = new Boolean(true);
let b = new Boolean(true);
let c = new Boolean(false)
console.log( a == b); // false
// Use Boolean() function to convert Boolean-object to Boolean-primitive
console.log( Boolean(a) == Boolean(b) ); // true
console.log( a == c); // false
Example: Use the == operator to compare the value of a Boolean primitive and the value of a Boolean object:
comparing-example4.js
let a = true;
let b = new Boolean(true);
let c = new Boolean(false);
console.log( a == b); // true
console.log( a == c); // false
3. Methods
Boolean data type has some methods:
- toString()
- valueOf()
toString()
This method returns a string of either "true" or "false" depending upon the value of the object.
toString-example.js
let a = true;
console.log( a.toString() ); // true
let b = new Boolean(false);
console.log( b.toString() ); // false
valueOf()
This method returns the primitive value of the current Boolean object.
valueOf-example.js
let a = new Boolean(true);
console.log( a ); // [Boolean: true]
console.log( typeof a ); // object
let a2 = a.valueOf();
console.log( a2 ); // true
console.log( typeof a2 ); // boolean
ECMAScript, Javascript Tutorials
- Introduction to Javascript and ECMAScript
- Quickstart with Javascript
- Alert, Confirm, Prompt Dialog Box in Javascript
- Quickstart with JavaScript
- JavaScript Variables Tutorial with Examples
- Bitwise Operations
- JavaScript Arrays Tutorial with Examples
- JavaScript Loops Tutorial with Examples
- JavaScript Functions Tutorial with Examples
- JavaScript Number Tutorial with Examples
- JavaScript Boolean Tutorial with Examples
- JavaScript Strings Tutorial with Examples
- JavaScript if else Statement Tutorial with Examples
- JavaScript Switch Statement
- JavaScript Error Handling Tutorial with Examples
- JavaScript Date Tutorial with Examples
- JavaScript Modules Tutorial with Examples
- The History of Modules in JavaScript
- JavaScript setTimeout and setInterval Function
- Javascript Form Validation Tutorial with Examples
- JavaScript Web Cookies Tutorial with Examples
- JavaScript void Keyword Tutorial with Examples
- Classes and Objects in JavaScript
- Class and inheritance simulation techniques in JavaScript
- Inheritance and polymorphism in JavaScript
- Undertanding Duck Typing in JavaScript
- JavaScript Symbols Tutorial with Examples
- JavaScript Set Collection Tutorial with Examples
- JavaScript Map Collection Tutorial with Examples
- Undertanding JavaScript Iterables and Iterators
- JavaScript Regular Expressions Tutorial with Examples
- JavaScript Promise, Async/Await Tutorial with Examples
- Javascript Window Tutorial with Examples
- Javascript Console Tutorial with Examples
- Javascript Screen Tutorial with Examples
- Javascript Navigator Tutorial with Examples
- Javascript Geolocation API Tutorial with Examples
- Javascript Location Tutorial with Examples
- Javascript History API Tutorial with Examples
- Javascript Statusbar Tutorial with Examples
- Javascript Locationbar Tutorial with Examples
- Javascript Scrollbars Tutorial with Examples
- Javascript Menubar Tutorial with Examples
- JavaScript JSON Tutorial with Examples
- JavaScript Event Handling Tutorial with Examples
- Javascript MouseEvent Tutorial with Examples
- Javascript WheelEvent Tutorial with Examples
- Javascript KeyboardEvent Tutorial with Examples
- Javascript FocusEvent Tutorial with Examples
- Javascript InputEvent Tutorial with Examples
- Javascript ChangeEvent Tutorial with Examples
- Javascript DragEvent Tutorial with Examples
- Javascript HashChangeEvent Tutorial with Examples
- Javascript URL Encoding Tutorial with Examples
- Javascript FileReader Tutorial with Examples
- Javascript XMLHttpRequest Tutorial with Examples
- Javascript Fetch API Tutorial with Examples
- Parsing XML in Javascript with DOMParser
- Introduction to Javascript HTML5 Canvas API
- Highlighting code with SyntaxHighlighter Javascript library
- What are polyfills in programming science?
Show More