JavaScript setTimeout and setInterval Function
In the ECMAScript, setTimeout(func, time) and setInterval(func,time) are two quite similar functions. They are timed to perform a duty. In this lesson, I am going to discuss each of these functions in turn.
1. setTimeout() function
The setTimeout(func, delay) function establishes a millisecond 'delay' time period, when this time period passes the func function will be called only one time.
Note: 1 second = 1000 miliseconds
The Syntax:
setTimeout(func, delay)
- func: This function will be called after 'delay' milliseconds.
- delay: delay time period (in milisecond)
Below is the simple example with the setTimeout() function. 3 seconds after the use clicks "Show greeting", a greeting will display.
setTimeout-example.html
<!DOCTYPE html>
<html>
<head>
<title>setTimeout function</title>
<script type="text/javascript">
function greeting() {
alert("Hello Everyone!");
}
function startAction() {
setTimeout(greeting, 3000); // 3 seconds.
}
</script>
</head>
<body>
<h2>setTimeout Function</h2>
<button onclick="startAction()">Show greeting</button>
</body>
</html>
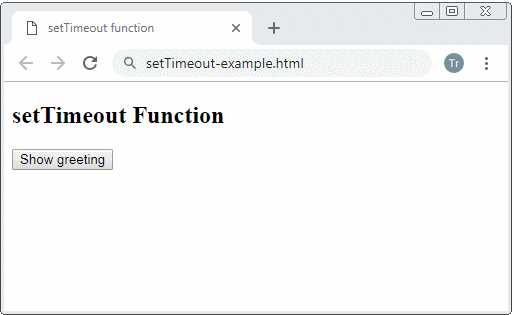
Another example with the setTimeout() function, you can run this example on the NodeJS environment:
setTimeout-example.js
console.log("3");
console.log("2");
console.log("1");
console.log("Call setTimeout!");
setTimeout( function() {
console.log("Hello Everyone!");
}, 3000); // 3 seconds
console.log("End!");
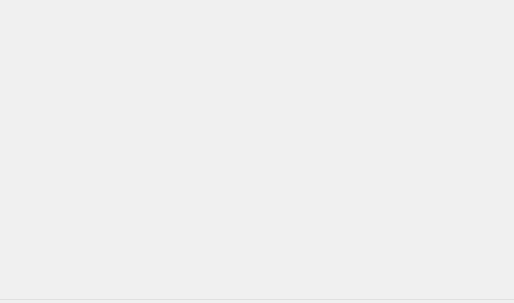
clearTimeout()
Suppose you call the setTimeout() function to schedule to perform a task, while the task is not started performing, you can cancel that task by calling the clearTimeout() function.
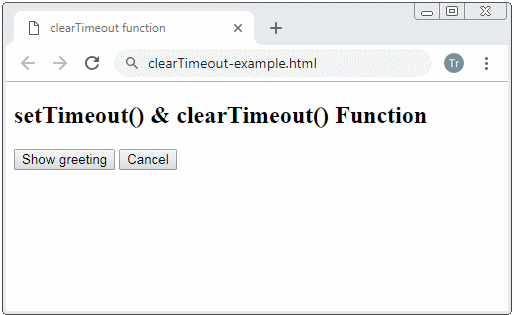
clearTimeout-example.html
<!DOCTYPE html>
<html>
<head>
<title>clearTimeout function</title>
<script type="text/javascript">
var myTask = null;
function greeting() {
alert("Hello Everyone!");
myTask = null;
}
function startAction() {
if(!myTask) {
myTask = setTimeout(greeting, 3000); // 3 seconds.
}
}
function cancelAction() {
if(myTask) {
clearTimeout(myTask);
myTask = null;
}
}
</script>
</head>
<body>
<h2>setTimeout() & clearTimeout() Function</h2>
<button onclick="startAction()">Show greeting</button>
<button onclick="cancelAction()">Cancel</button>
</body>
</html>
2. setInterval() function
The setInterval(func, delay) function establishes a milisecond 'delay' time period. After each milisecond 'delay', the func function is called.
Syntax:
setInterval(func, delay)
- func: This function will be called after each millisecond 'delay' time period.
- delay: Delay time period (in milisecond)
Below is a simple example with the setInterval() function.3 seconds after the user clicks the "Show greeting" button, a greeting will display, and it will display again after 3 seconds, ..
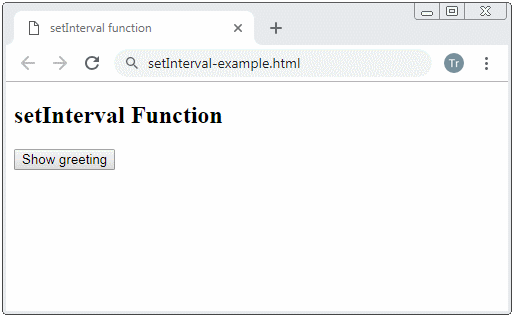
setInterval-example.html
<!DOCTYPE html>
<html>
<head>
<title>setInterval function</title>
<script type="text/javascript">
function greeting() {
alert("Hello Everyone!");
}
function startAction() {
setInterval(greeting, 3000); // 3 seconds.
}
</script>
</head>
<body>
<h2>setInterval Function</h2>
<button onclick="startAction()">Show greeting</button>
</body>
</html>
Another example with the setInterval() function, you can run this example on the NodeJS environment:
setInterval-example.js
console.log("3");
console.log("2");
console.log("1");
console.log("Call setInterval!");
setInterval( function() {
console.log("Hello Everyone!");
}, 3000); // 3 seconds
console.log("End!");
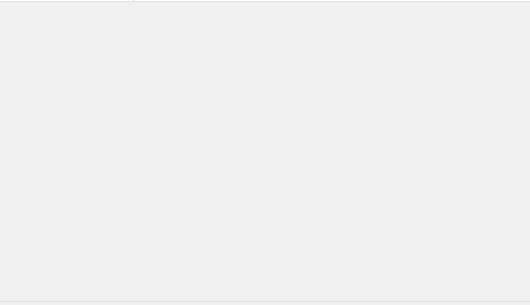
clearInterval()
Suppose you call the setInterval() function to schedule to perform a task, you can cancel that task by calling the clearInterval() function.
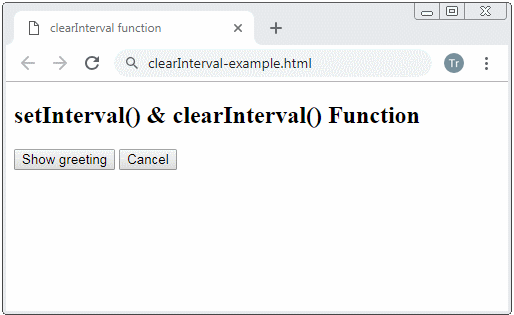
clearInterval-example.html
<!DOCTYPE html>
<html>
<head>
<title>clearInterval function</title>
<script type="text/javascript">
var myTask = null;
function greeting() {
alert("Hello Everyone!");
}
function startAction() {
if(!myTask) {
myTask = setInterval(greeting, 3000); // 3 seconds.
}
}
function cancelAction() {
if(myTask) {
clearInterval(myTask);
myTask = null;
}
}
</script>
</head>
<body>
<h2>setInterval() & clearInterval() Function</h2>
<button onclick="startAction()">Show greeting</button>
<button onclick="cancelAction()">Cancel</button>
</body>
</html>
ECMAScript, Javascript Tutorials
- Introduction to Javascript and ECMAScript
- Quickstart with Javascript
- Alert, Confirm, Prompt Dialog Box in Javascript
- Quickstart with JavaScript
- JavaScript Variables Tutorial with Examples
- Bitwise Operations
- JavaScript Arrays Tutorial with Examples
- JavaScript Loops Tutorial with Examples
- JavaScript Functions Tutorial with Examples
- JavaScript Number Tutorial with Examples
- JavaScript Boolean Tutorial with Examples
- JavaScript Strings Tutorial with Examples
- JavaScript if else Statement Tutorial with Examples
- JavaScript Switch Statement
- JavaScript Error Handling Tutorial with Examples
- JavaScript Date Tutorial with Examples
- JavaScript Modules Tutorial with Examples
- The History of Modules in JavaScript
- JavaScript setTimeout and setInterval Function
- Javascript Form Validation Tutorial with Examples
- JavaScript Web Cookies Tutorial with Examples
- JavaScript void Keyword Tutorial with Examples
- Classes and Objects in JavaScript
- Class and inheritance simulation techniques in JavaScript
- Inheritance and polymorphism in JavaScript
- Undertanding Duck Typing in JavaScript
- JavaScript Symbols Tutorial with Examples
- JavaScript Set Collection Tutorial with Examples
- JavaScript Map Collection Tutorial with Examples
- Undertanding JavaScript Iterables and Iterators
- JavaScript Regular Expressions Tutorial with Examples
- JavaScript Promise, Async/Await Tutorial with Examples
- Javascript Window Tutorial with Examples
- Javascript Console Tutorial with Examples
- Javascript Screen Tutorial with Examples
- Javascript Navigator Tutorial with Examples
- Javascript Geolocation API Tutorial with Examples
- Javascript Location Tutorial with Examples
- Javascript History API Tutorial with Examples
- Javascript Statusbar Tutorial with Examples
- Javascript Locationbar Tutorial with Examples
- Javascript Scrollbars Tutorial with Examples
- Javascript Menubar Tutorial with Examples
- JavaScript JSON Tutorial with Examples
- JavaScript Event Handling Tutorial with Examples
- Javascript MouseEvent Tutorial with Examples
- Javascript WheelEvent Tutorial with Examples
- Javascript KeyboardEvent Tutorial with Examples
- Javascript FocusEvent Tutorial with Examples
- Javascript InputEvent Tutorial with Examples
- Javascript ChangeEvent Tutorial with Examples
- Javascript DragEvent Tutorial with Examples
- Javascript HashChangeEvent Tutorial with Examples
- Javascript URL Encoding Tutorial with Examples
- Javascript FileReader Tutorial with Examples
- Javascript XMLHttpRequest Tutorial with Examples
- Javascript Fetch API Tutorial with Examples
- Parsing XML in Javascript with DOMParser
- Introduction to Javascript HTML5 Canvas API
- Highlighting code with SyntaxHighlighter Javascript library
- What are polyfills in programming science?
Show More