Javascript WheelEvent Tutorial with Examples
1. WheelEvent
WheelEvent is an interface representing for the events occurring when an user moves mouse wheel or similar equipment.
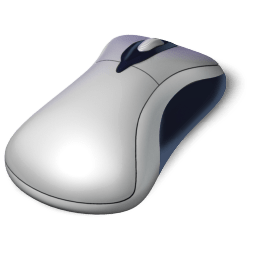
Browsers which support WheelEvent:

WheelEvent means sub-interface of MouseEvent. It will inherit all properties and methods of parent interface.
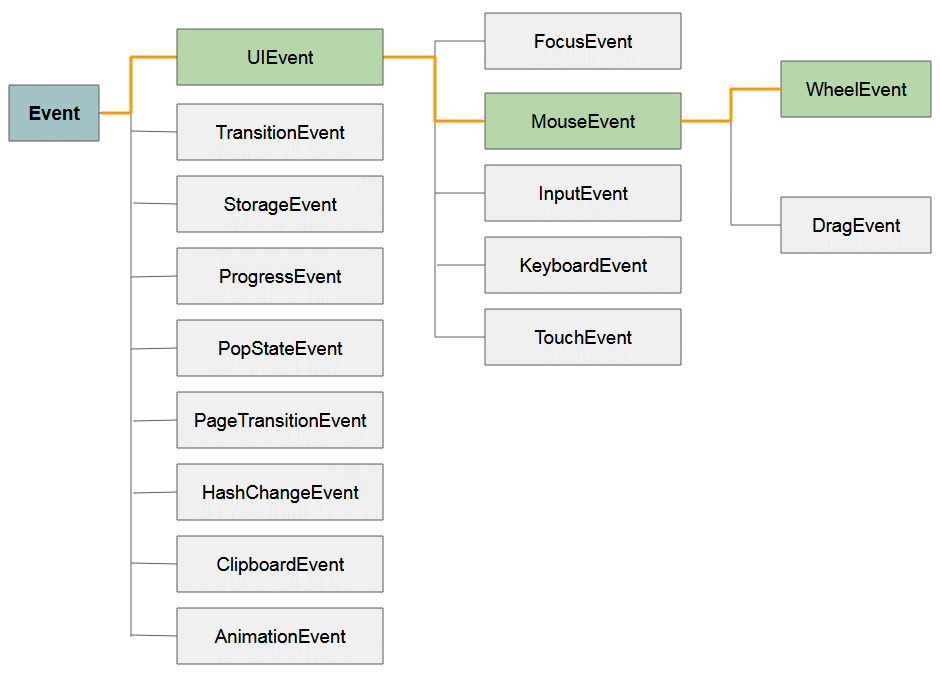
- Javascript UiEvent
- Hướng dẫn và ví dụ Javascript Event
Event | Description |
wheel | The event occurs when the mouse wheel rolls up or down over an element |
The properties of WheelEvent:
Property | Description |
deltaX | Returns the horizontal scroll amount of a mouse wheel (x-axis) |
deltaY | Returns the vertical scroll amount of a mouse wheel (y-axis) |
deltaZ | Returns the scroll amount of a mouse wheel for the z-axis |
deltaMode | Returns a number that represents the unit of measurements for delta values (DOM_DELTA_PIXEL, DOM_DELTA_PIXEL, DOM_DELTA_PAGE) |
Constants:
Constant | Value | Description |
DOM_DELTA_PIXEL | 0 | Measuring unit of delta is pixels. |
DOM_DELTA_LINE | 1 | Measuring unit of delta is lines. |
DOM_DELTA_PAGE | 2 | Measuring unit of delta is pages. |
Example with WheelEvent:
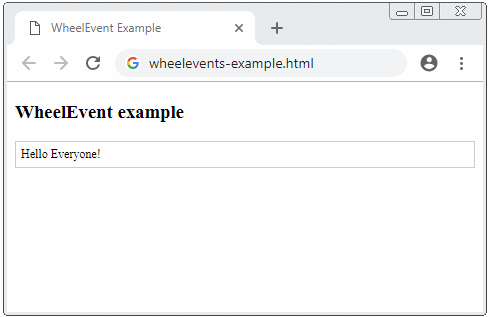
wheelevents-example.html
<!DOCTYPE html>
<html>
<head>
<title>WheelEvent Example</title>
<script src="wheelevents-example.js"></script>
</head>
<body>
<h3>WheelEvent example</h3>
<div style="font-size:12px; padding:5px; border:1px solid #ccc;"
onwheel="wheelHandler(event)">
Hello Everyone!
<div>
</body>
</html>
wheelevents-example.js
function wheelHandler(evt) {
var fontSize = evt.target.style.fontSize;// 12px, 13px,...
var value = Number(fontSize.substr(0, fontSize.length-2)); // 12, 13,..
// Scrolling up
if (evt.deltaY < 0) {
if(value < 50) {
value++;
}
}
// Scrolling down
if(evt.deltaY > 0) {
if(value > 5) {
value--;
}
}
evt.target.style.fontSize = value+"px";
}
ECMAScript, Javascript Tutorials
- Introduction to Javascript and ECMAScript
- Quickstart with Javascript
- Alert, Confirm, Prompt Dialog Box in Javascript
- Quickstart with JavaScript
- JavaScript Variables Tutorial with Examples
- Bitwise Operations
- JavaScript Arrays Tutorial with Examples
- JavaScript Loops Tutorial with Examples
- JavaScript Functions Tutorial with Examples
- JavaScript Number Tutorial with Examples
- JavaScript Boolean Tutorial with Examples
- JavaScript Strings Tutorial with Examples
- JavaScript if else Statement Tutorial with Examples
- JavaScript Switch Statement
- JavaScript Error Handling Tutorial with Examples
- JavaScript Date Tutorial with Examples
- JavaScript Modules Tutorial with Examples
- The History of Modules in JavaScript
- JavaScript setTimeout and setInterval Function
- Javascript Form Validation Tutorial with Examples
- JavaScript Web Cookies Tutorial with Examples
- JavaScript void Keyword Tutorial with Examples
- Classes and Objects in JavaScript
- Class and inheritance simulation techniques in JavaScript
- Inheritance and polymorphism in JavaScript
- Undertanding Duck Typing in JavaScript
- JavaScript Symbols Tutorial with Examples
- JavaScript Set Collection Tutorial with Examples
- JavaScript Map Collection Tutorial with Examples
- Undertanding JavaScript Iterables and Iterators
- JavaScript Regular Expressions Tutorial with Examples
- JavaScript Promise, Async/Await Tutorial with Examples
- Javascript Window Tutorial with Examples
- Javascript Console Tutorial with Examples
- Javascript Screen Tutorial with Examples
- Javascript Navigator Tutorial with Examples
- Javascript Geolocation API Tutorial with Examples
- Javascript Location Tutorial with Examples
- Javascript History API Tutorial with Examples
- Javascript Statusbar Tutorial with Examples
- Javascript Locationbar Tutorial with Examples
- Javascript Scrollbars Tutorial with Examples
- Javascript Menubar Tutorial with Examples
- JavaScript JSON Tutorial with Examples
- JavaScript Event Handling Tutorial with Examples
- Javascript MouseEvent Tutorial with Examples
- Javascript WheelEvent Tutorial with Examples
- Javascript KeyboardEvent Tutorial with Examples
- Javascript FocusEvent Tutorial with Examples
- Javascript InputEvent Tutorial with Examples
- Javascript ChangeEvent Tutorial with Examples
- Javascript DragEvent Tutorial with Examples
- Javascript HashChangeEvent Tutorial with Examples
- Javascript URL Encoding Tutorial with Examples
- Javascript FileReader Tutorial with Examples
- Javascript XMLHttpRequest Tutorial with Examples
- Javascript Fetch API Tutorial with Examples
- Parsing XML in Javascript with DOMParser
- Introduction to Javascript HTML5 Canvas API
- Highlighting code with SyntaxHighlighter Javascript library
- What are polyfills in programming science?
Show More