Javascript Window Tutorial with Examples
1. What is BOM?
Brower Object Model - BOM includes properties, methods provided by brower so that JavaScript can interact with the browser.
Many browsers exist at the same time such as Firefox, Chrome, IE, Opera,.. Each browser has its own standard, therefore, BOMs are slightly different for different browsers. There is no official standard for BOM. Fortunately, new browsers gradually support the widely used standards.
Let's see how to take information about width and height of the browser window to see the difference between browsers:
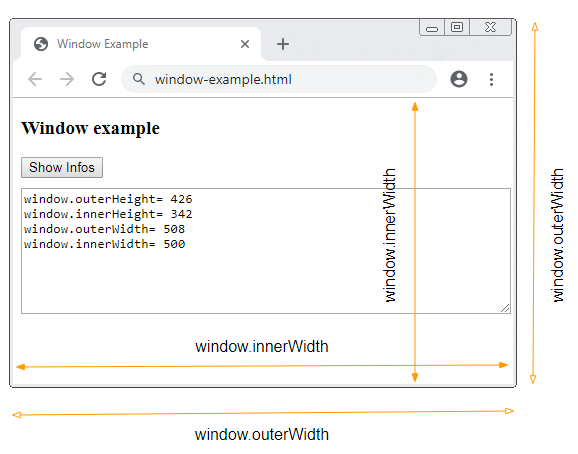
window.innerWidth is supported by the browsers such as Firefox, Chrome, Opera, IE 9+ but not by the IE 8 browser (or older), and you have to use document.documentElement.clientWidth or document.body.clientWidth.
2. Window object
The window object is the most important object in BOM. It represents the browser window and is supported by all browsers. All global Javascript objects, global functions, global variables are the members of the window object.
For example, document is a global object. It is an property of the window object. You can access it via window.document syntax or simply document.
var element = window.document.getElementById("someId");
// Same as:
var element = document.getElementById("someId");
The alert("something") function is a global function. It is a member of the window object. Therefore, you can use it under the following ways:
alert("Hello! I am an alert box!!");
// Same as:
window.alert("Hello! I am an alert box!!");
If you create a global variable, or a global function, they are members of the window object. See example:
global-func-variable-example.html
<!DOCTYPE html>
<html>
<head>
<title>Window Example</title>
<meta charset="UTF-8">
<script>
// A Global Variable
var COPY_RIGHT = "o7planning.org";
// A Global Function
function sayHello() {
alert("Hello Everyone!");
}
</script>
</head>
<body>
<h2>Global Variable, Global Function</h2>
<button onClick="alert(COPY_RIGHT)">Call alert(COPY_RIGHT)</button>
<button onClick="alert(window.COPY_RIGHT)">Call alert(window.COPY_RIGHT)</button>
<br><br>
<button onClick="sayHello()">Call sayHello()</button>
<button onClick="window.sayHello()">Call window.sayHello()</button>
</body>
</html>
3. Position, size, ..
Properties for determining size, position, ... of browser window:
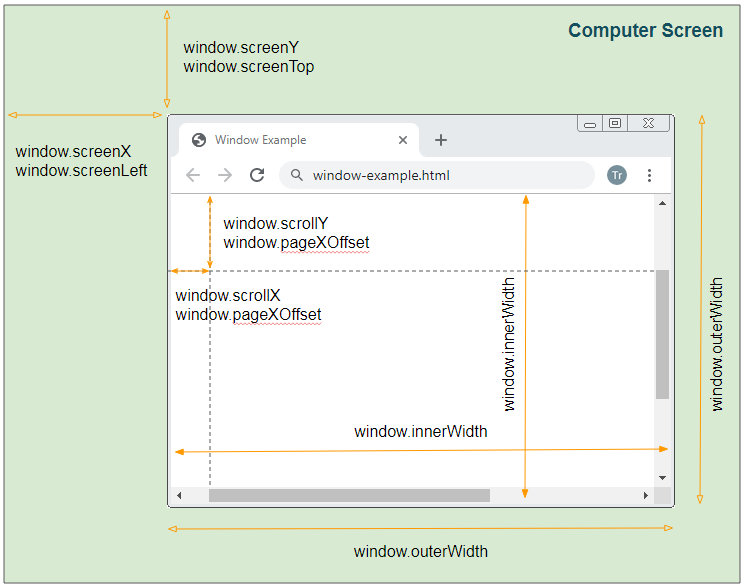
Property | Read Only | Description |
window.innerHeight | Y | Gets the height of the content area of the browser window including, if rendered, the horizontal scrollbar. |
window.innerWidth | Y | Gets the width of the content area of the browser window including, if rendered, the vertical scrollbar. |
window.outerHeight | Y | Gets the height of the outside of the browser window. |
window.outerWidth | Y | Gets the width of the outside of the browser window. |
window.screenX
window.screenLeft | Y | Both properties return the horizontal distance from the left border of the user's browser viewport to the left side of the screen. |
window.screenY
window.screenTop | Y | Both properties return the vertical distance from the top border of the user's browser viewport to the top side of the screen. |
window.scrollX
window.pageXOffset | Y | Returns the number of pixels that the document has already been scrolled horizontally. |
window.scrollY
window.pageYOffset | Y | Returns the number of pixels that the document has already been scrolled vertically. |
Example:
window-example.html
<!DOCTYPE html>
<html>
<head>
<title>Window Example</title>
<meta charset="UTF-8">
</head>
<body>
<h3>Window example</h3>
<button onClick="showInfos()">Show Infos</button>
<textarea name="name" style="width:100%;margin-top:10px;"
rows="8" id="log-area"></textarea>
<script>
function showInfos() {
var logArea = document.getElementById("log-area");
logArea.value = "";
logArea.value += "window.outerHeight= " + window.outerHeight +"\n";
logArea.value += "window.innerHeight= " + window.innerHeight +"\n";
logArea.value += "window.outerWidth= " + window.outerWidth +"\n";
logArea.value += "window.innerWidth= " + window.innerWidth +"\n";
logArea.value += "window.screenX= " + window.screenX +"\n";
logArea.value += "window.screenY= " + window.screenY +"\n";
logArea.value += "window.scrollX= " + window.scrollX +"\n";
logArea.value += "window.scrollY= " + window.scrollY +"\n";
}
showInfos();
</script>
</body>
</html>
Methods help change the size, position, .. of the browser window:
4. window.status
The status property of the window object help you establish a content of text shown on he status bar (The bottom of the browser). However, for security reasons, most browsers disable this feature for JavaScript. However, if an user wants, they are able to enable this feature for JavaScript by entering the "Options" of the browser.
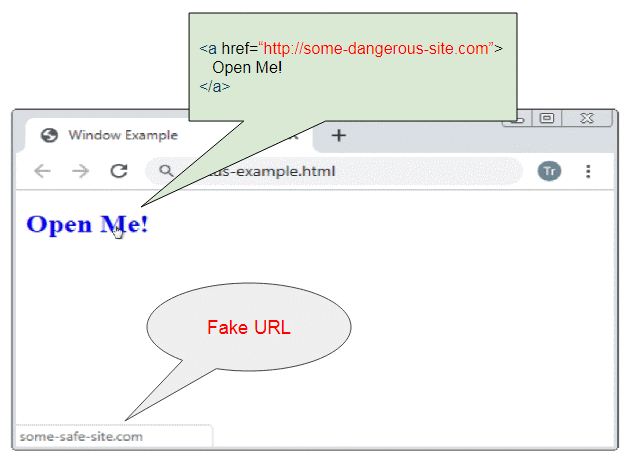
Before clicking on a link, users often move the mouse over the link's surface to preview its address displayed in the status bar, and only click this link when they feel safe. Some websites may take advantage of window.status to display a fake content.
5. Window, Frame
A page may contain Frames, and a Frame may contain other Frames, which form a hierarchy of Frames.
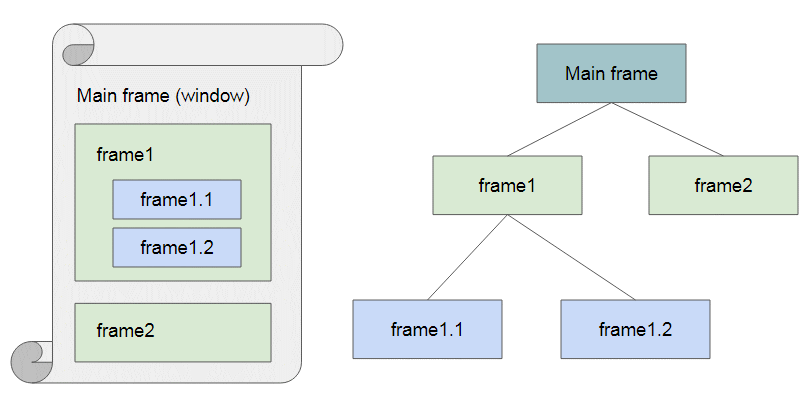
Property | Read Only | Description |
window.name | Gets/sets the name of the window. | |
window.frameElement | Y | Returns the element in which the window is embedded, or null if the window is not embedded. |
window.frames | Y | Returns an array of the frames in the current window. |
window.length | Y | Returns the number of frames in the window. See also window.frames. |
window.parent | Y | Returns a reference to the parent of the current window or subframe. |
window.self | Y | Returns an object reference to the window object itself. |
window.opener | Y | Returns a reference to the window that opened this current window. |
window[0],
window[1], etc. | Y | Returns a reference to the window object in the frames. See window.frames for more details. |
window.top | Y | Returns a reference to the topmost window in the window hierarchy. This property is read only. |
window.frameElement
Example with window.frameElement:
frameElement-example.html
<!DOCTYPE html>
<html>
<head>
<title>window.frameElement</title>
<meta charset="UTF-8">
<style>iframe {height: 120px; width: 100%}</style>
</head>
<body>
<h3>Main Frame</h3>
<a href="">Reset</a>
<p>window.frameElement example</p>
<p style="color:red;">Note: You need to test this example on a http server. </p>
<iframe src="frame-a.html"><iframe>
</body>
</html>
frame-a.html
<!DOCTYPE html>
<html>
<head>
<title>frame-a.html</title>
<meta charset="UTF-8">
<script>
function highlightIFrame() {
// <iframe> element.
var iframeElement = window.frameElement;
if(iframeElement) {
iframeElement.style.border="1px solid red";
}
}
</script>
</head>
<body>
<h2>frame-a.html</h2>
<button onClick="highlightIFrame()">Highlight IFrame</button>
</body>
</html>
6. Others
Property | Read Only | Description |
window.document | Y | Returns a reference to the document that the window contains. |
window.location | Y | Returns a reference to the location object containing the URL information of the document. |
window.locationbar | Y | Returns the locationbar object, whose visibility can be toggled in the window. |
window.personalbar | Y | Returns the personalbar object, whose visibility can be toggled in the window. |
window.scrollbars | Y | Returns the scrollbars object, whose visibility can be toggled in the window. |
window.menubar | Y | Returns the menubar object, whose visibility can be toggled in the window. |
window.navigator | Y | Returns a reference to the navigator object. |
window.screen | Y | Returns a reference to the screen object associated with the window. |
window.statusbar | Y | Returns the statusbar object, whose visibility can be toggled in the window. |
window.toolbar | Y | Returns the toolbar object, whose visibility can be toggled in the window. |
window.visualViewport | Y | Returns a VisualViewport object which represents the visual viewport for a given window. |
Property | Read Only | Description |
window.console | Y | Returns the reference to the console object, which helps programmers debug the application. |
window.localStorage | Y | Returns a reference to the local storage object, which contains special data, that only the origin of this data can access the data it creates. |
window.sessionStorage | Y | Returns a reference to the session storage object, containing data that exists in the life of a page. If the page is closed, this data will be removed. |
window.history | Y | Returns a reference to the history object. |
window.customElements | Y | Returns a reference to the CustomElementRegistry object, which can be used to register new custom elements and get information about previously registered custom elements. |
window.crypto | Y | Returns the browser crypto object. |
window.performance | Y | Returns a Performance object, which includes the timing and navigation properties, each of which is an object providing performance-related data. |
window.returnValue | When a window (or a dialog) is closed, it can assign a value to window.returnValue, and other windows can use this value. |
ECMAScript, Javascript Tutorials
- Introduction to Javascript and ECMAScript
- Quickstart with Javascript
- Alert, Confirm, Prompt Dialog Box in Javascript
- Quickstart with JavaScript
- JavaScript Variables Tutorial with Examples
- Bitwise Operations
- JavaScript Arrays Tutorial with Examples
- JavaScript Loops Tutorial with Examples
- JavaScript Functions Tutorial with Examples
- JavaScript Number Tutorial with Examples
- JavaScript Boolean Tutorial with Examples
- JavaScript Strings Tutorial with Examples
- JavaScript if else Statement Tutorial with Examples
- JavaScript Switch Statement
- JavaScript Error Handling Tutorial with Examples
- JavaScript Date Tutorial with Examples
- JavaScript Modules Tutorial with Examples
- The History of Modules in JavaScript
- JavaScript setTimeout and setInterval Function
- Javascript Form Validation Tutorial with Examples
- JavaScript Web Cookies Tutorial with Examples
- JavaScript void Keyword Tutorial with Examples
- Classes and Objects in JavaScript
- Class and inheritance simulation techniques in JavaScript
- Inheritance and polymorphism in JavaScript
- Undertanding Duck Typing in JavaScript
- JavaScript Symbols Tutorial with Examples
- JavaScript Set Collection Tutorial with Examples
- JavaScript Map Collection Tutorial with Examples
- Undertanding JavaScript Iterables and Iterators
- JavaScript Regular Expressions Tutorial with Examples
- JavaScript Promise, Async/Await Tutorial with Examples
- Javascript Window Tutorial with Examples
- Javascript Console Tutorial with Examples
- Javascript Screen Tutorial with Examples
- Javascript Navigator Tutorial with Examples
- Javascript Geolocation API Tutorial with Examples
- Javascript Location Tutorial with Examples
- Javascript History API Tutorial with Examples
- Javascript Statusbar Tutorial with Examples
- Javascript Locationbar Tutorial with Examples
- Javascript Scrollbars Tutorial with Examples
- Javascript Menubar Tutorial with Examples
- JavaScript JSON Tutorial with Examples
- JavaScript Event Handling Tutorial with Examples
- Javascript MouseEvent Tutorial with Examples
- Javascript WheelEvent Tutorial with Examples
- Javascript KeyboardEvent Tutorial with Examples
- Javascript FocusEvent Tutorial with Examples
- Javascript InputEvent Tutorial with Examples
- Javascript ChangeEvent Tutorial with Examples
- Javascript DragEvent Tutorial with Examples
- Javascript HashChangeEvent Tutorial with Examples
- Javascript URL Encoding Tutorial with Examples
- Javascript FileReader Tutorial with Examples
- Javascript XMLHttpRequest Tutorial with Examples
- Javascript Fetch API Tutorial with Examples
- Parsing XML in Javascript with DOMParser
- Introduction to Javascript HTML5 Canvas API
- Highlighting code with SyntaxHighlighter Javascript library
- What are polyfills in programming science?
Show More