Undertanding Duck Typing in JavaScript
1. What is Duck Typing?
Before giving a definition of "Duck Typing", I want to talk to you about the concept of Interface in the programming language.
Interface is a concept found in several programming languages, such as Java, CSharp, ... An interface will declare its list of methods. These methods have no content (no body). ). This Implements interface must have all methods declared in the Interface with full content. (Note: I'm not referring to abstract classes here).
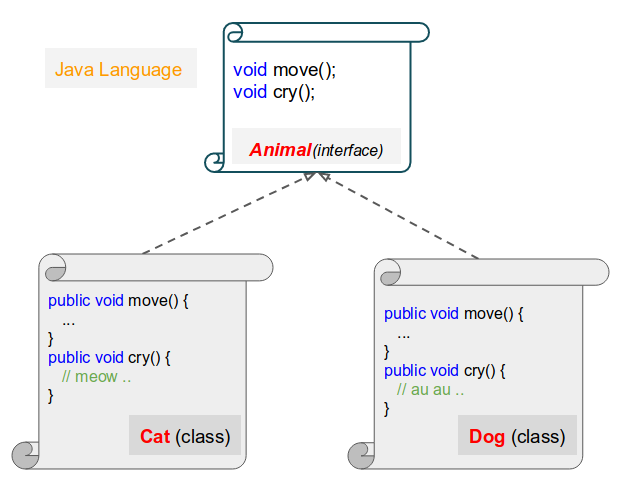
Thus, Interface and Class are two different concepts.Interface defines a standard that implements must comply with.
The languages such as Ruby, ECMAScript have no Interface concept explicitly. They have only Class concept. But "Duck Typing" can be a way for you to create something like the Interface in the ECMAScript.
Duck Typing?
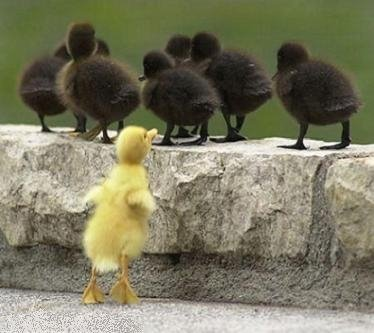
Duck Typing refers to a duck test program. You will test something, if it goes like a duck, and fly like a duck, it is duck.
Humorously, if you test an airplane, you find that it goes like a duck, it flies like a duck, so it's concluded to be a duck.
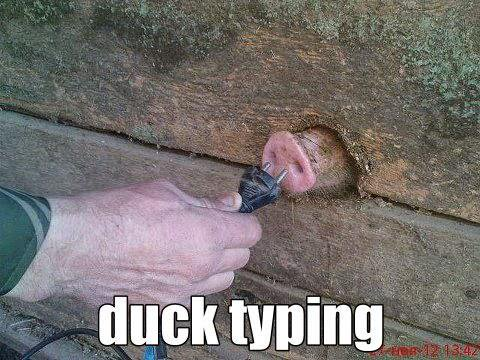
The disadvantage of Duck Typing:
Duck Typing is used in many programming languages, for example, Ruby, ECMAScript,.. and it is really useful but sometimes it creates unwanted acts in application because the rules of Duck Typing is too simple and can result in wrong conclusions. You can understand this warning more in examples.
2. Example with Duck Typing
OK, ECMAScript has no Interface concept. Below I have a Duck class. It has the 2 method such as walk() & fly().
class Duck {
fly() {
console.log("Duck fly");
}
walk() {
console.log("Duck walk");
}
}
The Airplane class also has all methods like the Duck class. According to the Duck Typing rule, you can say that Airplane belongs to theDuck type.
class Airplane {
fly() {
console.log("Airplane fly");
}
walk() {
console.log("Airplane walk");
}
shoot(target) {
console.log("Airplane shoot " + target);
}
}
The Cat class has walk() method but it has no fly() method. According to the Duck Typing rule, you can conclude that the Cat doesn't belong to the Duck type.
class Cat {
walk() {
console.log("Cat walk");
}
}
See full example:
duck-typing-example1.js
class Duck {
fly() {
console.log("Duck fly");
}
walk() {
console.log("Duck walk");
}
}
class Airplane {
fly() {
console.log("Airplane fly");
}
walk() {
console.log("Airplane walk");
}
shoot(target) {
console.log("Airplane shoot " + target);
}
}
class Cat {
walk() {
console.log("Cat walk");
}
}
let duck1 = new Duck();
let airplane1 = new Airplane();
let cat1 = new Cat();
function checkDuck(testObj) {
if(typeof testObj.fly == "function" && typeof testObj.walk == "function" ) {
return true;
}
return false;
}
// Array
let testArray = [duck1, airplane1, cat1];
for( let i = 0; i < testArray.length; i++) {
let testObj = testArray[i];
if( checkDuck(testObj) ) {
testObj.fly();
}
}
Output:
Duck fly
Airplane fly
Example 2:
duck-typing-example2.js
var duck = {
type: "bird",
cry: function duck_cry(what) {
console.log(what + " quack-quack!");
},
color: "black"
};
var someAnimal = {
type: "bird",
cry: function animal_cry(what) {
console.log(what + " whoof-whoof!");
},
eyes: "yellow"
};
function check(who) {
if ((who.type == "bird") && (typeof who.cry == "function")) {
who.cry("I look like a duck!\n");
return true;
}
return false;
}
check(duck); // true
check(someAnimal); // true
Output:
I look like a duck!
quack-quack
I lock like a duck!
whoof-whoof
ECMAScript, Javascript Tutorials
- Introduction to Javascript and ECMAScript
- Quickstart with Javascript
- Alert, Confirm, Prompt Dialog Box in Javascript
- Quickstart with JavaScript
- JavaScript Variables Tutorial with Examples
- Bitwise Operations
- JavaScript Arrays Tutorial with Examples
- JavaScript Loops Tutorial with Examples
- JavaScript Functions Tutorial with Examples
- JavaScript Number Tutorial with Examples
- JavaScript Boolean Tutorial with Examples
- JavaScript Strings Tutorial with Examples
- JavaScript if else Statement Tutorial with Examples
- JavaScript Switch Statement
- JavaScript Error Handling Tutorial with Examples
- JavaScript Date Tutorial with Examples
- JavaScript Modules Tutorial with Examples
- The History of Modules in JavaScript
- JavaScript setTimeout and setInterval Function
- Javascript Form Validation Tutorial with Examples
- JavaScript Web Cookies Tutorial with Examples
- JavaScript void Keyword Tutorial with Examples
- Classes and Objects in JavaScript
- Class and inheritance simulation techniques in JavaScript
- Inheritance and polymorphism in JavaScript
- Undertanding Duck Typing in JavaScript
- JavaScript Symbols Tutorial with Examples
- JavaScript Set Collection Tutorial with Examples
- JavaScript Map Collection Tutorial with Examples
- Undertanding JavaScript Iterables and Iterators
- JavaScript Regular Expressions Tutorial with Examples
- JavaScript Promise, Async/Await Tutorial with Examples
- Javascript Window Tutorial with Examples
- Javascript Console Tutorial with Examples
- Javascript Screen Tutorial with Examples
- Javascript Navigator Tutorial with Examples
- Javascript Geolocation API Tutorial with Examples
- Javascript Location Tutorial with Examples
- Javascript History API Tutorial with Examples
- Javascript Statusbar Tutorial with Examples
- Javascript Locationbar Tutorial with Examples
- Javascript Scrollbars Tutorial with Examples
- Javascript Menubar Tutorial with Examples
- JavaScript JSON Tutorial with Examples
- JavaScript Event Handling Tutorial with Examples
- Javascript MouseEvent Tutorial with Examples
- Javascript WheelEvent Tutorial with Examples
- Javascript KeyboardEvent Tutorial with Examples
- Javascript FocusEvent Tutorial with Examples
- Javascript InputEvent Tutorial with Examples
- Javascript ChangeEvent Tutorial with Examples
- Javascript DragEvent Tutorial with Examples
- Javascript HashChangeEvent Tutorial with Examples
- Javascript URL Encoding Tutorial with Examples
- Javascript FileReader Tutorial with Examples
- Javascript XMLHttpRequest Tutorial with Examples
- Javascript Fetch API Tutorial with Examples
- Parsing XML in Javascript with DOMParser
- Introduction to Javascript HTML5 Canvas API
- Highlighting code with SyntaxHighlighter Javascript library
- What are polyfills in programming science?
Show More