JavaScript Variables Tutorial with Examples
1. What is Variable?
a variable means a "Memory location" which a program uses to store values. Variable name is called an identifier.
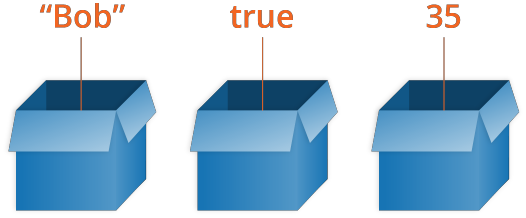
Variable name has to be given by a principle. Below are principles to give a name for a variable:
- Variable names are not allowed to be the same as keywords
- Variable names may contain alphabets or numbers but not start with a number.
- Variable names may not contain white spaces or special characters except for underscore (_) and dolar character ($).
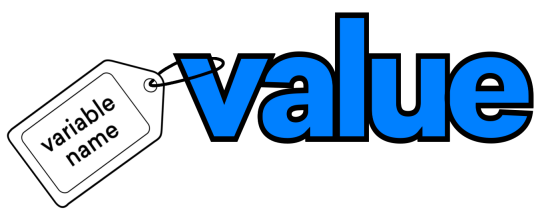
2. Declare variable
Declaring variable is necessary because you have to do so before using it. The ES5 syntax uses the var keyword to declare a variable. The ES6 adds two new keywords such as let & const, to declare a variable.
// Declare variables:
var a1;
console.log(a1); // undefined
var a2 = 100;
console.log(a2); // 100
var a3, a4;
var a5, a6 = 200;
console.log(a5); // undefined
console.log(a6); // 200
var a7= 100, a8 = 300;
let b1;
console.log(b1); // undefined
let b2 = "Hello";
console.log(b2); // Hello
const c = 100;
console.log(c); // 100
block
A block means a set of commands located in a "curly brackets" {}.
let
The let keyword is used to declare a block scope variable, which means that this variable will be realized by the program in such block or inside the sub-block, but is not realized by the program outside the block defining it.
if(true) {
let a = 200;
console.log(a); // 200
}
// Program will ignore this statement:
console.log(a);
If you use the let keyword to define two variables with the same name, one variable declared in the parent block, one variable declared in the sub-block, the program will treat it as two different variables.
let i = 1000;
let j = 2000;
if(true) {
i = 100; // Assign new value to 'i'
let j = 200; // A new variable (***)
console.log("Test1: " + i + " : " + j); // Test1: 100 : 200
}
console.log("Test2: "+ i + " : " + j); // Test2: 100 : 2000
var
The var keyword is used to declare a variable, which has a broader scope than the let variable. It is realized by the program inside the block defining it, in sub-blocks, even realized outside the block having declared it.
if(true) {
var a = 200;
console.log(a); // 200
}
console.log(a); // 200
If you declare two variables with the same name with the var keyword, a variable declared in the parent block, a variable in the sub-block, the program considers the two variables to be the same (Same position in memory).
var i = 1000;
var j = 2000;
if(true) {
i = 100; // Assign new value
var j = 200; // Assign new value
console.log("Test1: " + i + " : " + j); // Test1: 100 : 200
}
console.log("Test2: "+ i + " : " + j); // Test2: 100 : 200
The variables declared with the var keyword in a function will only be recognized by the program in such function. It is not recognized outside the function.
// A function
var test = function() {
var a = 200;
console.log(a); // 200
}
// Call function.
test();
console.log(a); // Not work!!
const
The const keyword is used to declare a constant. When declaring a constant, you have to always assign a value to it. But you can not assign a new value to this variable. Note: Like the let variable, the const variable has a block scope.
// Declare a constant with a value
const greeting = "Hello";
// Assign new value to 'greeting'
greeting = "Hi"; // ==> Error!!
// Declare a constant without a value
const i ; // ==> Error!!
A variable declared with the const keyword, it will be a constant in the sense that you can not assign a new value to it, but it is not immutable, you can still change its properties.
Let's look at the following example. A variable declared with the const keyword, whose value is an object with multiple properties. You can assign new values to the properties of this object.
// Declaring a constant is an object
const person = {
name: "Clinton",
gender : "Female"
};
console.log(person.name); // Clinton
// Can assign new values to properties of const object.
person.name = "Trump";
person.gender = "Male";
console.log(person.name); // Trump
// ** Error! (Cannot assign new value to const variable).
person = {
name : "Trump";
}
+ const: Object.freeze()
The Object.freeze() method helps freeze an object. You can not change or assign a new value to its properties.
Object.freeze()
// Declaring a constant is an object
const person = {
name: "Clinton",
gender : "Female"
};
console.log(person.name); // Clinton
Object.freeze(person); // Freeze object 'person'.
person.name = "Trump";
console.log(person.name); // Clinton
ECMAScript, Javascript Tutorials
- Introduction to Javascript and ECMAScript
- Quickstart with Javascript
- Alert, Confirm, Prompt Dialog Box in Javascript
- Quickstart with JavaScript
- JavaScript Variables Tutorial with Examples
- Bitwise Operations
- JavaScript Arrays Tutorial with Examples
- JavaScript Loops Tutorial with Examples
- JavaScript Functions Tutorial with Examples
- JavaScript Number Tutorial with Examples
- JavaScript Boolean Tutorial with Examples
- JavaScript Strings Tutorial with Examples
- JavaScript if else Statement Tutorial with Examples
- JavaScript Switch Statement
- JavaScript Error Handling Tutorial with Examples
- JavaScript Date Tutorial with Examples
- JavaScript Modules Tutorial with Examples
- The History of Modules in JavaScript
- JavaScript setTimeout and setInterval Function
- Javascript Form Validation Tutorial with Examples
- JavaScript Web Cookies Tutorial with Examples
- JavaScript void Keyword Tutorial with Examples
- Classes and Objects in JavaScript
- Class and inheritance simulation techniques in JavaScript
- Inheritance and polymorphism in JavaScript
- Undertanding Duck Typing in JavaScript
- JavaScript Symbols Tutorial with Examples
- JavaScript Set Collection Tutorial with Examples
- JavaScript Map Collection Tutorial with Examples
- Undertanding JavaScript Iterables and Iterators
- JavaScript Regular Expressions Tutorial with Examples
- JavaScript Promise, Async/Await Tutorial with Examples
- Javascript Window Tutorial with Examples
- Javascript Console Tutorial with Examples
- Javascript Screen Tutorial with Examples
- Javascript Navigator Tutorial with Examples
- Javascript Geolocation API Tutorial with Examples
- Javascript Location Tutorial with Examples
- Javascript History API Tutorial with Examples
- Javascript Statusbar Tutorial with Examples
- Javascript Locationbar Tutorial with Examples
- Javascript Scrollbars Tutorial with Examples
- Javascript Menubar Tutorial with Examples
- JavaScript JSON Tutorial with Examples
- JavaScript Event Handling Tutorial with Examples
- Javascript MouseEvent Tutorial with Examples
- Javascript WheelEvent Tutorial with Examples
- Javascript KeyboardEvent Tutorial with Examples
- Javascript FocusEvent Tutorial with Examples
- Javascript InputEvent Tutorial with Examples
- Javascript ChangeEvent Tutorial with Examples
- Javascript DragEvent Tutorial with Examples
- Javascript HashChangeEvent Tutorial with Examples
- Javascript URL Encoding Tutorial with Examples
- Javascript FileReader Tutorial with Examples
- Javascript XMLHttpRequest Tutorial with Examples
- Javascript Fetch API Tutorial with Examples
- Parsing XML in Javascript with DOMParser
- Introduction to Javascript HTML5 Canvas API
- Highlighting code with SyntaxHighlighter Javascript library
- What are polyfills in programming science?
Show More