Alert, Confirm, Prompt Dialog Box in Javascript
1. Javascript Dialog Box
Javascript Javascript provides three important Dialog Boxes, which include Alert Dialog Box for users, Confirmation DialogBox, and Prompt Dialog Box.
Note: The dialogs provided by Javascript have a very simple and non-customizable interface. In the actual application, you will probably use a library provided by a third party to get better dialog boxes and more options. However, the default Javascript dialog boxes are still useful in many cases.
In this lesson we are going to discuss each dialog box in turn.
2. Alert Dialog Box
Alert Dialog Box is mainly used to display a notice, warning, or error to users. Basically, you cannot customize dialog box icon or title, ... you can only provide the message that the dialog box will display. In addition, Alert Dialog Box has only one OK button to close a dialog box.
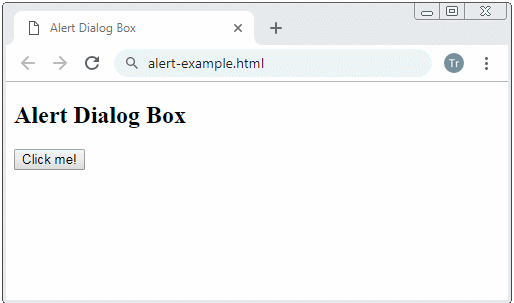
To display a Alert Dialog Box, you call the alert(message) function, in which the message is the content that the dialog box will display.
alert-example.js
<!DOCTYPE html>
<html>
<head>
<title>Alert Dialog Box</title>
<script type="text/javascript">
function testAlertDialog() {
alert("Something Error!");
}
</script>
</head>
<body>
<h2>Alert Dialog Box</h2>
<button onclick="testAlertDialog()">Click me!</button>
</body>
</html>
3. Confirmation Dialog Box
Confirmation Dialog Box is used to ask the user to confirm something. This dialog is very simple, you cannot customize the icon or the title of the dialog box, you can only provide a message asking the user to confirm. This dialog box has 2 OK and Cancel buttons.
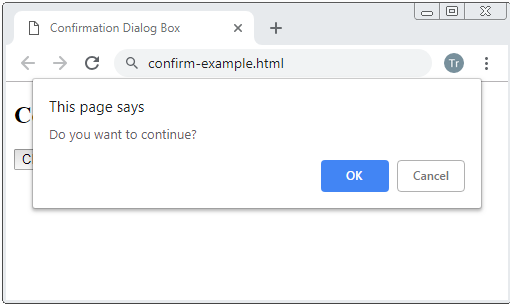
To display a Confirmation Dialog Box you call the confirm(message) function, in which the message is one requesting an user to confirm. If the user clicks the OK button, this function returns true, otherwise if the user clicks the No button, this function returns false.
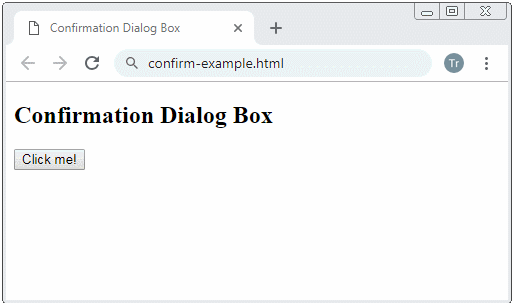
confirm-example.js
<!DOCTYPE html>
<html>
<head>
<title>Confirmation Dialog Box</title>
<script type="text/javascript">
function testConfirmDialog() {
var result = confirm("Do you want to continue?");
if(result) {
alert("OK Next lesson!");
} else {
alert("Bye!");
}
}
</script>
</head>
<body>
<h2>Confirmation Dialog Box</h2>
<button onclick="testConfirmDialog()">Click me!</button>
</body>
</html>
4. Prompt Dialog Box
Prompt Dialog Box is used for users to enter an information. This dialog box is very simple. It includes a Text Field for users to enter information. The dialog box has 2 OK and Cancel buttons.
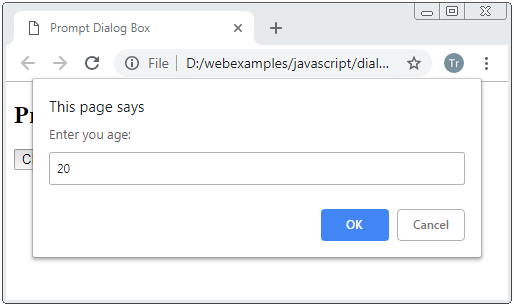
To display a Prompt Dialog Box you call the prompt(message, defaultValue) function in which the message is one for user. defaultValue is default value prefilled in the Text Field.
If an user clicks OK, the function returns contents on Text Field, otherwise, if the user clicks Cancel, the function returns null.
prompt-example.js
<!DOCTYPE html>
<html>
<head>
<title>Prompt Dialog Box</title>
<script type="text/javascript">
function testPromptDialog() {
var result = prompt("Enter you age:", "20");
if(result != null) {
alert("Your age is " + result);
}
}
</script>
</head>
<body>
<h2>Prompt Dialog Box</h2>
<button onclick="testPromptDialog()">Click me!</button>
</body>
</html>
ECMAScript, Javascript Tutorials
- Introduction to Javascript and ECMAScript
- Quickstart with Javascript
- Alert, Confirm, Prompt Dialog Box in Javascript
- Quickstart with JavaScript
- JavaScript Variables Tutorial with Examples
- Bitwise Operations
- JavaScript Arrays Tutorial with Examples
- JavaScript Loops Tutorial with Examples
- JavaScript Functions Tutorial with Examples
- JavaScript Number Tutorial with Examples
- JavaScript Boolean Tutorial with Examples
- JavaScript Strings Tutorial with Examples
- JavaScript if else Statement Tutorial with Examples
- JavaScript Switch Statement
- JavaScript Error Handling Tutorial with Examples
- JavaScript Date Tutorial with Examples
- JavaScript Modules Tutorial with Examples
- The History of Modules in JavaScript
- JavaScript setTimeout and setInterval Function
- Javascript Form Validation Tutorial with Examples
- JavaScript Web Cookies Tutorial with Examples
- JavaScript void Keyword Tutorial with Examples
- Classes and Objects in JavaScript
- Class and inheritance simulation techniques in JavaScript
- Inheritance and polymorphism in JavaScript
- Undertanding Duck Typing in JavaScript
- JavaScript Symbols Tutorial with Examples
- JavaScript Set Collection Tutorial with Examples
- JavaScript Map Collection Tutorial with Examples
- Undertanding JavaScript Iterables and Iterators
- JavaScript Regular Expressions Tutorial with Examples
- JavaScript Promise, Async/Await Tutorial with Examples
- Javascript Window Tutorial with Examples
- Javascript Console Tutorial with Examples
- Javascript Screen Tutorial with Examples
- Javascript Navigator Tutorial with Examples
- Javascript Geolocation API Tutorial with Examples
- Javascript Location Tutorial with Examples
- Javascript History API Tutorial with Examples
- Javascript Statusbar Tutorial with Examples
- Javascript Locationbar Tutorial with Examples
- Javascript Scrollbars Tutorial with Examples
- Javascript Menubar Tutorial with Examples
- JavaScript JSON Tutorial with Examples
- JavaScript Event Handling Tutorial with Examples
- Javascript MouseEvent Tutorial with Examples
- Javascript WheelEvent Tutorial with Examples
- Javascript KeyboardEvent Tutorial with Examples
- Javascript FocusEvent Tutorial with Examples
- Javascript InputEvent Tutorial with Examples
- Javascript ChangeEvent Tutorial with Examples
- Javascript DragEvent Tutorial with Examples
- Javascript HashChangeEvent Tutorial with Examples
- Javascript URL Encoding Tutorial with Examples
- Javascript FileReader Tutorial with Examples
- Javascript XMLHttpRequest Tutorial with Examples
- Javascript Fetch API Tutorial with Examples
- Parsing XML in Javascript with DOMParser
- Introduction to Javascript HTML5 Canvas API
- Highlighting code with SyntaxHighlighter Javascript library
- What are polyfills in programming science?
Show More