Javascript Scrollbars Tutorial with Examples
1. window.scrollbars
The window.scrollbars property returns a Scrollbars object, representing scroll bars surrounding the Viewport of browser. However, you can hardly interact with Scrollbars through Javascript because it has very few APIs for you.
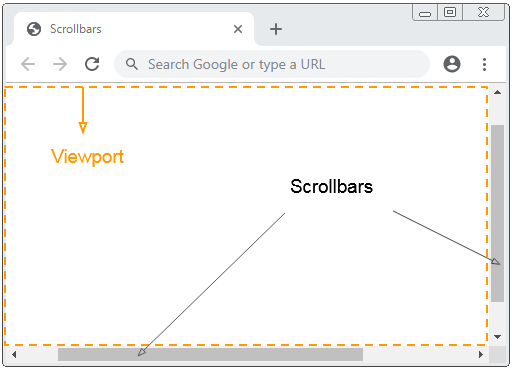
window.scrollbars
// Or Simple:
scrollbars
scrollbars.visible
The Scrollbars object has one only property such as visible. However, this property is unreliable. scrollbars.visible returns true value, which doesn't mean that you are seeing scrollbars.
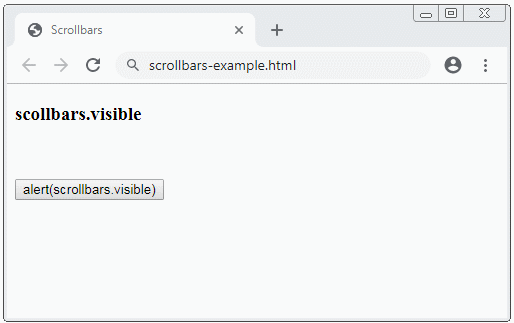
scrollbars-example.html
<!DOCTYPE html>
<html>
<head>
<title>Scrollbars</title>
<meta charset="UTF-8">
</head>
<body>
<h3>scollbars.visible</h3>
<br/><br/>
<button onclick="alert(scrollbars.visible)">
alert(scrollbars.visible)
</button>
</body>
</html>
Example:
Example, use the window.open(..) method to open a new window without scrollbar:
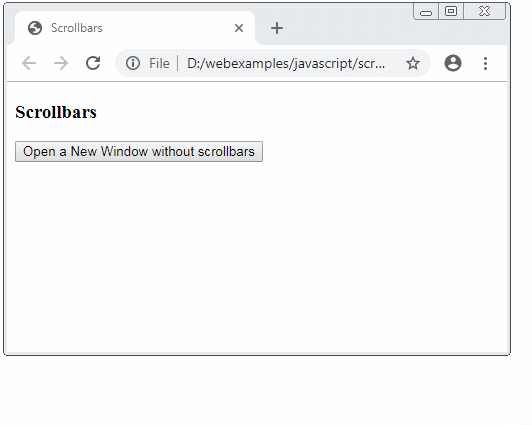
open-new-window-example.html
<!DOCTYPE html>
<html>
<head>
<title>Scrollbars</title>
<meta charset="UTF-8">
<script>
function openNewWindow() {
var winFeature = 'scrollbars=no,resizable=yes';
// Open a New Windows.
window.open('some-page.html','MyWinName',winFeature);
}
</script>
</head>
<body>
<h3>Scrollbars</h3>
<button onclick="openNewWindow()">
Open a New Window without scrollbars
</button>
</body>
</html>
some-page.html
<!DOCTYPE html>
<html>
<head>
<title>Some Page</title>
<meta charset="UTF-8">
<style>
/** CHROME: Make the scroll bar not appear */
body {
overflow: hidden;
}
</style>
</head>
<body>
<h3>Some Page</h3>
<p>1</p>
<p>1 2</p>
<p>1 2 3</p>
<p>1 2 3 4</p>
<p>1 2 3 4 5</p>
<p>1 2 3 4 5 6</p>
<p>1 2 3 4 5 6 7</p>
</body>
</html>
Example:
Example, what do you do so that scrollbars are never displayed?
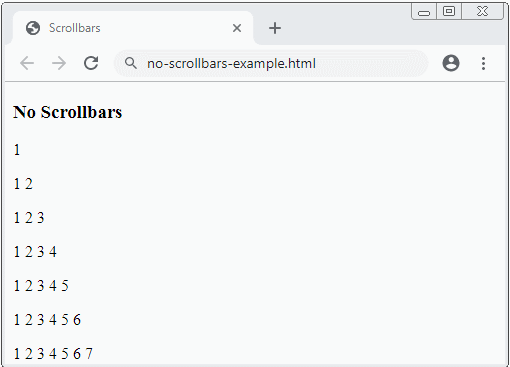
no-scrollbars-example.html
<!DOCTYPE html>
<html>
<head>
<title>Scrollbars</title>
<meta charset="UTF-8">
<style>
body {
overflow: hidden;
}
</style>
</head>
<body>
<h3>No Scrollbars</h3>
<p>1</p>
<p>1 2</p>
<p>1 2 3</p>
<p>1 2 3 4</p>
<p>1 2 3 4 5</p>
<p>1 2 3 4 5 6</p>
<p>1 2 3 4 5 6 7</p>
</body>
</html>
2. Detecting the scroll bar
Sometimes, you want to detect whether the scrollbar of a element is currently displayed Or you want to detect if the Viewport scrollbar is displayed. Below is a small library. It is useful for you in this case.
scrollbars-utils.js
// Private Function
// @axis: 'X', 'Y', 'x', 'y'
function __isScrollbarShowing__(domNode, axis, computedStyles) {
axis = axis.toUpperCase();
var type;
if(axis === 'Y') {
type = 'Height';
} else {
type = 'Width';
}
var scrollType = 'scroll' + type;
var clientType = 'client' + type;
var overflowAxis = 'overflow' + axis;
var hasScroll = domNode[scrollType] > domNode[clientType];
// Check the overflow and overflowY properties for "auto" and "visible" values
var cStyle = computedStyles || window.getComputedStyle(domNode)
return hasScroll && (cStyle[overflowAxis] == "visible"
|| cStyle[overflowAxis] == "auto"
)
|| cStyle[overflowAxis] == "scroll";
}
// @domNode: Optional.
function isScrollbarXShowing(domNode) {
if(!domNode) {
domNode = document.documentElement;
}
return __isScrollbarShowing__(domNode, 'x');
}
// @domNode: Optional.
function isScrollbarYShowing(domNode) {
if(!domNode) {
domNode = document.documentElement;
}
return __isScrollbarShowing__(domNode, 'y');
}
// Scrollbar X or Scrollbar Y is showing?
// @domNode: Optional.
function isScrollbarShowing(domNode) {
return isScrollbarXShowing(domNode) || isScrollbarYShowing(domNode);
}
Example: use the above library to detect the scrollbar of Viewport.
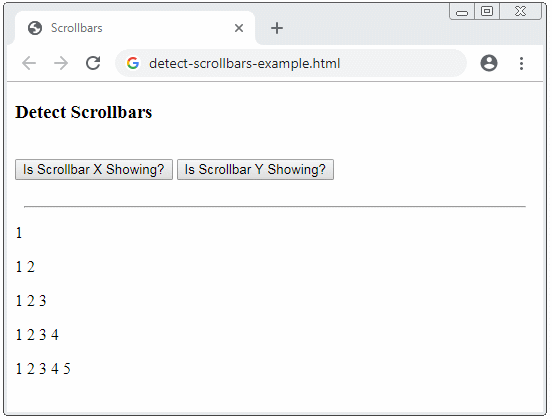
detect-scollbars-example.html
<!DOCTYPE html>
<html>
<head>
<title>Scrollbars</title>
<meta charset="UTF-8">
<script src="scrollbars-utils.js"></script>
</head>
<body>
<h3>Detect Scrollbars</h3>
<br/>
<button onclick="alert(isScrollbarXShowing())">
Is Scrollbar X Showing?
</button>
<button onclick="alert(isScrollbarYShowing())">
Is Scrollbar Y Showing?
</button>
<br/><br/>
<hr style="width: 500px;"/>
<p>1</p>
<p>1 2</p>
<p>1 2 3</p>
<p>1 2 3 4</p>
<p>1 2 3 4 5</p>
</body>
</html>
Example: detect the scrollbar of the <div> element:
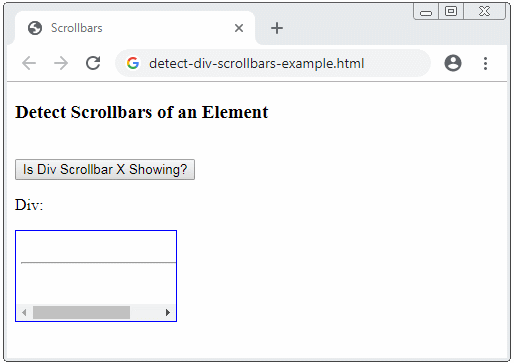
detect-div-scrollbars-example.html
<!DOCTYPE html>
<html>
<head>
<title>Scrollbars</title>
<meta charset="UTF-8">
<style>
#my-div {
border: 1px solid blue;
overflow-x: auto;
padding: 5px;
width: 150px;
height: 80px;
}
</style>
<script src="scrollbars-utils.js"></script>
<script>
function detectScrollbarXOfDiv() {
var domNode = document.getElementById("my-div");
var scX = isScrollbarXShowing(domNode);
var scY = isScrollbarYShowing(domNode);
alert("Is Div Scrollbar X Showing? " + scX);
}
</script>
</head>
<body>
<h3>Detect Scrollbars of an Element</h3>
<br/>
<button onclick="detectScrollbarXOfDiv()">
Is Div Scrollbar X Showing?
</button>
<p>Div:</p>
<div id="my-div">
<br>
<hr style="width:200px"/>
<br>
</div>
</body>
</html>
ECMAScript, Javascript Tutorials
- Introduction to Javascript and ECMAScript
- Quickstart with Javascript
- Alert, Confirm, Prompt Dialog Box in Javascript
- Quickstart with JavaScript
- JavaScript Variables Tutorial with Examples
- Bitwise Operations
- JavaScript Arrays Tutorial with Examples
- JavaScript Loops Tutorial with Examples
- JavaScript Functions Tutorial with Examples
- JavaScript Number Tutorial with Examples
- JavaScript Boolean Tutorial with Examples
- JavaScript Strings Tutorial with Examples
- JavaScript if else Statement Tutorial with Examples
- JavaScript Switch Statement
- JavaScript Error Handling Tutorial with Examples
- JavaScript Date Tutorial with Examples
- JavaScript Modules Tutorial with Examples
- The History of Modules in JavaScript
- JavaScript setTimeout and setInterval Function
- Javascript Form Validation Tutorial with Examples
- JavaScript Web Cookies Tutorial with Examples
- JavaScript void Keyword Tutorial with Examples
- Classes and Objects in JavaScript
- Class and inheritance simulation techniques in JavaScript
- Inheritance and polymorphism in JavaScript
- Undertanding Duck Typing in JavaScript
- JavaScript Symbols Tutorial with Examples
- JavaScript Set Collection Tutorial with Examples
- JavaScript Map Collection Tutorial with Examples
- Undertanding JavaScript Iterables and Iterators
- JavaScript Regular Expressions Tutorial with Examples
- JavaScript Promise, Async/Await Tutorial with Examples
- Javascript Window Tutorial with Examples
- Javascript Console Tutorial with Examples
- Javascript Screen Tutorial with Examples
- Javascript Navigator Tutorial with Examples
- Javascript Geolocation API Tutorial with Examples
- Javascript Location Tutorial with Examples
- Javascript History API Tutorial with Examples
- Javascript Statusbar Tutorial with Examples
- Javascript Locationbar Tutorial with Examples
- Javascript Scrollbars Tutorial with Examples
- Javascript Menubar Tutorial with Examples
- JavaScript JSON Tutorial with Examples
- JavaScript Event Handling Tutorial with Examples
- Javascript MouseEvent Tutorial with Examples
- Javascript WheelEvent Tutorial with Examples
- Javascript KeyboardEvent Tutorial with Examples
- Javascript FocusEvent Tutorial with Examples
- Javascript InputEvent Tutorial with Examples
- Javascript ChangeEvent Tutorial with Examples
- Javascript DragEvent Tutorial with Examples
- Javascript HashChangeEvent Tutorial with Examples
- Javascript URL Encoding Tutorial with Examples
- Javascript FileReader Tutorial with Examples
- Javascript XMLHttpRequest Tutorial with Examples
- Javascript Fetch API Tutorial with Examples
- Parsing XML in Javascript with DOMParser
- Introduction to Javascript HTML5 Canvas API
- Highlighting code with SyntaxHighlighter Javascript library
- What are polyfills in programming science?
Show More