Javascript DragEvent Tutorial with Examples
1. DragEvent
DragEvent is an interface that represents events occurring during drag & drop of an object from one place to another. An user initiates a drag by putting a pointer device (such as a mouse) on the surface of an object, press down, and move the pointer device to another place, then release the pointer device, to drop the object into a new location.
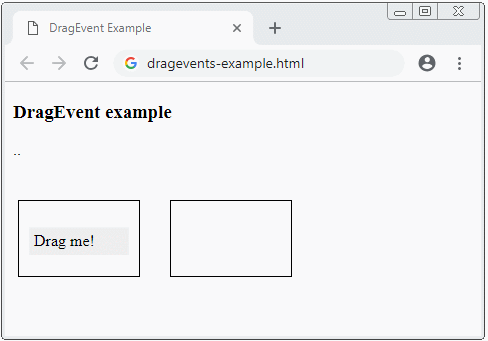
DragEvent is a sub-interface of MouseEvent, therefore, it inherits all the properties and methods of MouseEvent.
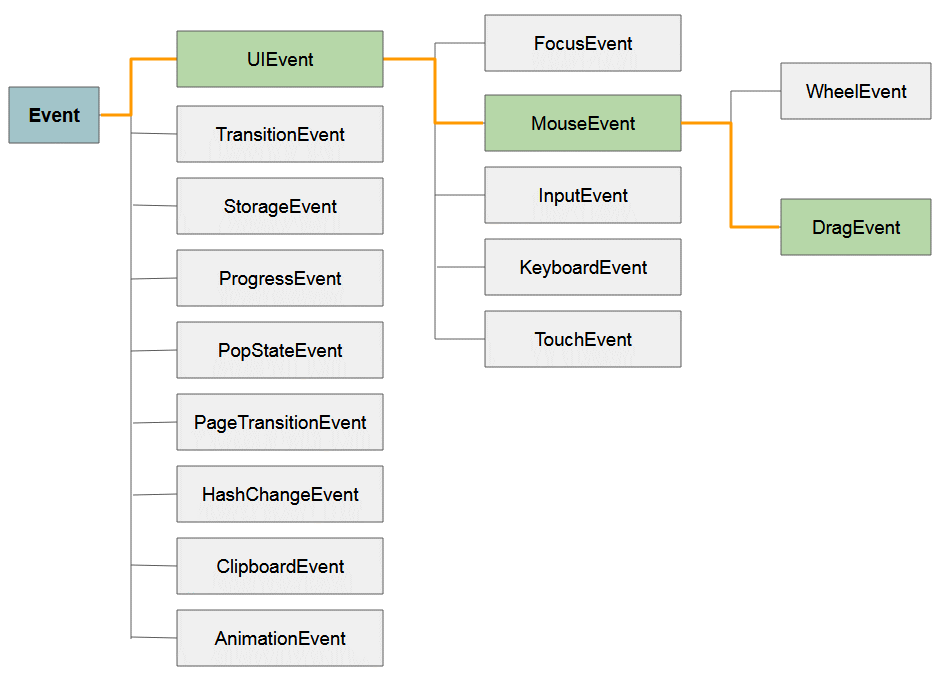
Below is the list of the types of events that will occur during drag and drop
Event | Element Type | Description |
dragstart | Dragged Element | The event occurs when the user starts to drag an element |
drag | Dragged Element | The event occurs when an element is being dragged |
dragend | Dragged Element | The event occurs when the user has finished dragging an element |
dragenter | Drop Target | The event occurs when the dragged element enters the drop target |
dragover | Drop Target | The event occurs when the dragged element is over the drop target |
dragleave | Drop Target | The event occurs when the dragged element leaves the drop target |
drop | Drop Target | The event occurs when the dragged element is dropped on the drop target |
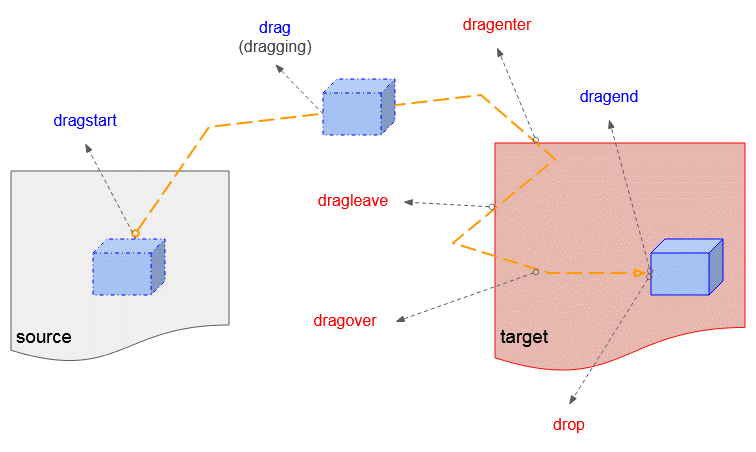
Illustrations of events occurring during drag drop.
Property | Description |
dataTransfer | Returns the data that is dragged/dropped |
See a simple example:
dragevents-example.html
<!DOCTYPE html>
<html>
<head>
<title>DragEvent Example</title>
<script src="dragevents-example.js"></script>
<style>
.droptarget-div {
float: left;
width: 100px;
height: 55px;
margin: 25px 25px 5px 5px;
padding: 10px;
border: 1px solid black;
}
#dragtarget-div {
background: #eee;
padding: 5px;
}
</style>
</head>
<body>
<h3>DragEvent example</h3>
<p id="log-div">..</p>
<!-- Drop Target 1 -->
<div class="droptarget-div"
ondragenter= "ondragenterHandler(event)"
ondragover="dragoverHandler(event)"
ondrop="dropHandler(event)">
<p id="dragtarget-div"
ondragstart="dragstartHandler(event)"
ondrag="dragHandler(event)"
draggable="true">
Drag me!
</p>
</div>
<!-- Drop Target 2 -->
<div class="droptarget-div"
ondragenter= "ondragenterHandler(event)"
ondragover="dragoverHandler(event)"
ondrop="dropHandler(event)">
</div>
</body>
</html>
dragevents-example.js
// --------- Handlers for 'Dragged Element' ------------------
function dragstartHandler(evt) {
evt.dataTransfer.setData("MyDraggedElementId", evt.target.id);
}
// dragging ...
function dragHandler(evt) {
showLog("The p element is being dragged");
}
// --------- Handlers for 'Drop Target' ------------------
function ondragenterHandler() {
showLog("The p element enter drop-target");
}
// When 'over' you can 'release mouse' to 'drop'.
function dragoverHandler(evt) {
evt.preventDefault(); // Important!!
}
function dropHandler(evt) {
evt.preventDefault(); // Important!!
var elementId = evt.dataTransfer.getData("MyDraggedElementId");
evt.target.appendChild(document.getElementById(elementId));
showLog("The p element was dropped");
}
// -------------------------------------------------------
function showLog(text) {
document.getElementById("log-div").innerHTML = text;
}
-
For example, users drag files on their computer and drop into a <div> element, you can read the basic information, and the content of the files that the user has dropped.
When the drop event occurs, event.dataTransfer.files returns a FileList object, which contains a list of File objects.
drop-files-example.html
<!DOCTYPE html>
<html>
<head>
<title>DragEvent Example</title>
<meta charset="UTF-8">
<script src="drop-files-example.js"></script>
<style>
#drop-area {
border: 1px solid blue;
padding: 15px;
}
</style>
</head>
<body>
<h3>Drop files example</h3>
<div id="drop-area" ondragover="dragoverHandler(event)" ondrop="dropHandler(event)">
Drop files here
</div>
<output id="log-div"></output>
</body>
</html>
drop-files-example.js
function dropHandler(evt) {
evt.stopPropagation();
evt.preventDefault();
// FileList object.
var files = evt.dataTransfer.files;
// files is a FileList of File objects. List some properties.
var output = [];
for (var i = 0, f; f = files[i]; i++) {
output.push('<li><strong>', escape(f.name), //
'</strong> (', f.type || 'n/a', ') - ', //
f.size, ' bytes, last modified: ',
f.lastModifiedDate ? f.lastModifiedDate.toLocaleDateString() : 'n/a',
'</li>');
}
document.getElementById('log-div').innerHTML = '<ul>' + output.join('') + '</ul>';
}
function dragoverHandler(evt) {
evt.stopPropagation();
evt.preventDefault();
// Explicitly show this is a copy.
evt.dataTransfer.dropEffect = 'copy';
}
ECMAScript, Javascript Tutorials
- Introduction to Javascript and ECMAScript
- Quickstart with Javascript
- Alert, Confirm, Prompt Dialog Box in Javascript
- Quickstart with JavaScript
- JavaScript Variables Tutorial with Examples
- Bitwise Operations
- JavaScript Arrays Tutorial with Examples
- JavaScript Loops Tutorial with Examples
- JavaScript Functions Tutorial with Examples
- JavaScript Number Tutorial with Examples
- JavaScript Boolean Tutorial with Examples
- JavaScript Strings Tutorial with Examples
- JavaScript if else Statement Tutorial with Examples
- JavaScript Switch Statement
- JavaScript Error Handling Tutorial with Examples
- JavaScript Date Tutorial with Examples
- JavaScript Modules Tutorial with Examples
- The History of Modules in JavaScript
- JavaScript setTimeout and setInterval Function
- Javascript Form Validation Tutorial with Examples
- JavaScript Web Cookies Tutorial with Examples
- JavaScript void Keyword Tutorial with Examples
- Classes and Objects in JavaScript
- Class and inheritance simulation techniques in JavaScript
- Inheritance and polymorphism in JavaScript
- Undertanding Duck Typing in JavaScript
- JavaScript Symbols Tutorial with Examples
- JavaScript Set Collection Tutorial with Examples
- JavaScript Map Collection Tutorial with Examples
- Undertanding JavaScript Iterables and Iterators
- JavaScript Regular Expressions Tutorial with Examples
- JavaScript Promise, Async/Await Tutorial with Examples
- Javascript Window Tutorial with Examples
- Javascript Console Tutorial with Examples
- Javascript Screen Tutorial with Examples
- Javascript Navigator Tutorial with Examples
- Javascript Geolocation API Tutorial with Examples
- Javascript Location Tutorial with Examples
- Javascript History API Tutorial with Examples
- Javascript Statusbar Tutorial with Examples
- Javascript Locationbar Tutorial with Examples
- Javascript Scrollbars Tutorial with Examples
- Javascript Menubar Tutorial with Examples
- JavaScript JSON Tutorial with Examples
- JavaScript Event Handling Tutorial with Examples
- Javascript MouseEvent Tutorial with Examples
- Javascript WheelEvent Tutorial with Examples
- Javascript KeyboardEvent Tutorial with Examples
- Javascript FocusEvent Tutorial with Examples
- Javascript InputEvent Tutorial with Examples
- Javascript ChangeEvent Tutorial with Examples
- Javascript DragEvent Tutorial with Examples
- Javascript HashChangeEvent Tutorial with Examples
- Javascript URL Encoding Tutorial with Examples
- Javascript FileReader Tutorial with Examples
- Javascript XMLHttpRequest Tutorial with Examples
- Javascript Fetch API Tutorial with Examples
- Parsing XML in Javascript with DOMParser
- Introduction to Javascript HTML5 Canvas API
- Highlighting code with SyntaxHighlighter Javascript library
- What are polyfills in programming science?
Show More