JavaScript void Keyword Tutorial with Examples
1. Void Keyword
void is an important keyword in ECMAScript. It has the following characteristics:
- void acts as an operator, standing before a single operand with any type.
- void is used to evaluate an expression. It will not return any value, or in other words it returns undefined value.
The void operator precedes any expression to get an undefined value
void-expression-example.js
void console.log("Test1"); // Test1
console.log( void ("Test2") ); // undefined
console.log( void (2 == "2") ); // undefined
console.log( void (2) == "2" ); // false
console.log( void (2) == undefined); // true
2. Void and JavaScript URIs
<a href ="javascript:URI">..</a> is common in HTML. Browsers will evaluate the URI and take the value returned by the URI to replace the content on the current page.
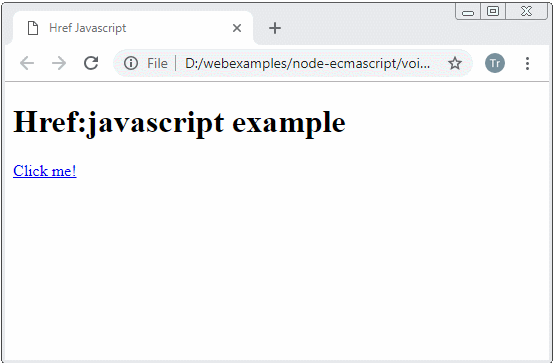
href-javascript-example.html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Href Javascript</title>
<script>
function getHtmlContentToShow() {
console.log("Do something here...");
alert("Do something here..");
// Return new HTML content to replace current Page.
return "<h1 style='color:red;'>Other HTML Content!</h1>";
}
</script>
</head>
<body>
<h1>Href:javascript example</h1>
<a href="javascript:getHtmlContentToShow()">Click me!</a>
</body>
</html>
If the URI returns undefined the browser will not replace the content of the current page.
href-javascript-void-example.html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Href Javascript Void</title>
<script>
function getHtmlContentToShow() {
console.log("Do something here...");
alert("Do something here..");
// Return new HTML content to replace current Page.
return "<h1 style='color:red;'>Other HTML Content!</h1>";
}
</script>
</head>
<body>
<h1>Href:javascript void example</h1>
<a href="javascript:void getHtmlContentToShow()">Click me!</a>
</body>
</html>
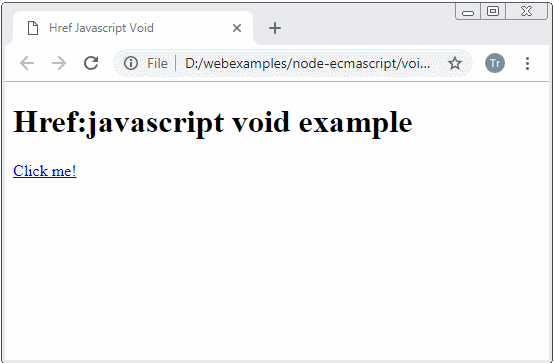
3. One-time use function
Normally, you need to define a function and then call it.
function-example.js
// Defind a function
function sayHello() {
console.log("Hello Everyone");
}
// Call function
sayHello();
The following example shows you how to create a function only for one time use purpose. It is called immediately. You will not be able to use this function anywhere else in the program because it does not exist after being used.
used-once-function-example.js
// Defind a function, and call it.
(function sayHello() {
console.log("Hello Everyone");
}) ();
try {
// This function does not exist.
sayHello();
}
catch(e) {
console.log(e); // ReferenceError: sayHello is not defined
}
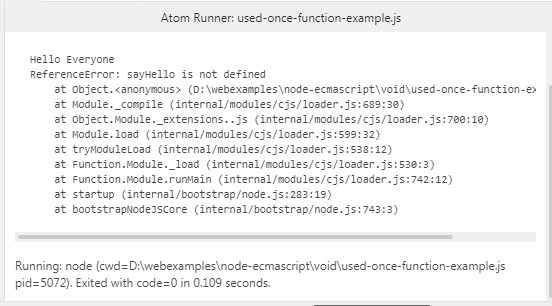
You can also create a function to use only one time with the void keyword. It is called immediately, and no longer exists after being called.
void-function-example.js
// Defind a function, and call it.
void function sayHello() {
console.log("Hello Everyone");
}();
try {
// This function does not exist.
sayHello();
}
catch(e) {
console.log(e); // ReferenceError: sayHello is not defined
}
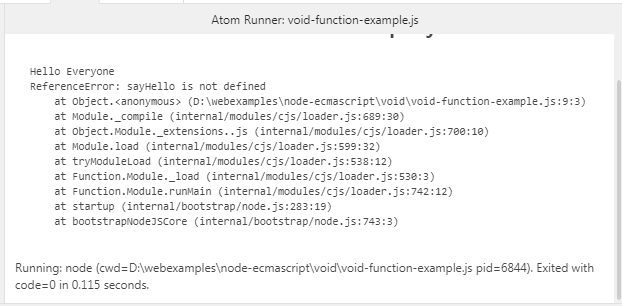
ECMAScript, Javascript Tutorials
- Introduction to Javascript and ECMAScript
- Quickstart with Javascript
- Alert, Confirm, Prompt Dialog Box in Javascript
- Quickstart with JavaScript
- JavaScript Variables Tutorial with Examples
- Bitwise Operations
- JavaScript Arrays Tutorial with Examples
- JavaScript Loops Tutorial with Examples
- JavaScript Functions Tutorial with Examples
- JavaScript Number Tutorial with Examples
- JavaScript Boolean Tutorial with Examples
- JavaScript Strings Tutorial with Examples
- JavaScript if else Statement Tutorial with Examples
- JavaScript Switch Statement
- JavaScript Error Handling Tutorial with Examples
- JavaScript Date Tutorial with Examples
- JavaScript Modules Tutorial with Examples
- The History of Modules in JavaScript
- JavaScript setTimeout and setInterval Function
- Javascript Form Validation Tutorial with Examples
- JavaScript Web Cookies Tutorial with Examples
- JavaScript void Keyword Tutorial with Examples
- Classes and Objects in JavaScript
- Class and inheritance simulation techniques in JavaScript
- Inheritance and polymorphism in JavaScript
- Undertanding Duck Typing in JavaScript
- JavaScript Symbols Tutorial with Examples
- JavaScript Set Collection Tutorial with Examples
- JavaScript Map Collection Tutorial with Examples
- Undertanding JavaScript Iterables and Iterators
- JavaScript Regular Expressions Tutorial with Examples
- JavaScript Promise, Async/Await Tutorial with Examples
- Javascript Window Tutorial with Examples
- Javascript Console Tutorial with Examples
- Javascript Screen Tutorial with Examples
- Javascript Navigator Tutorial with Examples
- Javascript Geolocation API Tutorial with Examples
- Javascript Location Tutorial with Examples
- Javascript History API Tutorial with Examples
- Javascript Statusbar Tutorial with Examples
- Javascript Locationbar Tutorial with Examples
- Javascript Scrollbars Tutorial with Examples
- Javascript Menubar Tutorial with Examples
- JavaScript JSON Tutorial with Examples
- JavaScript Event Handling Tutorial with Examples
- Javascript MouseEvent Tutorial with Examples
- Javascript WheelEvent Tutorial with Examples
- Javascript KeyboardEvent Tutorial with Examples
- Javascript FocusEvent Tutorial with Examples
- Javascript InputEvent Tutorial with Examples
- Javascript ChangeEvent Tutorial with Examples
- Javascript DragEvent Tutorial with Examples
- Javascript HashChangeEvent Tutorial with Examples
- Javascript URL Encoding Tutorial with Examples
- Javascript FileReader Tutorial with Examples
- Javascript XMLHttpRequest Tutorial with Examples
- Javascript Fetch API Tutorial with Examples
- Parsing XML in Javascript with DOMParser
- Introduction to Javascript HTML5 Canvas API
- Highlighting code with SyntaxHighlighter Javascript library
- What are polyfills in programming science?
Show More