Javascript FileReader Tutorial with Examples
1. FileReader
Interface FileReader in Javascript is designed to read data sources on users' computers. Note: In fact, Javascript can read only resources on the user's computer if the user has actions to allow, for example, the user clicks the <input type="file"> element to select the file on the computer, or drag and drop the files into the browser.
FileReader can read the File, Blob or DataTransfer objects (This object is obtained when users drug and drop a file into the browser).
The API of FileReader is designed with the same main purpose as XMLHttpRequest because both of them have the goal such as loading external resource. Data is read asynchronously to avoid blocking browser while the process is being carried out.
Constructor
// Create a FileReader object:
var fileReader = new FileReader();
Properties
Property | Description |
error | A the DOMException object that describe errors happening during reading data sources. |
readyState | A number that describes the status of FileReader. Values may be: 0, 1, 2. |
result | Content of data source after reading successfully. Its format depends on which method is used when starting reading. |
Possible values of fileReader.readyState:
FileReader.EMPTY | 0 | No data has been loaded yet. |
FileReader.LOADING | 1 | Data is currently being loaded. |
FileReader.DONE | 2 | The entire read request has been completed. |
Methods
Property | Description |
abort() | Aborts the read operation. Upon return, the readyState will be FileReader.DONE. |
readAsArrayBuffer(blobOrFile) | Starts reading the contents of blobOrFile, once finished, fileReader.result will be an ArrayBuffer object. |
readAsBinaryString(blobOrFile) | Starts reading the contents of blobOrFile, once finished, fileReader.result will be Binary String. |
readAsDataURL(blobOrFile) | Starts reading the contents of blobOrFile, once finished, fileReader.result will be a URL representing the file's data. |
readAsText(blobOrFile[,encoding]) | Starts reading the contents of blobOrFile, once finished, fileReader.result will be a String. |
Events
During loading data source with FileReader, the events described in ProgressEvent will be fired, specifically:
Event | Description |
loadstart | Indicates that the process of loading data has begun. This event always fires first. |
progress | Event fires multiple times as data is being loaded, giving access to intermediate data. |
error | Event fires when loading has failed. |
abort | Event fires when data loading has been canceled by calling abort() method (Method available on both XMLHttpRequest & FileReader). |
load | Event fires only when all data has been successfully read. |
loadend | Event fires when the object has finished transferring data. Always fires after error, abort, or load. |
- Javascript ProgressEvent
Event Handlers
Handler | Description |
onloadstart(progressEvt) | A handler for the loadstart event. |
onprogress(progressEvt) | A handler for the progress event. |
onerror(progressEvt) | A handler for the error event. |
onabort(progressEvt) | A handler for the abort event. |
onload(progressEvt) | A handler for the load event. |
onloadend(progressEvt) | A handler for the loadend event. |
Steps to work with FileReader:
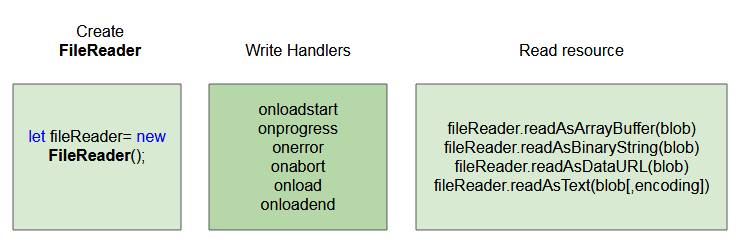
2. Example with FileReader
In this example, users drag and drop a file into a <div> element. FileReader will be used to read the basic information and contents of the file dropped by the user.
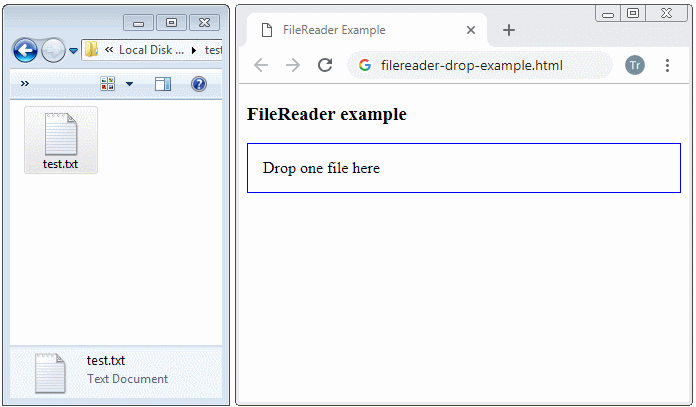
filereader-drop-example.html
<!DOCTYPE html>
<html>
<head>
<title>FileReader Example</title>
<meta charset="UTF-8">
<script src="filereader-drop-example.js"></script>
<style>
#drop-area {
border: 1px solid blue;
padding: 15px;
margin-top:5px;
}
</style>
</head>
<body>
<h3>FileReader example</h3>
<a href="">Reset</a>
<div id="drop-area" ondragover="dragoverHandler(event)" ondrop="dropHandler(event)">
Drop one file here
</div>
<output id="log-div"></output>
</body>
</html>
filereader-drop-example.js
function dropHandler(evt) {
evt.stopPropagation();
evt.preventDefault();
// FileList object.
var files = evt.dataTransfer.files;
if (files.length != 1) {
resetLog();
appendLog("Please drag and drop 1 file!");
return;
}
var file = files[0];
var fileReader = new FileReader();
fileReader.onloadstart = function(progressEvent) {
resetLog();
appendLog("onloadstart!");
var msg = "File Name: " + file.name + "<br>" +
"File Size: " + file.size + "<br>" +
"File Type: " + file.type;
appendLog(msg);
}
fileReader.onload = function(progressEvent) {
appendLog("onload!");
var stringData = fileReader.result;
appendLog(" ---------------- File Content ----------------: ");
appendLog(stringData);
}
fileReader.onloadend = function(progressEvent) {
appendLog("onloadend!");
// FileReader.EMPTY, FileReader.LOADING, FileReader.DONE
appendLog("readyState = " + fileReader.readyState);
}
fileReader.onerror = function(progressEvent) {
appendLog("onerror!");
appendLog("Has Error!");
}
// Read file asynchronously.
fileReader.readAsText(file, "UTF-8"); // fileReader.result -> String.
}
function dragoverHandler(evt) {
evt.stopPropagation();
evt.preventDefault();
// Explicitly show this is a copy.
evt.dataTransfer.dropEffect = 'copy';
}
function resetLog() {
document.getElementById('log-div').innerHTML = "";
}
function appendLog(msg) {
document.getElementById('log-div').innerHTML += "<br>" + msg;
}
-
For example, users choose a file through the <input type="file"> element. Use FileReader to read the basic information and contents of the file just selected by the user.
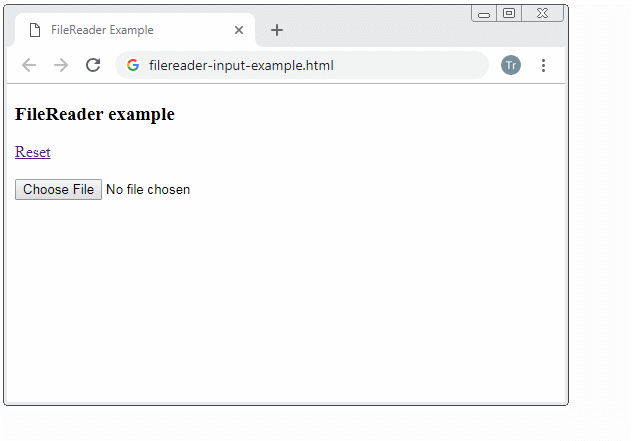
filereader-input-example.html
<!DOCTYPE html>
<html>
<head>
<title>FileReader Example</title>
<meta charset="UTF-8">
<script src="filereader-input-example.js"></script>
</head>
<body>
<h3>FileReader example</h3>
<a href="">Reset</a> <br><br>
<input type="file" onchange = "changeHandler(event)">
<br><br>
<output id="log-div"></output>
</body>
</html>
filereader-input-example.js
function changeHandler(evt) {
evt.stopPropagation();
evt.preventDefault();
// FileList object.
var files = evt.target.files;
var file = files[0];
var fileReader = new FileReader();
fileReader.onloadstart = function(progressEvent) {
resetLog();
appendLog("onloadstart!");
var msg = "File Name: " + file.name + "<br>" +
"File Size: " + file.size + "<br>" +
"File Type: " + file.type;
appendLog(msg);
}
fileReader.onload = function(progressEvent) {
appendLog("onload!");
var stringData = fileReader.result;
appendLog(" ---------------- File Content ----------------: ");
appendLog(stringData);
}
fileReader.onloadend = function(progressEvent) {
appendLog("onloadend!");
// FileReader.EMPTY, FileReader.LOADING, FileReader.DONE
appendLog("readyState = " + fileReader.readyState);
}
fileReader.onerror = function(progressEvent) {
appendLog("onerror!");
appendLog("Has Error!");
}
// Read file asynchronously.
fileReader.readAsText(file, "UTF-8"); // fileReader.result -> String.
}
function resetLog() {
document.getElementById('log-div').innerHTML = "";
}
function appendLog(msg) {
document.getElementById('log-div').innerHTML += "<br>" + msg;
}
-
If you use the fileReader.readAsDataURL(blobOrFile) method, when finishing, the fileReader.result will return an URL representing for the data read, for example:
data:application/x-msdownload;base64,TVqQAA.....ACAEA
data:image/png;base64,iVBORw...eYm57Ad6m6uHj96j
Example: Users select a picture file on a computer and use the filerReader.readAsDataURL(file) to read the file just selected by the users. The fileReader.result will return an URL. Set this URL for the src attribute of the <img> element to display the picture on browser.
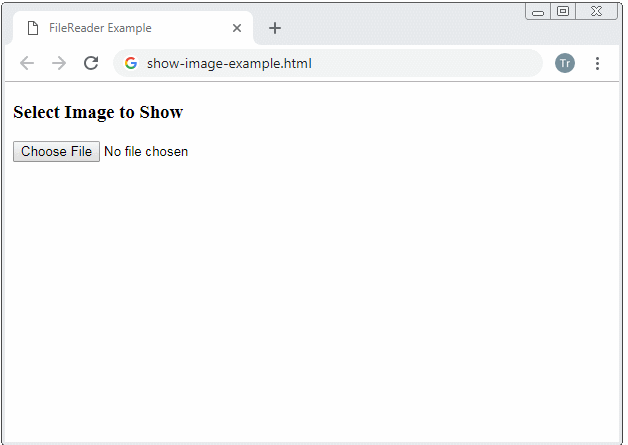
show-local-image-example.html
<!DOCTYPE html>
<html>
<head>
<title>FileReader Example</title>
<meta charset="UTF-8">
<script src="show-local-image-example.js"></script>
</head>
<body>
<h3>Select Image to Show</h3>
<input type="file" onchange = "changeHandler(event)">
<br><br>
<img id="myimage" src="">
</body>
</html>
show-local-image-example.js
function changeHandler(evt) {
evt.stopPropagation();
evt.preventDefault();
// FileList object.
var files = evt.target.files;
var file = files[0];
var fileReader = new FileReader();
fileReader.onload = function(progressEvent) {
var url = fileReader.result;
// Something like: data:image/png;base64,iVBORw...Ym57Ad6m6uHj96js
console.log(url);
//
var myImg = document.getElementById("myimage");
myImg.src= url;
}
// Read file asynchronously.
fileReader.readAsDataURL(file); // fileReader.result -> URL.
}
ECMAScript, Javascript Tutorials
- Introduction to Javascript and ECMAScript
- Quickstart with Javascript
- Alert, Confirm, Prompt Dialog Box in Javascript
- Quickstart with JavaScript
- JavaScript Variables Tutorial with Examples
- Bitwise Operations
- JavaScript Arrays Tutorial with Examples
- JavaScript Loops Tutorial with Examples
- JavaScript Functions Tutorial with Examples
- JavaScript Number Tutorial with Examples
- JavaScript Boolean Tutorial with Examples
- JavaScript Strings Tutorial with Examples
- JavaScript if else Statement Tutorial with Examples
- JavaScript Switch Statement
- JavaScript Error Handling Tutorial with Examples
- JavaScript Date Tutorial with Examples
- JavaScript Modules Tutorial with Examples
- The History of Modules in JavaScript
- JavaScript setTimeout and setInterval Function
- Javascript Form Validation Tutorial with Examples
- JavaScript Web Cookies Tutorial with Examples
- JavaScript void Keyword Tutorial with Examples
- Classes and Objects in JavaScript
- Class and inheritance simulation techniques in JavaScript
- Inheritance and polymorphism in JavaScript
- Undertanding Duck Typing in JavaScript
- JavaScript Symbols Tutorial with Examples
- JavaScript Set Collection Tutorial with Examples
- JavaScript Map Collection Tutorial with Examples
- Undertanding JavaScript Iterables and Iterators
- JavaScript Regular Expressions Tutorial with Examples
- JavaScript Promise, Async/Await Tutorial with Examples
- Javascript Window Tutorial with Examples
- Javascript Console Tutorial with Examples
- Javascript Screen Tutorial with Examples
- Javascript Navigator Tutorial with Examples
- Javascript Geolocation API Tutorial with Examples
- Javascript Location Tutorial with Examples
- Javascript History API Tutorial with Examples
- Javascript Statusbar Tutorial with Examples
- Javascript Locationbar Tutorial with Examples
- Javascript Scrollbars Tutorial with Examples
- Javascript Menubar Tutorial with Examples
- JavaScript JSON Tutorial with Examples
- JavaScript Event Handling Tutorial with Examples
- Javascript MouseEvent Tutorial with Examples
- Javascript WheelEvent Tutorial with Examples
- Javascript KeyboardEvent Tutorial with Examples
- Javascript FocusEvent Tutorial with Examples
- Javascript InputEvent Tutorial with Examples
- Javascript ChangeEvent Tutorial with Examples
- Javascript DragEvent Tutorial with Examples
- Javascript HashChangeEvent Tutorial with Examples
- Javascript URL Encoding Tutorial with Examples
- Javascript FileReader Tutorial with Examples
- Javascript XMLHttpRequest Tutorial with Examples
- Javascript Fetch API Tutorial with Examples
- Parsing XML in Javascript with DOMParser
- Introduction to Javascript HTML5 Canvas API
- Highlighting code with SyntaxHighlighter Javascript library
- What are polyfills in programming science?
Show More