JavaScript JSON Tutorial with Examples
1. What is JSON?
As you know data is always transmitted from one computer to the other through the Internet environment, and it is packaged in a certain format before sending it. Recipients must understand this format to analyze the original data.
XML is a quite common format for storing and transporting data.
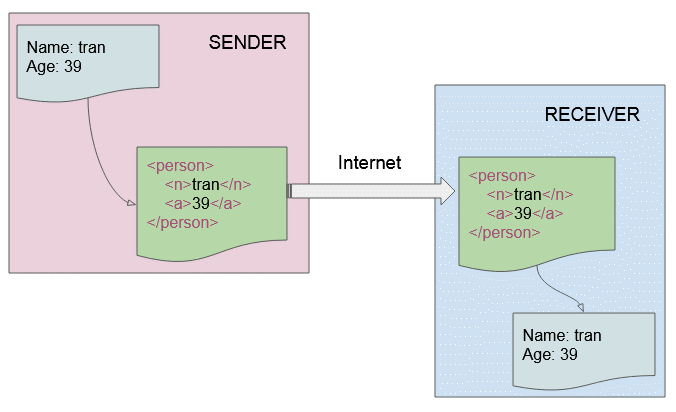
JSON stands for JavaScript Object Notation. It is also a format for storing and transporting data. The JSON format is derived from the object syntax of Javascript, therefore, it inherits simplicity, and is completely based on text.
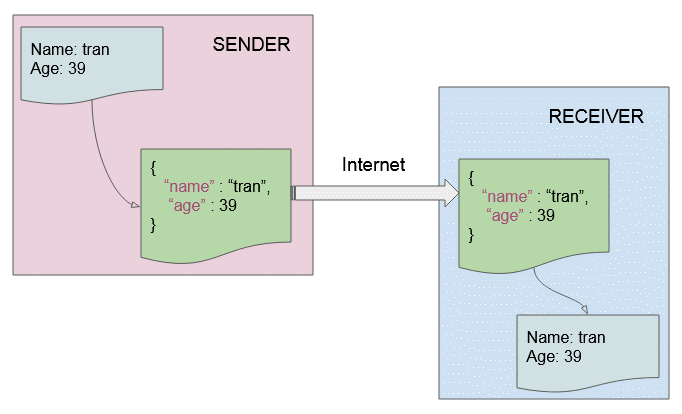
Below is a code snippet that declares an object in Javascript:
Javascript Object
var aCompany = {
name : "Amazon",
ceo : "Jeff Bezos",
employees: [
{firstName: "John", lastName: "Doe"},
{firstName: "Anna", lastName: "Smith"},
{firstName: "Peter", lastName: "Jones"}
]
};
And a data is stored in JSON format:
JSON Data
{
"name" : "Amazon",
"ceo" : "Jeff Bezos",
"employees":[
{"firstName":"John", "lastName":"Doe"},
{"firstName":"Anna", "lastName":"Smith"},
{"firstName":"Peter", "lastName":"Jones"}
]
}
Strong points of JSON:
- JSON is a Lightweight format for data exchange.
- The JSON data describes itself, therefore, is easy to understand for everyone.
- JSON is an independent language, and a text. You can use any language to read or create JSON data. Most programming languages have libraries to read and write JSON data.
Example:
// JSON Object:
{"firstName":"John", "lastName":"Doe"}
// JSON Array:
[
{"firstName":"John", "lastName":"Doe"},
{"firstName":"Anna", "lastName":"Smith"},
{"firstName":"Peter", "lastName":"Jones"}
]
JSON is purely a data format — it contains only properties, no methods.JSON requires double quotes to be used around strings and property names. Single quotes are not valid.Even a single misplaced comma or colon can cause a JSON file to go wrong, and not work. You should be careful to validate any data you are attempting to use.
2. JSON.parse(..)
Using the JSON.parse(text) method helps you parse a JSON data and convert it into an object.
json-parse-example.js
var text = '{ '
+ ' "name": "Amazon", '
+ ' "ceo" : "Jeff Bezos", '
+ ' "employees" : ['
+ ' { "firstName":"John" , "lastName":"Doe" },'
+ ' { "firstName":"Anna" , "lastName":"Smith" },'
+ ' { "firstName":"Peter" , "lastName":"Jones" } '
+ ' ] '
+ '}';
var obj = JSON.parse(text);
console.log(obj.employees);
console.log(obj.ceo); // Jeff Bezos
console.log(obj.employees[0].firstName); // John
console.log(obj.employees[1].firstName); // Anna
console.log(obj.employees[2].firstName); // Peter
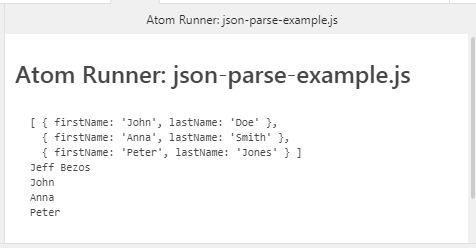
-
json-parse-example.html
<!DOCTYPE html>
<html>
<head>
<title>JSON.parse</title>
</head>
<body>
<div style="border:1px solid #ccc;padding:5px;">
<h3 id="name"></h3>
<i id="location"></i>
</div>
<script>
var s = '{"firstName" : "Sammy", "lastName" : "Shark", "location" : "Ocean"}';
var obj = JSON.parse(s);
document.getElementById("name").innerHTML = obj.firstName + " " + obj.lastName;
document.getElementById("location").innerHTML = obj.location;
</script>
</body>
</html>
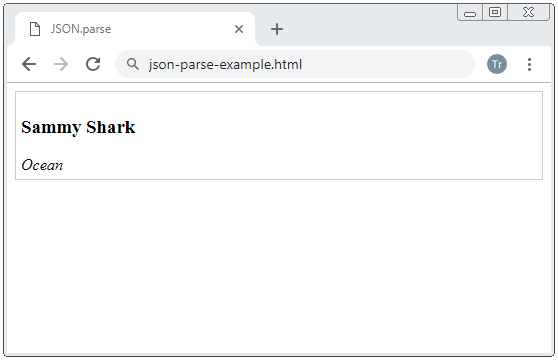
3. JSON.stringify(..)
Using the JSON.stringify(obj) method helps you convert a Javascript object into a text in JSON format.
json-stringify-example1.js
var obj = {name : "tran", age : 39};
var text = JSON.stringify(obj);
console.log(text); // {"name":"tran","age":39}
JSON.stringify(obj)
The JSON.stringify(obj) method is used to convert obj into a String. If the obj object has the toJSON() method, this method will be called to return String. On the contrary, it will be converted by default rules:
- Number, Boolean, String will be converted into primitive values.
- Infinity, NaN will be converted into null.
- If obj is undefined, Function, Symbol, it will be converted into undefined.
- If undefined, Function, Symbol appear in the object, they will be skipped.
- If undefined, Function, Symbol appear in array, they will be converted into null.
- All the properties that have the key such as Symbol will be skipped.
- The Date object has the toJSON() method, String is returned, like date.toISOString(), therefore, it is treated like a string.
json-stringify-example2.js
var obj = {
name : "tran",
age : 39,
// function in object will be ignored
sayHello : function() {
console.log("Hello");
},
// undefined, Symbol, function in Array will be converted to null.
"others": ["1", Symbol(), undefined, function(){}, "2"]
};
var text = JSON.stringify(obj);
console.log(text); // {"name":"tran","age":39,"others":["1",null,null,null,"2"]}
Example:
json-stringify-example.js
JSON.stringify({}); // '{}'
JSON.stringify(true); // 'true'
JSON.stringify('foo'); // '"foo"'
JSON.stringify([1, 'false', false]); // '[1,"false",false]'
JSON.stringify([NaN, null, Infinity]); // '[null,null,null]'
JSON.stringify({ x: 5 }); // '{"x":5}'
JSON.stringify(new Date(2006, 0, 2, 15, 4, 5)); // '"2006-01-02T15:04:05.000Z"'
JSON.stringify({ x: 5, y: 6 }); // '{"x":5,"y":6}'
JSON.stringify([new Number(3), new String('false'), new Boolean(false)]);
// '[3,"false",false]'
// String-keyed array elements are not enumerable and make no sense in JSON
let a = ['foo', 'bar'];
a['baz'] = 'quux'; // a: [ 0: 'foo', 1: 'bar', baz: 'quux' ]
JSON.stringify(a); // '["foo","bar"]'
JSON.stringify({ x: [10, undefined, function(){}, Symbol('')] });
// '{"x":[10,null,null,null]}'
// Standard data structures
JSON.stringify([new Set([1]), new Map([[1, 2]]), new WeakSet([{a: 1}]), new WeakMap([[{a: 1}, 2]])]);
// '[{},{},{},{}]'
// TypedArray
JSON.stringify([new Int8Array([1]), new Int16Array([1]), new Int32Array([1])]);
// '[{"0":1},{"0":1},{"0":1}]'
JSON.stringify([new Uint8Array([1]), new Uint8ClampedArray([1]), new Uint16Array([1]), new Uint32Array([1])]);
// '[{"0":1},{"0":1},{"0":1},{"0":1}]'
JSON.stringify([new Float32Array([1]), new Float64Array([1])]);
// '[{"0":1},{"0":1}]'
// toJSON()
JSON.stringify({ x: 5, y: 6, toJSON(){ return this.x + this.y; } }); // '11'
// Symbols:
JSON.stringify({ x: undefined, y: Object, z: Symbol('') }); // '{}'
JSON.stringify({ [Symbol('foo')]: 'foo' }); // '{}'
JSON.stringify({ [Symbol.for('foo')]: 'foo' }, [Symbol.for('foo')]); // '{}'
JSON.stringify({ [Symbol.for('foo')]: 'foo' }, function(k, v) {
if (typeof k === 'symbol') {
return 'a symbol';
}
});
// undefined
// Non-enumerable properties:
JSON.stringify( Object.create(null, { x: { value: 'x', enumerable: false }, y: { value: 'y', enumerable: true } }) );
// '{"y":"y"}'
JSON.stringify(obj [, replacer[, space]])
The JSON.stringify(..) method actually has 3 parameters of which, the second and third parameters are not mandatory.
Parameters:
obj
- An object will be converted to a JSON string.
replacer
- replacer can be a function to adjust behavior in converting objects into JSON strings.
- replacer can be an array of Strings. It helps to specify a whitelist of properties which will appear in the JSON string.
space
- space can be a string (if it is longer than 10 characters, it will be truncated to 10 characters). Used to insert to the JSON to help the JSON more beautiful and easier to see.
- space can be a number less than or equal to 10 (if it is greater than 10, it will be considered 10). Used to specify a string, consisting of 'space' -blank character. This string will be inserted into the JSON to make the JSON more beautiful and easier to see.
Examples:
Example with the replacer parameter which is an array of Strings:
json-stringify-replacer-example1.js
var obj = {
name: "Tom",
gender: "Male",
company:"Walt Disney",
friends: [
{name:"Jerry", gender:"Male"},
{name:"Donald", gender: "Male"}
]
};
var replacer = ["name", "friends"];
var json = JSON.stringify(obj, replacer);
console.log(json); // {"name":"Tom","friends":[{"name":"Jerry"},{"name":"Donald"}]}
Example with the replacer placer parameter which is a function:
json-stringify-replacer-example2.js
function replacer(key, value) {
console.log(typeof key);
console.log(key);
// Filtering out properties
if (typeof value === 'string') {
return undefined;
}
return value;
}
var obj = {
foundation: 'Mozilla',
model: 'box',
week: 45,
transport: 'car',
month: 7
};
var json = JSON.stringify(obj, replacer);
console.log(json); // '{"week":45,"month":7}'
Example with the replacer parameter which is a function and the obj parameter is an array.
json-stringify-replacer-example3.js
// If obj is an Array ==> @key: type of string (Index of array)
function replacer(key, value) {
console.log(key);
console.log(typeof key);
// Index
if (key === "0") {
return "@";
}
//
if (typeof value === 'string') {
return undefined;
}
return value;
}
// obj is an Array
var obj = ['Mozilla', 'box', 45, 'car', 7];
var json = JSON.stringify(obj, replacer);
console.log(json); // ["@",null,45,null,7]
Example with the space parameter:
json-stringify-space-example.js
var obj = {
b: 42,
c: "42",
d: [1, 2, 3]
};
console.log(JSON.stringify(obj, null, 3));
// "{
// "b": 42,
// "c": "42",
// "d": [
// 1,
// 2,
// 3
// ]
// }"
console.log(JSON.stringify(obj, null, "-----"));
// "{
// -----"b": 42,
// -----"c": "42",
// -----"d": [
// ----------1,
// ----------2,
// ----------3
// -----]
// }"
ECMAScript, Javascript Tutorials
- Introduction to Javascript and ECMAScript
- Quickstart with Javascript
- Alert, Confirm, Prompt Dialog Box in Javascript
- Quickstart with JavaScript
- JavaScript Variables Tutorial with Examples
- Bitwise Operations
- JavaScript Arrays Tutorial with Examples
- JavaScript Loops Tutorial with Examples
- JavaScript Functions Tutorial with Examples
- JavaScript Number Tutorial with Examples
- JavaScript Boolean Tutorial with Examples
- JavaScript Strings Tutorial with Examples
- JavaScript if else Statement Tutorial with Examples
- JavaScript Switch Statement
- JavaScript Error Handling Tutorial with Examples
- JavaScript Date Tutorial with Examples
- JavaScript Modules Tutorial with Examples
- The History of Modules in JavaScript
- JavaScript setTimeout and setInterval Function
- Javascript Form Validation Tutorial with Examples
- JavaScript Web Cookies Tutorial with Examples
- JavaScript void Keyword Tutorial with Examples
- Classes and Objects in JavaScript
- Class and inheritance simulation techniques in JavaScript
- Inheritance and polymorphism in JavaScript
- Undertanding Duck Typing in JavaScript
- JavaScript Symbols Tutorial with Examples
- JavaScript Set Collection Tutorial with Examples
- JavaScript Map Collection Tutorial with Examples
- Undertanding JavaScript Iterables and Iterators
- JavaScript Regular Expressions Tutorial with Examples
- JavaScript Promise, Async/Await Tutorial with Examples
- Javascript Window Tutorial with Examples
- Javascript Console Tutorial with Examples
- Javascript Screen Tutorial with Examples
- Javascript Navigator Tutorial with Examples
- Javascript Geolocation API Tutorial with Examples
- Javascript Location Tutorial with Examples
- Javascript History API Tutorial with Examples
- Javascript Statusbar Tutorial with Examples
- Javascript Locationbar Tutorial with Examples
- Javascript Scrollbars Tutorial with Examples
- Javascript Menubar Tutorial with Examples
- JavaScript JSON Tutorial with Examples
- JavaScript Event Handling Tutorial with Examples
- Javascript MouseEvent Tutorial with Examples
- Javascript WheelEvent Tutorial with Examples
- Javascript KeyboardEvent Tutorial with Examples
- Javascript FocusEvent Tutorial with Examples
- Javascript InputEvent Tutorial with Examples
- Javascript ChangeEvent Tutorial with Examples
- Javascript DragEvent Tutorial with Examples
- Javascript HashChangeEvent Tutorial with Examples
- Javascript URL Encoding Tutorial with Examples
- Javascript FileReader Tutorial with Examples
- Javascript XMLHttpRequest Tutorial with Examples
- Javascript Fetch API Tutorial with Examples
- Parsing XML in Javascript with DOMParser
- Introduction to Javascript HTML5 Canvas API
- Highlighting code with SyntaxHighlighter Javascript library
- What are polyfills in programming science?
Show More